Mastering the Least Common Multiple: A Comprehensive Guide for Coding Interviews
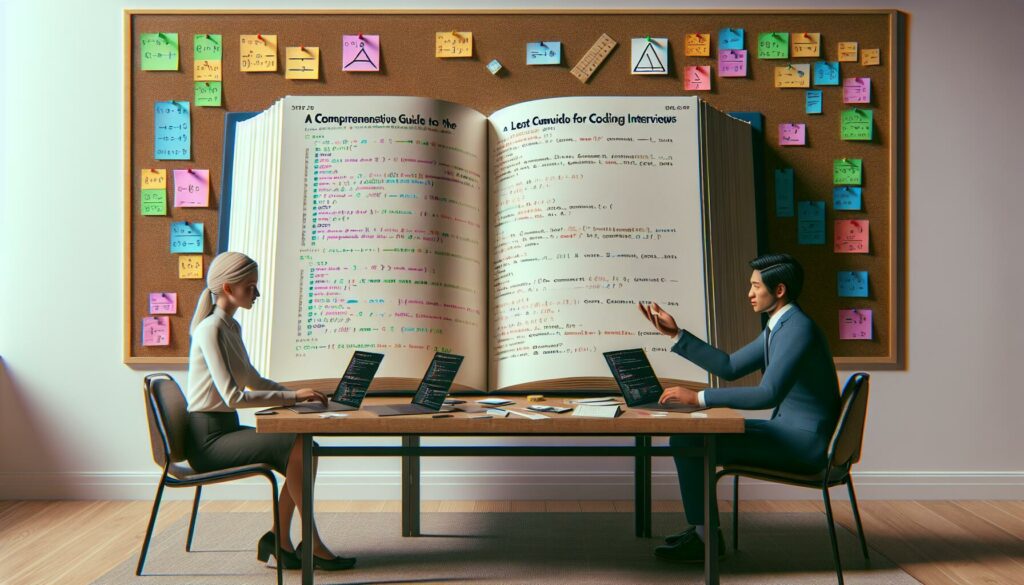
Welcome to AlgoCademy’s in-depth exploration of the Least Common Multiple (LCM), a fundamental concept in mathematics and computer science. Whether you’re preparing for a coding interview at a top tech company or simply looking to sharpen your problem-solving skills, understanding the LCM is crucial. In this comprehensive guide, we’ll dive deep into what the LCM is, why it’s important, and how to efficiently calculate it in various programming languages.
Table of Contents
- What is the Least Common Multiple?
- The Importance of LCM in Programming
- Methods for Calculating LCM
- LCM Algorithms and Implementations
- Real-world Applications of LCM
- Common LCM Interview Questions
- Optimizing LCM Calculations
- LCM Beyond the Basics
- Conclusion
1. What is the Least Common Multiple?
The Least Common Multiple (LCM) of two or more integers is the smallest positive integer that is divisible by all of them. In other words, it’s the smallest number that’s a multiple of each of the given numbers.
For example:
- The LCM of 4 and 6 is 12, because 12 is the smallest number divisible by both 4 and 6.
- The LCM of 3, 4, and 6 is 12, as it’s the smallest number divisible by all three.
Understanding the LCM is crucial for solving various mathematical and programming problems, especially those involving fractions, time intervals, or cyclic phenomena.
2. The Importance of LCM in Programming
In the world of coding and software development, the LCM plays a significant role in various applications:
- Scheduling and Time Management: LCM is used to determine cycle times in systems where multiple processes need to synchronize.
- Fraction Arithmetic: When adding or subtracting fractions with different denominators, finding the LCM of the denominators is a key step.
- Cryptography: Some encryption algorithms rely on properties of LCM for generating keys.
- Game Development: LCM can be useful in creating patterns or determining intervals for game events.
- Database Systems: In distributed systems, LCM can help in determining optimal intervals for data synchronization.
Given its wide-ranging applications, it’s no surprise that LCM-related questions often appear in coding interviews, especially for positions at major tech companies.
3. Methods for Calculating LCM
There are several methods to calculate the LCM. Let’s explore the most common ones:
3.1 Prime Factorization Method
This method involves breaking down the numbers into their prime factors and then multiplying the highest power of each prime factor.
Steps:
- Find the prime factorization of each number.
- Take each prime factor to the highest power in which it occurs in either number.
- Multiply these factors together.
Example: Find the LCM of 12 and 18
- 12 = 2² × 3
- 18 = 2 × 3²
- LCM = 2² × 3² = 4 × 9 = 36
3.2 Using the GCD (Greatest Common Divisor)
This method utilizes the relationship between LCM, GCD, and the product of the numbers:
LCM(a, b) = |a × b| / GCD(a, b)
This formula is particularly useful in programming as it’s easy to implement and efficient, especially when combined with the Euclidean algorithm for finding GCD.
3.3 Listing Multiples Method
While not efficient for large numbers, this method is intuitive and useful for understanding the concept:
- List the multiples of each number.
- Find the smallest number that appears in all lists.
Example: Find the LCM of 4 and 6
- Multiples of 4: 4, 8, 12, 16, 20, 24, …
- Multiples of 6: 6, 12, 18, 24, …
- The smallest common multiple is 12.
4. LCM Algorithms and Implementations
Now that we understand the concept and methods, let’s look at how to implement LCM calculations in various programming languages.
4.1 Python Implementation
Python provides a built-in function for GCD in the math
module, which we can use to calculate LCM efficiently:
import math
def lcm(a, b):
return abs(a * b) // math.gcd(a, b)
# Example usage
print(lcm(4, 6)) # Output: 12
print(lcm(15, 25)) # Output: 75
4.2 Java Implementation
In Java, we can implement the LCM function using a similar approach:
public class LCMCalculator {
public static int gcd(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
public static int lcm(int a, int b) {
return Math.abs(a * b) / gcd(a, b);
}
public static void main(String[] args) {
System.out.println(lcm(4, 6)); // Output: 12
System.out.println(lcm(15, 25)); // Output: 75
}
}
4.3 C++ Implementation
C++ also allows for a straightforward implementation of the LCM function:
#include <iostream>
#include <cstdlib>
int gcd(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
int lcm(int a, int b) {
return std::abs(a * b) / gcd(a, b);
}
int main() {
std::cout << lcm(4, 6) << std::endl; // Output: 12
std::cout << lcm(15, 25) << std::endl; // Output: 75
return 0;
}
5. Real-world Applications of LCM
Understanding the LCM isn’t just about solving abstract mathematical problems. It has numerous practical applications in various fields:
5.1 Computer Science and Programming
- Task Scheduling: In operating systems, LCM can be used to determine the schedule for periodic tasks with different frequencies.
- Memory Management: LCM helps in calculating efficient memory allocation sizes for different data types.
- Network Protocols: Some network protocols use LCM to synchronize packet sizes or transmission intervals.
5.2 Finance and Economics
- Investment Planning: LCM can help in determining investment cycles or when different investments will align.
- Loan Calculations: When dealing with loans with different payment frequencies, LCM can be used to find common payment dates.
5.3 Physics and Engineering
- Signal Processing: LCM is used in determining periods of combined signals.
- Gear Systems: In mechanical engineering, LCM helps in calculating gear ratios and determining when gear teeth will align.
5.4 Music Theory
- Rhythm Analysis: LCM can be used to analyze complex rhythmic patterns and determine when different rhythmic cycles will coincide.
6. Common LCM Interview Questions
When preparing for coding interviews, especially for positions at top tech companies, it’s crucial to be familiar with common LCM-related questions. Here are some examples you might encounter:
6.1 Basic LCM Calculation
Question: Implement a function to calculate the LCM of two positive integers.
Solution: We can use the GCD method we implemented earlier:
def lcm(a, b):
return abs(a * b) // math.gcd(a, b)
6.2 LCM of an Array
Question: Given an array of integers, find the LCM of all the elements in the array.
Solution: We can use the property that LCM(a,b,c) = LCM(a, LCM(b,c)):
from functools import reduce
def lcm_array(arr):
return reduce(lambda a, b: abs(a * b) // math.gcd(a, b), arr)
6.3 LCM in Problem Solving
Question: Two runners start at the same point and run in the same direction around a circular track. The first runner completes a lap every 3 minutes, and the second runner completes a lap every 5 minutes. After how many minutes will they meet again at the starting point?
Solution: This is a classic LCM problem. The runners will meet at the starting point after LCM(3, 5) = 15 minutes.
6.4 LCM and GCD Relationship
Question: Given two positive integers a and b, and knowing that LCM(a,b) × GCD(a,b) = a × b, implement a function to find GCD(a,b) if LCM(a,b) is given.
Solution:
def find_gcd(a, b, lcm):
return (a * b) // lcm
7. Optimizing LCM Calculations
While the basic LCM algorithm is efficient for most cases, there are scenarios where optimization becomes crucial, especially when dealing with very large numbers or when calculating LCM frequently.
7.1 Using Binary GCD (Stein’s Algorithm)
The Binary GCD algorithm, also known as Stein’s algorithm, can be more efficient than the standard Euclidean algorithm for GCD calculation, especially for large numbers:
def binary_gcd(a, b):
if a == 0:
return b
if b == 0:
return a
shift = 0
while ((a | b) & 1) == 0:
a >>= 1
b >>= 1
shift += 1
while (a & 1) == 0:
a >>= 1
while b != 0:
while (b & 1) == 0:
b >>= 1
if a > b:
a, b = b, a
b -= a
return a << shift
def optimized_lcm(a, b):
return abs(a * b) // binary_gcd(a, b)
7.2 Modular Arithmetic for Large Numbers
When dealing with very large numbers, we can use modular arithmetic to prevent overflow:
def mod_lcm(a, b, m):
return (a * b // binary_gcd(a, b)) % m
7.3 Memoization for Repeated Calculations
If you need to calculate LCM multiple times for the same set of numbers, consider using memoization:
from functools import lru_cache
@lru_cache(maxsize=None)
def memoized_lcm(a, b):
return abs(a * b) // math.gcd(a, b)
8. LCM Beyond the Basics
As you progress in your coding journey, you’ll encounter more advanced concepts related to LCM. Let’s explore some of these topics:
8.1 LCM in Number Theory
In number theory, LCM plays a crucial role in various theorems and properties:
- Divisibility Rules: LCM is closely related to divisibility properties of numbers.
- Diophantine Equations: LCM is often used in solving linear Diophantine equations.
- Euler’s Totient Function: There’s a relationship between LCM and Euler’s totient function in certain cases.
8.2 LCM in Cryptography
LCM is used in various cryptographic algorithms:
- RSA Algorithm: The LCM of p-1 and q-1 (where p and q are prime factors) is used in key generation.
- Diffie-Hellman Key Exchange: LCM properties are utilized in some variations of this protocol.
8.3 LCM in Combinatorics
LCM appears in various combinatorial problems:
- Cycle Lengths in Permutations: LCM is used to determine the length of cycles in permutation groups.
- Chinese Remainder Theorem: LCM is crucial in solving systems of linear congruences.
8.4 LCM in Competitive Programming
Advanced competitive programming problems often involve LCM in complex ways:
- Segment Tree with LCM: Using LCM in segment trees for range query problems.
- Dynamic Programming with LCM: Incorporating LCM in DP state transitions for optimization problems.
9. Conclusion
Mastering the Least Common Multiple is an essential skill for any programmer or computer scientist. From basic calculations to advanced applications in cryptography and number theory, LCM plays a crucial role in various aspects of computing and mathematics.
As you prepare for coding interviews, especially for positions at top tech companies, remember that understanding LCM goes beyond just knowing how to calculate it. It’s about recognizing when and how to apply it in problem-solving scenarios, optimizing its calculation for different contexts, and understanding its broader implications in computer science and mathematics.
At AlgoCademy, we believe in comprehensive learning that goes beyond surface-level understanding. By diving deep into concepts like LCM, you’re not just preparing for interviews – you’re building a solid foundation for a successful career in tech. Keep practicing, exploring, and pushing your boundaries. The world of algorithms and problem-solving is vast and exciting, and mastering fundamental concepts like LCM is your key to unlocking its potential.
Happy coding, and may your journey in mastering algorithms be as rewarding as finding the perfect LCM!