Mastering the Bellman-Ford Algorithm: A Comprehensive Guide for Coding Interviews
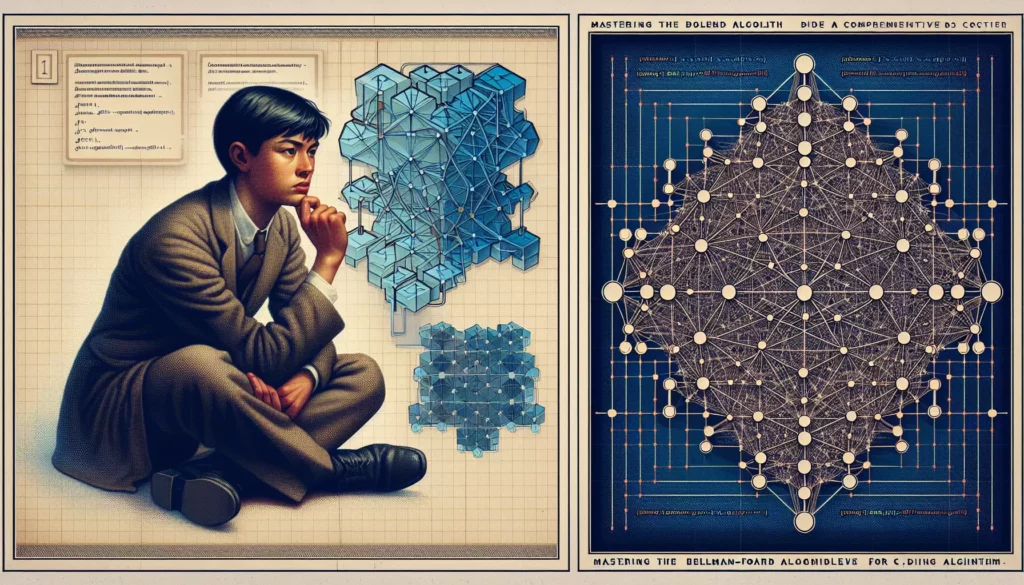
Welcome to AlgoCademy’s in-depth exploration of the Bellman-Ford algorithm! If you’re preparing for coding interviews, especially with top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding this powerful algorithm is crucial. In this comprehensive guide, we’ll dive deep into the Bellman-Ford algorithm, its applications, implementation, and how it can give you an edge in technical interviews.
Table of Contents
- Introduction to the Bellman-Ford Algorithm
- How the Bellman-Ford Algorithm Works
- Implementing Bellman-Ford in Python
- Time and Space Complexity Analysis
- Real-world Applications
- Bellman-Ford vs. Dijkstra’s Algorithm
- Interview Tips and Common Questions
- Practice Problems and Solutions
- Conclusion and Next Steps
1. Introduction to the Bellman-Ford Algorithm
The Bellman-Ford algorithm is a fundamental graph algorithm used to find the shortest paths from a single source vertex to all other vertices in a weighted graph. Named after its inventors, Richard Bellman and Lester Ford Jr., this algorithm is particularly useful because it can handle graphs with negative edge weights, unlike Dijkstra’s algorithm.
Key features of the Bellman-Ford algorithm include:
- Ability to detect negative cycles in a graph
- Guaranteed to find the shortest path if no negative cycles exist
- Works with both directed and undirected graphs
- Simple to implement and understand
Understanding the Bellman-Ford algorithm is essential for any programmer preparing for technical interviews, as it demonstrates a deep knowledge of graph algorithms and problem-solving skills.
2. How the Bellman-Ford Algorithm Works
The Bellman-Ford algorithm works by repeatedly relaxing all edges in the graph for V-1 iterations, where V is the number of vertices in the graph. Here’s a step-by-step breakdown of the algorithm:
- Initialize distances: Set the distance to the source vertex as 0 and all other vertices as infinity.
- Relax edges: For each edge (u, v) with weight w, if the distance to u plus w is less than the current distance to v, update the distance to v.
- Repeat step 2 for V-1 iterations.
- Check for negative cycles: Perform one more iteration of edge relaxation. If any distance is updated, a negative cycle exists.
Let’s visualize this process with a simple example:
A
/ \
1 4
/ \
B --2-- C
\ /
3 5
\ /
D
In this graph, we’ll find the shortest paths from vertex A to all other vertices.
Iteration 1:
– Distance to A: 0
– Distance to B: 1
– Distance to C: 4
– Distance to D: ∞
Iteration 2:
– Distance to A: 0
– Distance to B: 1
– Distance to C: 3 (via B)
– Distance to D: 4 (via B)
Iteration 3:
– Distance to A: 0
– Distance to B: 1
– Distance to C: 3
– Distance to D: 4
After three iterations (V-1, where V=4), we have found the shortest paths from A to all other vertices.
3. Implementing Bellman-Ford in Python
Now that we understand how the algorithm works, let’s implement it in Python. This implementation will be useful for coding interviews and practical applications.
def bellman_ford(graph, source):
# Step 1: Initialize distances and predecessor
distances = {node: float('inf') for node in graph}
distances[source] = 0
predecessor = {node: None for node in graph}
# Step 2: Relax edges |V| - 1 times
for _ in range(len(graph) - 1):
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
distances[neighbor] = distances[node] + weight
predecessor[neighbor] = node
# Step 3: Check for negative-weight cycles
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
raise ValueError("Graph contains a negative-weight cycle")
return distances, predecessor
# Example usage
graph = {
'A': {'B': 1, 'C': 4},
'B': {'C': 2, 'D': 3},
'C': {'D': 5},
'D': {}
}
source = 'A'
distances, predecessor = bellman_ford(graph, source)
print("Distances from source:", distances)
print("Predecessor map:", predecessor)
This implementation provides a clean and efficient way to apply the Bellman-Ford algorithm to any graph. It returns both the shortest distances and the predecessor map, which can be used to reconstruct the shortest paths.
4. Time and Space Complexity Analysis
Understanding the time and space complexity of the Bellman-Ford algorithm is crucial for optimizing its use and discussing its trade-offs in interview scenarios.
Time Complexity
The time complexity of the Bellman-Ford algorithm is O(V * E), where V is the number of vertices and E is the number of edges in the graph.
- The main loop runs V-1 times.
- In each iteration, we relax all E edges.
- The final check for negative cycles takes an additional O(E) time.
In the worst case, where E is O(V^2), the time complexity becomes O(V^3). This makes Bellman-Ford slower than Dijkstra’s algorithm for most cases, but it’s necessary when dealing with graphs that may contain negative edge weights.
Space Complexity
The space complexity of the Bellman-Ford algorithm is O(V), where V is the number of vertices.
- We store the distance and predecessor for each vertex.
- The graph representation (usually an adjacency list) takes O(V + E) space, but this is typically considered part of the input.
The relatively low space complexity makes Bellman-Ford suitable for large graphs where memory is a constraint.
5. Real-world Applications
The Bellman-Ford algorithm has numerous practical applications in various fields. Understanding these can help you discuss the algorithm’s relevance in interview scenarios.
- Networking Protocols: The algorithm is used in routing protocols like RIP (Routing Information Protocol) to find the best path for data packets.
- Forex Trading: In currency exchange markets, Bellman-Ford can be used to detect arbitrage opportunities by modeling exchange rates as a graph.
- Traffic Management Systems: It can be applied to find the shortest or most efficient routes in transportation networks, considering factors like traffic and road conditions.
- Game Development: In strategy games or RPGs, the algorithm can be used for pathfinding, especially in scenarios where paths may have varying costs or benefits.
- Circuit Design: Electrical engineers use Bellman-Ford to analyze timing constraints in digital circuits.
Being able to discuss these applications demonstrates a broader understanding of the algorithm’s significance beyond just coding interviews.
6. Bellman-Ford vs. Dijkstra’s Algorithm
In technical interviews, you may be asked to compare different algorithms for solving similar problems. Here’s a comparison between Bellman-Ford and Dijkstra’s algorithm:
Aspect | Bellman-Ford | Dijkstra’s Algorithm |
---|---|---|
Time Complexity | O(V * E) | O((V + E) log V) with binary heap |
Negative Edge Weights | Can handle | Cannot handle |
Negative Cycles | Can detect | Not applicable |
Efficiency for Dense Graphs | Less efficient | More efficient |
Implementation Complexity | Simpler | More complex (requires priority queue) |
Key takeaways:
- Use Bellman-Ford when negative edge weights are possible or when you need to detect negative cycles.
- Prefer Dijkstra’s algorithm for non-negative edge weights, especially in large, sparse graphs.
- Bellman-Ford is often easier to implement but generally slower for large graphs.
7. Interview Tips and Common Questions
When discussing the Bellman-Ford algorithm in a coding interview, keep these tips in mind:
- Understand the problem: Make sure you know whether negative edge weights are possible. If not, suggest Dijkstra’s algorithm as a potentially more efficient alternative.
- Explain your thought process: Walk the interviewer through your approach, discussing why Bellman-Ford is suitable for the given problem.
- Optimize where possible: Discuss potential optimizations, such as early termination if no changes are made in an iteration.
- Handle edge cases: Consider scenarios like disconnected graphs or graphs with no valid paths.
- Discuss time and space complexity: Be prepared to analyze the efficiency of your implementation.
Common interview questions related to Bellman-Ford:
- “How would you modify Bellman-Ford to return the actual shortest path, not just the distances?”
- “Can you implement Bellman-Ford to work with an adjacency matrix instead of an adjacency list?”
- “How would you parallelize the Bellman-Ford algorithm?”
- “Explain a scenario where Bellman-Ford would be preferable to Dijkstra’s algorithm.”
- “How would you optimize Bellman-Ford for sparse graphs?”
8. Practice Problems and Solutions
To solidify your understanding of the Bellman-Ford algorithm, try solving these practice problems:
Problem 1: Negative Cycle Detection
Problem: Modify the Bellman-Ford algorithm to not only detect the presence of a negative cycle but also to return the cycle if one exists.
Solution:
def bellman_ford_with_cycle(graph, source):
distances = {node: float('inf') for node in graph}
distances[source] = 0
predecessor = {node: None for node in graph}
for _ in range(len(graph) - 1):
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
distances[neighbor] = distances[node] + weight
predecessor[neighbor] = node
# Check for negative cycle and return it if found
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
# Negative cycle found, reconstruct the cycle
cycle = []
current = neighbor
for _ in range(len(graph)):
cycle.append(current)
current = predecessor[current]
if current in cycle:
start = cycle.index(current)
return cycle[start:]
return None # No negative cycle found
# Example usage
graph_with_cycle = {
'A': {'B': 1},
'B': {'C': -3},
'C': {'D': 2},
'D': {'B': 1}
}
cycle = bellman_ford_with_cycle(graph_with_cycle, 'A')
print("Negative cycle:", cycle if cycle else "No negative cycle found")
Problem 2: Maximum Path Value
Problem: Given a weighted graph, find the maximum path value from a source node to all other nodes. Assume all edge weights are positive.
Solution:
def max_path_bellman_ford(graph, source):
max_values = {node: float('-inf') for node in graph}
max_values[source] = 0
for _ in range(len(graph) - 1):
for node in graph:
for neighbor, weight in graph[node].items():
if max_values[node] + weight > max_values[neighbor]:
max_values[neighbor] = max_values[node] + weight
return max_values
# Example usage
graph = {
'A': {'B': 3, 'C': 6},
'B': {'C': 4, 'D': 2},
'C': {'D': 1},
'D': {}
}
max_path_values = max_path_bellman_ford(graph, 'A')
print("Maximum path values:", max_path_values)
These practice problems help reinforce your understanding of the Bellman-Ford algorithm and its applications. They also demonstrate how the basic algorithm can be modified to solve related problems, which is a valuable skill in coding interviews.
9. Conclusion and Next Steps
Congratulations! You’ve now gained a comprehensive understanding of the Bellman-Ford algorithm, its implementation, applications, and how to approach it in coding interviews. This knowledge will serve you well in your preparation for technical interviews, especially with top tech companies.
To further enhance your skills and preparation:
- Practice regularly: Implement the algorithm from scratch and solve related problems to build muscle memory.
- Explore variations: Look into optimized versions of Bellman-Ford, such as the SPFA (Shortest Path Faster Algorithm).
- Study related algorithms: Deepen your understanding of graph algorithms by studying Dijkstra’s algorithm, Floyd-Warshall, and others.
- Apply to real-world scenarios: Try to identify situations in your current projects where Bellman-Ford might be applicable.
- Mock interviews: Practice explaining the algorithm and its nuances in a mock interview setting.
Remember, mastering algorithms like Bellman-Ford is not just about memorizing code, but understanding the underlying principles and being able to apply them creatively to solve diverse problems. Keep practicing, stay curious, and you’ll be well-prepared for your coding interviews!
For more in-depth tutorials, practice problems, and interview preparation resources, don’t forget to explore other sections of AlgoCademy. Happy coding, and best of luck with your interview preparation!