Mastering the JavaScript Filter Method: A Comprehensive Guide
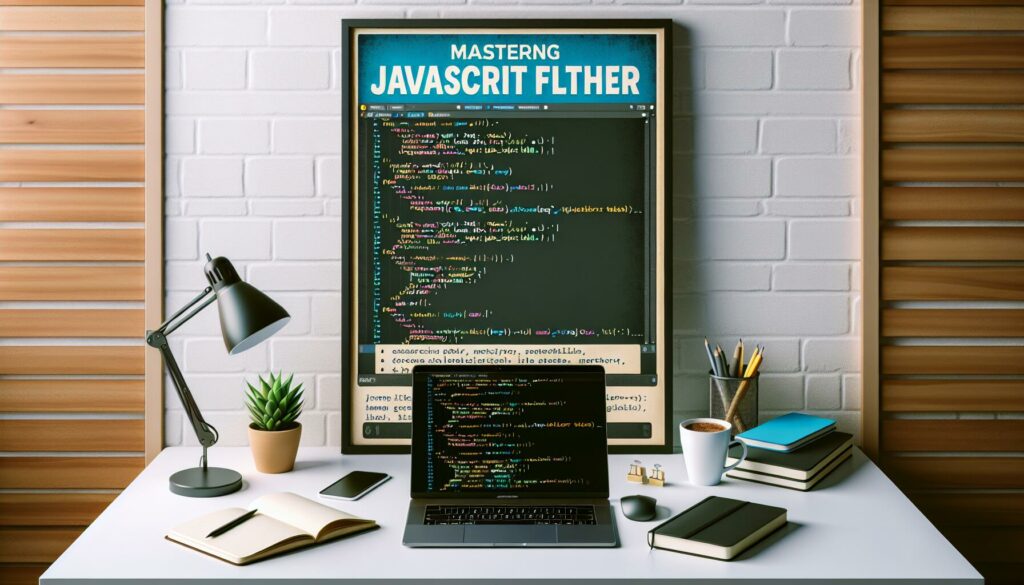
JavaScript is a versatile and powerful programming language, offering developers a wide array of built-in methods to manipulate data efficiently. One such method that stands out for its usefulness in array manipulation is the filter()
method. In this comprehensive guide, we’ll dive deep into the JavaScript filter method, exploring its syntax, use cases, and best practices to help you become proficient in using this invaluable tool.
What is the JavaScript Filter Method?
The filter()
method is a built-in array method in JavaScript that creates a new array containing all elements that pass a certain condition. It allows you to iterate through an array and selectively choose elements based on a specified criteria, without modifying the original array.
The filter method is part of the ECMAScript 5 (ES5) specification and is supported by all modern browsers, making it a reliable choice for array manipulation tasks.
Syntax of the Filter Method
The basic syntax of the filter method is as follows:
let newArray = array.filter(callback(element[, index[, array]])[, thisArg])
Let’s break down the components of this syntax:
newArray
: The new array that will contain all the elements that pass the test implemented by the callback function.array
: The original array on which the filter method is called.callback
: A function that tests each element of the array. It should return a boolean value –true
to keep the element,false
otherwise.element
: The current element being processed in the array.index
(optional): The index of the current element being processed in the array.array
(optional): The array on which the filter method was called.thisArg
(optional): A value to use asthis
when executing the callback function.
How the Filter Method Works
The filter method works by iterating through each element of the array and applying the callback function to each element. If the callback function returns true
for an element, that element is included in the new array. If it returns false
, the element is excluded.
Here’s a simple example to illustrate how the filter method works:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbers = numbers.filter(number => number % 2 === 0);
console.log(evenNumbers); // Output: [2, 4, 6, 8, 10]
In this example, we use the filter method to create a new array evenNumbers
containing only the even numbers from the original numbers
array.
Common Use Cases for the Filter Method
The filter method is incredibly versatile and can be used in various scenarios. Here are some common use cases:
1. Filtering Arrays Based on a Condition
One of the most straightforward uses of the filter method is to create a new array based on a specific condition. For example, filtering an array of objects based on a property value:
const products = [
{ name: 'Laptop', price: 1000 },
{ name: 'Phone', price: 500 },
{ name: 'Tablet', price: 300 },
{ name: 'Smartwatch', price: 200 }
];
const affordableProducts = products.filter(product => product.price
2. Removing Duplicate Values from an Array
The filter method can be used in conjunction with the indexOf()
method to remove duplicate values from an array:
const numbers = [1, 2, 2, 3, 4, 4, 5];
const uniqueNumbers = numbers.filter((number, index, array) =>
array.indexOf(number) === index
);
console.log(uniqueNumbers); // Output: [1, 2, 3, 4, 5]
3. Filtering Out Falsy Values
You can use the filter method to remove falsy values (such as null
, undefined
, 0
, false
, NaN
, and empty strings) from an array:
const mixedArray = [0, 1, false, 2, '', 3, null, undefined, NaN];
const truthyValues = mixedArray.filter(Boolean);
console.log(truthyValues); // Output: [1, 2, 3]
4. Implementing Search Functionality
The filter method is excellent for implementing simple search functionality in arrays of objects:
const books = [
{ title: 'To Kill a Mockingbird', author: 'Harper Lee' },
{ title: '1984', author: 'George Orwell' },
{ title: 'Pride and Prejudice', author: 'Jane Austen' }
];
function searchBooks(query) {
return books.filter(book =>
book.title.toLowerCase().includes(query.toLowerCase()) ||
book.author.toLowerCase().includes(query.toLowerCase())
);
}
console.log(searchBooks('or'));
// Output: [{ title: '1984', author: 'George Orwell' }]
Advanced Techniques with the Filter Method
While the basic usage of the filter method is straightforward, there are some advanced techniques that can make it even more powerful:
1. Chaining Filter with Other Array Methods
The filter method can be chained with other array methods like map()
, reduce()
, or even another filter()
for more complex data transformations:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const sumOfSquaresOfEvenNumbers = numbers
.filter(num => num % 2 === 0)
.map(num => num * num)
.reduce((sum, num) => sum + num, 0);
console.log(sumOfSquaresOfEvenNumbers); // Output: 220
2. Using Filter with Arrow Functions and Destructuring
Combining arrow functions with object destructuring can make your filter callbacks more concise and readable:
const people = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 },
{ name: 'David', age: 40 }
];
const youngPeople = people.filter(({ age }) => age
3. Implementing Complex Filtering Logic
For more complex filtering scenarios, you can define a separate function to handle the filtering logic:
const transactions = [
{ id: 1, amount: 100, type: 'debit', category: 'food' },
{ id: 2, amount: 200, type: 'credit', category: 'salary' },
{ id: 3, amount: 50, type: 'debit', category: 'transportation' },
{ id: 4, amount: 300, type: 'debit', category: 'rent' }
];
function filterTransactions(transactions, filters) {
return transactions.filter(transaction => {
for (let key in filters) {
if (transaction[key] !== filters[key]) {
return false;
}
}
return true;
});
}
const filteredTransactions = filterTransactions(transactions, { type: 'debit', amount: 100 });
console.log(filteredTransactions);
// Output: [{ id: 1, amount: 100, type: 'debit', category: 'food' }]
Performance Considerations
While the filter method is powerful and convenient, it’s important to consider performance, especially when working with large arrays or in performance-critical applications. Here are some tips to optimize your use of the filter method:
1. Avoid Unnecessary Filtering
If you only need to find the first occurrence of an element that matches a condition, consider using find()
instead of filter()
. The find()
method stops iterating as soon as it finds a match, which can be more efficient for large arrays.
2. Use Early Returns
In your callback function, try to return false
as early as possible for elements that don’t meet the criteria. This can help avoid unnecessary computations:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenNumbersGreaterThanFive = numbers.filter(num => {
if (num
3. Consider Using Set for Removing Duplicates
While the filter method can be used to remove duplicates, using a Set might be more efficient for large arrays:
const numbers = [1, 2, 2, 3, 4, 4, 5];
const uniqueNumbers = [...new Set(numbers)];
console.log(uniqueNumbers); // Output: [1, 2, 3, 4, 5]
Common Pitfalls and How to Avoid Them
While the filter method is relatively straightforward to use, there are some common mistakes that developers might encounter. Here are a few pitfalls to watch out for:
1. Modifying the Original Array
Remember that the filter method does not modify the original array. If you need to update the original array, you’ll need to reassign it:
let numbers = [1, 2, 3, 4, 5];
numbers = numbers.filter(num => num > 2);
console.log(numbers); // Output: [3, 4, 5]
2. Forgetting to Return a Boolean Value
Always ensure that your callback function returns a boolean value. If you forget to return a value, or return a non-boolean value, you might get unexpected results:
const numbers = [1, 2, 3, 4, 5];
// Incorrect
const evenNumbers = numbers.filter(num => {
if (num % 2 === 0) {
return num;
}
});
console.log(evenNumbers); // Output: [2, 4]
// Correct
const correctEvenNumbers = numbers.filter(num => num % 2 === 0);
console.log(correctEvenNumbers); // Output: [2, 4]
3. Using Filter When Map is More Appropriate
Sometimes developers use filter when they actually want to transform elements. In such cases, map()
is more appropriate:
const numbers = [1, 2, 3, 4, 5];
// Incorrect use of filter
const doubledNumbers = numbers.filter(num => num * 2);
console.log(doubledNumbers); // Output: [1, 2, 3, 4, 5]
// Correct use of map
const correctDoubledNumbers = numbers.map(num => num * 2);
console.log(correctDoubledNumbers); // Output: [2, 4, 6, 8, 10]
Browser Compatibility and Polyfills
The filter method is well-supported across modern browsers, including:
- Chrome 1+
- Firefox 1.5+
- Safari 3+
- Edge 12+
- Internet Explorer 9+
- Opera 9.5+
However, if you need to support older browsers, you might want to use a polyfill. Here’s a simple polyfill for the filter method:
if (!Array.prototype.filter) {
Array.prototype.filter = function(callback, thisArg) {
if (this == null) {
throw new TypeError('Array.prototype.filter called on null or undefined');
}
if (typeof callback !== 'function') {
throw new TypeError(callback + ' is not a function');
}
const array = Object(this);
const length = array.length >>> 0;
const result = [];
for (let i = 0; i
Conclusion
The JavaScript filter method is a powerful tool for array manipulation, allowing developers to create new arrays based on specific conditions. Its versatility makes it useful in a wide range of scenarios, from simple data filtering to implementing search functionality and beyond.
By mastering the filter method, along with other array methods like map and reduce, you can write more concise, readable, and efficient code. Remember to consider performance implications when working with large datasets, and always be mindful of common pitfalls to ensure your code behaves as expected.
As you continue to work with JavaScript, you’ll find that the filter method becomes an indispensable part of your toolkit, enabling you to handle complex data transformations with ease and elegance.