Mastering the Coin Change Problem: A Comprehensive Guide for Coding Interviews
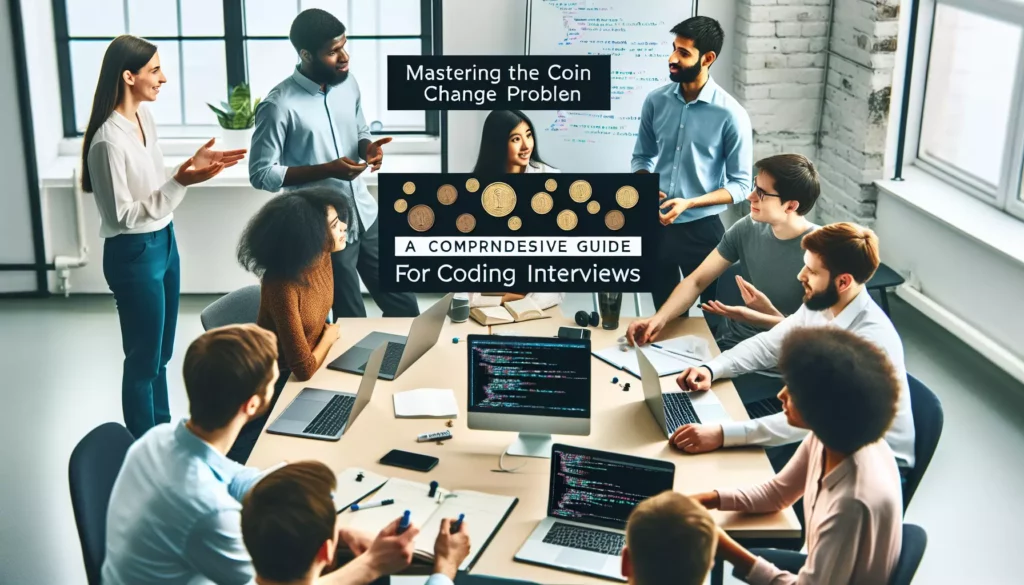
Welcome to another in-depth tutorial from AlgoCademy! Today, we’re diving into a classic algorithmic challenge that frequently appears in coding interviews, especially those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). Our focus? The Coin Change Problem.
Whether you’re a beginner looking to enhance your problem-solving skills or an experienced developer preparing for technical interviews, understanding the Coin Change Problem and its solutions is crucial. This problem not only tests your ability to think algorithmically but also challenges you to optimize your solution for efficiency.
What is the Coin Change Problem?
The Coin Change Problem is a classic dynamic programming problem that asks: Given a set of coin denominations and a target amount, what is the minimum number of coins needed to make up that amount? If it’s not possible to make the amount with the given coins, the problem typically asks to return -1 or some indicator of impossibility.
For example, if you have coins of denominations [1, 2, 5] and you need to make an amount of 11, the minimum number of coins needed would be 3 (5 + 5 + 1).
Why is the Coin Change Problem Important?
The Coin Change Problem is significant for several reasons:
- Real-world applications: It has practical applications in various fields, including financial systems, vending machines, and currency exchange algorithms.
- Algorithmic thinking: It’s an excellent exercise in dynamic programming, teaching you how to break down complex problems into smaller, manageable subproblems.
- Interview favorite: It’s a popular question in coding interviews, especially at top tech companies, due to its ability to test both problem-solving skills and coding proficiency.
- Optimization challenge: It provides an opportunity to showcase your ability to optimize algorithms, as there are multiple ways to solve this problem with varying time and space complexities.
Understanding the Problem
Before we dive into solutions, let’s break down the problem statement:
- You are given an array of integers representing coin denominations.
- You are also given a target amount.
- Your task is to find the minimum number of coins needed to make up that amount.
- You can use each coin denomination as many times as you want.
- If it’s impossible to make the amount with the given coins, you should return -1 (or a similar indicator).
Approaching the Solution
There are multiple ways to approach the Coin Change Problem, each with its own trade-offs in terms of time and space complexity. We’ll explore three main approaches:
- Recursive Solution (Brute Force)
- Dynamic Programming (Bottom-Up)
- Dynamic Programming with Space Optimization
Let’s dive into each of these approaches, understand their implementation, and analyze their complexities.
1. Recursive Solution (Brute Force)
The recursive approach is the most intuitive way to solve the Coin Change Problem. It follows the natural thought process of trying each coin denomination and recursively solving for the remaining amount.
Algorithm:
- If the target amount is 0, return 0 (we need 0 coins to make 0).
- If the target amount is negative, return -1 (it’s impossible to make a negative amount).
- Initialize a variable ‘min_coins’ to infinity.
- For each coin denomination:
- Recursively call the function with (amount – coin).
- If the result is not -1 (i.e., it’s possible to make the remaining amount), update ‘min_coins’ if needed.
- If ‘min_coins’ is still infinity, return -1 (it was impossible to make the amount).
- Otherwise, return ‘min_coins + 1’ (adding 1 for the coin we used in this step).
Implementation:
def coin_change_recursive(coins, amount):
if amount == 0:
return 0
if amount < 0:
return -1
min_coins = float('inf')
for coin in coins:
result = coin_change_recursive(coins, amount - coin)
if result != -1:
min_coins = min(min_coins, result + 1)
return min_coins if min_coins != float('inf') else -1
# Example usage
coins = [1, 2, 5]
amount = 11
print(coin_change_recursive(coins, amount)) # Output: 3
Time and Space Complexity:
Time Complexity: O(c^n), where c is the number of coin denominations and n is the amount. This is because for each amount, we have c choices, and the depth of the recursion tree can go up to n.
Space Complexity: O(n) due to the recursion stack. In the worst case, the depth of the recursion can be equal to the amount.
While this recursive solution is intuitive, it’s highly inefficient for large inputs due to its exponential time complexity. It will likely exceed the time limit for most interview or online judge scenarios.
2. Dynamic Programming (Bottom-Up)
The dynamic programming approach significantly improves the time complexity by storing and reusing the results of subproblems. We’ll use a bottom-up approach, building our solution from smaller amounts to the target amount.
Algorithm:
- Create a DP array of size amount + 1, initialized with infinity (or a large number).
- Set dp[0] = 0 (it takes 0 coins to make an amount of 0).
- For each amount from 1 to the target amount:
- For each coin denomination:
- If the coin value is less than or equal to the current amount:
- Update dp[amount] with the minimum of its current value and dp[amount – coin] + 1.
- If the coin value is less than or equal to the current amount:
- For each coin denomination:
- If dp[amount] is still infinity, return -1 (it’s impossible to make the amount).
- Otherwise, return dp[amount].
Implementation:
def coin_change_dp(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for i in range(1, amount + 1):
for coin in coins:
if coin <= i:
dp[i] = min(dp[i], dp[i - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
# Example usage
coins = [1, 2, 5]
amount = 11
print(coin_change_dp(coins, amount)) # Output: 3
Time and Space Complexity:
Time Complexity: O(amount * len(coins)). We iterate through each amount from 1 to the target amount, and for each amount, we consider all coin denominations.
Space Complexity: O(amount) for the DP array.
This dynamic programming solution is much more efficient than the recursive approach and is typically fast enough for most practical scenarios and coding interviews.
3. Dynamic Programming with Space Optimization
While the previous DP solution is efficient in terms of time complexity, we can optimize its space usage. Instead of using an array of size amount + 1, we can use just two variables to keep track of the current and previous states.
Algorithm:
- Initialize two variables: ‘prev’ (set to 0) and ‘curr’ (set to infinity).
- For each amount from 1 to the target amount:
- Reset ‘curr’ to infinity.
- For each coin denomination:
- If the coin value is less than or equal to the current amount:
- Update ‘curr’ with the minimum of its current value and prev + 1.
- If the coin value is less than or equal to the current amount:
- Update ‘prev’ with the value of ‘curr’.
- If ‘curr’ is still infinity, return -1 (it’s impossible to make the amount).
- Otherwise, return ‘curr’.
Implementation:
def coin_change_dp_optimized(coins, amount):
prev, curr = 0, float('inf')
for i in range(1, amount + 1):
curr = float('inf')
for coin in coins:
if coin <= i:
curr = min(curr, prev + 1)
prev = curr
return curr if curr != float('inf') else -1
# Example usage
coins = [1, 2, 5]
amount = 11
print(coin_change_dp_optimized(coins, amount)) # Output: 3
Time and Space Complexity:
Time Complexity: O(amount * len(coins)), same as the previous DP solution.
Space Complexity: O(1), as we only use two variables regardless of the input size.
This space-optimized version maintains the time efficiency of the DP solution while significantly reducing the space usage, making it an excellent choice for scenarios where memory is a constraint.
Comparing the Approaches
Let’s compare our three approaches:
Approach | Time Complexity | Space Complexity | Pros | Cons |
---|---|---|---|---|
Recursive (Brute Force) | O(c^n) | O(n) | Intuitive, easy to implement | Extremely slow for large inputs, may cause stack overflow |
Dynamic Programming | O(amount * len(coins)) | O(amount) | Efficient time complexity, suitable for most scenarios | Uses extra space proportional to the amount |
DP with Space Optimization | O(amount * len(coins)) | O(1) | Best balance of time and space efficiency | Slightly more complex implementation |
Interview Tips
When tackling the Coin Change Problem in an interview setting, keep these tips in mind:
- Start with the brute force: Begin by explaining the recursive approach. This shows you understand the problem and can think through a solution step-by-step.
- Identify inefficiencies: Point out the limitations of the recursive approach, such as repeated calculations and potential stack overflow.
- Propose optimization: Suggest using dynamic programming to overcome these limitations. Explain how you can store and reuse results of subproblems.
- Implement the DP solution: Code the bottom-up DP solution, explaining your thought process as you go.
- Analyze complexity: Discuss the time and space complexity of your solution.
- Further optimization: If time allows, mention or implement the space-optimized version. This showcases your ability to think beyond the standard solution.
- Test your code: Use example inputs to verify your solution, including edge cases like amount = 0 or an impossible amount.
- Discuss real-world applications: If asked, be prepared to talk about where this problem might be applicable in real-world scenarios.
Common Variations and Follow-up Questions
Interviewers often like to twist the problem slightly or ask follow-up questions. Here are some common variations you might encounter:
- Coin Change 2 (Number of Ways): Instead of finding the minimum number of coins, find the total number of ways to make up the amount.
- Limited Supply of Coins: What if each coin denomination has a limited supply?
- Print the Coins Used: Modify the algorithm to not only return the minimum number of coins but also print which coins were used.
- Maximize the Number of Coins: Find the maximum number of coins needed to make up the amount (using the smallest denominations as much as possible).
- Custom Denomination Set: How would your solution change if the coin denominations weren’t standard and could be any positive integers?
Conclusion
The Coin Change Problem is a fantastic example of how dynamic programming can dramatically improve the efficiency of an algorithm. By understanding this problem and its solutions, you’re not just preparing for a potential interview question; you’re developing problem-solving skills that are applicable to a wide range of algorithmic challenges.
Remember, the key to mastering such problems is practice. Try implementing these solutions yourself, experiment with different inputs, and challenge yourself with the variations we discussed. As you become more comfortable with the Coin Change Problem, you’ll find that the principles you’ve learned here can be applied to many other dynamic programming challenges.
Keep coding, keep learning, and don’t hesitate to dive deep into complex problems. They’re not just obstacles; they’re opportunities to grow as a programmer and problem solver. Happy coding!