Mastering Subarray Problem-Solving Techniques: A Comprehensive Guide
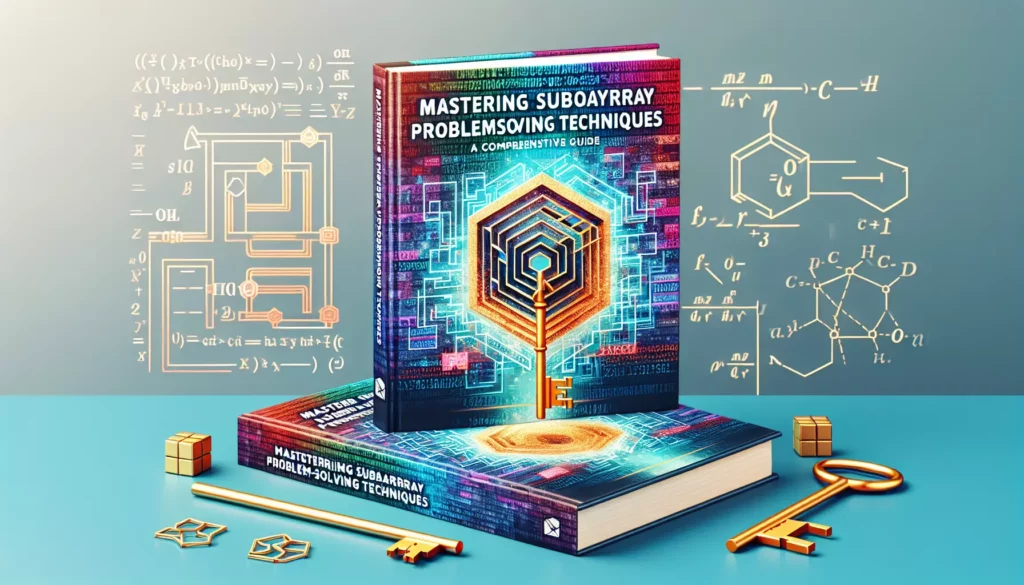
Welcome to our in-depth guide on techniques for solving subarray problems! If you’re preparing for technical interviews or looking to enhance your algorithmic problem-solving skills, you’ve come to the right place. Subarray problems are a common type of coding challenge that frequently appear in interviews at top tech companies, including FAANG (Facebook, Amazon, Apple, Netflix, and Google). In this comprehensive tutorial, we’ll explore various techniques and strategies to tackle these problems efficiently.
Table of Contents
- Introduction to Subarray Problems
- Brute Force Approach
- Sliding Window Technique
- Kadane’s Algorithm
- Prefix Sum Technique
- Two Pointers Technique
- Divide and Conquer Approach
- Dynamic Programming for Subarray Problems
- Advanced Techniques and Data Structures
- Practice Problems and Solutions
- Conclusion and Next Steps
1. Introduction to Subarray Problems
Before we dive into the techniques, let’s first understand what subarray problems are and why they’re important in coding interviews.
What is a Subarray?
A subarray is a contiguous part of an array. For example, given the array [1, 2, 3, 4], some of its subarrays are [1], [1, 2], [2, 3, 4], and [1, 2, 3, 4]. Note that [1, 3] is not a subarray as it’s not contiguous.
Common Types of Subarray Problems
- Finding the maximum/minimum sum subarray
- Finding the longest subarray with a specific property
- Counting subarrays that satisfy certain conditions
- Optimizing a value over all possible subarrays
These problems test your ability to think algorithmically and optimize for time and space complexity, making them crucial for technical interviews.
2. Brute Force Approach
The brute force approach is the most straightforward way to solve subarray problems. It involves generating all possible subarrays and checking each one for the required condition.
How It Works
- Use nested loops to generate all possible subarrays
- For each subarray, compute the required value or check the condition
- Keep track of the best result found so far
Example: Maximum Subarray Sum
Let’s implement the brute force approach for finding the maximum subarray sum:
def max_subarray_sum_brute_force(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
for j in range(i, n):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum_brute_force(arr)) # Output: 6
Time and Space Complexity
The brute force approach has a time complexity of O(n³) for this problem, as we have three nested operations: two loops and the sum calculation. The space complexity is O(1) as we only use a constant amount of extra space.
Pros and Cons
Pros:
- Simple to implement and understand
- Works for all subarray problems
Cons:
- Very inefficient for large inputs
- Not suitable for most interview scenarios due to poor time complexity
3. Sliding Window Technique
The sliding window technique is a powerful method for solving subarray problems, especially when dealing with contiguous sequences. It’s particularly useful when you need to maintain a running calculation over a specific window of elements.
How It Works
- Define a window with a start and end point
- Slide the window over the array, usually from left to right
- Adjust the window size based on certain conditions
- Maintain relevant information about the elements in the current window
Types of Sliding Windows
- Fixed-size window: The window size remains constant throughout the iteration.
- Variable-size window: The window size can grow or shrink based on certain conditions.
Example: Maximum Sum Subarray of Size K
Let’s implement a solution to find the maximum sum subarray of a fixed size K:
def max_sum_subarray_size_k(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
print(max_sum_subarray_size_k(arr, k)) # Output: 39
Time and Space Complexity
The sliding window approach typically has a time complexity of O(n), where n is the length of the array. The space complexity is usually O(1) as we only maintain a constant amount of extra information.
When to Use Sliding Window
The sliding window technique is particularly useful for problems involving:
- Contiguous subarrays or subsequences
- Problems asking for optimization over a specific window size
- Scenarios where you need to maintain a running calculation
4. Kadane’s Algorithm
Kadane’s algorithm is a classic dynamic programming approach used to solve the maximum subarray sum problem in linear time. It’s an excellent example of how dynamic programming can simplify and optimize solutions to subarray problems.
How It Works
- Iterate through the array, keeping track of the maximum sum encountered so far
- For each element, decide whether to start a new subarray or extend the existing one
- Update the global maximum sum if the current sum is greater
Implementation
Here’s an implementation of Kadane’s algorithm in Python:
def kadanes_algorithm(arr):
max_ending_here = max_so_far = arr[0]
for num in arr[1:]:
max_ending_here = max(num, max_ending_here + num)
max_so_far = max(max_so_far, max_ending_here)
return max_so_far
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(kadanes_algorithm(arr)) # Output: 6
Time and Space Complexity
Kadane’s algorithm has a time complexity of O(n) and a space complexity of O(1), making it highly efficient for large inputs.
Variations and Extensions
Kadane’s algorithm can be modified to solve various related problems, such as:
- Finding the maximum sum subarray with at least K elements
- Finding the maximum product subarray
- Handling circular arrays
5. Prefix Sum Technique
The prefix sum technique is a powerful tool for efficiently solving a wide range of subarray problems, especially those involving sum calculations over ranges.
How It Works
- Create a prefix sum array where each element is the sum of all previous elements in the original array
- Use the prefix sum array to calculate sums of subarrays in constant time
Implementation
Here’s how to create a prefix sum array:
def create_prefix_sum(arr):
prefix_sum = [0] * (len(arr) + 1)
for i in range(1, len(prefix_sum)):
prefix_sum[i] = prefix_sum[i-1] + arr[i-1]
return prefix_sum
# Example usage
arr = [1, 2, 3, 4, 5]
prefix_sum = create_prefix_sum(arr)
print(prefix_sum) # Output: [0, 1, 3, 6, 10, 15]
Using Prefix Sum for Range Sum Queries
To find the sum of elements from index i to j (inclusive), you can use:
def range_sum(prefix_sum, i, j):
return prefix_sum[j+1] - prefix_sum[i]
# Example usage
print(range_sum(prefix_sum, 1, 3)) # Sum of arr[1:4], Output: 9
Time and Space Complexity
Creating the prefix sum array takes O(n) time and O(n) space. After that, range sum queries can be answered in O(1) time.
Applications
The prefix sum technique is useful for:
- Range sum queries
- Finding subarrays with a given sum
- Optimizing cumulative calculations over subarrays
6. Two Pointers Technique
The two pointers technique is a simple and effective way to solve many subarray problems, especially those involving searching or optimization over contiguous sequences.
How It Works
- Initialize two pointers, usually at the start of the array
- Move the pointers based on certain conditions
- Process the subarray defined by the two pointers
Types of Two Pointer Approaches
- Same direction: Both pointers move in the same direction, usually from left to right.
- Opposite directions: Pointers start at opposite ends and move towards each other.
Example: Subarray with Given Sum
Let’s implement a solution to find a subarray with a given sum using the two pointers technique:
def find_subarray_with_sum(arr, target_sum):
left = right = current_sum = 0
while right < len(arr):
current_sum += arr[right]
while current_sum > target_sum and left < right:
current_sum -= arr[left]
left += 1
if current_sum == target_sum:
return arr[left:right+1]
right += 1
return None # No subarray found
# Example usage
arr = [1, 4, 20, 3, 10, 5]
target_sum = 33
result = find_subarray_with_sum(arr, target_sum)
print(result) # Output: [20, 3, 10]
Time and Space Complexity
The two pointers technique typically has a time complexity of O(n) and a space complexity of O(1), making it very efficient for many subarray problems.
When to Use Two Pointers
The two pointers technique is particularly useful for:
- Finding subarrays with specific properties
- Searching for pairs in a sorted array
- Partitioning arrays
7. Divide and Conquer Approach
The divide and conquer approach is a powerful problem-solving technique that breaks down a problem into smaller subproblems, solves them recursively, and then combines the results to solve the original problem.
How It Works
- Divide: Break the problem into smaller subproblems
- Conquer: Recursively solve the subproblems
- Combine: Merge the solutions of the subproblems to create a solution to the original problem
Example: Maximum Subarray Sum using Divide and Conquer
Let’s implement the maximum subarray sum problem using the divide and conquer approach:
def max_crossing_sum(arr, low, mid, high):
left_sum = float('-inf')
sum = 0
for i in range(mid, low-1, -1):
sum += arr[i]
if sum > left_sum:
left_sum = sum
right_sum = float('-inf')
sum = 0
for i in range(mid+1, high+1):
sum += arr[i]
if sum > right_sum:
right_sum = sum
return left_sum + right_sum
def max_subarray_sum(arr, low, high):
if low == high:
return arr[low]
mid = (low + high) // 2
return max(max_subarray_sum(arr, low, mid),
max_subarray_sum(arr, mid+1, high),
max_crossing_sum(arr, low, mid, high))
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum(arr, 0, len(arr)-1)) # Output: 6
Time and Space Complexity
The time complexity of this divide and conquer approach is O(n log n), and the space complexity is O(log n) due to the recursive call stack.
Advantages and Disadvantages
Advantages:
- Can be more intuitive for some problems
- Often leads to efficient parallel algorithms
Disadvantages:
- May not always be the most efficient approach
- Can be more complex to implement than iterative solutions
8. Dynamic Programming for Subarray Problems
Dynamic Programming (DP) is a powerful technique for solving optimization problems by breaking them down into simpler subproblems. It’s particularly useful for many subarray problems where we need to find optimal solutions over different ranges.
Key Concepts in Dynamic Programming
- Optimal Substructure: The optimal solution to the problem contains optimal solutions to subproblems.
- Overlapping Subproblems: The same subproblems are solved multiple times.
Approaches to Dynamic Programming
- Top-down (Memoization): Start with the main problem and recursively break it down, storing results to avoid redundant calculations.
- Bottom-up (Tabulation): Start with the smallest subproblems and work your way up to the main problem, typically using an array to store results.
Example: Longest Increasing Subarray
Let’s solve the problem of finding the length of the longest increasing subarray using dynamic programming:
def longest_increasing_subarray(arr):
n = len(arr)
if n == 0:
return 0
dp = [1] * n # Initialize DP array with 1s
max_length = 1
for i in range(1, n):
if arr[i] > arr[i-1]:
dp[i] = dp[i-1] + 1
max_length = max(max_length, dp[i])
return max_length
# Example usage
arr = [1, 3, 5, 4, 7]
print(longest_increasing_subarray(arr)) # Output: 3
Time and Space Complexity
The time complexity of this DP solution is O(n), and the space complexity is also O(n) due to the DP array.
When to Use Dynamic Programming
Dynamic Programming is particularly useful for subarray problems that involve:
- Optimization over different ranges
- Counting problems
- Problems with overlapping subproblems
9. Advanced Techniques and Data Structures
As you progress in your problem-solving journey, you’ll encounter more complex subarray problems that require advanced techniques and data structures. Here are some advanced concepts that can be applied to subarray problems:
Segment Trees
Segment Trees are versatile data structures that allow for efficient range queries and updates on arrays. They’re particularly useful for problems involving multiple range operations.
Key Features:
- Supports range sum, minimum, maximum queries in O(log n) time
- Allows for updates in O(log n) time
- Requires O(n) space
Fenwick Trees (Binary Indexed Trees)
Fenwick Trees are efficient data structures for handling range sum queries and point updates in arrays.
Key Features:
- Supports range sum queries in O(log n) time
- Allows for point updates in O(log n) time
- More space-efficient than Segment Trees, requiring O(n) space
Monotonic Stack/Queue
Monotonic stacks and queues are powerful tools for solving problems involving finding the nearest smaller/greater elements or maintaining a sliding window minimum/maximum.
Key Applications:
- Finding the next greater/smaller element
- Solving sliding window minimum/maximum problems
- Optimizing certain types of dynamic programming problems
Example: Next Greater Element using Monotonic Stack
Here’s an implementation of finding the next greater element for each element in an array using a monotonic stack:
def next_greater_element(arr):
n = len(arr)
result = [-1] * n
stack = []
for i in range(n-1, -1, -1):
while stack and stack[-1] <= arr[i]:
stack.pop()
if stack:
result[i] = stack[-1]
stack.append(arr[i])
return result
# Example usage
arr = [4, 5, 2, 25]
print(next_greater_element(arr)) # Output: [5, 25, 25, -1]
Sparse Table
Sparse Tables are data structures used for efficient range queries on static arrays, particularly for associative and idempotent functions like minimum, maximum, and GCD.
Key Features:
- Preprocessing time: O(n log n)
- Query time: O(1) for idempotent functions
- Space complexity: O(n log n)
10. Practice Problems and Solutions
To reinforce your understanding of subarray problem-solving techniques, here are some practice problems along with brief solution outlines:
1. Maximum Product Subarray
Problem: Given an integer array nums, find a contiguous non-empty subarray within the array that has the largest product, and return the product.
Solution Outline: Use a variation of Kadane’s algorithm, keeping track of both the maximum and minimum product ending at each position.
2. Subarray Sum Equals K
Problem: Given an array of integers nums and an integer k, return the total number of continuous subarrays whose sum equals to k.
Solution Outline: Use the prefix sum technique with a hash map to count the number of subarrays efficiently.
3. Longest Subarray with Sum Divisible by K
Problem: Given an array of integers and an integer k, find the length of the longest subarray with sum divisible by k.
Solution Outline: Use the prefix sum technique with modular arithmetic and a hash map to store the first occurrence of each remainder.
4. Sliding Window Maximum
Problem: Given an array nums and a sliding window of size k moving from the very left of the array to the very right, return the maximum for each window.
Solution Outline: Use a deque (double-ended queue) to maintain a monotonic decreasing queue of indices within the current window.
5. Shortest Subarray with Sum at Least K
Problem: Return the length of the shortest, non-empty, contiguous subarray of nums with sum at least k. If there is no such subarray, return -1.
Solution Outline: Use a monotonic queue with the prefix sum technique to efficiently find the shortest subarray.
These problems cover a range of techniques we’ve discussed, from simple sliding window to more advanced data structures like monotonic queues. Practice implementing these solutions to strengthen your subarray problem-solving skills.
11. Conclusion and Next Steps
Congratulations on making it through this comprehensive guide on techniques for solving subarray problems! Let’s recap what we’ve covered and discuss where to go from here.
Key Takeaways
- Diverse Techniques: We’ve explored a wide range of techniques, from simple brute force to advanced data structures like segment trees and monotonic stacks.
- Efficiency Matters: Many subarray problems have efficient solutions that significantly outperform naive approaches. Always consider time and space complexity.
- Pattern Recognition: With practice, you’ll start recognizing patterns in subarray problems that hint at which technique to use.
- Adaptability: Often, you’ll need to combine or modify these techniques to solve complex problems.
Next Steps
- Practice Regularly: Solve subarray problems on platforms like LeetCode, HackerRank, or CodeForces to reinforce your skills.
- Analyze Solutions: After solving a problem, look at other solutions to learn different approaches and optimizations.
- Mock Interviews: Practice explaining your thought process and coding solutions in mock interview settings.
- Explore Related Topics: Dive deeper into dynamic programming, graph algorithms, and other advanced topics that often intersect with subarray problems.
- Real-world Applications: Look for opportunities to apply these techniques in real-world programming scenarios or projects.
Final Thoughts
Mastering subarray problem-solving techniques is a valuable skill that will serve you well in coding interviews and beyond. Remember that proficiency comes with practice and patience. Don’t be discouraged if some problems seem challenging at first – with time and effort, you’ll develop the intuition to tackle even the most complex subarray problems.
Keep coding, stay curious, and happy problem-solving!