Mastering String Manipulation: Essential Techniques for Coding Interviews
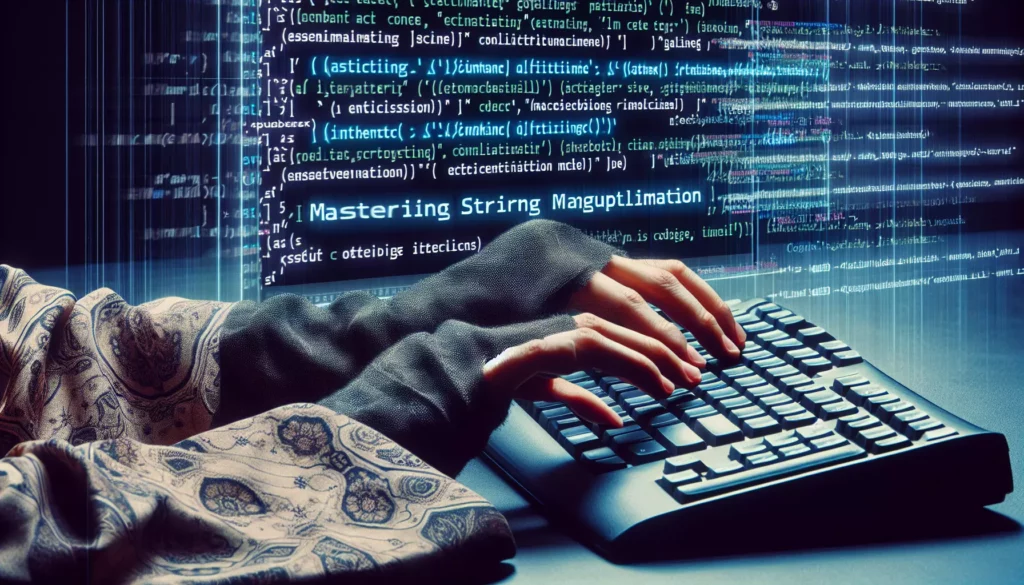
String manipulation is a fundamental skill that every programmer must master, especially when preparing for coding interviews at top tech companies. Whether you’re aiming for a position at a FAANG (Facebook, Amazon, Apple, Netflix, Google) company or any other tech giant, proficiency in string operations can make or break your performance during technical assessments. In this comprehensive guide, we’ll explore efficient techniques for string manipulation that will not only help you ace coding questions but also improve your overall programming skills.
1. Understanding the Basics of Strings
Before diving into advanced techniques, it’s crucial to have a solid grasp of what strings are and how they’re represented in various programming languages.
1.1 What is a String?
A string is a sequence of characters, typically used to represent text. In most programming languages, strings are immutable, meaning once created, their contents cannot be changed without creating a new string object.
1.2 String Representation in Different Languages
Different programming languages handle strings in various ways:
- Python: Strings are immutable sequences of Unicode characters.
- Java: Strings are objects of the String class, which are also immutable.
- C++: Strings can be represented using C-style char arrays or the std::string class.
- JavaScript: Strings are primitive values and immutable.
2. Essential String Operations
Let’s explore some fundamental string operations that form the building blocks of more complex manipulations.
2.1 Accessing Characters
Most languages allow you to access individual characters in a string using index notation:
// Python
s = "Hello"
print(s[0]) # Output: H
// Java
String s = "Hello";
System.out.println(s.charAt(0)); // Output: H
// C++
string s = "Hello";
cout << s[0] << endl; // Output: H
// JavaScript
let s = "Hello";
console.log(s[0]); // Output: H
2.2 String Concatenation
Combining strings is a common operation:
// Python
s1 = "Hello"
s2 = "World"
result = s1 + " " + s2 # Result: "Hello World"
// Java
String s1 = "Hello";
String s2 = "World";
String result = s1 + " " + s2; // Result: "Hello World"
// C++
string s1 = "Hello";
string s2 = "World";
string result = s1 + " " + s2; // Result: "Hello World"
// JavaScript
let s1 = "Hello";
let s2 = "World";
let result = s1 + " " + s2; // Result: "Hello World"
2.3 Substring Extraction
Extracting a portion of a string is crucial for many string manipulation tasks:
// Python
s = "Hello World"
sub = s[0:5] # Result: "Hello"
// Java
String s = "Hello World";
String sub = s.substring(0, 5); // Result: "Hello"
// C++
string s = "Hello World";
string sub = s.substr(0, 5); // Result: "Hello"
// JavaScript
let s = "Hello World";
let sub = s.substring(0, 5); // Result: "Hello"
3. Advanced String Manipulation Techniques
Now that we’ve covered the basics, let’s explore more advanced techniques that are often required in coding interviews.
3.1 Reversing a String
Reversing a string is a common interview question. Here’s how you can do it efficiently:
// Python
def reverse_string(s):
return s[::-1]
# Java
public String reverseString(String s) {
return new StringBuilder(s).reverse().toString();
}
// C++
string reverseString(string s) {
return string(s.rbegin(), s.rend());
}
// JavaScript
function reverseString(s) {
return s.split('').reverse().join('');
}
3.2 Checking for Palindromes
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. Here’s an efficient way to check for palindromes:
// Python
def is_palindrome(s):
return s == s[::-1]
# Java
public boolean isPalindrome(String s) {
int left = 0, right = s.length() - 1;
while (left < right) {
if (s.charAt(left) != s.charAt(right)) return false;
left++;
right--;
}
return true;
}
// C++
bool isPalindrome(string s) {
int left = 0, right = s.length() - 1;
while (left < right) {
if (s[left] != s[right]) return false;
left++;
right--;
}
return true;
}
// JavaScript
function isPalindrome(s) {
return s === s.split('').reverse().join('');
}
3.3 String Compression
String compression is another common interview question. The goal is to compress repeated characters:
// Python
def compress_string(s):
if not s:
return s
result = []
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
result.append(s[i-1] + str(count))
count = 1
result.append(s[-1] + str(count))
compressed = ''.join(result)
return compressed if len(compressed) < len(s) else s
# Java
public String compressString(String s) {
if (s == null || s.length() <= 1) return s;
StringBuilder result = new StringBuilder();
int count = 1;
for (int i = 1; i < s.length(); i++) {
if (s.charAt(i) == s.charAt(i-1)) {
count++;
} else {
result.append(s.charAt(i-1)).append(count);
count = 1;
}
}
result.append(s.charAt(s.length()-1)).append(count);
return result.length() < s.length() ? result.toString() : s;
}
// C++
string compressString(string s) {
if (s.length() <= 1) return s;
string result;
int count = 1;
for (int i = 1; i < s.length(); i++) {
if (s[i] == s[i-1]) {
count++;
} else {
result += s[i-1] + to_string(count);
count = 1;
}
}
result += s.back() + to_string(count);
return result.length() < s.length() ? result : s;
}
// JavaScript
function compressString(s) {
if (s.length <= 1) return s;
let result = '';
let count = 1;
for (let i = 1; i < s.length; i++) {
if (s[i] === s[i-1]) {
count++;
} else {
result += s[i-1] + count;
count = 1;
}
}
result += s[s.length-1] + count;
return result.length < s.length ? result : s;
}
4. Using Regular Expressions for String Manipulation
Regular expressions (regex) are powerful tools for pattern matching and string manipulation. While they can be complex, mastering regex can significantly enhance your string manipulation skills.
4.1 Basic Regex Operations
Here are some common regex operations:
// Python
import re
# Matching a pattern
pattern = r'\d+' # Matches one or more digits
text = "I have 123 apples and 456 oranges"
matches = re.findall(pattern, text)
print(matches) # Output: ['123', '456']
# Replacing a pattern
new_text = re.sub(r'\d+', 'X', text)
print(new_text) # Output: "I have X apples and X oranges"
// Java
import java.util.regex.*;
// Matching a pattern
Pattern pattern = Pattern.compile("\\d+");
Matcher matcher = pattern.matcher("I have 123 apples and 456 oranges");
while (matcher.find()) {
System.out.println(matcher.group());
}
// Replacing a pattern
String newText = "I have 123 apples and 456 oranges".replaceAll("\\d+", "X");
System.out.println(newText);
// C++
#include <regex>
#include <iostream>
// Matching a pattern
std::string text = "I have 123 apples and 456 oranges";
std::regex pattern("\\d+");
std::sregex_iterator iter(text.begin(), text.end(), pattern);
std::sregex_iterator end;
while (iter != end) {
std::cout << iter->str() << std::endl;
++iter;
}
// Replacing a pattern
std::string newText = std::regex_replace(text, pattern, "X");
std::cout << newText << std::endl;
// JavaScript
// Matching a pattern
let text = "I have 123 apples and 456 oranges";
let matches = text.match(/\d+/g);
console.log(matches); // Output: ['123', '456']
// Replacing a pattern
let newText = text.replace(/\d+/g, 'X');
console.log(newText); // Output: "I have X apples and X oranges"
4.2 Advanced Regex Techniques
For more complex string manipulations, you can use advanced regex features like lookaheads, lookbehinds, and capturing groups. Here’s an example of using a positive lookahead to find words followed by specific punctuation:
// Python
import re
text = "Hello, world! How are you? I'm fine."
pattern = r'\b\w+(?=[,.?!])'
matches = re.findall(pattern, text)
print(matches) # Output: ['Hello', 'world', 'you', 'fine']
// Java
import java.util.regex.*;
String text = "Hello, world! How are you? I'm fine.";
Pattern pattern = Pattern.compile("\\b\\w+(?=[,.?!])");
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
System.out.println(matcher.group());
}
// C++
#include <regex>
#include <iostream>
std::string text = "Hello, world! How are you? I'm fine.";
std::regex pattern("\\b\\w+(?=[,.?!])");
std::sregex_iterator iter(text.begin(), text.end(), pattern);
std::sregex_iterator end;
while (iter != end) {
std::cout << iter->str() << std::endl;
++iter;
}
// JavaScript
let text = "Hello, world! How are you? I'm fine.";
let matches = text.match(/\b\w+(?=[,.?!])/g);
console.log(matches); // Output: ['Hello', 'world', 'you', 'fine']
5. Optimizing String Manipulation for Performance
When working with large strings or performing many string operations, performance becomes crucial. Here are some tips to optimize your string manipulation code:
5.1 Use StringBuilder or StringBuffer in Java
For concatenating many strings, use StringBuilder (for single-threaded operations) or StringBuffer (for thread-safe operations) instead of the + operator:
// Less efficient
String result = "";
for (int i = 0; i < 1000; i++) {
result += "a";
}
// More efficient
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("a");
}
String result = sb.toString();
5.2 Use join() Method for Concatenating Multiple Strings
When concatenating a list of strings, use the join() method (available in many languages) instead of a loop:
// Python
strings = ["Hello", "World", "!"]
result = " ".join(strings) # Result: "Hello World !"
// Java
String[] strings = {"Hello", "World", "!"};
String result = String.join(" ", strings); // Result: "Hello World !"
// C++
vector<string> strings = {"Hello", "World", "!"};
string result = boost::algorithm::join(strings, " "); // Result: "Hello World !"
// JavaScript
let strings = ["Hello", "World", "!"];
let result = strings.join(" "); // Result: "Hello World !"
5.3 Use Appropriate Data Structures
Sometimes, using a different data structure can lead to more efficient string manipulation. For example, when you need to perform many insertions or deletions in the middle of a string, consider using a list of characters instead of a string:
// Python
# Less efficient for many insertions
s = "Hello World"
s = s[:5] + "Beautiful " + s[5:]
# More efficient
s_list = list("Hello World")
s_list[5:5] = list("Beautiful ")
result = "".join(s_list)
// Java
// Less efficient for many insertions
String s = "Hello World";
s = s.substring(0, 5) + "Beautiful " + s.substring(5);
// More efficient
List<Character> sList = new ArrayList<>();
for (char c : "Hello World".toCharArray()) {
sList.add(c);
}
sList.addAll(5, Arrays.asList('B', 'e', 'a', 'u', 't', 'i', 'f', 'u', 'l', ' '));
StringBuilder sb = new StringBuilder();
for (Character c : sList) {
sb.append(c);
}
String result = sb.toString();
6. Common String Manipulation Interview Questions
To help you prepare for coding interviews, here are some common string manipulation questions you might encounter:
6.1 Longest Palindromic Substring
Find the longest palindromic substring in a given string.
// Python
def longest_palindrome(s):
if not s:
return ""
start, max_len = 0, 1
for i in range(len(s)):
len1 = expand_around_center(s, i, i)
len2 = expand_around_center(s, i, i + 1)
length = max(len1, len2)
if length > max_len:
start = i - (length - 1) // 2
max_len = length
return s[start:start+max_len]
def expand_around_center(s, left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
# Test
print(longest_palindrome("babad")) # Output: "bab" or "aba"
print(longest_palindrome("cbbd")) # Output: "bb"
6.2 Valid Anagram
Determine if two strings are anagrams of each other (contain the same characters in a different order).
// Java
public boolean isAnagram(String s, String t) {
if (s.length() != t.length()) return false;
int[] count = new int[26];
for (int i = 0; i < s.length(); i++) {
count[s.charAt(i) - 'a']++;
count[t.charAt(i) - 'a']--;
}
for (int i : count) {
if (i != 0) return false;
}
return true;
}
// Test
System.out.println(isAnagram("anagram", "nagaram")); // Output: true
System.out.println(isAnagram("rat", "car")); // Output: false
6.3 Implement strStr()
Implement a function that finds the first occurrence of a substring in a string (similar to the strstr() function in C).
// C++
class Solution {
public:
int strStr(string haystack, string needle) {
if (needle.empty()) return 0;
int m = haystack.length(), n = needle.length();
for (int i = 0; i <= m - n; i++) {
int j;
for (j = 0; j < n; j++) {
if (haystack[i+j] != needle[j]) break;
}
if (j == n) return i;
}
return -1;
}
};
// Test
Solution sol;
cout << sol.strStr("hello", "ll") << endl; // Output: 2
cout << sol.strStr("aaaaa", "bba") << endl; // Output: -1
7. Advanced String Algorithms
For those aiming for top tech companies, it’s beneficial to be familiar with more advanced string algorithms. While these might not come up in every interview, understanding them can give you an edge and help you solve complex string problems more efficiently.
7.1 KMP Algorithm
The Knuth-Morris-Pratt (KMP) algorithm is an efficient string matching algorithm that can find all occurrences of a pattern string within a main text string in linear time complexity.
// JavaScript
function KMP(text, pattern) {
let lps = computeLPS(pattern);
let i = 0, j = 0;
let results = [];
while (i < text.length) {
if (pattern[j] === text[i]) {
i++;
j++;
}
if (j === pattern.length) {
results.push(i - j);
j = lps[j - 1];
} else if (i < text.length && pattern[j] !== text[i]) {
if (j !== 0) {
j = lps[j - 1];
} else {
i++;
}
}
}
return results;
}
function computeLPS(pattern) {
let lps = [0];
let len = 0;
let i = 1;
while (i < pattern.length) {
if (pattern[i] === pattern[len]) {
len++;
lps[i] = len;
i++;
} else {
if (len !== 0) {
len = lps[len - 1];
} else {
lps[i] = 0;
i++;
}
}
}
return lps;
}
// Test
console.log(KMP("ABABDABACDABABCABAB", "ABABCABAB")); // Output: [10]
7.2 Rabin-Karp Algorithm
The Rabin-Karp algorithm is another string matching algorithm that uses hashing to find patterns in strings.
// Python
def rabin_karp(text, pattern):
n, m = len(text), len(pattern)
p, t = 0, 0 # hash value for pattern and text
h = 1
d = 256 # number of characters in the input alphabet
q = 101 # a prime number
# The value of h would be "pow(d, m-1) % q"
for i in range(m-1):
h = (h * d) % q
# Calculate the hash value of pattern and first window of text
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
results = []
# Slide the pattern over text one by one
for i in range(n - m + 1):
# Check the hash values of current window of text and pattern
# If the hash values match then only check for characters one by one
if p == t:
# Check for characters one by one
if text[i:i+m] == pattern:
results.append(i)
# Calculate hash value for next window of text: Remove leading digit,
# add trailing digit
if i < n - m:
t = (d * (t - ord(text[i]) * h) + ord(text[i + m])) % q
# We might get negative values of t, converting it to positive
if t < 0:
t = t + q
return results
# Test
print(rabin_karp("ABABDABACDABABCABAB", "ABABCABAB")) # Output: [10]
7.3 Suffix Array and LCP Array
Suffix arrays and Longest Common Prefix (LCP) arrays are powerful data structures for string processing. They can be used to solve various string problems efficiently.
// Java
import java.util.*;
class SuffixArray {
public static int[] buildSuffixArray(String s) {
int n = s.length();
Integer[] order = new Integer[n];
for (int i = 0; i < n; i++) order[i] = i;
Arrays.sort(order, (a, b) -> s.charAt(a) - s.charAt(b));
int[] clazz = new int[n];
clazz[order[0]] = 0;
for (int i = 1; i < n; i++) {
clazz[order[i]] = clazz[order[i-1]];
if (s.charAt(order[i]) != s.charAt(order[i-1])) {
clazz[order[i]]++;
}
}
for (int k = 0; (1 << k) < n; k++) {
int[] c = clazz.clone();
for (int i = 0; i < n; i++) {
order[i] = (order[i] - (1 << k) + n) % n;
}
countSort(order, c);
clazz[order[0]] = 0;
for (int i = 1; i < n; i++) {
int mid1 = (order[i] + (1 << k)) % n;
int mid2 = (order[i-1] + (1 << k)) % n;
clazz[order[i]] = clazz[order[i-1]];
if (c[order[i]] != c[order[i-1]] || c[mid1] != c[mid2]) {
clazz[order[i]]++;
}
}
}
int[] suffixArray = new int[n];
for (int i = 0; i < n; i++) suffixArray[i] = order[i];
return suffixArray;
}
private static void countSort(Integer[] p, int[] c) {
int n = p.length;
int[] cnt = new int[n];
for (int x : c) cnt[x]++;
int[] p_new = new int[n];
int[] pos = new int[n];
pos[0] = 0;
for (int i = 1; i < n; i++) pos[i] = pos[i-1] + cnt[i-1];
for (int x : p) {
int i = c[x];
p_new[pos[i]] = x;
pos[i]++;
}
System.arraycopy(p_new, 0, p, 0, n);
}
public static int[] buildLCP(String s, int[] suffixArray) {
int n = s.length();
int[] rank = new int[n];
for (int i = 0; i < n; i++) rank[suffixArray[i]] = i;
int k = 0;
int[] lcp = new int[n-1];
for (int i = 0; i < n; i++) {
if (rank[i] == n - 1) {
k = 0;
continue;
}
int j = suffixArray[rank[i] + 1];
while (i + k < n && j + k < n && s.charAt(i+k) == s.charAt(j+k)) k++;
lcp[rank[i]] = k;
if (k > 0) k--;
}
return lcp;
}
public static void main(String[] args) {
String s = "banana$";
int[] suffixArray = buildSuffixArray(s);
int[] lcp = buildLCP(s, suffixArray);
System.out.println("Suffix Array:");
for (int i : suffixArray) System.out.print(i + " ");
System.out.println("\nLCP Array:");
for (int i : lcp) System.out.print(i + " ");
}
}
8. Conclusion
Mastering string manipulation techniques is crucial for success in coding interviews, especially when aiming for positions at top tech companies. By understanding the fundamentals, learning advanced algorithms, and practicing with common interview questions, you’ll be well-prepared to tackle any string-related problem that comes your way.
Remember, the key to excelling in string manipulation (and coding interviews in general) is not just knowing the algorithms, but understanding when and how to apply them efficiently. Always consider the time and space complexity of your solutions, and be prepared to discuss trade-offs between different approaches.
As you continue your journey in coding education and programming skills development, platforms like AlgoCademy can provide valuable resources and interactive coding tutorials to help you hone your skills. With consistent practice and a solid understanding of these techniques, you’ll be well-equipped to handle string manipulation challenges in your technical interviews and future coding projects.
Keep coding, keep learning, and best of luck in your programming journey!