Mastering the 0/1 Knapsack Problem: A Comprehensive Guide
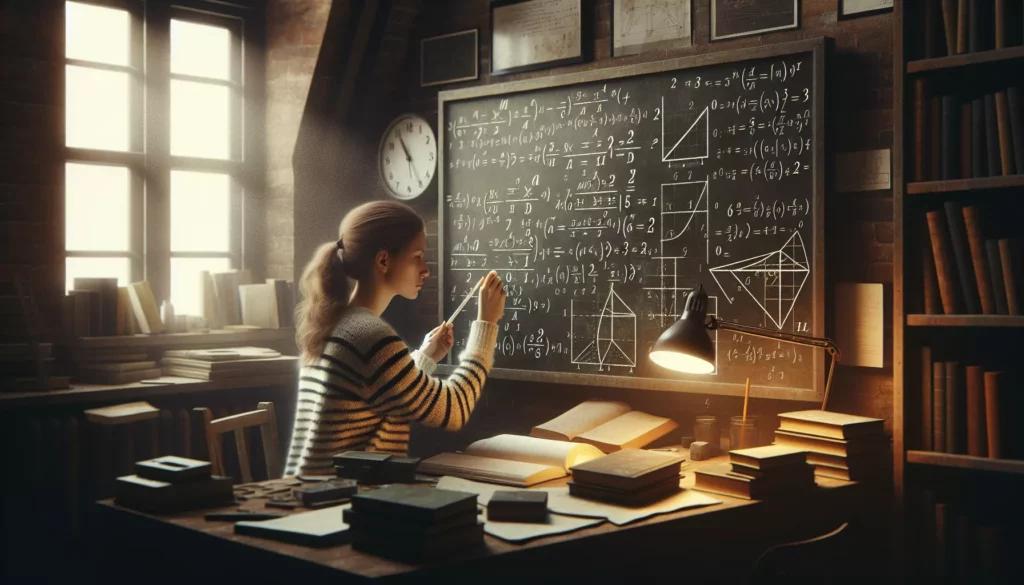
Welcome to our in-depth exploration of the 0/1 Knapsack Problem, a classic algorithmic challenge that has fascinated computer scientists and programmers for decades. This problem is not just an academic exercise; it has real-world applications in fields ranging from finance to logistics. In this comprehensive guide, we’ll break down the problem, explore various solution strategies, and provide practical coding examples to help you master this fundamental concept in computer science.
Table of Contents
- Introduction to the 0/1 Knapsack Problem
- Problem Statement and Formal Definition
- The Naive Approach: Brute Force
- Dynamic Programming Solution
- Top-Down Approach (Memoization)
- Bottom-Up Approach (Tabulation)
- Space Optimization
- Time and Space Complexity Analysis
- Variations of the Knapsack Problem
- Real-World Applications
- Interview Tips and Common Pitfalls
- Conclusion
1. Introduction to the 0/1 Knapsack Problem
The 0/1 Knapsack Problem is a fundamental problem in computer science and optimization. It’s called “0/1” because for each item, you have two choices: take it (1) or leave it (0). This problem is an excellent example of how dynamic programming can be used to solve complex optimization problems efficiently.
Imagine you’re a thief (a very ethical one, of course) who has broken into a store with a knapsack. You can only carry a certain weight, and each item in the store has a weight and a value. Your goal is to fill your knapsack with the most valuable combination of items without exceeding the weight limit.
This problem is NP-complete, which means there’s no known polynomial-time solution for all instances. However, we can solve it efficiently for practical sizes using dynamic programming techniques.
2. Problem Statement and Formal Definition
Let’s formally define the 0/1 Knapsack Problem:
- Given a set of n items numbered from 1 up to n
- Each item i has a weight w[i] and a value v[i]
- Also given is a maximum weight capacity W
The goal is to determine the maximum value subset of the items you can take that will not exceed W weight in total.
Mathematically, we want to maximize:
∑(v[i] * x[i]) for i = 1 to n
Subject to the constraint:
∑(w[i] * x[i]) ≤ W for i = 1 to n
Where x[i] is 0 or 1, representing whether the item is included in the knapsack or not.
3. The Naive Approach: Brute Force
Before diving into more efficient solutions, let’s consider the brute force approach. This method involves generating all possible combinations of items and selecting the one with the highest value that doesn’t exceed the weight limit.
Here’s a Python implementation of the brute force approach:
def knapsack_brute_force(values, weights, W):
n = len(values)
def knapsack_recursive(i, w):
if i == n or w == 0:
return 0
if weights[i] > w:
return knapsack_recursive(i + 1, w)
else:
return max(knapsack_recursive(i + 1, w),
values[i] + knapsack_recursive(i + 1, w - weights[i]))
return knapsack_recursive(0, W)
# Example usage
values = [60, 100, 120]
weights = [10, 20, 30]
W = 50
print(knapsack_brute_force(values, weights, W)) # Output: 220
While this approach works, it has a time complexity of O(2^n), making it impractical for large inputs. This is where dynamic programming comes to the rescue.
4. Dynamic Programming Solution
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems like the 0/1 Knapsack Problem. There are two main approaches to dynamic programming: top-down (memoization) and bottom-up (tabulation).
5. Top-Down Approach (Memoization)
The top-down approach, also known as memoization, involves solving the problem recursively but storing the results of subproblems to avoid redundant calculations. Here’s an implementation:
def knapsack_memoization(values, weights, W):
n = len(values)
memo = {}
def knapsack_recursive(i, w):
if i == n or w == 0:
return 0
if (i, w) in memo:
return memo[(i, w)]
if weights[i] > w:
result = knapsack_recursive(i + 1, w)
else:
result = max(knapsack_recursive(i + 1, w),
values[i] + knapsack_recursive(i + 1, w - weights[i]))
memo[(i, w)] = result
return result
return knapsack_recursive(0, W)
# Example usage
values = [60, 100, 120]
weights = [10, 20, 30]
W = 50
print(knapsack_memoization(values, weights, W)) # Output: 220
This approach significantly improves the time complexity to O(nW) where n is the number of items and W is the knapsack capacity. However, it still uses recursive calls, which can lead to stack overflow for very large inputs.
6. Bottom-Up Approach (Tabulation)
The bottom-up approach, or tabulation, solves the problem iteratively by filling a table with solutions to subproblems. This method is often more efficient than the top-down approach as it avoids the overhead of recursive calls.
def knapsack_tabulation(values, weights, W):
n = len(values)
dp = [[0 for _ in range(W + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, W + 1):
if weights[i-1] <= w:
dp[i][w] = max(dp[i-1][w], values[i-1] + dp[i-1][w-weights[i-1]])
else:
dp[i][w] = dp[i-1][w]
return dp[n][W]
# Example usage
values = [60, 100, 120]
weights = [10, 20, 30]
W = 50
print(knapsack_tabulation(values, weights, W)) # Output: 220
This approach has the same time complexity of O(nW) as the memoization approach, but it’s generally faster in practice due to the lack of recursive calls.
7. Space Optimization
We can optimize the space usage of the bottom-up approach by observing that we only need the previous row to calculate the current row. This allows us to reduce the space complexity from O(nW) to O(W).
def knapsack_space_optimized(values, weights, W):
n = len(values)
dp = [0 for _ in range(W + 1)]
for i in range(n):
for w in range(W, weights[i]-1, -1):
dp[w] = max(dp[w], values[i] + dp[w-weights[i]])
return dp[W]
# Example usage
values = [60, 100, 120]
weights = [10, 20, 30]
W = 50
print(knapsack_space_optimized(values, weights, W)) # Output: 220
This optimization is particularly useful when dealing with large numbers of items or high weight capacities.
8. Time and Space Complexity Analysis
Let’s summarize the time and space complexities of the different approaches we’ve discussed:
- Brute Force:
- Time Complexity: O(2^n)
- Space Complexity: O(n) (due to recursion stack)
- Memoization (Top-Down DP):
- Time Complexity: O(nW)
- Space Complexity: O(nW)
- Tabulation (Bottom-Up DP):
- Time Complexity: O(nW)
- Space Complexity: O(nW)
- Space-Optimized Tabulation:
- Time Complexity: O(nW)
- Space Complexity: O(W)
As we can see, dynamic programming significantly improves the time complexity from exponential to pseudo-polynomial. The space-optimized version provides the best balance of time and space efficiency.
9. Variations of the Knapsack Problem
The 0/1 Knapsack Problem is just one variant of a family of knapsack problems. Here are some other interesting variations:
- Unbounded Knapsack Problem: In this variation, you can take any number of instances of each item, not just 0 or 1.
- Fractional Knapsack Problem: Here, you can take fractions of items, which makes it solvable using a greedy approach.
- Multiple Knapsack Problem: You have multiple knapsacks with different capacities.
- Multidimensional Knapsack Problem: Instead of just weight, there are multiple constraints (e.g., volume, cost).
Each of these variations requires different solving techniques and has its own set of real-world applications.
10. Real-World Applications
The 0/1 Knapsack Problem and its variations have numerous practical applications:
- Finance: Portfolio optimization, where you need to choose the best combination of investments given a limited budget.
- Logistics: Cargo loading, where you need to maximize the value of goods in a container with limited capacity.
- Resource Allocation: Deciding how to allocate limited resources (e.g., time, money) across different projects or tasks.
- Cryptography: The knapsack problem is used in certain cryptographic systems.
- Computer Network Design: Optimizing network layouts given constraints on bandwidth and costs.
Understanding the Knapsack Problem and its solutions can provide valuable insights into solving these real-world optimization challenges.
11. Interview Tips and Common Pitfalls
If you’re preparing for technical interviews, especially for major tech companies, here are some tips related to the 0/1 Knapsack Problem:
- Recognize the problem: The interviewer might present a scenario that’s not explicitly called a “knapsack problem.” Be prepared to recognize the underlying structure.
- Start simple: Begin with the brute force approach to show you understand the problem, then optimize.
- Explain trade-offs: Discuss the time and space complexity trade-offs between different approaches.
- Consider constraints: Ask about the size of the input and any memory constraints, as this can guide your choice of solution.
- Think about related problems: Be prepared to discuss variations of the knapsack problem and how you might approach them.
Common pitfalls to avoid:
- Jumping straight to the optimized solution without explaining your thought process.
- Forgetting to handle edge cases (e.g., when the knapsack capacity is 0 or when there are no items).
- Misunderstanding the problem constraints (e.g., assuming you can take fractions of items in the 0/1 version).
- Not considering the space complexity of your solution, especially with recursive approaches.
12. Conclusion
The 0/1 Knapsack Problem is a classic example of how dynamic programming can be used to solve complex optimization problems efficiently. By breaking down the problem into smaller subproblems and storing their solutions, we can avoid redundant calculations and achieve a much better time complexity than the naive approach.
As we’ve seen, there are multiple ways to approach this problem, each with its own trade-offs in terms of time and space complexity. The choice of approach often depends on the specific constraints of the problem at hand.
Mastering the Knapsack Problem and its variations is not just an academic exercise. It provides valuable insights into problem-solving techniques that can be applied to a wide range of real-world optimization challenges. Whether you’re preparing for technical interviews or working on complex software systems, the principles learned from studying the Knapsack Problem will serve you well.
Remember, the key to solving dynamic programming problems like the Knapsack Problem is to practice regularly and to always think about how you can break down complex problems into simpler, manageable subproblems. Happy coding!