Mastering the Gas Station Problem: A Comprehensive Guide for Coding Interviews
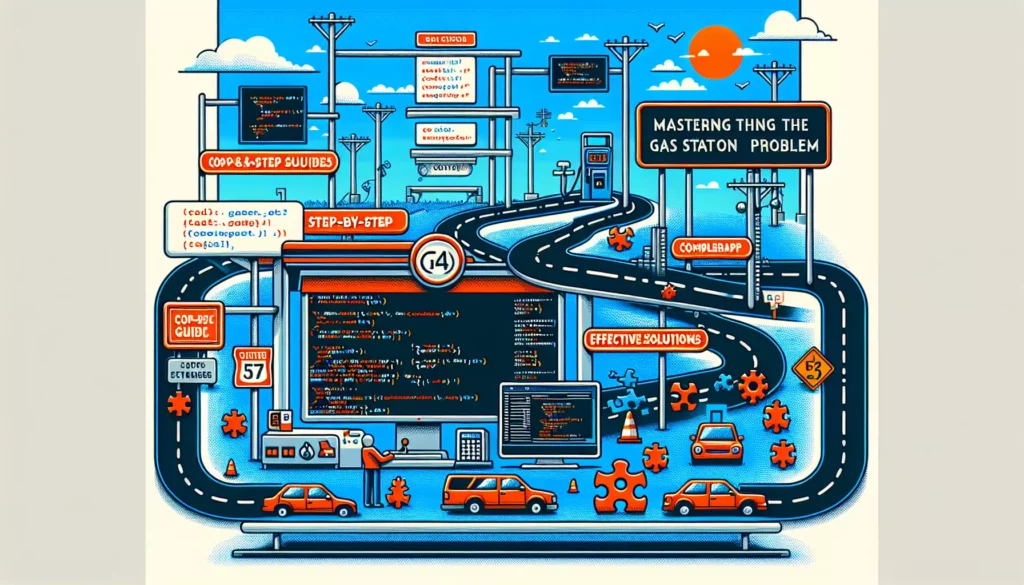
Welcome to AlgoCademy’s deep dive into one of the most intriguing algorithmic challenges you might encounter in your coding interviews: the Gas Station Problem. This problem is a favorite among tech giants like Google, Amazon, and Facebook, making it an essential part of your interview preparation toolkit. In this comprehensive guide, we’ll break down the problem, explore various approaches, and provide you with the skills to tackle this challenge with confidence.
What is the Gas Station Problem?
The Gas Station Problem, also known as the “Circular Tour” or “Gas Station Circuit” problem, is a classic algorithmic challenge that tests your ability to think critically about optimization and circular data structures. Here’s the typical problem statement:
There are N gas stations along a circular route, where the amount of gas at station i is gas[i]. You have a car with an unlimited gas tank and it costs cost[i] of gas to travel from station i to its next station (i+1). You begin the journey with an empty tank at one of the gas stations. Return the starting gas station’s index if you can travel around the circuit once in the clockwise direction, otherwise return -1.
This problem might seem straightforward at first glance, but it requires careful consideration of edge cases and efficient problem-solving techniques to arrive at an optimal solution.
Why is the Gas Station Problem Important?
Understanding and solving the Gas Station Problem is crucial for several reasons:
- Interview Frequency: This problem frequently appears in coding interviews at top tech companies, including FAANG (Facebook, Amazon, Apple, Netflix, Google).
- Algorithmic Thinking: It tests your ability to think algorithmically and optimize solutions.
- Real-world Applications: The concepts behind this problem have practical applications in resource allocation, route planning, and circular dependency resolution.
- Problem-solving Skills: Solving this problem demonstrates your capacity to break down complex issues and devise efficient solutions.
Breaking Down the Problem
Before we dive into solutions, let’s break down the key components of the Gas Station Problem:
- Circular Route: The gas stations are arranged in a circle, meaning that the last station connects back to the first.
- Gas Availability: Each station has a certain amount of gas available (gas[i]).
- Travel Cost: Moving from one station to the next has a cost associated with it (cost[i]).
- Starting Condition: The car starts with an empty tank.
- Goal: Find a starting point that allows a complete circuit, or determine that no such point exists.
Approaching the Solution
Now that we understand the problem, let’s explore different approaches to solving it, starting with the most intuitive and moving towards more optimized solutions.
1. Brute Force Approach
The brute force method is the most straightforward way to solve this problem. Here’s how it works:
- Start from each gas station.
- Attempt to make a complete circuit from each starting point.
- If a complete circuit is possible, return that starting index.
- If no complete circuit is possible from any starting point, return -1.
Here’s a Python implementation of the brute force approach:
def canCompleteCircuit(gas: List[int], cost: List[int]) -> int:
n = len(gas)
for start in range(n):
fuel = 0
possible = True
for i in range(n):
station = (start + i) % n
fuel += gas[station] - cost[station]
if fuel < 0:
possible = False
break
if possible:
return start
return -1
While this approach works, it has a time complexity of O(n^2), which is not efficient for large inputs. Let’s look at how we can optimize this solution.
2. One-pass Solution
A more efficient approach involves making a single pass through the array. The key insight here is that if a station cannot be reached from the starting point, then any station between the starting point and the unreachable station cannot be the answer.
Here’s how this optimized approach works:
- Initialize variables to keep track of total surplus, current surplus, and starting station.
- Iterate through all stations once.
- At each station, add the difference between gas and cost to both surpluses.
- If current surplus becomes negative, reset it and move the starting station to the next one.
- After the loop, if total surplus is non-negative, return the starting station; otherwise, return -1.
Let’s implement this in Python:
def canCompleteCircuit(gas: List[int], cost: List[int]) -> int:
n = len(gas)
total_surplus = 0
current_surplus = 0
start_station = 0
for i in range(n):
total_surplus += gas[i] - cost[i]
current_surplus += gas[i] - cost[i]
if current_surplus < 0:
start_station = i + 1
current_surplus = 0
return start_station if total_surplus >= 0 else -1
This solution has a time complexity of O(n) and space complexity of O(1), making it much more efficient than the brute force approach.
Understanding the One-pass Solution
The one-pass solution might seem a bit magical at first glance, so let’s break down why it works:
- Total Surplus: This keeps track of the overall gas surplus/deficit across all stations. If this is negative at the end, it means no solution exists.
- Current Surplus: This tracks the running surplus from the current starting station. If it goes negative, we know we can’t reach the next station from the current starting point.
- Start Station: This is updated whenever the current surplus goes negative, effectively eliminating all stations between the old start and the current station as potential starting points.
The key insight is that if you can’t reach station i from station j, you also can’t reach station i from any station between j and i. This allows us to eliminate multiple starting points in one go, achieving O(n) time complexity.
Edge Cases and Considerations
When implementing your solution, be sure to consider these edge cases:
- Empty Input: What if the gas and cost arrays are empty?
- Single Station: How does your solution handle a circuit with only one station?
- Equal Gas and Cost: What if the total gas exactly equals the total cost?
- Large Numbers: Can your solution handle very large numbers without overflow?
It’s crucial to discuss these considerations during an interview to demonstrate your thorough understanding of the problem.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our optimized one-pass solution:
- Time Complexity: O(n), where n is the number of gas stations. We make a single pass through the array.
- Space Complexity: O(1), as we only use a constant amount of extra space regardless of the input size.
This optimal solution significantly outperforms the brute force approach, especially for large inputs.
Real-world Applications
The Gas Station Problem isn’t just an academic exercise. Its concepts have several real-world applications:
- Resource Allocation: In systems where resources are distributed in a circular manner, this algorithm can help find optimal starting points for resource utilization.
- Route Planning: For vehicles with limited fuel capacity, this algorithm can help determine viable routes and refueling points.
- Network Flow: In computer networks, similar principles can be applied to optimize data flow in circular network topologies.
- Task Scheduling: In scenarios where tasks have dependencies and form a circular chain, this algorithm can help find a valid starting point for execution.
Common Interview Questions and Variations
During an interview, you might encounter variations or follow-up questions related to the Gas Station Problem. Here are some examples:
- Multiple Circuits: Can you modify the algorithm to find all possible starting points?
- Minimum Gas: What’s the minimum initial gas needed to complete the circuit from a given starting point?
- Optimization: If you can choose the order of stations, how would you maximize the number of stations visited with a fixed amount of initial gas?
- Dynamic Costs: How would you modify your solution if the costs between stations were not fixed but depended on the direction of travel?
Being prepared for these variations can help you stand out in an interview setting.
Tips for Solving the Gas Station Problem in Interviews
When tackling this problem in an interview setting, keep these tips in mind:
- Clarify the Problem: Make sure you understand all aspects of the problem. Don’t hesitate to ask questions.
- Think Aloud: Explain your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach.
- Start Simple: Begin with the brute force approach if you can’t immediately see the optimized solution. You can then work on optimizing it.
- Consider Edge Cases: Discuss potential edge cases and how your solution handles them.
- Analyze Complexity: Be prepared to discuss the time and space complexity of your solution.
- Test Your Code: If coding on a whiteboard or in a code editor, walk through your solution with a simple example to catch any logical errors.
Practice Problems
To further hone your skills, try these related problems:
Regular practice with these problems will help you become more comfortable with the concepts and variations of the Gas Station Problem.
Conclusion
The Gas Station Problem is a classic algorithmic challenge that tests your ability to think critically about optimization and circular data structures. By understanding the problem thoroughly, exploring different approaches, and practicing related problems, you’ll be well-prepared to tackle this challenge in your next coding interview.
Remember, the key to mastering algorithmic problems like this is consistent practice and a deep understanding of the underlying principles. Keep refining your problem-solving skills, and you’ll be well on your way to acing your technical interviews at top tech companies.
Happy coding, and best of luck with your interview preparation!