Mastering the Art of Debugging: Essential Techniques for Technical Interviews
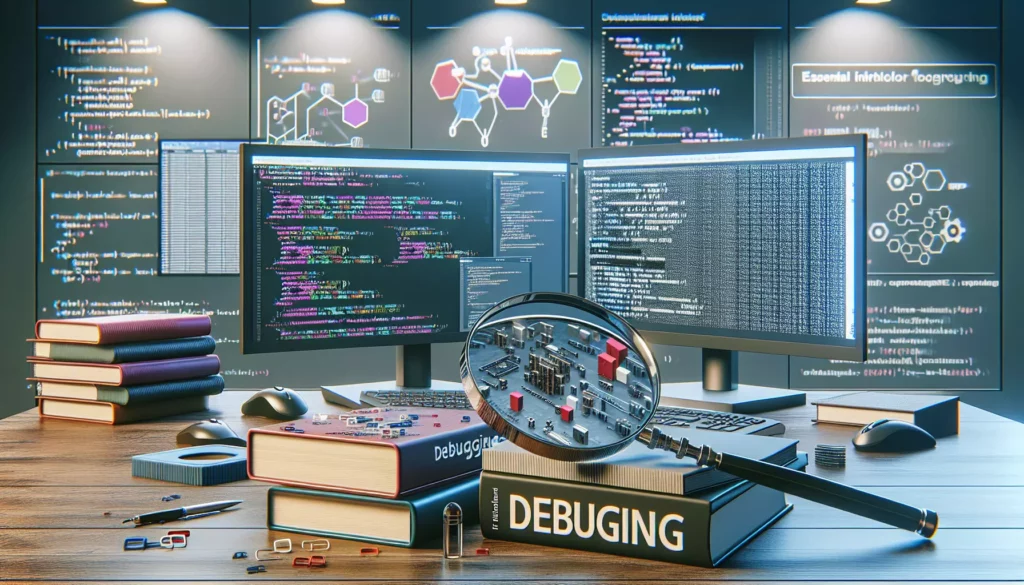
In the high-pressure environment of a technical interview, your ability to debug code efficiently can make or break your chances of landing that dream job. Whether you’re a seasoned developer or just starting your journey in the world of programming, having a solid grasp of debugging techniques is crucial. This comprehensive guide will walk you through various strategies and tools that will help you shine during your next coding interview.
Why Debugging Skills Matter in Interviews
Before we dive into the techniques, let’s understand why debugging skills are so vital in the context of technical interviews:
- Problem-solving prowess: Debugging showcases your analytical thinking and ability to dissect complex issues.
- Attention to detail: It demonstrates your meticulousness and capacity to spot subtle errors.
- Code comprehension: Debugging requires a deep understanding of how code works, which is highly valued by interviewers.
- Communication skills: Explaining your debugging process allows you to showcase your ability to articulate technical concepts clearly.
- Real-world relevance: Debugging is a significant part of a developer’s day-to-day work, making it a crucial skill to assess.
Essential Debugging Techniques for Interviews
1. The Print Statement Method
One of the simplest yet most effective debugging techniques is using print statements. This method involves strategically placing output statements throughout your code to track variable values, function calls, and program flow.
How to use it:
- Insert print statements at key points in your code
- Output variable values, function parameters, and return values
- Use descriptive labels to identify each print statement
Example:
def calculate_sum(a, b):
print(f"Input: a = {a}, b = {b}")
result = a + b
print(f"Result: {result}")
return result
total = calculate_sum(5, 3)
print(f"Final total: {total}")
This technique is particularly useful when you don’t have access to a full-fledged debugger during an interview.
2. Rubber Duck Debugging
Rubber duck debugging is a method where you explain your code line-by-line to an inanimate object (traditionally a rubber duck). This technique forces you to articulate your thought process and often leads to discovering the bug on your own.
Steps to follow:
- Grab an imaginary rubber duck (or any object)
- Explain your code to the “duck” as if it knows nothing about programming
- Go through each line methodically, describing what it does and why
- Pay attention to any inconsistencies or errors you notice while explaining
This method is particularly effective in interviews as it allows you to demonstrate your communication skills and thought process to the interviewer.
3. Binary Search Debugging
When dealing with larger codebases or complex algorithms, the binary search method can be incredibly efficient. This technique involves systematically narrowing down the location of a bug by dividing the code into sections.
How to apply binary search debugging:
- Identify the start and end points where the code is known to work correctly
- Find the midpoint between these two points
- Test the code at the midpoint
- If the bug occurs before the midpoint, focus on the first half; otherwise, focus on the second half
- Repeat the process until you isolate the bug
This method is particularly useful when you’re dealing with a large function or algorithm during an interview and need to quickly pinpoint the source of an error.
4. Debugging by Simplification
Sometimes, the best approach is to simplify the problem. This technique involves removing or commenting out parts of the code to isolate the issue.
Steps for debugging by simplification:
- Identify the minimal test case that reproduces the bug
- Comment out or remove sections of code that aren’t directly related to the problem
- Gradually add back pieces of code until the bug reappears
- Focus on the last piece of code added, as it’s likely the source of the bug
This method can be particularly effective in interview scenarios where you’re given a complex piece of code and asked to find and fix a bug.
5. Using Assertions
Assertions are a powerful tool for catching and identifying bugs early. They allow you to state assumptions about your code that should always be true.
How to use assertions effectively:
- Place assertions at critical points in your code where certain conditions must be met
- Use assertions to check input parameters, return values, and invariants
- Write clear, descriptive error messages for your assertions
Example:
def divide(a, b):
assert b != 0, "Cannot divide by zero"
return a / b
result = divide(10, 2)
print(result) # Output: 5.0
result = divide(10, 0) # This will raise an AssertionError
Using assertions during an interview demonstrates your commitment to writing robust, error-resistant code.
Advanced Debugging Techniques
6. Time Travel Debugging
While not always available during interviews, understanding time travel debugging can impress your interviewer and showcase your knowledge of advanced debugging concepts.
Time travel debugging allows you to step backwards through your code execution, helping you understand the sequence of events that led to a bug.
Key concepts of time travel debugging:
- Reverse execution: Moving backwards through the program’s execution history
- State inspection: Examining variable values at different points in time
- Conditional breakpoints: Pausing execution when specific conditions are met
While you may not have access to time travel debugging tools during an interview, discussing this technique can demonstrate your awareness of cutting-edge debugging methodologies.
7. Log-Based Debugging
Log-based debugging involves strategically placing log statements throughout your code to track the program’s execution and state over time.
Best practices for log-based debugging:
- Use different log levels (e.g., INFO, DEBUG, ERROR) to categorize messages
- Include timestamps and contextual information in log messages
- Log input parameters, return values, and important state changes
- Use log aggregation and analysis tools for complex applications
Example of log-based debugging:
import logging
logging.basicConfig(level=logging.DEBUG)
def calculate_average(numbers):
logging.info(f"Calculating average for: {numbers}")
if not numbers:
logging.warning("Empty list provided")
return 0
total = sum(numbers)
count = len(numbers)
average = total / count
logging.debug(f"Total: {total}, Count: {count}, Average: {average}")
return average
result = calculate_average([1, 2, 3, 4, 5])
logging.info(f"Final result: {result}")
While you may not implement full logging during an interview, discussing this approach shows your understanding of debugging in larger, more complex systems.
8. Debugging Memory Issues
In languages like C and C++, memory-related bugs can be particularly challenging. Understanding how to debug memory issues can set you apart in interviews for roles involving low-level programming.
Key aspects of debugging memory issues:
- Memory leaks: Identifying and fixing unfreed memory allocations
- Buffer overflows: Detecting and preventing out-of-bounds memory access
- Use-after-free: Catching attempts to use memory that has been deallocated
- Memory corruption: Identifying and resolving invalid memory modifications
Tools for memory debugging:
- Valgrind: A powerful tool suite for memory debugging, detection of memory leaks, and profiling
- AddressSanitizer: A fast memory error detector for C/C++
- Electric Fence: A debugger that detects memory allocation bugs
While you may not use these tools during an interview, demonstrating knowledge of memory debugging concepts and tools can be valuable, especially for systems programming positions.
Debugging Strategies for Different Types of Bugs
9. Tackling Logic Errors
Logic errors are often the trickiest to debug because the code runs without throwing exceptions, but produces incorrect results. Here’s how to approach them:
- Understand the expected behavior thoroughly
- Use print statements or logging to track the program’s flow and variable states
- Compare the actual output with the expected output at each step
- Use assertions to verify assumptions about your code’s behavior
- Break down complex logic into smaller, testable units
Example of debugging a logic error:
def is_prime(n):
if n < 2:
return False
for i in range(2, n): # Bug: should be range(2, int(n**0.5) + 1)
if n % i == 0:
return False
return True
print(is_prime(9)) # Incorrectly outputs True
To debug this, you might add print statements to track the values of i
and the result of n % i
, helping you identify why the function is failing for certain inputs.
10. Resolving Runtime Errors
Runtime errors occur during program execution and often result in the program crashing. Here’s how to approach them:
- Read the error message carefully – it often points directly to the issue
- Identify the line number where the error occurred
- Check for common causes like null pointer exceptions, division by zero, or index out of bounds
- Use a debugger to step through the code leading up to the error
- Verify that all variables have expected values before the error occurs
Example of a runtime error:
def get_element(lst, index):
return lst[index]
numbers = [1, 2, 3]
print(get_element(numbers, 3)) # Raises IndexError
To debug this, you might add bounds checking to the get_element
function or use a try-except block to handle the potential IndexError gracefully.
11. Fixing Syntax Errors
Syntax errors are usually the easiest to fix, as they’re caught by the compiler or interpreter before the program runs. Here’s how to handle them:
- Read the error message, which typically indicates the nature and location of the error
- Check for common syntax issues like missing parentheses, brackets, or semicolons
- Verify that all keywords are spelled correctly
- Ensure proper indentation, especially in languages like Python
- Use an IDE with syntax highlighting and real-time error checking
Example of a syntax error:
def greet(name)
print(f"Hello, {name}!") # Missing colon after function definition
greet("Alice")
This error is straightforward to fix by adding the missing colon after the function definition.
Debugging Tools and IDE Features
12. Leveraging Integrated Debuggers
Most modern IDEs come with powerful integrated debuggers. While you may not have access to your preferred IDE during an interview, understanding these features can help you articulate your debugging process:
- Breakpoints: Allow you to pause program execution at specific lines
- Step Over/Into/Out: Navigate through code execution line by line or function by function
- Watch Windows: Monitor the values of specific variables as the program runs
- Call Stack: View the sequence of function calls that led to the current point in execution
- Conditional Breakpoints: Pause execution only when specific conditions are met
Familiarize yourself with these features in your preferred IDE, as they can significantly speed up your debugging process in real-world scenarios.
13. Using Version Control for Debugging
Version control systems like Git can be powerful allies in debugging, especially for tracking down when and where a bug was introduced:
- Git Bisect: Perform a binary search through your commit history to find the commit that introduced a bug
- Diff Tools: Compare different versions of your code to identify changes that might have caused issues
- Blame: Identify who last modified each line of code, which can be helpful in understanding the context of changes
While you won’t use version control during an interview, understanding these concepts demonstrates your knowledge of professional development practices.
Debugging in Different Programming Paradigms
14. Debugging Object-Oriented Code
When debugging object-oriented code, consider these specific strategies:
- Verify object state: Ensure object properties have expected values
- Check method overriding: Confirm that the correct method implementation is being called
- Investigate inheritance hierarchies: Debug issues related to inheritance and polymorphism
- Use the debugger to step into constructors and methods
Example of debugging an OOP issue:
class Animal:
def make_sound(self):
print("Generic animal sound")
class Dog(Animal):
def make_sound(self):
print("Woof!")
class Cat(Animal):
def meow_sound(self): # Bug: method name doesn't override the parent class method
print("Meow!")
animals = [Dog(), Cat()]
for animal in animals:
animal.make_sound() # Cat will use the Animal class's make_sound method
To debug this, you’d need to recognize that the Cat
class’s method name should be make_sound
to properly override the parent class method.
15. Debugging Functional Code
Functional programming presents unique debugging challenges due to its emphasis on immutability and pure functions:
- Use function composition to break down complex operations into smaller, testable units
- Leverage higher-order functions for debugging, such as creating debug wrappers around functions
- Pay special attention to recursion and ensure base cases are correctly handled
- Use lazy evaluation features carefully, as they can make debugging more challenging
Example of debugging functional code:
def add(a, b):
return a + b
def multiply(a, b):
return a * b
def calculate(operation, x, y):
return operation(x, y)
result = calculate(add, 5, 3)
print(result) # Output: 8
result = calculate(multiply, 5, 3)
print(result) # Output: 15
# Debugging challenge: What if we want to log each operation?
def debug_wrapper(func):
def wrapper(x, y):
result = func(x, y)
print(f"{func.__name__}({x}, {y}) = {result}")
return result
return wrapper
add_with_logging = debug_wrapper(add)
multiply_with_logging = debug_wrapper(multiply)
result = calculate(add_with_logging, 5, 3)
result = calculate(multiply_with_logging, 5, 3)
This example demonstrates how to create a debug wrapper for functions, which can be particularly useful when debugging functional code.
Advanced Debugging Scenarios
16. Debugging Multithreaded Code
Multithreaded programming introduces a new level of complexity in debugging due to race conditions, deadlocks, and other concurrency issues:
- Use thread-safe logging to track the execution of different threads
- Employ thread sanitizers to detect data races and other concurrency bugs
- Utilize debuggers that support multithreaded debugging, allowing you to switch between thread contexts
- Be aware of the limitations of print statement debugging in multithreaded environments
Example of a multithreading bug:
import threading
counter = 0
def increment():
global counter
for _ in range(100000):
counter += 1 # This operation is not atomic
threads = []
for _ in range(5):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(f"Final counter value: {counter}") # Expected: 500000, Actual: Less than 500000
To debug this, you’d need to recognize the race condition and implement proper synchronization, such as using a lock or employing atomic operations.
17. Debugging Network and Distributed Systems
Debugging network and distributed systems presents unique challenges due to their complex, asynchronous nature:
- Use network protocol analyzers like Wireshark to inspect network traffic
- Implement comprehensive logging across all system components
- Utilize distributed tracing tools to track requests across multiple services
- Simulate various network conditions (latency, packet loss) to test system robustness
While you may not encounter distributed systems debugging in a typical coding interview, understanding these concepts can be valuable for certain roles or companies.
Debugging Best Practices and Tips
18. Developing a Systematic Approach
Having a structured approach to debugging can significantly improve your efficiency and effectiveness:
- Reproduce the bug consistently
- Isolate the problem to the smallest possible code snippet
- Form a hypothesis about the cause of the bug
- Test your hypothesis through targeted modifications or debugging techniques
- Fix the bug and verify the solution
- Document the bug and its solution for future reference
19. Effective Communication During Debugging
In an interview setting, clearly communicating your debugging process is crucial:
- Think aloud as you debug, explaining your thought process to the interviewer
- Clearly state your assumptions and the reasoning behind your debugging steps
- Ask clarifying questions if you’re unsure about any aspect of the problem or expected behavior
- Summarize your findings and proposed solutions concisely
20. Learning from Bugs
Every bug is an opportunity to improve your coding and debugging skills:
- Analyze the root cause of each bug you encounter
- Identify patterns in the types of bugs you frequently encounter
- Develop coding practices that help prevent similar bugs in the future
- Share your learnings with team members to improve overall code quality
Conclusion
Mastering the art of debugging is an essential skill for any programmer, and it’s particularly crucial for success in technical interviews. By familiarizing yourself with these techniques and practicing them regularly, you’ll be well-prepared to tackle any debugging challenge that comes your way during an interview.
Remember, debugging is not just about fixing errors; it’s about understanding code deeply, thinking critically, and communicating effectively. These are all qualities that interviewers highly value. So, embrace debugging as an opportunity to showcase your skills and stand out as a candidate.
As you continue to hone your debugging skills, keep in mind that practice makes perfect. Work on diverse coding projects, contribute to open-source repositories, and seek out challenging problems to solve. The more you debug, the more intuitive and efficient you’ll become at identifying and resolving issues.
Lastly, stay curious and keep learning. The field of software development is constantly evolving, and new debugging tools and techniques are always emerging. By staying up-to-date with the latest practices and continuously improving your skills, you’ll not only excel in interviews but also become a more valuable and effective developer in your career.