Mastering System Design: A Comprehensive Guide
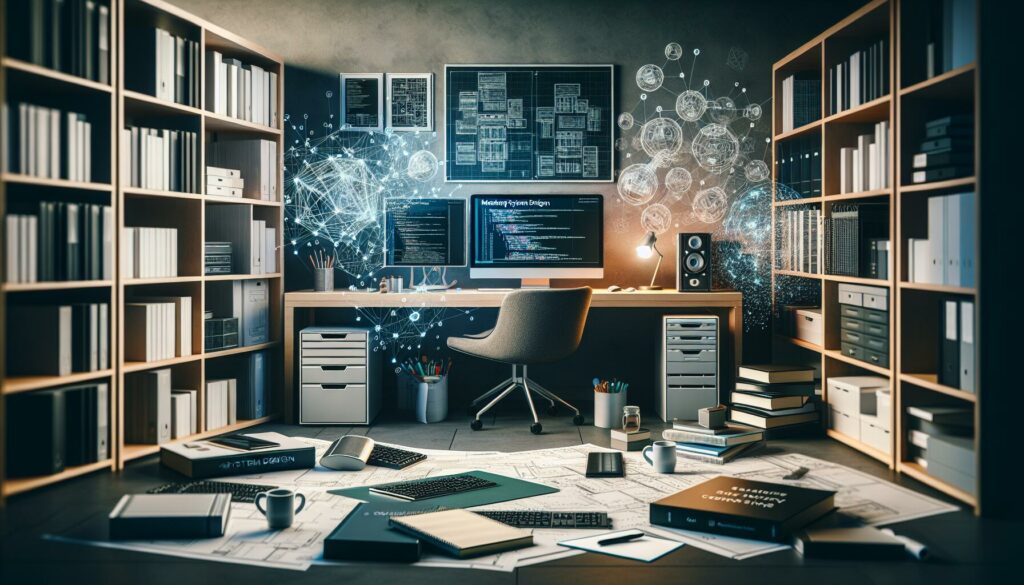
Introduction
In today’s rapidly evolving technological landscape, system design has emerged as a critical skill for software engineers and architects. But what exactly is system design, and why has it become so crucial in the tech industry?
System design is the process of defining the architecture, components, modules, interfaces, and data for a system to satisfy specified requirements. It’s the blueprint that guides the development of complex software systems, ensuring they can handle the demands of modern applications, from scalability and performance to reliability and security.
The importance of system design in tech companies cannot be overstated. As applications grow in complexity and user bases expand, the ability to design robust, scalable systems becomes paramount. Companies like Google, Amazon, and Facebook rely on well-designed systems to serve billions of users every day. This is why system design has become a key component of technical interviews at many top tech firms.
But system design isn’t just about acing interviews; it’s a fundamental skill for real-world software development. As engineers progress in their careers, they’re increasingly expected to contribute to architectural decisions and design systems that can evolve with changing requirements and growing user bases.
So why is system design so challenging? The answer lies in its complexity and the breadth of knowledge it requires. Designing scalable, reliable systems demands a deep understanding of various technologies, architectural patterns, and trade-offs. It’s not just about writing efficient code; it’s about making high-level decisions that impact the entire lifecycle of a software system.
Moreover, there’s often a significant gap between learning algorithms and data structures – the focus of many computer science curricula – and understanding system architecture. While algorithmic skills are crucial, system design requires a broader perspective, considering factors like network latency, data consistency, and fault tolerance.
In this comprehensive guide, we’ll bridge that gap, exploring the core concepts of system design, diving into key components, and examining real-world case studies. Whether you’re preparing for a technical interview or looking to enhance your skills as a software engineer, this guide will provide you with the knowledge and tools to master the art of system design.
1. Core Concepts of System Design
1.1 Scalability
At the heart of system design lies the concept of scalability – the ability of a system to handle growth. As user bases expand and data volumes increase, a well-designed system should be able to accommodate this growth without a proportional increase in resources or degradation in performance.
There are two primary approaches to scaling:
- Vertical Scaling (Scaling Up): This involves adding more power to an existing machine, such as increasing CPU, RAM, or storage. While straightforward, this approach has limits and can be costly.
- Horizontal Scaling (Scaling Out): This involves adding more machines to your pool of resources. It’s generally more cost-effective and offers better fault tolerance, but it introduces complexity in data consistency and distribution.
Techniques to scale systems include:
- Database sharding: Distributing data across multiple machines to handle increased load.
- Caching: Using in-memory data stores to reduce database load and improve response times.
- Asynchronous processing: Offloading time-consuming tasks to background processes to improve responsiveness.
Case Study: Scaling a Web Application
Imagine you’re scaling a popular e-commerce platform. Initially, a single server might handle web requests, application logic, and database queries. As traffic grows, you might first opt for vertical scaling, upgrading the server. However, you’ll eventually hit a ceiling.
At this point, you’d transition to horizontal scaling:
- Implement a load balancer to distribute traffic across multiple web servers.
- Separate the database onto its own server, eventually sharding it across multiple machines.
- Introduce caching layers to reduce database load.
- Use message queues for asynchronous processing of tasks like order fulfillment and email notifications.
1.2 Load Balancing
Load balancing is a critical component in distributed systems, ensuring that incoming network traffic is distributed efficiently across a group of backend servers. It’s essential for improving the availability and responsiveness of applications.
Key load balancing strategies include:
- Round Robin: Requests are distributed sequentially across the server group.
- Least Connection: New requests are sent to the server with the fewest active connections.
- IP Hash: The client’s IP address is used to determine which server receives the request, ensuring that a client always connects to the same server.
Example: Load Balancing a Web Server Cluster
Consider a news website experiencing high traffic during major events. A load balancer could distribute incoming requests across multiple web servers:
- The load balancer sits between clients and the web servers.
- As requests come in, the load balancer forwards them to different servers based on the chosen algorithm.
- If a server goes down, the load balancer redirects traffic to healthy servers, ensuring high availability.
1.3 Caching
Caching is a technique used to store copies of frequently accessed data in a layer that can be retrieved faster than the original source. It’s crucial for improving application performance and reducing database load.
Types of caches include:
- Client-side caching: Browsers can cache static assets like images and CSS files.
- Server-side caching: Application servers can cache database query results or rendered page fragments.
- Content Delivery Networks (CDNs): Distributed networks of servers that cache content closer to end-users.
Use Case: Improving Response Times for Static Content
For a media-heavy website:
- Implement browser caching for static assets, setting appropriate cache-control headers.
- Use a CDN to serve images, videos, and other static files from servers geographically closer to users.
- Implement server-side caching for database queries and API responses.
1.4 Consistency and Availability
In distributed systems, there’s often a trade-off between consistency (all nodes seeing the same data at the same time) and availability (every request receiving a response). This trade-off is formalized in the CAP theorem, which states that in the presence of a network partition, a distributed system can either maintain consistency or availability, but not both simultaneously.
Strong consistency ensures that all clients see the same data at the same time, but it can impact availability and performance. Eventual consistency, on the other hand, allows for temporary inconsistencies but guarantees that all replicas will eventually converge to the same state.
Example: Designing a System that Optimizes for Availability
Consider a social media application where users can post status updates:
- Prioritize availability by allowing users to post updates even if some servers are down.
- Use a multi-master replication setup for the database, allowing writes to any node.
- Implement eventual consistency, where updates are propagated asynchronously to all nodes.
- Use conflict resolution strategies (like vector clocks) to handle simultaneous updates to the same data.
This approach ensures the system remains available for both reads and writes, even in the face of network partitions or server failures, at the cost of potentially showing slightly outdated information to some users for short periods.
2. Key Components of System Design
2.1 Databases and Storage Solutions
Choosing the right database is crucial in system design. The two main categories are SQL (relational) and NoSQL databases, each with its strengths and use cases.
SQL Databases:
- Ideal for structured data with complex relationships
- Support ACID transactions
- Examples: PostgreSQL, MySQL
NoSQL Databases:
- Designed for unstructured or semi-structured data
- Often more scalable and flexible
- Examples: MongoDB (document store), Cassandra (wide-column store), Redis (key-value store)
Partitioning and Sharding:
Partitioning involves splitting a database into smaller, more manageable parts. Sharding is a specific type of partitioning that distributes data across multiple machines.
Replication:
Replication creates and maintains copies of data across different nodes, improving availability and read performance.
Use Case Comparison:
- E-commerce Platform:
- Choose PostgreSQL for its ACID compliance, crucial for financial transactions
- Implement sharding based on customer ID for horizontal scaling
- Use read replicas to handle high-volume product catalog queries
- Real-time Analytics System:
- Opt for Cassandra for its ability to handle high write throughput
- Leverage Cassandra’s built-in partitioning for scalability
- Implement eventual consistency model to prioritize availability
2.2 Message Queues and Pub/Sub Systems
Message queues and publish-subscribe (pub/sub) systems are essential for building loosely coupled, scalable applications. They enable asynchronous communication between different parts of a system.
When to use message queues:
- Decoupling components of a system
- Handling background jobs
- Implementing event-driven architectures
Popular message queue systems:
- Apache Kafka: High-throughput distributed messaging system
- RabbitMQ: Feature-rich message broker supporting multiple protocols
- AWS SQS: Fully managed message queuing service
Example: Designing a Distributed Task Queue
Consider a video processing application that needs to handle user uploads and transcode videos into multiple formats:
- Use AWS SQS as the message queue
- When a user uploads a video, push a message to the queue with video details
- Have multiple worker instances listening to the queue
- Workers pick up messages, process videos, and update the database with results
- Implement dead-letter queues for handling failed processing attempts
This design allows for easy scaling of video processing capacity and ensures that the upload process remains responsive even under heavy load.
2.3 Microservices Architecture
Microservices architecture is an approach to developing a single application as a suite of small services, each running in its own process and communicating with lightweight mechanisms, often HTTP/REST APIs.
Monolithic vs Microservices:
- Monolithic: Entire application is a single, tightly-coupled unit
- Microservices: Application is composed of loosely-coupled, independently deployable services
Communication between microservices:
- REST APIs: Simple, stateless communication over HTTP
- gRPC: High-performance RPC framework using protocol buffers
Pros of Microservices:
- Independent scaling of services
- Technology diversity (different services can use different tech stacks)
- Faster deployment and easier maintenance
Cons of Microservices:
- Increased complexity in service discovery and orchestration
- Challenges in maintaining data consistency across services
- Potentially increased latency due to network calls between services
2.4 APIs and Endpoints
Well-designed APIs are crucial for system integration and scalability. They define how different components of a system or different systems interact with each other.
RESTful API Design Principles:
- Use HTTP methods correctly (GET for retrieval, POST for creation, etc.)
- Use nouns, not verbs, in endpoint paths
- Use hierarchy to represent relationships
- Use query parameters for filtering, sorting, and pagination
- Use proper HTTP status codes to indicate request outcomes
GraphQL vs REST:
- REST: Multiple endpoints, each returning fixed data structures
- GraphQL: Single endpoint, clients specify exactly what data they need
Strengths of REST:
- Simplicity and wide adoption
- Caching can be implemented easily
- Suitable for most CRUD operations
Strengths of GraphQL:
- Clients can request exactly the data they need
- Reduces over-fetching and under-fetching of data
- Strongly typed schema
Designing Scalable APIs for Heavy Traffic:
- Implement rate limiting to prevent abuse
- Use caching aggressively (e.g., Redis for application-level caching)
- Consider using a CDN for frequently accessed, static responses
- Implement pagination for large result sets
- Use asynchronous processing for time-consuming operations
3. Designing Large-Scale Systems
3.1 Case Study 1: Designing a URL Shortener
Let’s walk through the process of designing a URL shortener service similar to bit.ly or tinyurl.com.
Requirements Gathering:
- Functional Requirements:
- Given a long URL, generate a unique short URL
- When users access the short URL, redirect to the original long URL
- Allow users to create custom short URLs (optional)
- Track click statistics (optional)
- Non-Functional Requirements:
- High availability (the service should be up 99.9% of the time)
- Low latency for URL redirection
- The system should scale to handle millions of URLs
System Architecture:
- API Layer:
- REST API endpoints for URL shortening and redirection
- Implement rate limiting to prevent abuse
- Application Layer:
- URL shortening service: Generates short codes and handles custom URLs
- URL redirection service: Looks up long URLs and performs redirects
- Database Layer:
- Choose a NoSQL database like Cassandra for its high write throughput
- Schema: {short_url, long_url, user_id, creation_date, expiration_date}
- Cache Layer:
- Use Redis to cache frequently accessed URL mappings
Database Choice:
Cassandra is chosen for its ability to handle high write loads and its natural support for consistent hashing, which aids in sharding.
Load Balancing:
Implement a load balancer (e.g., NGINX) in front of the application servers to distribute traffic evenly.
Caching Strategy:
- When a URL is shortened, store the mapping in both Cassandra and Redis
- For URL redirection, first check Redis. If not found, query Cassandra and update Redis
Handling High Traffic and Scaling Issues:
- Use consistent hashing to shard the database based on the short URL
- Implement a CDN to handle redirection for the most popular URLs
- Use read replicas of the database to handle high read loads
- Implement auto-scaling for the application servers based on traffic patterns
3.2 Case Study 2: Designing a Social Media Feed
Designing a social media feed presents unique challenges due to its high write/read ratio and the need for real-time updates. Let’s design a system similar to Twitter’s home timeline.
Challenges:
- High volume of tweets (writes)
- Even higher volume of feed reads
- Need for real-time feed updates
- Complex relationships (following/followers)
System Architecture:
- Data Ingestion:
- Write API for posting tweets
- Fanout service to propagate tweets to followers’ timelines
- Storage:
- Tweet Storage: NoSQL database like Cassandra
- User Graph: Graph database like Neo4j
- Timeline Storage: Redis for active users, Cassandra for long-term storage
- Feed Generation:
- Timeline service to compile and serve user feeds
- Push notifications for real-time updates
Data Modeling:
- Tweet Object:
{
tweet_id: unique identifier,
user_id: author's ID,
content: tweet text,
media_urls: array of media links,
timestamp: creation time,
likes: count,
retweets: count
}
- User Graph:
Store follower relationships in Neo4j for efficient traversal - Timeline:
Sorted set in Redis, with tweet IDs as members and timestamps as scores
Efficient Data Modeling for Timelines:
- Home Timeline:
- Store recent tweets (e.g., last 1000) from followed users in Redis
- Use a background job to periodically merge this with older tweets in Cassandra
- User Timeline:
- Store in Cassandra, partitioned by user_id
- Use materialized views in Cassandra for efficient reads
Scaling Databases:
- Shard tweet storage in Cassandra based on tweet_id
- Partition the user graph in Neo4j based on user_id
- For Redis, use a cluster to distribute timeline data across multiple nodes
Caching Strategies:
- Cache hot users’ timelines in Redis
- Use a distributed cache like Memcached for frequently accessed tweets and user profiles
Real-time Updates:
- Use WebSockets for real-time feed updates to active users
- Implement a pub/sub system (e.g., Apache Kafka) to propagate new tweets to relevant services
3.3 Case Study 3: Designing a Video Streaming Platform
Designing a video streaming platform like YouTube or Netflix involves handling large files, efficient content delivery, and managing real-time data. Let’s break down the key components and strategies.
Challenges:
- Storing and serving large video files
- Efficient content delivery across different geographical locations
- Handling different video qualities and formats
- Managing user data and recommendations
- Scaling to millions of concurrent viewers
System Architecture:
- Content Ingestion:
- Upload API for content creators
- Transcoding service to convert videos into multiple formats and qualities
- Storage:
- Object storage (e.g., Amazon S3) for video files
- Relational database (e.g., PostgreSQL) for user data, video metadata
- NoSQL database (e.g., Cassandra) for user activity, recommendations
- Content Delivery:
- Content Delivery Network (CDN) for efficient global distribution
- Edge servers for caching popular content
- Streaming Service:
- Adaptive Bitrate Streaming to adjust video quality based on user’s connection
- Support for multiple streaming protocols (HLS, DASH)
- User Interface:
- Web application
- Mobile apps (iOS, Android)
- Smart TV apps
Handling Large Files and Content Delivery:
- Chunked Upload:
- Break large video files into smaller chunks for upload
- Allows for pause/resume functionality and better error handling
- Transcoding:
- Use a distributed transcoding system to convert uploaded videos into multiple formats and qualities
- Store different versions in object storage
- Content Delivery Network (CDN):
- Use a global CDN to cache and serve video content from locations closer to the end-user
- Implement geo-based routing to direct users to the nearest CDN edge server
Use of CDNs and Encoding Systems:
- CDN Strategy:
- Push popular content to CDN edge servers proactively
- Use pull strategy for less popular content (edge servers request from origin when needed)
- Encoding System:
- Implement a distributed encoding system using technologies like FFmpeg
- Use a queue system (e.g., RabbitMQ) to manage encoding jobs
- Support adaptive bitrate streaming by creating multiple quality versions of each video
Streaming Protocols and Real-time Data Management:
- Streaming Protocols:
- Use HLS (HTTP Live Streaming) and DASH (Dynamic Adaptive Streaming over HTTP) for broad device compatibility
- Implement low-latency extensions for live streaming scenarios
- Real-time Data Management:
- Use WebSockets for real-time features like live chat during streams
- Implement a pub/sub system (e.g., Redis Pub/Sub) for propagating real-time events
- Analytics and Recommendations:
- Use a stream processing system like Apache Flink for real-time analytics
- Implement a recommendation system using collaborative filtering and content-based filtering techniques
Scaling Considerations:
- Horizontal Scaling:
- Scale out transcoding servers to handle increased upload load
- Use auto-scaling groups for streaming servers based on concurrent viewer metrics
- Database Scaling:
- Shard the database based on user ID or video ID
- Use read replicas for handling high read loads on video metadata
- Caching:
- Implement multi-layer caching (CDN, Application layer, Database layer)
- Use Redis for caching user sessions, video metadata, and recommendation lists
This design allows for efficient handling of large video files, global content delivery, and scalability to support millions of concurrent viewers.
4. Performance Optimization and Monitoring
4.1 Performance Optimization Techniques
Optimizing system performance is crucial for maintaining user satisfaction and managing resources efficiently. Here are some key techniques:
Profiling and Identifying Bottlenecks:
- Use Application Performance Management (APM) tools like New Relic or Datadog
- Implement distributed tracing to understand request flow across microservices
- Use flame graphs to visualize CPU and memory usage
Database Optimizations:
- Indexing:
- Create appropriate indexes based on common query patterns
- Be cautious of over-indexing, which can slow down write operations
- Query Optimization:
- Use EXPLAIN to analyze query execution plans
- Optimize slow queries by rewriting or adding appropriate indexes
- Consider denormalization for read-heavy workloads
- Connection Pooling:
- Implement connection pooling to reduce the overhead of creating new database connections
Optimizing Network Performance:
- Minimize HTTP Requests:
- Use CSS sprites to combine multiple images
- Concatenate and minify CSS and JavaScript files
- Implement HTTP/2:
- Enables multiplexing, header compression, and server push
- Use Content Delivery Networks (CDNs):
- Distribute static assets globally to reduce latency
Reducing Latency:
- Implement Caching:
- Use in-memory caches like Redis for frequently accessed data
- Implement browser caching for static assets
- Asynchronous Processing:
- Use message queues to handle time-consuming tasks asynchronously
- Database Read Replicas:
- Direct read queries to replicas to reduce load on the primary database
4.2 Monitoring and Alerting Systems
Effective monitoring is essential for maintaining the health and performance of large-scale systems. It enables teams to detect and respond to issues quickly, often before they impact users.
Importance of Observability:
Observability goes beyond basic monitoring, providing deep insights into system behavior. It typically encompasses three pillars:
- Metrics: Quantitative data about system performance
- Logs: Detailed records of events within the system
- Traces: Information about request flows through distributed systems
Tools for Monitoring:
- Prometheus:
- Open-source monitoring and alerting toolkit
- Pull-based metrics collection model
- Powerful query language (PromQL) for data analysis
- Grafana:
- Open-source platform for monitoring and observability
- Supports multiple data sources (including Prometheus)
- Provides rich visualization options and alerting capabilities
- ELK Stack (Elasticsearch, Logstash, Kibana):
- Elasticsearch: Search and analytics engine
- Logstash: Data processing pipeline
- Kibana: Visualization platform for Elasticsearch data
Example: Setting up Monitoring for a Microservices Architecture
- Metrics Collection:
- Deploy Prometheus server to scrape metrics from services
- Implement custom metrics in services using Prometheus client libraries
- Visualization:
- Set up Grafana dashboards to visualize key metrics:
- Request rates, error rates, and latencies
- Resource utilization (CPU, memory, disk, network)
- Business-specific metrics (e.g., active users, transaction volume)
- Log Management:
- Use Filebeat to ship logs from services to Logstash
- Process and enrich logs with Logstash
- Store logs in Elasticsearch for easy searching and analysis
- Alerting:
- Configure Grafana or Prometheus Alertmanager to send notifications for critical issues
- Set up on-call rotations using tools like PagerDuty
- Distributed Tracing:
- Implement distributed tracing using Jaeger or Zipkin
- Trace requests across services to identify performance bottlenecks
4.3 Autoscaling and Fault Tolerance
Autoscaling allows systems to automatically adjust resources based on demand, while fault tolerance ensures systems can continue operating despite failures.
Autoscaling in Cloud Environments:
- AWS Auto Scaling:
- Supports scaling based on various metrics (CPU utilization, request count, custom metrics)
- Can be used with EC2 instances, ECS tasks, DynamoDB tables, and more
- Google Cloud Autoscaler:
- Supports both horizontal (instance count) and vertical (machine type) scaling
- Can scale based on CPU utilization, load balancing capacity, or custom metrics
- Azure Autoscale:
- Supports scaling for App Service, Virtual Machine Scale Sets, and other services
- Can scale based on metrics or on a schedule
Strategies for Building Fault-Tolerant Systems:
- Circuit Breakers:
- Detect failures and encapsulate logic for preventing a failure from constantly recurring
- Example: Use Hystrix library in Java applications
- Retry Mechanisms:
- Implement exponential backoff and jitter for retrying failed operations
- Be cautious of retry storms in distributed systems
- Redundancy:
- Deploy services across multiple availability zones or regions
- Implement database replication for data redundancy
- Graceful Degradation:
- Design systems to provide reduced functionality when some components fail
- Prioritize critical features during partial system failures
Use Case: Autoscaling a Web Application to Handle Traffic Spikes
Scenario: An e-commerce platform experiencing daily traffic patterns and occasional marketing-driven spikes.
Solution:
- Infrastructure Setup:
- Deploy the application across multiple AWS availability zones
- Use Amazon EC2 for application servers behind an Elastic Load Balancer
- Auto Scaling Configuration:
- Create an Auto Scaling group for EC2 instances
- Set up scaling policies based on average CPU utilization and request count
- Database Scaling:
- Use Amazon RDS with read replicas for the relational database
- Implement a caching layer with Amazon ElastiCache (Redis) to reduce database load
- Fault Tolerance:
- Implement circuit breakers for external service calls
- Use Amazon SQS for decoupling components and ensuring message persistence
- Monitoring and Alerting:
- Use Amazon CloudWatch for monitoring metrics and setting up alarms
- Configure alerts for scaling events and potential issues
This setup allows the system to automatically handle daily traffic fluctuations and scale rapidly during unexpected traffic spikes, while maintaining fault tolerance and performance.
5. Security in System Design
5.1 Security Considerations in Distributed Systems
Security is a critical aspect of system design, especially in distributed systems where there are more potential points of vulnerability. Here are some key security considerations:
Common Security Threats:
- Distributed Denial of Service (DDoS) Attacks:
- Use cloud-based DDoS protection services
- Implement rate limiting and traffic analysis
- Data Breaches:
- Encrypt sensitive data at rest and in transit
- Implement proper access controls and authentication mechanisms
- Man-in-the-Middle (MITM) Attacks:
- Use SSL/TLS for all communications
- Implement certificate pinning in mobile apps
Implementing SSL/TLS:
- Use strong, up-to-date TLS versions (TLS 1.2 or 1.3)
- Properly configure server-side SSL settings
- Implement HSTS (HTTP Strict Transport Security) to prevent downgrade attacks
Secure API Design:
- Use OAuth 2.0 or OpenID Connect for authentication and authorization
- Implement rate limiting to prevent abuse
- Validate and sanitize all input to prevent injection attacks
- Use API keys or JWT (JSON Web Tokens) for API authentication
5.2 Authentication and Authorization
Designing scalable and secure authentication systems is crucial for protecting user data and system resources.
OAuth 2.0 and OpenID Connect:
- OAuth 2.0 is an authorization framework that allows applications to obtain limited access to user accounts on an HTTP service
- OpenID Connect is an identity layer on top of OAuth 2.0, adding authentication capabilities
JSON Web Tokens (JWT):
- Stateless authentication mechanism
- Encodes claims in JSON format
- Signed to ensure integrity
Single Sign-On (SSO):
- Allows users to access multiple applications with a single set of credentials
- Improves user experience and simplifies credential management
Role-Based Access Control (RBAC) vs Attribute-Based Access Control (ABAC):
- RBAC:
- Access decisions are based on the roles assigned to users
- Simpler to implement and manage for smaller systems
- Example: Admin, Editor, Viewer roles
- ABAC:
- Access decisions based on attributes of the user, resource, and environment
- More flexible and granular than RBAC
- Example: Allow access if (user.department == “Finance” AND resource.type == “Financial Report” AND time.isBetween(9AM, 5PM))
5.3 Data Privacy and Compliance
Ensuring data privacy and compliance with regulations is increasingly important in system design.
Data Encryption:
- Encryption at Rest:
- Use strong encryption algorithms (e.g., AES-256) for stored data
- Properly manage encryption keys, potentially using a key management service
- Encryption in Transit:
- Use TLS for all network communications
- Implement end-to-end encryption for highly sensitive data
Handling GDPR and Data Compliance:
- Data Minimization:
- Collect and retain only necessary data
- Implement data retention policies
- User Consent:
- Obtain and manage user consent for data collection and processing
- Provide mechanisms for users to withdraw consent
- Data Portability:
- Design systems to allow easy export of user data in a common format
- Right to be Forgotten:
- Implement mechanisms to completely delete user data upon request
Use Case: Designing a Secure Payment System for E-commerce
- PCI DSS Compliance:
- Ensure compliance with Payment Card Industry Data Security Standard
- Use a PCI-compliant payment gateway to minimize direct handling of card data
- Tokenization:
- Replace sensitive card data with tokens for storage and processing
- Encryption:
- Implement end-to-end encryption for payment transactions
- Use Hardware Security Modules (HSMs) for cryptographic operations
- Authentication:
- Implement multi-factor authentication for user accounts
- Use 3D Secure for additional verification of card transactions
- Audit Logging:
- Maintain detailed logs of all payment-related activities
- Ensure logs are securely stored and cannot be tampered with
- Secure Communication:
- Use TLS 1.2 or higher for all communications
- Implement certificate pinning in mobile apps to prevent MITM attacks
- Fraud Detection:
- Implement real-time fraud detection systems using machine learning
- Set up alerts for suspicious activities
By implementing these security measures, the e-commerce platform can provide a secure payment environment, protect user data, and maintain compliance with relevant regulations.
6. Handling Real-World Constraints and Trade-offs
6.1 Dealing with Latency and Throughput Constraints
In real-world systems, latency and throughput are often key performance indicators that need careful optimization.
Optimizing for Low-Latency Systems:
- Reduce Network Hops:
- Collocate related services
- Use CDNs to bring content closer to users
- Optimize Database Queries:
- Use appropriate indexes
- Implement query caching
- Caching Strategies:
- Implement multi-level caching (client-side, CDN, application layer, database layer)
- Use read-through and write-through caching patterns
- Asynchronous Processing:
- Use message queues for non-critical, time-consuming tasks
- Implement server-sent events or WebSockets for real-time updates
Understanding Network Limitations:
- Bandwidth Considerations:
- Optimize payload sizes (compression, minimization)
- Implement lazy loading for web applications
- Data Center Strategies:
- Use multiple data centers for geographical distribution
- Implement intelligent routing to direct users to the nearest data center
Case Study: Designing a Low-Latency Messaging System
Requirements:
- Support millions of concurrent users
- Deliver messages in near real-time (< 100ms)
- Support one-to-one and group messaging
Architecture:
- Connection Management:
- Use WebSockets for persistent connections
- Implement a connection pool to manage WebSocket connections
- Message Routing:
- Use a pub/sub system (e.g., Redis Pub/Sub or Apache Kafka) for message distribution
- Implement a routing layer to determine message recipients
- Storage:
- Use a distributed NoSQL database (e.g., Cassandra) for message persistence
- Implement a caching layer for recent messages
- Load Balancing:
- Use DNS-based load balancing for initial connection distribution
- Implement application-layer load balancing for WebSocket connections
- Optimizations:
- Use protocol buffers for efficient message serialization
- Implement message batching for group messages
This architecture allows for low-latency message delivery while handling high throughput and maintaining scalability.
6.2 Trade-offs Between Consistency, Availability, and Partition Tolerance
The CAP theorem states that in a distributed system, you can only guarantee two out of three properties: Consistency, Availability, and Partition Tolerance. Understanding these trade-offs is crucial for designing robust distributed systems.
Revisiting the CAP Theorem:
- Consistency: All nodes see the same data at the same time
- Availability: Every request receives a response, without guarantee that it contains the most recent version
- Availability: Every request receives a response, without guarantee that it contains the most recent version of the information
- Partition Tolerance: The system continues to operate despite arbitrary partitioning due to network failures
In practice, partition tolerance is necessary for distributed systems, so the real trade-off is often between consistency and availability.
Real-World Examples:
- Cassandra (AP System):
- Prioritizes availability and partition tolerance
- Uses eventual consistency model
- Suitable for use cases where high availability is critical and some inconsistency can be tolerated (e.g., social media status updates)
- Google Spanner (CP System):
- Prioritizes consistency and partition tolerance
- Uses TrueTime API and atomic clocks for global consistency
- Suitable for use cases requiring strong consistency (e.g., financial transactions)
Trade-offs When Designing Distributed Databases:
- Strong Consistency vs. Performance:
- Strong consistency often requires synchronous replication, which can increase latency
- Eventual consistency allows for asynchronous replication, improving performance at the cost of temporary inconsistencies
- Availability vs. Consistency:
- Highly available systems might serve stale data during network partitions
- Strongly consistent systems might become unavailable during partitions to avoid serving inconsistent data
- Scalability vs. Consistency:
- Strongly consistent systems often have limits on write scalability
- Eventually consistent systems can typically scale writes more easily
Example: Designing a Distributed E-commerce Inventory System
Requirements:
- Track inventory across multiple warehouses
- Handle high volume of concurrent orders
- Maintain accurate inventory counts to prevent overselling
Solution:
- Use a multi-master database setup (e.g., Cassandra) for high availability and write scalability
- Implement eventual consistency for inventory updates across warehouses
- Use optimistic locking for order processing to handle concurrent orders
- Implement a reservation system to temporarily hold inventory during the checkout process
- Use periodic reconciliation jobs to correct any inconsistencies
This design prioritizes availability and partition tolerance over strong consistency, which is often acceptable for inventory systems where small discrepancies can be managed operationally.
7. Tips for System Design Interviews
7.1 How to Approach System Design Problems in Interviews
System design interviews can be challenging due to their open-ended nature. Here’s a structured approach to tackle these problems effectively:
- Clarify Requirements (2-3 minutes):
- Ask questions to understand the problem scope
- Identify functional and non-functional requirements
- Establish scale (number of users, data volume, etc.)
- Sketch the High-Level Design (5-10 minutes):
- Draw the main components of the system
- Identify key services and data stores
- Show how components interact
- Deep Dive into Core Components (10-15 minutes):
- Choose 2-3 core components to elaborate on
- Discuss data models, APIs, and algorithms
- Address scalability and performance considerations
- Identify and Address Bottlenecks (5-10 minutes):
- Discuss potential system bottlenecks
- Propose solutions (caching, load balancing, etc.)
- Consider failure scenarios and how to handle them
- Summarize and Discuss Trade-offs (3-5 minutes):
- Recap the main design decisions
- Discuss alternative approaches and their trade-offs
- Show openness to feedback and ability to iterate on the design
Focusing on Scalability, Reliability, and Trade-offs:
- Scalability:
- Discuss both vertical and horizontal scaling options
- Consider database sharding and caching strategies
- Address read vs. write scalability separately
- Reliability:
- Discuss redundancy and fault tolerance
- Consider data replication strategies
- Address how the system handles various failure scenarios
- Trade-offs:
- Discuss consistency vs. availability trade-offs
- Consider performance vs. cost trade-offs
- Address simplicity vs. flexibility in the design
Communicating Your Design Effectively:
- Use Clear Diagrams:
- Draw neat, easy-to-understand system diagrams
- Use standard symbols for different components (e.g., cylinders for databases, rectangles for services)
- Explain Your Reasoning:
- Articulate why you’re making certain design choices
- Discuss alternatives you considered
- Be Collaborative:
- Treat the interview as a discussion, not a test
- Be open to suggestions and feedback from the interviewer
- Manage Time Effectively:
- Keep an eye on the clock and pace yourself
- If running out of time, mention areas you would like to discuss further if there was more time
7.2 Common Mistakes to Avoid in System Design Interviews
- Diving into Details Too Quickly:
- Mistake: Starting to code or discussing low-level implementation details immediately
- Better Approach: Start with a high-level design and gradually add details
- Neglecting to Clarify Requirements:
- Mistake: Making assumptions about the system requirements without verifying
- Better Approach: Ask clarifying questions to understand the problem scope and constraints
- Ignoring Scalability:
- Mistake: Designing a system that works for small scale but doesn’t address growth
- Better Approach: Always consider how the system will scale and handle increased load
- Overlooking Data Consistency and Integrity:
- Mistake: Not addressing how data will remain consistent across distributed systems
- Better Approach: Discuss consistency models and how to ensure data integrity
- Failing to Consider Failure Scenarios:
- Mistake: Designing for the happy path only
- Better Approach: Discuss how the system handles various failure modes and recovers from them
- Not Justifying Design Decisions:
- Mistake: Making design choices without explaining the rationale
- Better Approach: Clearly articulate why you’re choosing certain technologies or approaches
- Sticking to a Single Solution:
- Mistake: Not considering alternative approaches or being inflexible
- Better Approach: Discuss trade-offs between different solutions and be open to alternatives
- Neglecting Non-Functional Requirements:
- Mistake: Focusing solely on functionality and ignoring aspects like performance, security, and maintainability
- Better Approach: Address both functional and non-functional requirements in your design
Conclusion
Mastering system design is a journey that requires continuous learning and practical experience. The landscape of technologies and best practices is always evolving, making it an exciting and challenging field.
Key Takeaways:
- Start with the Basics: Understand core concepts like scalability, load balancing, and caching thoroughly.
- Learn from Real-World Systems: Study how large-scale systems are built and operated by tech giants.
- Practice Regularly: Work on design problems, contribute to open-source projects, or build your own systems.
- Stay Updated: Keep abreast of new technologies, architectural patterns, and industry best practices.
- Understand Trade-offs: There’s rarely a perfect solution in system design. Learn to evaluate and communicate trade-offs effectively.
- Focus on Scalability and Reliability: Design systems that can grow and remain resilient under various conditions.
- Consider Security and Privacy: In today’s digital landscape, these aspects are crucial for any system.
- Communicate Effectively: The ability to articulate your design decisions clearly is as important as the technical knowledge itself.
Remember, system design is not just about creating a blueprint for software systems. It’s about solving real-world problems at scale, considering various constraints and requirements. Whether you’re preparing for interviews or designing systems in your job, the principles and approaches discussed in this guide will serve as a solid foundation.
As you continue your journey in system design, don’t hesitate to dive deeper into specific areas that interest you or are relevant to your work. The field is vast, and there’s always more to learn and explore.
Happy designing!
References
- Designing Data-Intensive Applications by Martin Kleppmann
- System Design Interview – An Insider’s Guide by Alex Xu
- Building Microservices by Sam Newman
- Web Scalability for Startup Engineers by Artur Ejsmont
- Designing Distributed Systems by Brendan Burns
- The System Design Primer (GitHub repository) by Donne Martin
- High Scalability Blog (highscalability.com)
- Netflix Tech Blog (netflixtechblog.com)
- AWS Architecture Center (aws.amazon.com/architecture)
- Google Cloud Architecture Center (cloud.google.com/architecture)
Remember to stay curious, keep practicing, and never stop learning. The world of system design is vast and ever-evolving, offering endless opportunities for growth and innovation.