Mastering String Searching Algorithms: Techniques for Efficient Searching
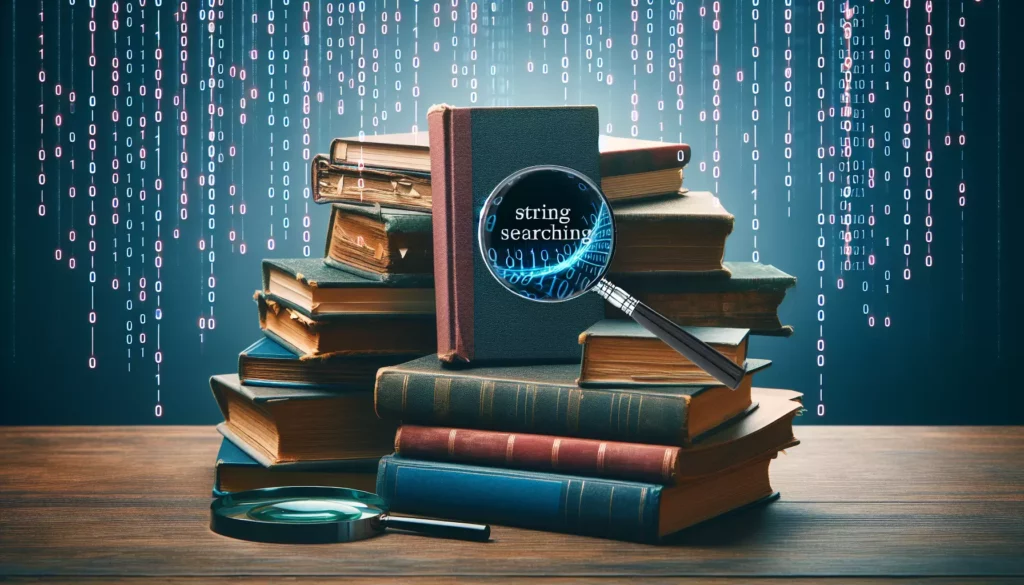
In the world of computer science and programming, efficient string searching algorithms play a crucial role in various applications, from text editors to search engines. As aspiring programmers and those preparing for technical interviews at major tech companies, understanding these algorithms is essential. In this comprehensive guide, we’ll explore various techniques for efficient string searching, their implementations, and their applications in real-world scenarios.
1. Introduction to String Searching
String searching, also known as string matching, is the process of finding occurrences of a pattern string within a larger text string. This fundamental operation is used in numerous applications, including:
- Text editors for find and replace functionality
- Search engines for locating web pages containing specific keywords
- Bioinformatics for DNA sequence analysis
- Spam filters for identifying unwanted emails
- Plagiarism detection systems
The efficiency of string searching algorithms is crucial, especially when dealing with large datasets or real-time applications. In this article, we’ll discuss several techniques, ranging from naive approaches to more sophisticated algorithms that offer improved performance.
2. Naive String Searching Algorithm
The naive approach, also known as the brute-force method, is the simplest string searching algorithm. It involves checking for a match at every possible position in the text.
How it works:
- Align the pattern at the beginning of the text.
- Compare characters of the pattern with the text one by one.
- If all characters match, a pattern occurrence is found.
- If a mismatch occurs, shift the pattern one position to the right and repeat steps 2-4.
Implementation in Python:
def naive_search(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
j = 0
while j < m and text[i + j] == pattern[j]:
j += 1
if j == m:
print(f"Pattern found at index {i}")
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
naive_search(text, pattern)
While simple to implement, the naive approach has a time complexity of O(nm) in the worst case, where n is the length of the text and m is the length of the pattern. This can be inefficient for large texts or patterns.
3. Knuth-Morris-Pratt (KMP) Algorithm
The Knuth-Morris-Pratt (KMP) algorithm improves upon the naive approach by utilizing information about the pattern itself to avoid unnecessary comparisons. It preprocesses the pattern to create a failure function, which helps in skipping characters that are known not to match.
Key concepts:
- Prefix function: Computes the longest proper prefix that is also a suffix for each prefix of the pattern.
- Partial match table: Stores the values of the prefix function for efficient lookup during the search process.
How it works:
- Preprocess the pattern to create the partial match table.
- Perform the search by comparing characters and using the partial match table to skip unnecessary comparisons.
Implementation in Python:
def kmp_search(text, pattern):
n = len(text)
m = len(pattern)
# Compute the partial match table
lps = [0] * m
length = 0
i = 1
while i < m:
if pattern[i] == pattern[length]:
length += 1
lps[i] = length
i += 1
elif length != 0:
length = lps[length - 1]
else:
lps[i] = 0
i += 1
# Perform the search
i = j = 0
while i < n:
if pattern[j] == text[i]:
i += 1
j += 1
if j == m:
print(f"Pattern found at index {i - j}")
j = lps[j - 1]
elif i < n and pattern[j] != text[i]:
if j != 0:
j = lps[j - 1]
else:
i += 1
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
kmp_search(text, pattern)
The KMP algorithm has a time complexity of O(n + m), which is a significant improvement over the naive approach, especially for large texts and patterns.
4. Boyer-Moore Algorithm
The Boyer-Moore algorithm is considered one of the most efficient string searching algorithms in practice. It uses two main heuristics to improve performance: the bad character heuristic and the good suffix heuristic.
Key concepts:
- Bad character heuristic: Skips alignments where the mismatched character in the text doesn’t occur in the pattern.
- Good suffix heuristic: Uses information about the occurrence of suffixes in the pattern to skip alignments.
How it works:
- Preprocess the pattern to create the bad character and good suffix tables.
- Start comparing characters from right to left in the pattern.
- If a mismatch occurs, use the maximum of the shifts suggested by the two heuristics.
Implementation in Python:
def boyer_moore_search(text, pattern):
n = len(text)
m = len(pattern)
# Preprocessing
bad_char = {c: max(1, m - pattern.index(c) - 1) for c in set(pattern)}
# Searching
i = 0
while i <= n - m:
j = m - 1
while j >= 0 and pattern[j] == text[i + j]:
j -= 1
if j < 0:
print(f"Pattern found at index {i}")
i += m
else:
i += max(1, j - bad_char.get(text[i + j], m))
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
boyer_moore_search(text, pattern)
The Boyer-Moore algorithm has a best-case time complexity of O(n/m) and a worst-case time complexity of O(nm). In practice, it often outperforms other string searching algorithms, especially for large alphabets and long patterns.
5. Rabin-Karp Algorithm
The Rabin-Karp algorithm uses hashing to find pattern matches in the text. It calculates hash values for the pattern and substrings of the text, comparing them to identify potential matches.
Key concepts:
- Rolling hash: Efficiently computes hash values for substrings of the text.
- Modular arithmetic: Used to prevent integer overflow in hash calculations.
How it works:
- Compute the hash value of the pattern.
- Compute the hash value of the first m characters of the text.
- Compare hash values and check for exact matches if they’re equal.
- Use the rolling hash to efficiently update the hash value for the next text window.
Implementation in Python:
def rabin_karp_search(text, pattern):
n = len(text)
m = len(pattern)
d = 256 # Number of characters in the alphabet
q = 101 # A prime number for modular arithmetic
# Compute hash values
pattern_hash = 0
text_hash = 0
h = pow(d, m - 1) % q
for i in range(m):
pattern_hash = (d * pattern_hash + ord(pattern[i])) % q
text_hash = (d * text_hash + ord(text[i])) % q
# Searching
for i in range(n - m + 1):
if pattern_hash == text_hash:
if text[i:i+m] == pattern:
print(f"Pattern found at index {i}")
if i < n - m:
text_hash = (d * (text_hash - ord(text[i]) * h) + ord(text[i + m])) % q
if text_hash < 0:
text_hash += q
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
rabin_karp_search(text, pattern)
The Rabin-Karp algorithm has an average and best-case time complexity of O(n + m), but its worst-case time complexity is O(nm). It’s particularly useful when searching for multiple patterns simultaneously.
6. Aho-Corasick Algorithm
The Aho-Corasick algorithm is designed for efficient multi-pattern string matching. It constructs a finite state machine from the set of patterns and uses it to locate all occurrences of any pattern in the text in a single pass.
Key concepts:
- Trie data structure: Represents the set of patterns efficiently.
- Failure links: Connect states in the trie to handle mismatches.
- Output function: Identifies matched patterns at each state.
How it works:
- Construct a trie from the set of patterns.
- Add failure links to the trie.
- Compute the output function for each state.
- Traverse the text using the constructed automaton to find matches.
Implementation in Python:
from collections import deque
class AhoCorasick:
def __init__(self, patterns):
self.patterns = patterns
self.trie = {}
self.failure = {}
self.output = {}
self.build_trie()
self.build_failure_and_output()
def build_trie(self):
for i, pattern in enumerate(self.patterns):
node = self.trie
for char in pattern:
node = node.setdefault(char, {})
node["#"] = i
def build_failure_and_output(self):
queue = deque(self.trie.values())
while queue:
node = queue.popleft()
for char, child in node.items():
if char != "#":
queue.append(child)
failure = self.trie
while True:
failure = failure.get(char)
if failure is not None:
self.failure[child] = failure
self.output[child] = self.output.get(failure, [])
if "#" in failure:
self.output[child].append(failure["#"])
break
if failure is self.trie:
self.failure[child] = self.trie
break
def search(self, text):
node = self.trie
results = []
for i, char in enumerate(text):
while char not in node and node is not self.trie:
node = self.failure[node]
if char in node:
node = node[char]
if "#" in node:
results.append((i - len(self.patterns[node["#"]]) + 1, node["#"]))
if node in self.output:
for pattern_index in self.output[node]:
results.append((i - len(self.patterns[pattern_index]) + 1, pattern_index))
return results
patterns = ["ABABC", "BABC", "ABC"]
text = "ABABDABACDABABCABAB"
ac = AhoCorasick(patterns)
results = ac.search(text)
for index, pattern_index in results:
print(f"Pattern '{patterns[pattern_index]}' found at index {index}")
The Aho-Corasick algorithm has a time complexity of O(n + m + z), where n is the length of the text, m is the total length of all patterns, and z is the number of pattern occurrences in the text. It’s particularly efficient for searching multiple patterns simultaneously.
7. Suffix Array and Longest Common Prefix (LCP) Array
Suffix arrays and LCP arrays are powerful data structures that can be used for efficient string searching, especially when multiple queries need to be performed on the same text.
Key concepts:
- Suffix array: An array of all suffixes of a string, sorted lexicographically.
- LCP array: Stores the length of the longest common prefix between adjacent suffixes in the suffix array.
How it works:
- Construct the suffix array for the text.
- Build the LCP array.
- Use binary search on the suffix array to find pattern occurrences.
Implementation in Python:
class SuffixArray:
def __init__(self, text):
self.text = text
self.n = len(text)
self.suffixes = sorted(range(self.n), key=lambda i: text[i:])
self.lcp = self._build_lcp()
def _build_lcp(self):
lcp = [0] * self.n
k = 0
for i in range(self.n - 1):
j = self.suffixes[self.rank[i] - 1]
while i + k < self.n and j + k < self.n and self.text[i + k] == self.text[j + k]:
k += 1
lcp[self.rank[i] - 1] = k
k = max(k - 1, 0)
return lcp
@property
def rank(self):
return [0] * self.n if not hasattr(self, '_rank') else self._rank
@rank.setter
def rank(self, value):
self._rank = value
def search(self, pattern):
m = len(pattern)
left, right = 0, self.n
while left < right:
mid = (left + right) // 2
suffix = self.text[self.suffixes[mid]:]
if suffix[:m] < pattern:
left = mid + 1
else:
right = mid
start = left
right = self.n
while left < right:
mid = (left + right) // 2
suffix = self.text[self.suffixes[mid]:]
if suffix[:m] <= pattern:
left = mid + 1
else:
right = mid
end = left
return [self.suffixes[i] for i in range(start, end)]
text = "ABABDABACDABABCABAB$"
sa = SuffixArray(text)
pattern = "ABABC"
results = sa.search(pattern)
print(f"Pattern '{pattern}' found at indices: {results}")
The suffix array construction has a time complexity of O(n log n), where n is the length of the text. Once constructed, pattern searching can be done in O(m log n) time, where m is the length of the pattern.
8. Comparing String Searching Algorithms
When choosing a string searching algorithm, consider the following factors:
- Text size: For small texts, simpler algorithms like naive search or KMP may be sufficient.
- Pattern length: Boyer-Moore performs well for longer patterns.
- Alphabet size: Larger alphabets favor algorithms like Boyer-Moore.
- Number of patterns: Aho-Corasick is efficient for multiple pattern searches.
- Preprocessing time: Some algorithms require more preprocessing but offer faster search times.
- Memory usage: Suffix arrays and Aho-Corasick automata can consume significant memory for large texts.
Algorithm | Preprocessing Time | Searching Time | Space Complexity |
---|---|---|---|
Naive | O(1) | O(nm) | O(1) |
KMP | O(m) | O(n + m) | O(m) |
Boyer-Moore | O(m + |Σ|) | O(nm) worst, O(n/m) best | O(|Σ|) |
Rabin-Karp | O(m) | O(n + m) average, O(nm) worst | O(1) |
Aho-Corasick | O(m) | O(n + m + z) | O(m) |
Suffix Array | O(n log n) | O(m log n) | O(n) |
Where n is the text length, m is the pattern length, |Σ| is the alphabet size, and z is the number of pattern occurrences.
9. Real-world Applications and Optimizations
String searching algorithms are used in various real-world applications:
- Text editors: Implementing find and replace functionality
- Bioinformatics: Analyzing DNA sequences
- Plagiarism detection: Comparing documents for similarities
- Network security: Inspecting network packets for malicious patterns
- Search engines: Indexing and retrieving web pages
To optimize string searching in practice, consider the following techniques:
- Use specialized libraries: Many programming languages have optimized string searching libraries.
- Implement bit-parallel algorithms: Leverage bitwise operations for faster comparisons.
- Utilize SIMD instructions: Take advantage of hardware-level parallelism for improved performance.
- Apply approximate matching: Use techniques like fuzzy string matching for more flexible searches.
- Implement indexing: For repeated searches on the same text, create an index structure like a suffix array or inverted index.
10. Conclusion
Efficient string searching algorithms are fundamental to many applications in computer science and software engineering. Understanding these algorithms and their trade-offs is crucial for developing optimized solutions and performing well in technical interviews.
As you prepare for interviews or work on projects involving string searching, practice implementing these algorithms and analyze their performance on different types of inputs. Remember that the choice of algorithm depends on the specific requirements of your application, such as input size, pattern characteristics, and performance constraints.
By mastering these techniques, you’ll be well-equipped to tackle string searching problems efficiently and demonstrate your problem-solving skills to potential employers in the tech industry.