Mastering Subset Problems: Strategies and Techniques for Efficient Problem-Solving
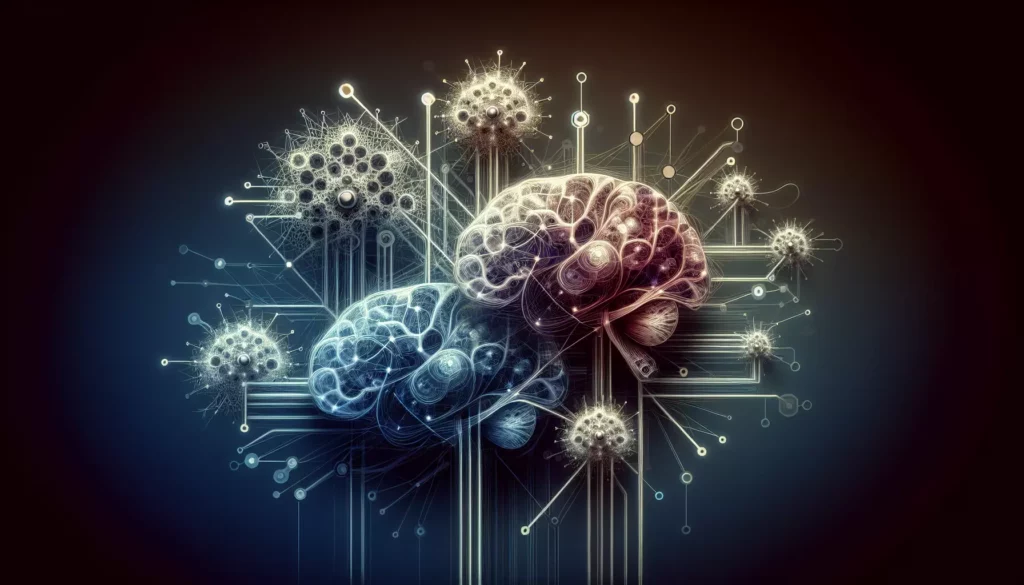
In the realm of algorithmic problem-solving, subset problems stand out as a fundamental category that frequently appears in coding interviews and competitive programming challenges. These problems involve working with combinations of elements from a given set, often requiring efficient solutions to handle large datasets. In this comprehensive guide, we’ll explore various strategies and techniques for solving subset problems, providing you with the tools to tackle these challenges confidently.
Understanding Subset Problems
Before diving into specific strategies, it’s crucial to understand what subset problems are and why they’re important in computer science and programming interviews.
What are Subset Problems?
Subset problems involve finding or manipulating subsets of a given set of elements. A subset is a collection of elements that are part of a larger set. For example, given the set {1, 2, 3}, its subsets include {}, {1}, {2}, {3}, {1, 2}, {1, 3}, {2, 3}, and {1, 2, 3}.
Why are Subset Problems Important?
Subset problems are essential for several reasons:
- They test a programmer’s ability to think combinatorially and handle complex logic.
- They often require optimized solutions, challenging candidates to consider time and space complexity.
- Many real-world problems can be modeled as subset problems, making them practical for various applications.
- They are a favorite topic in technical interviews, especially for positions at major tech companies.
Common Types of Subset Problems
Subset problems come in various forms. Here are some common types you might encounter:
1. Generate All Subsets
This problem involves generating all possible subsets of a given set. It’s a fundamental problem that forms the basis for many other subset-related challenges.
2. Subset Sum
Given a set of integers and a target sum, find a subset of the integers that add up to the target sum. This problem has many variations and is often used as a building block for more complex problems.
3. Combination Sum
Similar to the subset sum problem, but elements can be used multiple times. The goal is to find all unique combinations that sum up to a target value.
4. Power Set
Generate the power set of a given set, which includes all possible subsets, including the empty set and the set itself.
5. Partition Problem
Determine if a given set can be partitioned into two subsets with equal sum.
Strategies for Solving Subset Problems
Now that we’ve covered the basics, let’s dive into some powerful strategies for tackling subset problems efficiently.
1. Recursive Backtracking
Recursive backtracking is a versatile technique that’s particularly useful for generating all subsets or finding specific subsets that meet certain criteria.
How it works:
- Start with an empty subset.
- For each element in the set, make two recursive calls:
- One including the current element in the subset.
- One excluding the current element from the subset.
- Use a base case to stop the recursion (e.g., when all elements have been considered).
Example: Generating all subsets
def generate_subsets(nums):
def backtrack(start, current):
result.append(current[:])
for i in range(start, len(nums)):
current.append(nums[i])
backtrack(i + 1, current)
current.pop()
result = []
backtrack(0, [])
return result
# Example usage
nums = [1, 2, 3]
subsets = generate_subsets(nums)
print(subsets)
# Output: [[], [1], [1, 2], [1, 2, 3], [1, 3], [2], [2, 3], [3]]
This recursive backtracking approach generates all possible subsets by making decisions to include or exclude each element.
2. Bit Manipulation
Bit manipulation is an efficient technique for generating subsets, especially when working with small sets. It leverages the binary representation of numbers to create subsets.
How it works:
- Represent each subset as a binary number, where each bit corresponds to an element in the set.
- A ‘1’ bit means the element is included in the subset, while a ‘0’ bit means it’s excluded.
- Generate all numbers from 0 to 2^n – 1, where n is the number of elements in the set.
- For each number, create a subset based on its binary representation.
Example: Generating all subsets using bit manipulation
def generate_subsets_bit(nums):
n = len(nums)
subsets = []
for i in range(1 << n): # 2^n
subset = []
for j in range(n):
if i & (1 << j):
subset.append(nums[j])
subsets.append(subset)
return subsets
# Example usage
nums = [1, 2, 3]
subsets = generate_subsets_bit(nums)
print(subsets)
# Output: [[], [1], [2], [1, 2], [3], [1, 3], [2, 3], [1, 2, 3]]
This bit manipulation approach is concise and efficient, especially for small sets.
3. Dynamic Programming
Dynamic programming is a powerful technique for solving subset problems, particularly when dealing with optimization or counting problems.
How it works:
- Break down the problem into smaller subproblems.
- Store the results of subproblems to avoid redundant calculations.
- Build up the solution to the original problem using the results of subproblems.
Example: Subset Sum Problem using Dynamic Programming
def subset_sum(nums, target):
n = len(nums)
dp = [[False] * (target + 1) for _ in range(n + 1)]
# Initialize base cases
for i in range(n + 1):
dp[i][0] = True
# Fill the dp table
for i in range(1, n + 1):
for j in range(1, target + 1):
if j < nums[i-1]:
dp[i][j] = dp[i-1][j]
else:
dp[i][j] = dp[i-1][j] or dp[i-1][j-nums[i-1]]
return dp[n][target]
# Example usage
nums = [3, 34, 4, 12, 5, 2]
target = 9
result = subset_sum(nums, target)
print(result) # Output: True (because 4 + 5 = 9)
This dynamic programming solution efficiently solves the subset sum problem by building a table of subproblems.
4. Two Pointers Technique
The two pointers technique is useful for subset problems that involve searching or manipulating sorted arrays.
How it works:
- Use two pointers to track different positions in the array.
- Move the pointers based on certain conditions to find the desired subset or solution.
Example: Finding a subset with a given sum in a sorted array
def find_subset_with_sum(nums, target):
nums.sort() # Ensure the array is sorted
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [nums[left], nums[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return None # No subset found
# Example usage
nums = [1, 2, 3, 4, 5]
target = 7
result = find_subset_with_sum(nums, target)
print(result) # Output: [2, 5]
This two-pointer approach efficiently finds a subset with a given sum in a sorted array.
5. Meet in the Middle
The meet in the middle technique is useful for problems where a brute force approach would be too slow, but the input size is still too large for standard dynamic programming solutions.
How it works:
- Divide the input set into two halves.
- Generate all subsets for each half.
- Combine the results from both halves to find the solution.
Example: Subset Sum Problem using Meet in the Middle
from itertools import combinations
def subset_sum_meet_in_middle(nums, target):
n = len(nums)
mid = n // 2
def generate_subset_sums(arr):
sums = []
for i in range(len(arr) + 1):
for subset in combinations(arr, i):
sums.append(sum(subset))
return sorted(sums)
left_sums = generate_subset_sums(nums[:mid])
right_sums = generate_subset_sums(nums[mid:])
for left in left_sums:
complement = target - left
if complement in right_sums:
return True
return False
# Example usage
nums = [3, 34, 4, 12, 5, 2]
target = 9
result = subset_sum_meet_in_middle(nums, target)
print(result) # Output: True
This meet in the middle approach can handle larger inputs compared to the standard dynamic programming solution for the subset sum problem.
Advanced Techniques and Optimizations
As you become more comfortable with the basic strategies, consider these advanced techniques and optimizations to further improve your subset problem-solving skills:
1. Pruning in Recursive Approaches
When using recursive backtracking, you can often improve efficiency by pruning branches of the recursion tree that won’t lead to valid solutions.
Example: Optimized Combination Sum
def combination_sum(candidates, target):
def backtrack(start, target, path):
if target == 0:
result.append(path[:])
return
for i in range(start, len(candidates)):
if candidates[i] > target:
break # Pruning: no need to continue if candidate is larger than remaining target
path.append(candidates[i])
backtrack(i, target - candidates[i], path)
path.pop()
candidates.sort() # Sort for efficient pruning
result = []
backtrack(0, target, [])
return result
# Example usage
candidates = [2, 3, 6, 7]
target = 7
result = combination_sum(candidates, target)
print(result) # Output: [[2, 2, 3], [7]]
This optimized version sorts the candidates and uses pruning to avoid unnecessary recursive calls.
2. Bitmask Dynamic Programming
Bitmask DP combines the power of dynamic programming with efficient bit manipulation, often leading to more concise and faster solutions for subset problems.
Example: Traveling Salesman Problem using Bitmask DP
def tsp(graph):
n = len(graph)
all_visited = (1 << n) - 1
dp = [[float('inf')] * n for _ in range(1 << n)]
dp[1][0] = 0 # Start at node 0
for mask in range(1, 1 << n):
for u in range(n):
if mask & (1 << u):
for v in range(n):
if mask & (1 << v) and u != v:
dp[mask][u] = min(dp[mask][u], dp[mask ^ (1 << u)][v] + graph[v][u])
return min(dp[all_visited][i] + graph[i][0] for i in range(1, n))
# Example usage
graph = [
[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]
]
result = tsp(graph)
print(result) # Output: 80
This bitmask DP solution efficiently solves the Traveling Salesman Problem, a classic subset problem.
3. Iterative Subset Generation
While recursive approaches are intuitive, iterative methods can sometimes be more efficient and avoid stack overflow for large inputs.
Example: Iterative Power Set Generation
def power_set_iterative(nums):
power_set = [[]]
for num in nums:
power_set += [subset + [num] for subset in power_set]
return power_set
# Example usage
nums = [1, 2, 3]
result = power_set_iterative(nums)
print(result)
# Output: [[], [1], [2], [1, 2], [3], [1, 3], [2, 3], [1, 2, 3]]
This iterative approach generates the power set efficiently without recursion.
Common Pitfalls and How to Avoid Them
When solving subset problems, be aware of these common pitfalls:
1. Overlooking Base Cases
In recursive solutions, forgetting to handle base cases properly can lead to infinite recursion or incorrect results.
Solution: Always start by clearly defining and implementing base cases before moving on to the recursive steps.
2. Inefficient Subset Generation
Generating subsets inefficiently can lead to time limit exceeded errors in coding challenges.
Solution: Use bit manipulation or iterative approaches for efficient subset generation, especially for larger sets.
3. Not Considering Duplicates
When working with sets that contain duplicates, naive subset generation can lead to duplicate subsets.
Solution: Sort the input and skip duplicate elements during subset generation, or use a set data structure to store unique subsets.
4. Ignoring Space Complexity
While focusing on time complexity, it’s easy to overlook space complexity, which can be a significant factor in subset problems.
Solution: Consider space-efficient solutions, such as iterative approaches or in-place modifications when possible.
Practice Problems and Resources
To master subset problems, practice is key. Here are some recommended problems and resources:
LeetCode Problems:
Additional Resources:
- GeeksforGeeks: Subset Sum Problem
- TopCoder: Dynamic Programming from Novice to Advanced
- CSES Problem Set (contains various subset and related problems)
Conclusion
Mastering subset problems is a crucial skill for any programmer aiming to excel in algorithmic problem-solving and coding interviews. By understanding the various strategies and techniques presented in this guide, you’ll be well-equipped to tackle a wide range of subset problems efficiently.
Remember that the key to mastery is practice. Start with simpler problems and gradually work your way up to more complex challenges. As you practice, focus on understanding the underlying principles and patterns, rather than memorizing specific solutions.
With dedication and consistent practice, you’ll find that subset problems become not just manageable, but an opportunity to showcase your problem-solving skills and algorithmic thinking. Keep honing your skills, and you’ll be well-prepared for any subset problem that comes your way in coding interviews or competitive programming contests.