Mastering the Algorithmic/Problem-Solving Interview: A Comprehensive Guide
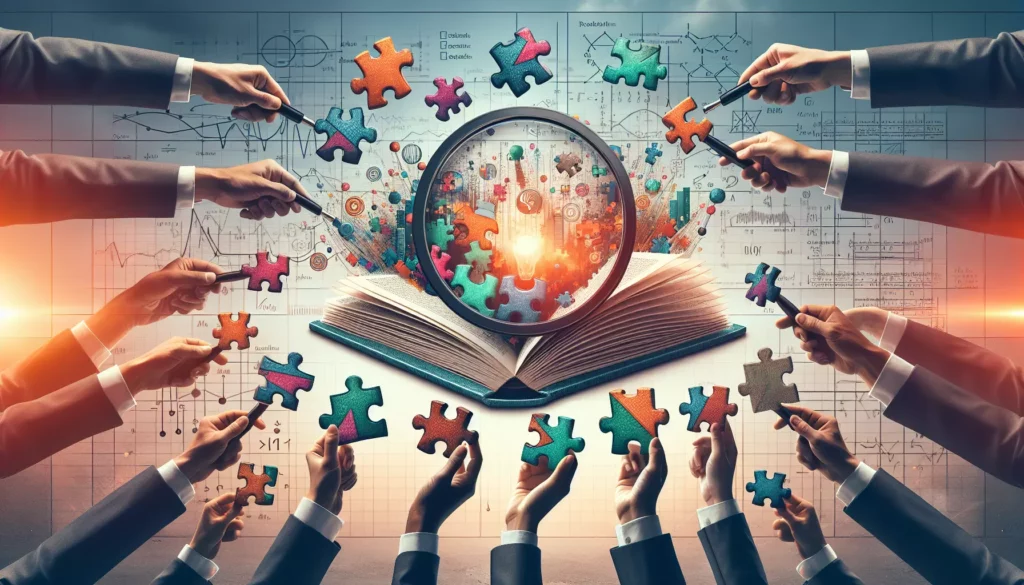
In the competitive world of software engineering, the algorithmic/problem-solving interview stands as a formidable challenge for aspiring developers. This crucial stage of the hiring process is designed to evaluate a candidate’s ability to think critically, solve complex problems, and write efficient code. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, mastering this type of interview is essential. In this comprehensive guide, we’ll dive deep into the world of algorithmic interviews, exploring strategies, common problems, and tips to help you excel.
Understanding the Algorithmic/Problem-Solving Interview
Before we delve into the specifics, let’s break down what an algorithmic/problem-solving interview entails:
- Focus: These interviews center on solving algorithmic problems that test your understanding of data structures and algorithms.
- Skills Tested: Interviewers assess your problem-solving abilities, grasp of time and space complexity, and coding proficiency.
- Format: Typically conducted on a whiteboard or in an online coding environment like HackerRank or LeetCode.
- Typical Roles: This interview style is common for software engineers, full-stack developers, and backend developers.
Now that we have a clear picture of what to expect, let’s explore how to prepare and excel in these interviews.
Essential Components of Algorithmic Problem-Solving
1. Data Structures
A solid understanding of data structures is crucial for solving algorithmic problems efficiently. Here are some key data structures you should be familiar with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
Each of these structures has its own strengths and weaknesses, and knowing when to use which one is a crucial skill.
2. Algorithms
Alongside data structures, you need to be well-versed in various algorithmic techniques:
- Sorting algorithms (QuickSort, MergeSort, HeapSort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
- Recursion
Understanding these algorithms and their applications will give you a strong foundation for problem-solving.
3. Time and Space Complexity Analysis
One of the most critical aspects of algorithmic problem-solving is the ability to analyze and optimize the time and space complexity of your solutions. This involves:
- Understanding Big O notation
- Analyzing the worst-case, average-case, and best-case scenarios
- Identifying and eliminating inefficiencies in your code
- Balancing trade-offs between time and space complexity
Being able to discuss the complexity of your solutions demonstrates a deep understanding of algorithmic efficiency.
Preparing for the Interview
Now that we’ve covered the essentials, let’s look at how to prepare effectively for an algorithmic/problem-solving interview.
1. Practice, Practice, Practice
The key to success in these interviews is consistent practice. Here are some ways to hone your skills:
- Online Platforms: Utilize websites like LeetCode, HackerRank, and CodeSignal to practice a wide range of problems.
- Coding Challenges: Participate in coding competitions on platforms like Codeforces or TopCoder to test your skills under time pressure.
- Mock Interviews: Conduct mock interviews with friends or use services like Pramp to simulate real interview conditions.
2. Study Classic Problems and Solutions
Familiarize yourself with classic algorithmic problems and their solutions. Some examples include:
- Two Sum Problem
- Reverse a Linked List
- Implement a Stack using Queues
- Validate a Binary Search Tree
- Find the Shortest Path in a Graph
Understanding these problems and their optimal solutions will help you recognize patterns in new problems you encounter.
3. Master the Art of Problem-Solving
Develop a systematic approach to problem-solving. Here’s a recommended strategy:
- Understand the Problem: Carefully read and analyze the problem statement. Ask clarifying questions if needed.
- Plan Your Approach: Think about potential solutions and choose the most appropriate one.
- Implement the Solution: Write clean, readable code to implement your chosen approach.
- Test and Debug: Run through test cases, including edge cases, to verify your solution.
- Optimize: Consider ways to improve the time and space complexity of your solution.
Common Types of Algorithmic Problems
Let’s explore some common types of problems you might encounter in an algorithmic interview, along with examples and approaches to solve them.
1. Array and String Manipulation
These problems often involve searching, sorting, or manipulating elements in arrays or strings.
Example: Two Sum Problem
Given an array of integers and a target sum, find two numbers in the array that add up to the target.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Example usage
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
This solution uses a hash table to achieve O(n) time complexity.
2. Linked List Problems
These problems test your ability to manipulate linked data structures.
Example: Reverse a Linked List
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
# Example usage
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the linked list
new_head = reverse_linked_list(head)
# Print the reversed list
while new_head:
print(new_head.val, end=" ")
new_head = new_head.next
# Output: 5 4 3 2 1
This iterative solution reverses the linked list in-place with O(n) time complexity and O(1) space complexity.
3. Tree and Graph Problems
These problems often involve traversal, searching, or manipulation of tree or graph structures.
Example: Validate Binary Search Tree
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def is_valid_bst(root):
def validate(node, low=float('-inf'), high=float('inf')):
if not node:
return True
if node.val <= low or node.val >= high:
return False
return (validate(node.left, low, node.val) and
validate(node.right, node.val, high))
return validate(root)
# Example usage
# Create a valid BST: 2 -> (1, 3)
root = TreeNode(2)
root.left = TreeNode(1)
root.right = TreeNode(3)
print(is_valid_bst(root)) # Output: True
# Create an invalid BST: 5 -> (1, 4 -> (3, 6))
root = TreeNode(5)
root.left = TreeNode(1)
root.right = TreeNode(4)
root.right.left = TreeNode(3)
root.right.right = TreeNode(6)
print(is_valid_bst(root)) # Output: False
This recursive solution validates a binary search tree by checking if each node’s value is within the valid range defined by its ancestors.
4. Dynamic Programming
Dynamic programming problems involve breaking down a complex problem into simpler subproblems and storing the results for future use.
Example: Fibonacci Sequence
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
print(fibonacci(10)) # Output: 55
This dynamic programming solution calculates the nth Fibonacci number in O(n) time complexity, avoiding the exponential time complexity of a naive recursive approach.
Tips for Success in Algorithmic Interviews
Now that we’ve covered the types of problems you might encounter, here are some tips to help you succeed in your algorithmic interviews:
1. Think Aloud
Verbalize your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
2. Start with a Brute Force Solution
If you’re stuck, start by explaining a brute force solution. This shows that you understand the problem and gives you a starting point for optimization.
3. Analyze Time and Space Complexity
Always discuss the time and space complexity of your solutions. This demonstrates your understanding of algorithmic efficiency.
4. Consider Edge Cases
Think about and discuss potential edge cases, such as empty inputs, negative numbers, or boundary conditions.
5. Write Clean, Readable Code
Even if you’re writing on a whiteboard, strive for clean, well-organized code. Use meaningful variable names and proper indentation.
6. Test Your Solution
After implementing your solution, walk through it with a few test cases to catch any bugs or edge cases you might have missed.
7. Be Open to Feedback
If the interviewer suggests improvements or alternative approaches, be receptive and engage in a discussion about the pros and cons of different solutions.
Handling Difficult Problems
Sometimes, you might encounter a problem that seems particularly challenging. Here’s how to approach these situations:
1. Break It Down
Try to break the problem into smaller, more manageable subproblems. Solve these subproblems individually and then combine the solutions.
2. Look for Patterns
Many algorithmic problems have underlying patterns. Try to identify if the problem is similar to any classic problems you’ve encountered before.
3. Consider Multiple Approaches
Don’t fixate on a single approach. Consider different data structures or algorithms that might be applicable to the problem.
4. Use Examples
Work through small examples by hand to gain insights into the problem and potential solutions.
5. Ask for Hints
If you’re truly stuck, it’s okay to ask the interviewer for a hint. This shows that you’re engaged with the problem and willing to collaborate.
After the Interview
Once the interview is over, take some time to reflect on your performance:
- Review the problems you encountered and research optimal solutions.
- Identify areas where you struggled and focus on improving those skills.
- Continue practicing regularly, even if you’re not actively interviewing.
Conclusion
Mastering algorithmic/problem-solving interviews is a challenging but rewarding process. By developing a strong foundation in data structures and algorithms, practicing regularly, and honing your problem-solving skills, you can significantly improve your performance in these interviews.
Remember that the goal of these interviews is not just to arrive at the correct solution, but to demonstrate your thought process, problem-solving abilities, and coding skills. Even if you don’t solve every problem perfectly, showing a systematic approach and strong fundamentals can leave a positive impression on your interviewer.
As you continue your journey in software engineering, platforms like AlgoCademy can be invaluable resources. With its focus on interactive coding tutorials, AI-powered assistance, and step-by-step guidance, AlgoCademy can help you progress from beginner-level coding to mastering the skills needed for technical interviews at major tech companies.
Keep practicing, stay curious, and approach each problem as an opportunity to learn and grow. With dedication and the right resources, you’ll be well-prepared to tackle even the most challenging algorithmic interviews. Good luck on your coding journey!