Mastering the Hardest Parts of Coding Interview Preparation: A Comprehensive Guide
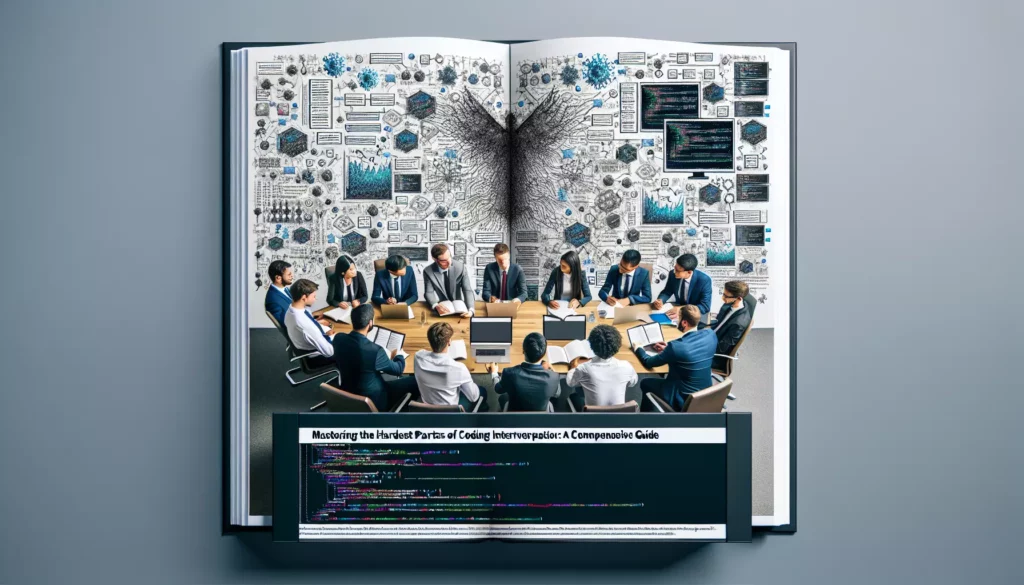
Preparing for coding interviews can be a daunting task, especially when aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). As aspiring developers navigate through this challenging process, they often find themselves wondering: what’s the hardest part about coding interview preparation? In this comprehensive guide, we’ll explore the most challenging aspects of interview prep and provide strategies to overcome them, helping you boost your confidence and increase your chances of success.
1. Developing a Consistent Study Routine
One of the most challenging aspects of coding interview preparation is establishing and maintaining a consistent study routine. With busy schedules and numerous distractions, it’s easy to procrastinate or lose focus. However, consistency is key to mastering the skills required for technical interviews.
Why it’s difficult:
- Balancing preparation with work or school commitments
- Overcoming burnout and maintaining motivation
- Finding the right pace to avoid overwhelming yourself
How to overcome it:
- Set realistic goals and create a structured study plan
- Use time management techniques like the Pomodoro method
- Join study groups or find an accountability partner
- Utilize platforms like AlgoCademy to track your progress and stay motivated
2. Mastering Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for success in coding interviews. However, this topic can be overwhelming due to its breadth and complexity.
Why it’s difficult:
- Wide range of concepts to learn and memorize
- Applying theoretical knowledge to practical problem-solving
- Understanding the time and space complexity of different algorithms
How to overcome it:
- Break down the learning process into smaller, manageable chunks
- Practice implementing data structures from scratch
- Solve problems that utilize various data structures and algorithms
- Analyze the time and space complexity of your solutions
- Use visualization tools to better understand how algorithms work
3. Improving Problem-Solving Skills
Technical interviews often focus on assessing a candidate’s problem-solving abilities. Developing these skills can be challenging, as it requires a combination of analytical thinking, creativity, and technical knowledge.
Why it’s difficult:
- Each problem is unique and may require a different approach
- Time pressure during interviews can affect problem-solving abilities
- Overcoming the initial overwhelm when faced with a complex problem
How to overcome it:
- Practice solving a wide variety of coding problems
- Learn and apply problem-solving frameworks like UMPIRE (Understand, Match, Plan, Implement, Review, Evaluate)
- Participate in coding competitions and hackathons
- Analyze and learn from others’ solutions to the same problems
- Use platforms like AlgoCademy that offer step-by-step guidance and AI-powered assistance
4. Handling Interview Anxiety and Pressure
Even the most well-prepared candidates can struggle with anxiety and pressure during coding interviews. The high-stakes nature of these interviews can lead to nervousness and affect performance.
Why it’s difficult:
- Fear of failure or making mistakes in front of the interviewer
- Time constraints adding pressure to solve problems quickly
- The unpredictable nature of interview questions
How to overcome it:
- Practice mock interviews with friends or mentors
- Use timed coding exercises to simulate interview conditions
- Learn and practice relaxation techniques like deep breathing
- Focus on the problem-solving process rather than the outcome
- Prepare a strategy for handling moments when you’re stuck
5. Staying Up-to-Date with Industry Trends
The tech industry is constantly evolving, and staying current with the latest trends, technologies, and best practices can be challenging but essential for interview success.
Why it’s difficult:
- Rapid pace of technological advancements
- Balancing depth and breadth of knowledge
- Distinguishing between lasting trends and temporary hype
How to overcome it:
- Follow reputable tech blogs, podcasts, and YouTube channels
- Participate in online communities and forums
- Attend tech meetups and conferences (virtual or in-person)
- Experiment with new technologies through personal projects
- Use platforms like AlgoCademy that regularly update their content to reflect industry trends
6. Optimizing Solutions for Efficiency
In coding interviews, it’s not enough to simply solve the problem. Candidates are often expected to provide efficient solutions that optimize both time and space complexity.
Why it’s difficult:
- Requires a deep understanding of algorithmic complexity
- Balancing readability and efficiency in code
- Identifying opportunities for optimization in existing solutions
How to overcome it:
- Study and practice common optimization techniques
- Analyze the time and space complexity of your solutions
- Learn to identify and apply appropriate data structures for efficiency
- Practice optimizing brute force solutions to more efficient algorithms
- Use tools and platforms that provide performance analysis of your code
7. Effective Communication During Interviews
Technical skills alone are not enough to ace a coding interview. Candidates must also effectively communicate their thought process, explain their approach, and collaborate with the interviewer.
Why it’s difficult:
- Articulating complex technical concepts clearly
- Balancing talking and coding during the interview
- Responding to interviewer feedback and hints
How to overcome it:
- Practice explaining your code and thought process out loud
- Participate in pair programming sessions
- Record yourself solving problems and review your communication
- Learn to ask clarifying questions and seek feedback
- Use platforms like AlgoCademy that offer interactive coding environments to practice communication
8. Balancing Breadth and Depth of Knowledge
Coding interviews can cover a wide range of topics, making it challenging to decide how much depth to pursue in each area while maintaining a broad understanding of various concepts.
Why it’s difficult:
- Limited time to prepare for numerous potential topics
- Uncertainty about which areas will be emphasized in the interview
- Avoiding the trap of superficial knowledge across many topics
How to overcome it:
- Prioritize core computer science fundamentals
- Research the company and role to focus on relevant topics
- Create a study plan that covers essential topics while allowing for deep dives
- Use spaced repetition techniques to retain information
- Leverage comprehensive learning platforms like AlgoCademy that offer both breadth and depth
9. Adapting to Different Interview Formats
Coding interviews can take various formats, including whiteboard coding, pair programming, take-home assignments, and online coding assessments. Adapting to these different formats can be challenging.
Why it’s difficult:
- Each format requires a different set of skills and preparation
- Unfamiliarity with certain formats can increase anxiety
- Balancing speed and accuracy in timed online assessments
How to overcome it:
- Research common interview formats for your target companies
- Practice coding without an IDE to prepare for whiteboard interviews
- Participate in mock interviews that simulate different formats
- Use online platforms that offer various types of coding challenges
- Develop strategies for time management in different interview scenarios
10. Handling Rejection and Learning from Failures
Facing rejection is an inevitable part of the job search process, but it can be particularly challenging in the context of coding interviews due to the intense preparation involved.
Why it’s difficult:
- Emotional impact of rejection after significant preparation
- Difficulty in obtaining detailed feedback from interviews
- Maintaining motivation to continue preparing after setbacks
How to overcome it:
- View rejections as learning opportunities rather than personal failures
- Seek feedback whenever possible and reflect on areas for improvement
- Maintain a growth mindset and focus on continuous learning
- Connect with others who have gone through similar experiences for support
- Use platforms like AlgoCademy that provide personalized feedback and improvement suggestions
Practical Coding Example: Optimizing a Solution
To illustrate the challenge of optimizing solutions, let’s consider a common coding interview problem: finding the two numbers in an array that add up to a target sum. We’ll start with a brute force solution and then optimize it for better efficiency.
Brute Force Solution:
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = two_sum_brute_force(nums, target)
print(result) # Output: [0, 1]
This brute force solution has a time complexity of O(n^2) because it uses nested loops to check all possible pairs of numbers. While it works, it’s not efficient for large input sizes.
Optimized Solution:
def two_sum_optimized(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = two_sum_optimized(nums, target)
print(result) # Output: [0, 1]
This optimized solution uses a hash table (dictionary in Python) to achieve a time complexity of O(n). It demonstrates the importance of choosing appropriate data structures and algorithms to improve efficiency.
Conclusion
Preparing for coding interviews is a challenging but rewarding process. By recognizing and addressing the hardest parts of interview preparation, you can significantly improve your chances of success. Remember that consistent practice, a structured approach to learning, and the right tools and resources are key to overcoming these challenges.
Platforms like AlgoCademy can be invaluable in your preparation journey, offering a comprehensive curriculum, interactive coding environments, and AI-powered assistance to help you tackle the hardest aspects of coding interview preparation. With dedication, the right strategies, and continuous learning, you can master the skills needed to excel in technical interviews and launch your career in the tech industry.
Keep in mind that the journey to becoming a skilled developer extends beyond just acing interviews. The problem-solving abilities, algorithmic thinking, and coding proficiency you develop during this process will serve you well throughout your career. Embrace the challenges, stay persistent, and remember that every obstacle overcome is a step towards your goal of becoming a world-class programmer.