Mastering String Manipulation for Better Problem Solving
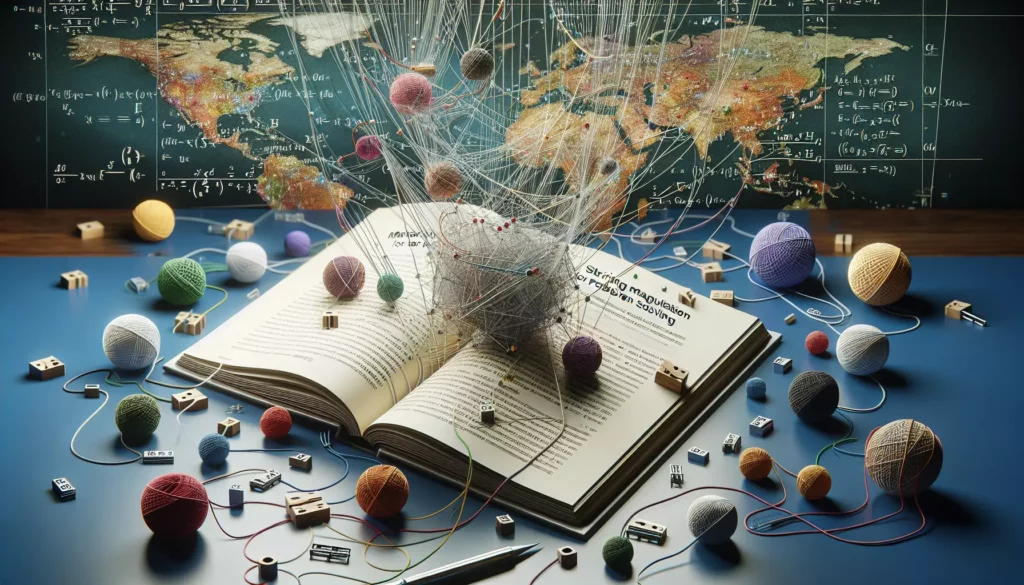
In the world of coding and programming, string manipulation is a fundamental skill that every developer should master. Whether you’re a beginner just starting your coding journey or an experienced programmer preparing for technical interviews at major tech companies, understanding how to effectively manipulate strings can significantly enhance your problem-solving abilities. In this comprehensive guide, we’ll dive deep into the art of string manipulation, exploring various techniques, common problems, and practical applications that will help you become a more proficient coder.
Why String Manipulation Matters
Before we delve into the specifics, let’s understand why string manipulation is so crucial in programming:
- Ubiquity: Strings are present in almost every programming language and are used extensively in various applications, from simple text processing to complex data analysis.
- Data Processing: Many real-world problems involve working with text data, which requires efficient string manipulation skills.
- Algorithm Implementation: Many algorithms, especially in areas like text searching and pattern matching, rely heavily on string manipulation techniques.
- Interview Preparation: String manipulation questions are common in technical interviews, particularly at major tech companies (FAANG).
- Performance Optimization: Efficient string manipulation can significantly improve the performance of your code.
Basic String Operations
Let’s start with some fundamental string operations that form the building blocks of more complex manipulations:
1. String Concatenation
Concatenation is the process of combining two or more strings. Most programming languages provide simple ways to concatenate strings. Here’s an example in Python:
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
print(full_name) # Output: John Doe
2. String Length
Determining the length of a string is a common operation. In most languages, there’s a built-in function or property to get the string length:
text = "Hello, World!"
length = len(text)
print(length) # Output: 13
3. Accessing Characters
Strings can be treated as arrays of characters, allowing you to access individual characters by their index:
text = "Python"
first_char = text[0]
last_char = text[-1]
print(first_char) # Output: P
print(last_char) # Output: n
4. Substring Extraction
Extracting a portion of a string, known as a substring, is a crucial operation:
text = "Programming is fun!"
substring = text[0:11]
print(substring) # Output: Programming
Advanced String Manipulation Techniques
Now that we’ve covered the basics, let’s explore some more advanced string manipulation techniques that will elevate your problem-solving skills:
1. String Reversal
Reversing a string is a common problem in coding interviews. Here’s a simple way to reverse a string in Python:
def reverse_string(s):
return s[::-1]
text = "Hello, World!"
reversed_text = reverse_string(text)
print(reversed_text) # Output: !dlroW ,olleH
2. String Splitting and Joining
Splitting a string into an array of substrings and joining an array of strings into a single string are powerful operations:
# Splitting
sentence = "The quick brown fox jumps over the lazy dog"
words = sentence.split()
print(words) # Output: ['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog']
# Joining
new_sentence = " ".join(words)
print(new_sentence) # Output: The quick brown fox jumps over the lazy dog
3. String Formatting
String formatting allows you to create dynamic strings with placeholders for variables:
name = "Alice"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string) # Output: My name is Alice and I am 30 years old.
4. Regular Expressions
Regular expressions (regex) are powerful tools for pattern matching and text manipulation. Here’s a simple example of using regex to find all email addresses in a text:
import re
text = "Contact us at info@example.com or support@example.com"
pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
emails = re.findall(pattern, text)
print(emails) # Output: ['info@example.com', 'support@example.com']
Common String Manipulation Problems and Solutions
Let’s tackle some common string manipulation problems that you might encounter in coding interviews or real-world scenarios:
1. Palindrome Check
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. Here’s a function to check if a string is a palindrome:
def is_palindrome(s):
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(c.lower() for c in s if c.isalnum())
return s == s[::-1]
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
print(is_palindrome("race a car")) # Output: False
2. Anagram Check
An anagram is a word or phrase formed by rearranging the letters of a different word or phrase. Here’s a function to check if two strings are anagrams:
def is_anagram(s1, s2):
# Remove spaces and convert to lowercase
s1 = s1.replace(" ", "").lower()
s2 = s2.replace(" ", "").lower()
# Check if the sorted strings are equal
return sorted(s1) == sorted(s2)
print(is_anagram("listen", "silent")) # Output: True
print(is_anagram("hello", "world")) # Output: False
3. Longest Common Prefix
Finding the longest common prefix among an array of strings is another common problem. Here’s a solution:
def longest_common_prefix(strs):
if not strs:
return ""
# Find the shortest string in the array
shortest = min(strs, key=len)
for i, char in enumerate(shortest):
for other in strs:
if other[i] != char:
return shortest[:i]
return shortest
print(longest_common_prefix(["flower", "flow", "flight"])) # Output: "fl"
print(longest_common_prefix(["dog", "racecar", "car"])) # Output: ""
4. String Compression
String compression is the process of replacing consecutive repeated characters with the character followed by the count of repetitions. Here’s a simple implementation:
def compress_string(s):
if not s:
return ""
compressed = []
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
compressed.append(s[i-1] + str(count))
count = 1
compressed.append(s[-1] + str(count))
compressed_str = ''.join(compressed)
return compressed_str if len(compressed_str) < len(s) else s
print(compress_string("aabcccccaaa")) # Output: "a2b1c5a3"
print(compress_string("abcdef")) # Output: "abcdef"
Advanced String Manipulation Algorithms
As you progress in your coding journey, you’ll encounter more complex string manipulation algorithms. Let’s explore a few advanced techniques:
1. Knuth-Morris-Pratt (KMP) Algorithm
The KMP algorithm is an efficient string matching algorithm that finds occurrences of a “word” within a main “text string” by employing the observation that when a mismatch occurs, the word itself embodies sufficient information to determine where the next match could begin, thus bypassing re-examination of previously matched characters.
def kmp_search(text, pattern):
if not pattern:
return 0
# Compute failure function
failure = [0] * len(pattern)
i = 1
j = 0
while i < len(pattern):
if pattern[i] == pattern[j]:
failure[i] = j + 1
i += 1
j += 1
elif j > 0:
j = failure[j-1]
else:
failure[i] = 0
i += 1
# Perform the search
i = 0 # index for text
j = 0 # index for pattern
while i < len(text):
if text[i] == pattern[j]:
if j == len(pattern) - 1:
return i - j
i += 1
j += 1
elif j > 0:
j = failure[j-1]
else:
i += 1
return -1
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
print(kmp_search(text, pattern)) # Output: 10
2. Rabin-Karp Algorithm
The Rabin-Karp algorithm is a string-searching algorithm that uses hashing to find any one of a set of pattern strings in a text. It’s particularly useful when searching for multiple patterns simultaneously.
def rabin_karp(text, pattern):
d = 256 # number of characters in the input alphabet
q = 101 # a prime number
m = len(pattern)
n = len(text)
i = 0
j = 0
p = 0 # hash value for pattern
t = 0 # hash value for text
h = 1
# The value of h would be "pow(d, m-1)%q"
for i in range(m-1):
h = (h * d) % q
# Calculate the hash value of pattern and first window of text
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
# Slide the pattern over text one by one
for i in range(n-m+1):
# Check the hash values of current window of text and pattern
# If the hash values match then only check for characters one by one
if p == t:
# Check for characters one by one
for j in range(m):
if text[i+j] != pattern[j]:
break
j += 1
# If p == t and pat[0...m-1] = txt[i, i+1, ...i+m-1]
if j == m:
return i
# Calculate hash value for next window of text: Remove leading digit, add trailing digit
if i < n-m:
t = (d*(t-ord(text[i])*h) + ord(text[i+m])) % q
# We might get negative value of t, converting it to positive
if t < 0:
t = t + q
return -1
text = "GEEKS FOR GEEKS"
pattern = "GEEK"
print(rabin_karp(text, pattern)) # Output: 0
3. Longest Palindromic Substring
Finding the longest palindromic substring in a given string is a classic problem that can be solved efficiently using dynamic programming or the Manacher’s algorithm. Here’s a simple implementation using dynamic programming:
def longest_palindromic_substring(s):
n = len(s)
# Table to store results of subproblems
dp = [[False for _ in range(n)] for _ in range(n)]
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
start = 0
max_length = 1
# Check for substrings of length 2
for i in range(n-1):
if s[i] == s[i+1]:
dp[i][i+1] = True
start = i
max_length = 2
# Check for lengths greater than 2
for k in range(3, n+1):
for i in range(n-k+1):
j = i + k - 1
if dp[i+1][j-1] and s[i] == s[j]:
dp[i][j] = True
if k > max_length:
start = i
max_length = k
return s[start:start+max_length]
s = "babad"
print(longest_palindromic_substring(s)) # Output: "bab" or "aba"
Practical Applications of String Manipulation
String manipulation skills are not just for coding interviews; they have numerous practical applications in real-world scenarios. Let’s explore some areas where strong string manipulation skills can make a significant difference:
1. Data Cleaning and Preprocessing
In data science and analytics, raw data often comes in the form of strings that need to be cleaned and preprocessed before analysis. This might involve tasks such as:
- Removing leading/trailing whitespace
- Converting text to uppercase or lowercase
- Removing or replacing special characters
- Extracting specific patterns (e.g., dates, email addresses) from text
Here’s an example of a function that cleans and standardizes a dataset of names:
import re
def clean_name(name):
# Convert to lowercase
name = name.lower()
# Remove leading/trailing whitespace
name = name.strip()
# Remove special characters
name = re.sub(r'[^a-z\s]', '', name)
# Capitalize first letter of each word
name = ' '.join(word.capitalize() for word in name.split())
return name
names = ["John DOE", "Alice Smith", "bob-johnson", "Emma Watson123"]
cleaned_names = [clean_name(name) for name in names]
print(cleaned_names)
# Output: ['John Doe', 'Alice Smith', 'Bob Johnson', 'Emma Watson']
2. Natural Language Processing (NLP)
NLP involves processing and analyzing large amounts of natural language data. String manipulation is fundamental to many NLP tasks, such as:
- Tokenization (splitting text into words or sentences)
- Stemming and lemmatization (reducing words to their root form)
- Named Entity Recognition (identifying and classifying named entities in text)
Here’s a simple example of tokenization and stemming using the NLTK library in Python:
import nltk
from nltk.tokenize import word_tokenize
from nltk.stem import PorterStemmer
nltk.download('punkt')
def tokenize_and_stem(text):
# Tokenize
tokens = word_tokenize(text)
# Stem
stemmer = PorterStemmer()
stemmed_tokens = [stemmer.stem(token) for token in tokens]
return stemmed_tokens
text = "The quick brown foxes are jumping over the lazy dogs"
result = tokenize_and_stem(text)
print(result)
# Output: ['the', 'quick', 'brown', 'fox', 'are', 'jump', 'over', 'the', 'lazi', 'dog']
3. Web Scraping and Data Extraction
When extracting data from websites or HTML documents, string manipulation skills are crucial. You’ll often need to parse HTML, extract specific elements, and clean the extracted text. Here’s an example using the BeautifulSoup library to extract all links from a webpage:
import requests
from bs4 import BeautifulSoup
def extract_links(url):
# Fetch the webpage
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract all links
links = []
for a in soup.find_all('a', href=True):
links.append(a['href'])
return links
url = "https://www.example.com"
links = extract_links(url)
print(links)
4. Text-based Games and Chatbots
String manipulation is essential when developing text-based games or chatbots. You’ll need to parse user input, extract commands or keywords, and generate appropriate responses. Here’s a simple example of a text-based game that uses string manipulation:
def text_adventure():
inventory = []
location = "start"
while True:
if location == "start":
print("You're at the start. You can go 'north' or 'south'.")
action = input("What do you want to do? ").lower().strip()
if "north" in action:
location = "forest"
elif "south" in action:
location = "beach"
else:
print("I don't understand that command.")
elif location == "forest":
print("You're in a dense forest. There's a 'key' on the ground.")
action = input("What do you want to do? ").lower().strip()
if "take key" in action or "pick up key" in action:
inventory.append("key")
print("You picked up the key.")
elif "south" in action:
location = "start"
elif "inventory" in action:
print(f"Inventory: {inventory}")
else:
print("I don't understand that command.")
elif location == "beach":
print("You're on a sandy beach. There's a locked 'chest'.")
action = input("What do you want to do? ").lower().strip()
if "open chest" in action and "key" in inventory:
print("You opened the chest with the key. You win!")
break
elif "north" in action:
location = "start"
elif "inventory" in action:
print(f"Inventory: {inventory}")
else:
print("I don't understand that command.")
text_adventure()
Best Practices for String Manipulation
As you work on improving your string manipulation skills, keep these best practices in mind:
- Use Built-in Functions: Most programming languages provide a rich set of built-in string manipulation functions. Familiarize yourself with these to avoid reinventing the wheel.
- Consider Performance: String operations can be costly in terms of time and memory. For large-scale applications, consider using more efficient data structures like StringBuilder (in Java) or io.StringIO (in Python) for string concatenation.
- Validate Input: Always validate and sanitize input strings, especially when dealing with user input, to prevent security vulnerabilities like SQL injection or cross-site scripting (XSS).
- Handle Encodings: Be aware of string encodings (e.g., UTF-8, ASCII) and handle them appropriately, especially when working with international text or special characters.
- Use Regular Expressions Judiciously: While powerful, regular expressions can be complex and potentially slow for large inputs. Use them wisely and consider alternatives for simple string operations.
- Write Clear and Readable Code: String manipulation can quickly become complex. Write clear, well-commented code and consider breaking complex operations into smaller, more manageable functions.
Conclusion
Mastering string manipulation is a crucial skill for any programmer, from beginners to those preparing for technical interviews at major tech companies. By understanding the fundamentals, learning advanced techniques, and practicing with real-world problems, you can significantly enhance your problem-solving abilities and become a more effective coder.
Remember that string manipulation is not just about solving coding challenges; it’s a skill that you’ll use frequently in various aspects of software development, from data processing to building user interfaces. As you continue your coding journey, keep exploring new string manipulation techniques and applying them to diverse problems.
Whether you’re using AlgoCademy to prepare for technical interviews or simply to improve your coding skills, focusing on string manipulation will undoubtedly pay dividends in your programming career. Happy coding!