Mastering the Art of Flowcharts for Algorithm Design
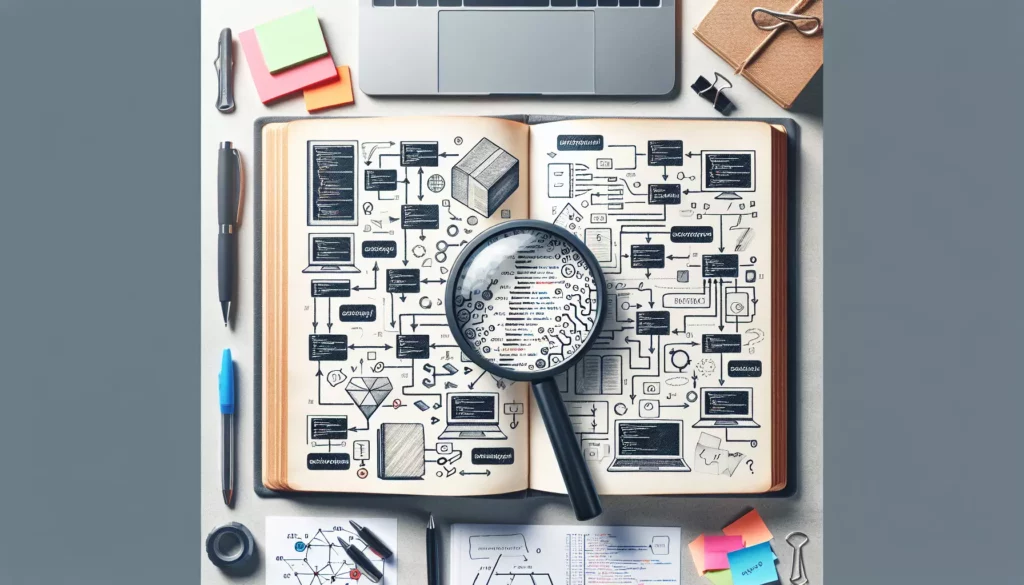
In the world of programming and algorithm design, visual representations can be incredibly powerful tools. Among these, flowcharts stand out as an essential technique for mapping out the logic and structure of algorithms. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, mastering the art of flowcharts can significantly enhance your problem-solving skills and algorithm design process.
In this comprehensive guide, we’ll explore the ins and outs of using flowcharts for algorithm design, from basic concepts to advanced techniques. We’ll cover why flowcharts are crucial in coding education, how they can improve your algorithmic thinking, and provide practical tips for creating effective flowcharts. By the end of this article, you’ll have a solid understanding of how to leverage flowcharts to tackle complex programming challenges and streamline your coding process.
Table of Contents
- Understanding Flowcharts: The Basics
- The Importance of Flowcharts in Algorithm Design
- Key Elements of Flowcharts
- Creating Effective Flowcharts: Step-by-Step Guide
- Best Practices for Flowchart Design
- Tools and Software for Creating Flowcharts
- Translating Flowcharts into Code
- Advanced Flowchart Techniques for Complex Algorithms
- Common Pitfalls and How to Avoid Them
- Real-World Examples: Flowcharts in Action
- Using Flowcharts in Technical Interviews
- Conclusion: Elevating Your Algorithm Design Skills
Understanding Flowcharts: The Basics
Before diving into the intricacies of using flowcharts for algorithm design, let’s start with the fundamentals. A flowchart is a diagram that visually represents a process, system, or algorithm. It uses standardized symbols connected by arrows to show the sequence of steps and decision points within a process.
Flowcharts serve several purposes in programming and algorithm design:
- Visualizing the logical flow of an algorithm
- Breaking down complex problems into manageable steps
- Identifying decision points and potential branches in the process
- Communicating ideas clearly to team members or stakeholders
- Debugging and optimizing existing algorithms
By mastering flowcharts, you’ll be better equipped to tackle complex coding challenges and articulate your solutions effectively.
The Importance of Flowcharts in Algorithm Design
In the context of algorithm design, flowcharts play a crucial role in the development process. Here’s why they’re so important:
- Visual Representation: Flowcharts provide a clear, visual representation of an algorithm’s logic, making it easier to understand and analyze the process.
- Problem Decomposition: They help break down complex problems into smaller, more manageable steps, facilitating a systematic approach to problem-solving.
- Logic Verification: Flowcharts allow you to verify the logic of your algorithm before writing any code, potentially saving time and reducing errors.
- Communication Tool: They serve as an excellent communication tool, enabling you to explain your algorithm to others, including non-technical stakeholders.
- Documentation: Flowcharts act as valuable documentation, providing a quick reference for understanding the algorithm’s structure and flow.
- Optimization: By visualizing the entire process, flowcharts can help identify areas for optimization and improvement in your algorithm.
Incorporating flowcharts into your algorithm design process can significantly enhance your problem-solving skills and lead to more efficient, well-structured code.
Key Elements of Flowcharts
To create effective flowcharts for algorithm design, it’s essential to understand the standard symbols and their meanings. Here are the key elements you’ll commonly use:
- Start/End: Oval shapes represent the beginning and end of the algorithm.
- Process: Rectangles indicate a specific action or operation to be performed.
- Decision: Diamond shapes represent a decision point or conditional statement, typically with “Yes” and “No” branches.
- Input/Output: Parallelograms denote input or output operations, such as reading data or displaying results.
- Connector: Small circles are used to connect different parts of the flowchart, especially when it spans multiple pages.
- Arrows: Lines with arrowheads show the flow direction and sequence of steps in the algorithm.
- Subroutine: Rectangles with double-lined sides represent a predefined process or subroutine.
Understanding these elements and their proper usage is crucial for creating clear and effective flowcharts that accurately represent your algorithms.
Creating Effective Flowcharts: Step-by-Step Guide
Now that we’ve covered the basics, let’s walk through the process of creating an effective flowchart for algorithm design:
- Define the Problem: Clearly articulate the problem your algorithm needs to solve.
- Identify Inputs and Outputs: Determine what data your algorithm will receive and what results it should produce.
- Break Down the Process: Divide the algorithm into distinct steps or operations.
- Arrange Steps Logically: Organize the steps in a logical sequence, considering the flow of data and operations.
- Add Decision Points: Incorporate decision symbols where the algorithm needs to make choices based on conditions.
- Connect Elements: Use arrows to connect the various elements, showing the flow of the algorithm.
- Review and Refine: Analyze your flowchart for completeness and clarity, making adjustments as needed.
- Test with Examples: Walk through the flowchart with sample inputs to ensure it correctly represents the algorithm’s logic.
Following this step-by-step approach will help you create comprehensive and accurate flowcharts for your algorithms.
Best Practices for Flowchart Design
To ensure your flowcharts are as effective as possible, consider these best practices:
- Keep it Simple: Avoid overcomplicated designs. If a flowchart becomes too complex, consider breaking it into smaller sub-flowcharts.
- Use Consistent Symbols: Stick to standard flowchart symbols to ensure clarity and universal understanding.
- Maintain a Clear Flow: Arrange elements so that the flow generally moves from top to bottom and left to right.
- Label Clearly: Use concise, descriptive labels for each step and decision point.
- Include Necessary Details: Provide enough information to understand each step without cluttering the diagram.
- Use Connectors Wisely: When lines must cross, use connector symbols to maintain clarity.
- Consider Scale: Ensure your flowchart is readable at different zoom levels, especially for complex algorithms.
- Color-Code Thoughtfully: If using colors, do so consistently and with purpose, keeping in mind accessibility for color-blind individuals.
By adhering to these best practices, you’ll create flowcharts that are both visually appealing and highly functional for algorithm design.
Tools and Software for Creating Flowcharts
While you can create flowcharts using pen and paper, various digital tools can streamline the process and produce professional-looking results. Here are some popular options:
- Lucidchart: A web-based diagramming application that offers a wide range of templates and shapes for flowcharts.
- Draw.io: A free, open-source tool that integrates with Google Drive and offers a vast library of shapes and icons.
- Microsoft Visio: A powerful diagramming tool with advanced features, ideal for complex flowcharts and integrations with other Microsoft products.
- Creately: An online tool with a user-friendly interface and real-time collaboration features.
- Miro: A collaborative online whiteboard platform that includes flowchart creation capabilities.
- Figma: While primarily a design tool, Figma can be used to create visually appealing flowcharts with its vector editing capabilities.
Experiment with different tools to find the one that best suits your needs and workflow. Many of these offer free trials or basic versions, allowing you to explore their features before committing.
Translating Flowcharts into Code
One of the key benefits of using flowcharts in algorithm design is the ease with which they can be translated into actual code. Here’s a general approach to converting your flowchart into a working program:
- Identify Main Structures: Look for patterns in your flowchart that correspond to common programming structures like loops, conditionals, and functions.
- Define Variables: Determine what variables you’ll need based on the inputs, outputs, and intermediate steps in your flowchart.
- Implement Step by Step: Follow the flowchart’s sequence, implementing each step as a line of code or a block of related code.
- Handle Decision Points: Translate diamond shapes into if-else statements or switch-case structures in your code.
- Create Functions: If your flowchart includes subroutines or repeated processes, implement these as functions in your code.
- Add Error Handling: Incorporate error checking and exception handling where appropriate, especially at input/output points.
- Test and Debug: Use the same test cases you applied to your flowchart to verify your code’s functionality.
Let’s look at a simple example of translating a flowchart into Python code:
Flowchart for calculating the factorial of a number:
Start
|
v
Input n
|
v
Initialize result = 1
|
v
Is n > 0? --No--> Display result
| |
Yes v
| End
v
result = result * n
|
v
n = n - 1
|
v
(back to "Is n > 0?")
Translated into Python code:
def factorial(n):
result = 1
while n > 0:
result *= n
n -= 1
return result
# Get input from user
num = int(input("Enter a number to calculate its factorial: "))
# Calculate and display the result
print(f"The factorial of {num} is {factorial(num)}")
This example demonstrates how each element of the flowchart translates directly into code structures, making the implementation process more straightforward and less error-prone.
Advanced Flowchart Techniques for Complex Algorithms
As you tackle more complex algorithms, you’ll need to employ advanced flowcharting techniques to effectively represent intricate logic and processes. Here are some advanced concepts to consider:
- Nested Structures: Use nested shapes or sub-flowcharts to represent complex conditional statements or nested loops.
- Parallel Processing: Employ parallel lines or swim lanes to show concurrent operations in multi-threaded algorithms.
- Data Structures: Develop custom symbols or notations to represent complex data structures like trees, graphs, or hash tables.
- Recursive Algorithms: Use special notations or connectors to indicate recursive calls within your flowchart.
- Error Handling: Incorporate dedicated paths and symbols for exception handling and error scenarios.
- Modularization: Break down complex algorithms into smaller, manageable sub-flowcharts, using connectors to link them together.
- Annotations: Add explanatory notes or comments to clarify complex steps or decisions in your flowchart.
These advanced techniques will allow you to create more comprehensive and detailed flowcharts that accurately represent even the most complex algorithms.
Common Pitfalls and How to Avoid Them
When creating flowcharts for algorithm design, be aware of these common pitfalls and how to avoid them:
- Overcomplexity: Avoid making your flowchart too detailed or convoluted. If it becomes hard to follow, break it down into smaller, linked flowcharts.
- Inconsistent Symbols: Stick to standard flowchart symbols and use them consistently throughout your diagram.
- Unclear Flow: Ensure that the direction of flow is always clear, using arrows to guide the reader through the process.
- Missing Steps: Double-check that you’ve included all necessary steps and haven’t omitted any crucial operations.
- Ignoring Edge Cases: Consider and include paths for all possible scenarios, including error conditions and edge cases.
- Poor Labeling: Use clear, concise labels for each step and decision point to avoid ambiguity.
- Lack of Testing: Always test your flowchart with various inputs to ensure it accurately represents your algorithm’s logic.
By being mindful of these potential issues, you can create more effective and accurate flowcharts for your algorithms.
Real-World Examples: Flowcharts in Action
To better understand how flowcharts are used in real-world algorithm design, let’s look at a couple of examples:
1. Binary Search Algorithm
Here’s a simplified flowchart for the binary search algorithm:
Start
|
v
Initialize low = 0, high = array length - 1
|
v
While low <= high
|
v
Calculate mid = (low + high) / 2
|
v
Is target == array[mid]? --Yes--> Return mid
| |
No v
| End
v
Is target < array[mid]? --Yes--> high = mid - 1
| |
No |
| |
v |
low = mid + 1 |
| |
v |
(back to "While low <= high") |
| |
v |
Return -1 (not found) |
| |
v |
End <----------------------------+
This flowchart clearly shows the logic of the binary search algorithm, including the main loop, comparison steps, and how the search range is narrowed down.
2. Quicksort Algorithm
Here’s a high-level flowchart for the Quicksort algorithm:
Start
|
v
Is array length <= 1? --Yes--> Return array
| |
No v
| End
v
Choose pivot element
|
v
Partition array around pivot
|
v
Recursively quicksort left partition
|
v
Recursively quicksort right partition
|
v
Combine sorted partitions
|
v
Return sorted array
|
v
End
This flowchart provides a high-level overview of the Quicksort algorithm, showing its recursive nature and main steps. For a more detailed implementation, each step could be expanded into its own sub-flowchart.
These examples demonstrate how flowcharts can effectively represent both iterative and recursive algorithms, providing a clear visual representation of their logic and flow.
Using Flowcharts in Technical Interviews
Flowcharts can be a powerful tool during technical interviews, especially when tackling complex algorithmic problems. Here’s how you can leverage flowcharts to your advantage:
- Problem Understanding: Use a flowchart to quickly sketch out your understanding of the problem, helping to clarify requirements and edge cases.
- Solution Planning: Before diving into code, create a high-level flowchart of your proposed solution to organize your thoughts and approach.
- Communication: Use the flowchart to explain your algorithm to the interviewer, demonstrating your problem-solving process and logical thinking.
- Optimization: Analyze your flowchart to identify potential bottlenecks or areas for improvement in your algorithm.
- Code Structure: Use the flowchart as a guide when writing your code, ensuring you cover all the steps and conditions you’ve outlined.
- Debugging: If issues arise during coding, refer back to your flowchart to trace the logic and identify where problems might be occurring.
Remember, while flowcharts are useful, they should complement, not replace, your ability to write and explain code. Use them as a tool to enhance your problem-solving and communication skills during interviews.
Conclusion: Elevating Your Algorithm Design Skills
Mastering the art of flowcharts for algorithm design is a valuable skill that can significantly enhance your abilities as a programmer and problem-solver. By visualizing complex processes, breaking down problems into manageable steps, and clearly communicating your ideas, flowcharts serve as a powerful tool in your coding arsenal.
As you continue to develop your skills in algorithm design and prepare for technical interviews, remember these key takeaways:
- Flowcharts provide a clear, visual representation of your algorithm’s logic and flow.
- They help in problem decomposition, logic verification, and optimization of your algorithms.
- Understanding and consistently using standard flowchart symbols is crucial for effective communication.
- Practice creating flowcharts for various algorithms to improve your skills and speed.
- Use flowcharts as a stepping stone to writing clean, well-structured code.
- Leverage flowcharts in technical interviews to demonstrate your problem-solving approach and communicate your ideas effectively.
By incorporating flowcharts into your algorithm design process, you’ll not only improve your own understanding and problem-solving capabilities but also enhance your ability to collaborate with others and tackle complex coding challenges. Whether you’re just starting your coding journey or preparing for interviews with top tech companies, mastering flowcharts will undoubtedly give you a competitive edge in the world of programming and algorithm design.