Mastering the Fibonacci Sequence: A Comprehensive Guide for Programmers
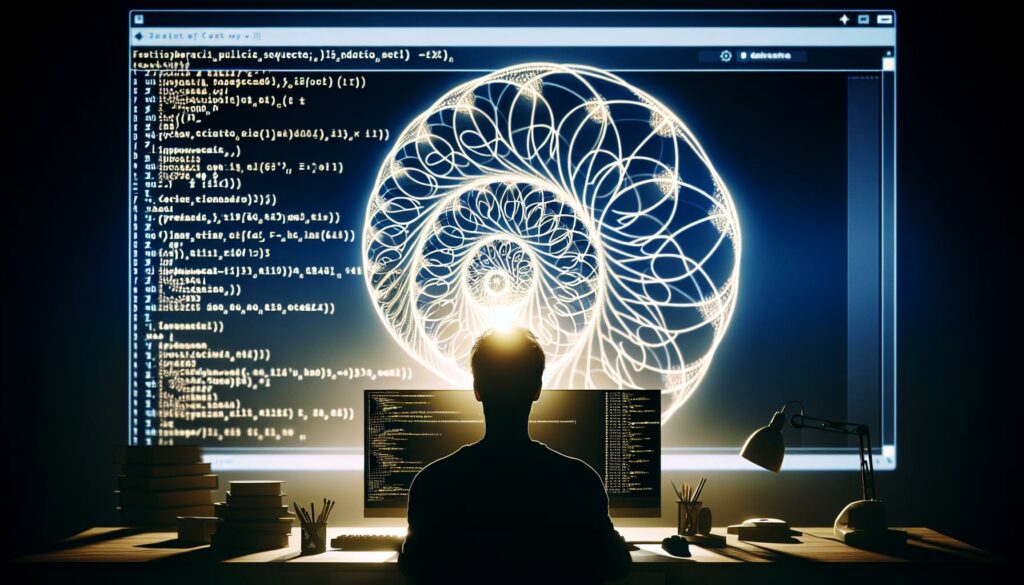
The Fibonacci sequence is a fundamental concept in mathematics and computer science, often serving as a gateway to understanding recursive algorithms and dynamic programming. In this comprehensive guide, we’ll explore the Fibonacci sequence in depth, covering its mathematical properties, implementation techniques, and practical applications in programming. Whether you’re a beginner looking to grasp the basics or an experienced developer preparing for technical interviews, this article will provide valuable insights and coding examples to enhance your understanding of this fascinating sequence.
Table of Contents
- What is the Fibonacci Sequence?
- Mathematical Properties of the Fibonacci Sequence
- Implementing Fibonacci in Various Programming Languages
- The Recursive Approach
- The Iterative Approach
- Dynamic Programming and Memoization
- Matrix Exponentiation for Faster Computation
- Time and Space Complexity Analysis
- Real-world Applications of the Fibonacci Sequence
- Common Interview Questions and Solutions
- Advanced Topics and Variations
- Conclusion
1. What is the Fibonacci Sequence?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. It typically starts with 0 and 1, although some variations begin with 1 and 1. The sequence continues indefinitely, creating a pattern that appears frequently in nature and has numerous applications in mathematics and computer science.
The first few numbers in the Fibonacci sequence are:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, ...
Mathematically, the Fibonacci sequence can be defined as follows:
F(n) = F(n-1) + F(n-2)
where F(0) = 0 and F(1) = 1
This recursive definition forms the basis for many of the algorithms we’ll explore in this article.
2. Mathematical Properties of the Fibonacci Sequence
The Fibonacci sequence possesses several intriguing mathematical properties that make it a subject of fascination for mathematicians and computer scientists alike:
- Golden Ratio: The ratio of consecutive Fibonacci numbers converges to the golden ratio (φ ≈ 1.618033988749895) as the sequence progresses.
- Binet’s Formula: This formula allows for direct calculation of the nth Fibonacci number without computing previous terms:
F(n) = (φ^n - (-φ)^-n) / √5 where φ = (1 + √5) / 2
- Divisibility Properties: Every 3rd Fibonacci number is divisible by 2, every 4th by 3, every 5th by 5, and so on.
- Sum of Squares: The sum of the squares of any two consecutive Fibonacci numbers is equal to the Fibonacci number that is one further in the sequence.
- Fibonacci Identities: There are numerous identities involving Fibonacci numbers, such as F(n+m) = F(n-1)F(m) + F(n)F(m+1).
Understanding these properties can provide insights into the behavior of Fibonacci-based algorithms and can sometimes lead to more efficient implementations.
3. Implementing Fibonacci in Various Programming Languages
Let’s explore how to implement the Fibonacci sequence in some popular programming languages. We’ll start with simple implementations and gradually move to more optimized versions.
Python Implementation
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Test the function
for i in range(10):
print(fibonacci(i), end=' ')
# Output: 0 1 1 2 3 5 8 13 21 34
JavaScript Implementation
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n-1) + fibonacci(n-2);
}
// Test the function
for (let i = 0; i < 10; i++) {
console.log(fibonacci(i));
}
// Output: 0 1 1 2 3 5 8 13 21 34
Java Implementation
public class Fibonacci {
static int fibonacci(int n) {
if (n <= 1)
return n;
return fibonacci(n-1) + fibonacci(n-2);
}
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
System.out.print(fibonacci(i) + " ");
}
}
}
// Output: 0 1 1 2 3 5 8 13 21 34
These simple recursive implementations are straightforward but inefficient for large values of n due to redundant calculations. In the following sections, we’ll explore more efficient approaches.
4. The Recursive Approach
The recursive approach to calculating Fibonacci numbers is intuitive and closely mirrors the mathematical definition of the sequence. However, it has significant drawbacks in terms of performance.
Advantages of the Recursive Approach:
- Simple and easy to understand
- Directly translates the mathematical definition into code
- Useful for teaching recursion concepts
Disadvantages of the Recursive Approach:
- Exponential time complexity O(2^n)
- Redundant calculations of the same Fibonacci numbers
- Can lead to stack overflow for large n due to deep recursion
Here’s a visualization of the recursive calls for calculating F(5):
F(5)
/ \
F(4) F(3)
/ \ / \
F(3) F(2) F(2) F(1)
/ \ / \ / \
F(2) F(1) F(1) F(0) F(1) F(0)
/ \
F(1) F(0)
As you can see, there are multiple redundant calculations of F(3), F(2), F(1), and F(0). This redundancy leads to the exponential time complexity of the naive recursive approach.
5. The Iterative Approach
The iterative approach addresses the inefficiencies of the recursive method by calculating Fibonacci numbers in a bottom-up manner, storing only the two most recent numbers in the sequence.
Python Implementation of Iterative Fibonacci
def fibonacci_iterative(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
# Test the function
for i in range(10):
print(fibonacci_iterative(i), end=' ')
# Output: 0 1 1 2 3 5 8 13 21 34
Advantages of the Iterative Approach:
- Linear time complexity O(n)
- Constant space complexity O(1)
- Avoids stack overflow issues
- More efficient for larger values of n
Disadvantages of the Iterative Approach:
- Less intuitive than the recursive approach
- Doesn’t directly reflect the mathematical definition
The iterative approach is generally preferred in practice due to its efficiency and ability to handle larger inputs without running into stack overflow issues.
6. Dynamic Programming and Memoization
Dynamic programming is a powerful technique that can be applied to the Fibonacci sequence to achieve efficient computation while maintaining the recursive structure. There are two main approaches to dynamic programming: top-down (memoization) and bottom-up (tabulation).
Top-down Approach (Memoization)
Memoization involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This approach maintains the recursive structure while eliminating redundant calculations.
def fibonacci_memoized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memoized(n-1, memo) + fibonacci_memoized(n-2, memo)
return memo[n]
# Test the function
for i in range(10):
print(fibonacci_memoized(i), end=' ')
# Output: 0 1 1 2 3 5 8 13 21 34
Bottom-up Approach (Tabulation)
The bottom-up approach builds a table of results for all subproblems, starting from the smallest and working up to the desired result.
def fibonacci_tabulation(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
for i in range(10):
print(fibonacci_tabulation(i), end=' ')
# Output: 0 1 1 2 3 5 8 13 21 34
Both memoization and tabulation approaches have a time complexity of O(n) and space complexity of O(n). They are significantly more efficient than the naive recursive approach, especially for larger values of n.
7. Matrix Exponentiation for Faster Computation
For very large values of n, even the O(n) solutions may not be fast enough. In such cases, we can use matrix exponentiation to compute Fibonacci numbers in O(log n) time.
The key insight is that we can represent the Fibonacci recurrence relation as a matrix equation:
[F(n+1)] [1 1] [F(n) ]
[F(n) ] = [1 0] [F(n-1)]
By raising this matrix to the power of n-1, we can compute F(n) efficiently:
def fibonacci_matrix(n):
def matrix_multiply(a, b):
return [
[a[0][0]*b[0][0] + a[0][1]*b[1][0], a[0][0]*b[0][1] + a[0][1]*b[1][1]],
[a[1][0]*b[0][0] + a[1][1]*b[1][0], a[1][0]*b[0][1] + a[1][1]*b[1][1]]
]
def matrix_power(matrix, n):
if n == 1:
return matrix
if n % 2 == 0:
half = matrix_power(matrix, n // 2)
return matrix_multiply(half, half)
return matrix_multiply(matrix, matrix_power(matrix, n - 1))
if n <= 1:
return n
result = matrix_power([[1, 1], [1, 0]], n - 1)
return result[0][0]
# Test the function
for i in range(10):
print(fibonacci_matrix(i), end=' ')
# Output: 0 1 1 2 3 5 8 13 21 34
This matrix exponentiation approach has a time complexity of O(log n) and is particularly useful for computing very large Fibonacci numbers or when working with modular arithmetic.
8. Time and Space Complexity Analysis
Let’s summarize the time and space complexities of the different approaches we’ve discussed:
Approach | Time Complexity | Space Complexity |
---|---|---|
Naive Recursive | O(2^n) | O(n) (call stack) |
Iterative | O(n) | O(1) |
Memoization | O(n) | O(n) |
Tabulation | O(n) | O(n) |
Matrix Exponentiation | O(log n) | O(1) |
The choice of approach depends on the specific requirements of your problem, such as the size of n, memory constraints, and whether you need to compute multiple Fibonacci numbers or just one.
9. Real-world Applications of the Fibonacci Sequence
The Fibonacci sequence has numerous applications beyond just being an interesting mathematical curiosity:
- Nature and Biology: The sequence appears in the arrangement of leaves on some plants, the spiral of shells, and the branching of trees.
- Financial Markets: Fibonacci retracements are used in technical analysis to identify potential reversal levels.
- Computer Algorithms: The sequence is often used in the analysis of algorithms, particularly when studying divide-and-conquer strategies.
- Music and Art: Some composers and artists have used the Fibonacci sequence to guide their creative processes.
- Network Planning: Fibonacci heap data structures are used in some network optimization algorithms.
- Pseudorandom Number Generation: The sequence can be used as part of algorithms for generating pseudorandom numbers.
10. Common Interview Questions and Solutions
Fibonacci-related problems are popular in coding interviews. Here are some common questions you might encounter:
1. Find the nth Fibonacci number
This is the basic problem we’ve been discussing throughout this article. The most efficient solution for large n is the matrix exponentiation approach.
2. Check if a number is a Fibonacci number
A number is Fibonacci if and only if one or both of (5*n^2 + 4) or (5*n^2 – 4) is a perfect square.
def is_fibonacci(n):
return is_perfect_square(5*n*n + 4) or is_perfect_square(5*n*n - 4)
def is_perfect_square(n):
sqrt = int(n**0.5)
return sqrt*sqrt == n
# Test the function
for i in range(10):
print(f"{i}: {is_fibonacci(i)}")
3. Generate Fibonacci numbers up to n
This can be efficiently done using the iterative approach:
def fibonacci_up_to_n(n):
fib = [0, 1]
while fib[-1] < n:
fib.append(fib[-1] + fib[-2])
return fib[:-1] # Remove the last element if it exceeds n
# Test the function
print(fibonacci_up_to_n(100))
# Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
4. Find the sum of even-valued Fibonacci numbers up to n
This problem is inspired by Project Euler Problem 2:
def sum_even_fibonacci(n):
a, b = 1, 2
total = 0
while b <= n:
if b % 2 == 0:
total += b
a, b = b, a + b
return total
# Test the function
print(sum_even_fibonacci(4000000))
# Output: 4613732
11. Advanced Topics and Variations
For those looking to delve deeper into the world of Fibonacci numbers, here are some advanced topics and variations to explore:
Fibonacci Coding
Fibonacci coding is a universal code which encodes positive integers into binary code words. It’s based on Fibonacci numbers and has applications in data compression.
Negative Fibonacci Numbers
The Fibonacci sequence can be extended to negative indices, resulting in a bi-directional infinite sequence.
Fibonacci Primes
Fibonacci primes are Fibonacci numbers that are also prime numbers. The study of Fibonacci primes involves interesting open problems in number theory.
Fibonacci Heap
A Fibonacci heap is a data structure for priority queue operations, consisting of a collection of trees. It has good amortized running time for many operations.
Generalizations of Fibonacci Numbers
There are many sequences that generalize or are similar to the Fibonacci sequence, such as:
- Lucas numbers
- Tribonacci numbers
- Padovan sequence
- Fibonacci polynomials
12. Conclusion
The Fibonacci sequence is a fundamental concept in computer science and mathematics, offering a rich playground for exploring algorithmic techniques, performance optimization, and mathematical properties. From its simple recursive definition to advanced topics like matrix exponentiation and its applications in nature and technology, the Fibonacci sequence continues to fascinate and challenge programmers and mathematicians alike.
As you prepare for coding interviews or work on algorithm design, remember that understanding the Fibonacci sequence and its various implementation techniques can provide valuable insights into recursive thinking, dynamic programming, and algorithm optimization. The skills you develop while working with Fibonacci problems are transferable to many other areas of computer science and software development.
Keep practicing, exploring different approaches, and challenging yourself with increasingly complex problems related to the Fibonacci sequence. With time and dedication, you’ll not only master this specific concept but also enhance your overall problem-solving and coding skills, setting yourself up for success in technical interviews and real-world programming challenges.