Mastering the Coding Interview: Sliding Window Technique
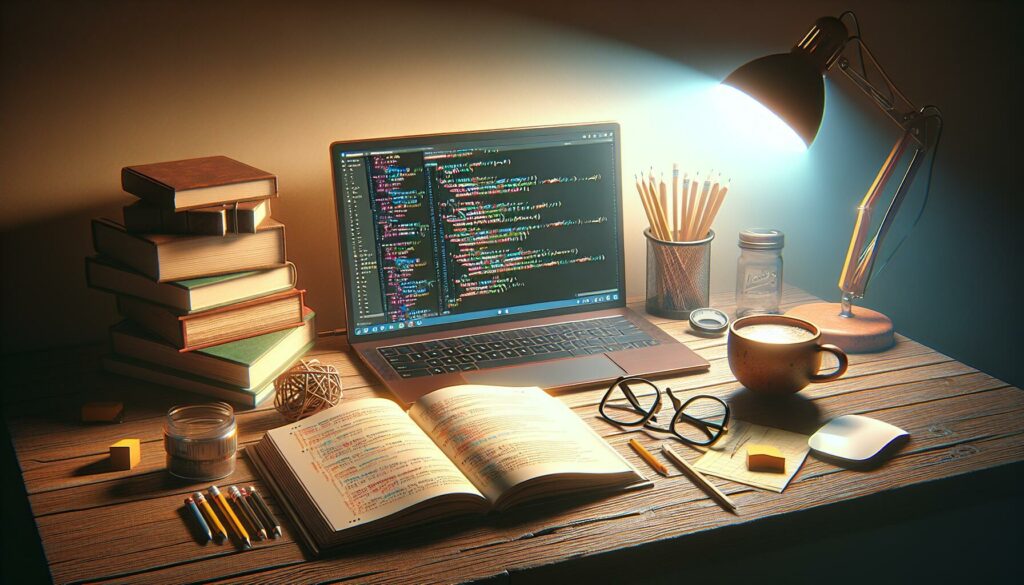
In the competitive world of software engineering, coding interviews remain a crucial step in landing your dream job. These interviews often involve solving algorithmic problems that test your problem-solving skills, coding ability, and understanding of fundamental computer science concepts. Today, we’re going to dive deep into a common interview problem and explore an elegant solution using the sliding window technique.
The Problem: Finding a Contiguous Subarray
Let’s start with the problem statement:
Given a list of non-negative numbers and a target value
S
, find a contiguous subarray that adds up toS
. If such a subarray doesn’t exist, returnnull
. You can return any valid subarray if multiple exist.
For example:
Input: arr = [1, 2, 3, 7, 5], S = 12
Output: [2, 3, 7]
At first glance, this problem might seem straightforward. You might be tempted to use a brute-force approach, checking every possible subarray. However, that would lead to a time complexity of O(n^2), which is inefficient for large inputs. Instead, we’ll explore a more optimal solution using the sliding window technique.
Understanding the Sliding Window Technique
The sliding window technique is a powerful method for solving problems involving arrays or strings, especially when we need to find a contiguous sequence that satisfies certain conditions. It’s called “sliding window” because we maintain a window (a range of elements) that slides through the array, expanding or contracting as needed.
This technique is particularly useful when:
- We’re dealing with a linear structure like an array or string.
- We’re looking for a contiguous subsequence or subarray.
- There’s a way to incrementally update our solution as we slide the window.
In our problem, all these conditions are met:
- We have an array of numbers.
- We’re looking for a contiguous subarray.
- We can incrementally update the sum as we slide the window.
The Solution: Step by Step
Let’s break down the solution into steps:
- Initialize two pointers,
start
andend
, both at the beginning of the array. - Maintain a variable
current_sum
to store the sum of elements in the current window. - Move the
end
pointer to the right, adding elements to the window and updatingcurrent_sum
. - If
current_sum
becomes greater thanS
, move thestart
pointer to the right, removing elements from the window and updatingcurrent_sum
. - If
current_sum
equalsS
, we’ve found our subarray! - If we reach the end of the array without finding a valid subarray, return
null
.
Now, let’s implement this solution in Python:
def find_subarray(arr, S):
start = 0
current_sum = 0
for end in range(len(arr)):
# Add the current element to current_sum
current_sum += arr[end]
# Shrink the window while the sum is greater than S
while current_sum > S and start <= end:
current_sum -= arr[start]
start += 1
# If we find a subarray that sums to S, return it
if current_sum == S:
return arr[start:end + 1]
# If no such subarray exists, return null
return None
Let’s walk through this code with our example input: arr = [1, 2, 3, 7, 5]
and S = 12
.
- We start with
start = 0
,end = 0
, andcurrent_sum = 0
. - We add
arr[0] = 1
tocurrent_sum
. Nowcurrent_sum = 1
. - Move to
end = 1
, addarr[1] = 2
. Nowcurrent_sum = 3
. - Move to
end = 2
, addarr[2] = 3
. Nowcurrent_sum = 6
. - Move to
end = 3
, addarr[3] = 7
. Nowcurrent_sum = 13
. - Since
current_sum > S
, we start shrinking the window from the left. - Subtract
arr[0] = 1
and movestart
to 1. Nowcurrent_sum = 12
. - We’ve found our subarray! Return
[2, 3, 7]
.
Time and Space Complexity Analysis
One of the key advantages of the sliding window technique is its efficiency. Let’s analyze the time and space complexity of our solution:
Time Complexity: O(n), where n is the length of the input array. Although we have nested loops, each element is added to the window and removed from the window at most once. The start
and end
pointers traverse the array only once.
Space Complexity: O(1), as we only use a constant amount of extra space to store our pointers and the current sum. Note that we’re not counting the space used to return the subarray, as that’s part of the output.
This optimal time complexity is a significant improvement over the O(n^2) brute-force approach, making our solution scalable for large inputs.
Edge Cases and Error Handling
In a real interview scenario, it’s crucial to consider edge cases and potential errors. Here are some edge cases we should consider for this problem:
- Empty array: If the input array is empty, we should immediately return
None
. - No valid subarray: If no subarray sums up to
S
, our function correctly returnsNone
. - Single element equal to S: Our function correctly handles this case, returning a subarray with a single element.
- S larger than the sum of all elements: If
S
is larger than the sum of all elements in the array, no valid subarray exists, and our function correctly returnsNone
.
While our current implementation handles these cases correctly, it’s always good practice to discuss these edge cases with your interviewer and potentially add explicit checks if required.
Beyond the Problem: Key Takeaways
This problem and its solution offer several valuable lessons for coding interviews and software development in general:
- Recognize pattern-matching opportunities: The sliding window technique is applicable to many problems involving contiguous sequences in arrays or strings. Recognizing when to apply this technique can save you time and lead to more efficient solutions.
- Optimize for time and space complexity: In interviews, it’s not enough to solve the problem; you need to solve it efficiently. Always consider the time and space complexity of your solution and look for ways to optimize.
- Incremental problem-solving: Notice how we built our solution step by step, starting with a basic approach and then optimizing it. This incremental approach is often more effective than trying to come up with the perfect solution immediately.
- Clear communication: Throughout the interview simulation, the candidate clearly explained their thought process, asked clarifying questions, and walked through their solution step-by-step. This level of communication is crucial in real interviews.
- Consider edge cases: Thinking about and handling edge cases demonstrates attention to detail and a thorough understanding of the problem.
Variations and Related Problems
The sliding window technique can be applied to a variety of problems. Here are a few related problems you might encounter:
- Maximum Sum Subarray of Size K: Find the maximum sum of any contiguous subarray of size K.
- Longest Substring with K Distinct Characters: Find the longest substring with at most K distinct characters.
- Minimum Window Substring: Find the smallest substring of a string containing all characters of another string.
- Longest Subarray with Ones after Replacement: Given an array of 0s and 1s, find the length of the longest contiguous subarray with at most K zeroes that can be replaced.
Practicing these related problems can help reinforce your understanding of the sliding window technique and prepare you for variations you might encounter in interviews.
Conclusion
Mastering techniques like the sliding window can significantly improve your problem-solving skills and prepare you for coding interviews. This problem demonstrates how a seemingly complex task can be solved efficiently with the right approach.
Remember, the key to success in coding interviews isn’t just about knowing algorithms and data structures. It’s about:
- Understanding the problem thoroughly
- Communicating your thought process clearly
- Considering different approaches and their trade-offs
- Writing clean, efficient code
- Testing your solution with various inputs, including edge cases
By following these principles and practicing regularly, you’ll be well-prepared to tackle any coding interview that comes your way. Happy coding, and best of luck with your interviews!