Mastering Software Engineering Interviews: A Comprehensive Guide
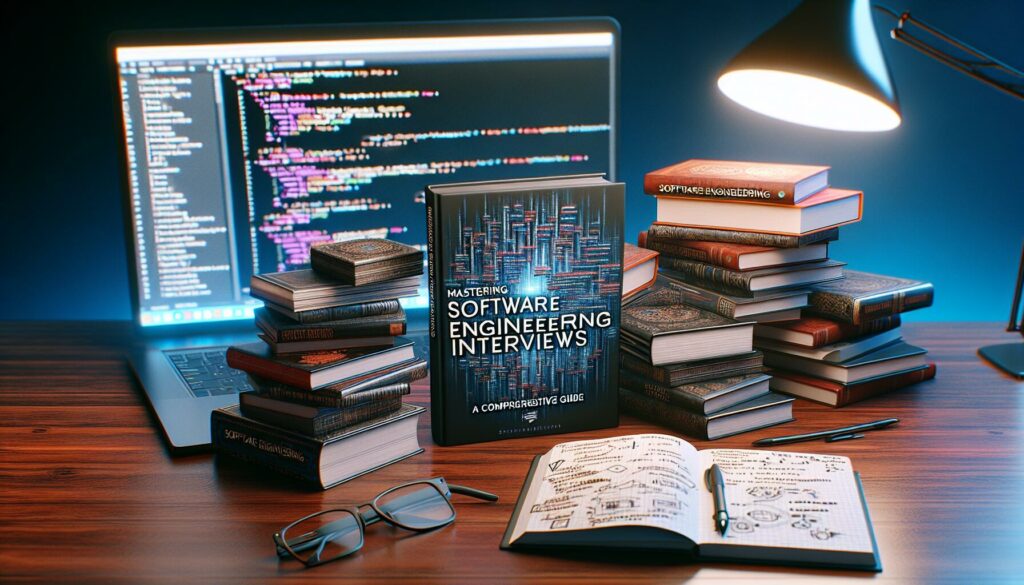
Software engineering interviews can be daunting, especially when you’re aiming for positions at top tech companies. Whether you’re a fresh graduate or an experienced developer looking to level up your career, preparing for these interviews requires dedication, strategy, and a deep understanding of both technical and soft skills. In this comprehensive guide, we’ll explore the intricacies of software engineering interviews, providing you with valuable insights and practical tips to help you succeed.
Understanding the Software Engineering Interview Process
Before diving into specific preparation strategies, it’s crucial to understand the typical software engineering interview process. While it may vary slightly from company to company, most follow a similar structure:
- Initial Screening: This often involves a phone or video call with a recruiter to assess your background and interest in the role.
- Technical Phone Screen: A brief technical interview, usually conducted remotely, to evaluate your basic coding and problem-solving skills.
- Take-Home Assignment: Some companies may ask you to complete a coding project on your own time.
- On-Site Interviews: A series of face-to-face interviews (or virtual equivalents) that typically include:
- Coding interviews
- System design discussions
- Behavioral interviews
- Final Decision: The hiring team evaluates your performance across all stages and makes an offer decision.
Key Areas to Focus On
To excel in software engineering interviews, you need to be well-versed in several key areas:
1. Data Structures and Algorithms
A solid grasp of data structures and algorithms is fundamental to passing technical interviews. You should be comfortable with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Recursion
Practice implementing these data structures from scratch and solving problems that utilize them efficiently.
2. Problem-Solving Skills
Interviewers are not just looking for correct answers; they want to see your problem-solving approach. Develop a systematic method for tackling coding challenges:
- Clarify the problem and requirements
- Discuss potential approaches
- Choose an optimal solution
- Implement the solution step-by-step
- Test and debug your code
- Analyze time and space complexity
3. System Design
For more senior positions, system design questions are crucial. You should be able to design scalable, reliable, and efficient systems. Key topics include:
- Distributed Systems
- Database Design
- Caching Mechanisms
- Load Balancing
- Microservices Architecture
- API Design
4. Programming Languages
While most companies allow you to code in your preferred language, having a deep understanding of at least one programming language is essential. Be prepared to discuss:
- Language-specific features and best practices
- Memory management
- Concurrency and multithreading
- Object-oriented programming principles
5. Soft Skills and Behavioral Questions
Technical skills alone are not enough. Interviewers also assess your:
- Communication skills
- Teamwork and collaboration abilities
- Problem-solving approach
- Adaptability and learning mindset
- Leadership potential (for senior roles)
Preparation Strategies
Now that we’ve covered the key areas, let’s discuss how to prepare effectively for software engineering interviews:
1. Consistent Practice
Regular practice is the cornerstone of interview preparation. Set aside dedicated time each day to solve coding problems and work on projects. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide range of problems to practice with.
2. Mock Interviews
Simulate real interview conditions by participating in mock interviews. You can do this with peers, mentors, or through online platforms that offer mock interview services. This helps you get comfortable with the pressure and time constraints of actual interviews.
3. Review Computer Science Fundamentals
Brush up on core computer science concepts, including:
- Operating Systems
- Computer Networks
- Database Management Systems
- Object-Oriented Design
4. Study System Design
For system design preparation:
- Read books like “Designing Data-Intensive Applications” by Martin Kleppmann
- Study existing system architectures of popular services
- Practice designing systems on a whiteboard or collaborative online tool
5. Build Projects
Develop personal projects to demonstrate your coding skills and creativity. This also provides real-world experience in dealing with design decisions and trade-offs.
6. Stay Updated with Industry Trends
Keep abreast of the latest developments in software engineering by:
- Following tech blogs and news sites
- Participating in coding communities and forums
- Attending tech conferences or webinars
Tackling Coding Interviews
When it comes to the actual coding interview, follow these best practices:
1. Communicate Clearly
Explain your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
2. Start with a Brute Force Solution
Begin by outlining a simple, straightforward solution. This shows you can quickly come up with a working answer, even if it’s not optimal.
3. Optimize Your Approach
After presenting the initial solution, discuss ways to optimize it. Consider time and space complexity trade-offs.
4. Write Clean, Readable Code
Even under pressure, strive to write clean, well-structured code. Use meaningful variable names, proper indentation, and add comments where necessary.
5. Test Your Code
Before declaring you’re done, walk through your code with a few test cases, including edge cases. This demonstrates attention to detail and a thorough approach.
Example Coding Problem and Solution
Let’s walk through a common interview problem and its solution to illustrate the problem-solving process:
Problem: Two Sum
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
Solution Approach:
- Clarify the problem: Can we assume there’s exactly one solution? Are the numbers sorted? Can we use additional space?
- Discuss approaches: Brute force (nested loops) vs. Hash table for optimization
- Implement the optimal solution
- Test with example cases
- Analyze time and space complexity
Python Implementation:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
# Test the function
print(two_sum([2, 7, 11, 15], 9)) # Expected output: [0, 1]
print(two_sum([3, 2, 4], 6)) # Expected output: [1, 2]
print(two_sum([3, 3], 6)) # Expected output: [0, 1]
This solution uses a hash table (dictionary in Python) to achieve O(n) time complexity and O(n) space complexity, which is more efficient than the O(n^2) brute force approach.
System Design Interview Tips
System design interviews assess your ability to architect large-scale systems. Here are some tips to excel in these interviews:
1. Clarify Requirements
Start by asking questions to understand the scope and constraints of the system you’re designing. Consider factors like:
- Expected scale (users, data volume)
- Performance requirements
- Reliability and availability needs
- Budget constraints
2. Start with a High-Level Design
Begin by sketching out the major components of the system. This might include:
- Client (web, mobile)
- Load balancers
- Application servers
- Databases
- Caching layers
- Message queues
3. Deep Dive into Specific Components
Based on the interviewer’s guidance, be prepared to drill down into specific areas of the system. This might involve discussing:
- Database schema design
- API design
- Caching strategies
- Data partitioning and sharding
4. Consider Trade-offs
Discuss the pros and cons of different design decisions. For example:
- SQL vs. NoSQL databases
- Monolithic vs. microservices architecture
- Real-time processing vs. batch processing
5. Address Scalability and Reliability
Explain how your design handles increased load and ensures high availability. This might involve discussing:
- Horizontal scaling strategies
- Replication and data consistency
- Failover mechanisms
- Content delivery networks (CDNs)
Behavioral Interview Preparation
Behavioral interviews assess your soft skills and cultural fit. Prepare for these by:
1. Using the STAR Method
When answering behavioral questions, use the STAR (Situation, Task, Action, Result) method to structure your responses:
- Situation: Describe the context
- Task: Explain your responsibility
- Action: Detail the steps you took
- Result: Share the outcome and what you learned
2. Preparing Specific Examples
Have ready examples for common behavioral questions, such as:
- A time you resolved a conflict in a team
- How you handled a challenging technical problem
- An instance where you demonstrated leadership
- How you deal with ambiguity or changing requirements
3. Researching the Company
Understand the company’s values, culture, and recent developments. This helps you tailor your responses and ask informed questions.
Post-Interview Follow-Up
After the interview:
- Send a thank-you email to your interviewers within 24 hours
- Reflect on your performance and note areas for improvement
- Follow up with the recruiter if you haven’t heard back within the expected timeframe
Continuous Learning and Improvement
Remember that interview preparation is an ongoing process. Even after securing a position, continue to:
- Practice coding regularly
- Contribute to open-source projects
- Attend tech meetups and conferences
- Read technical blogs and books
- Mentor others and share your knowledge
Conclusion
Mastering software engineering interviews requires a combination of technical prowess, problem-solving skills, and effective communication. By focusing on core computer science concepts, practicing coding problems regularly, and honing your system design and behavioral interview skills, you can significantly increase your chances of success.
Remember that interview preparation is a journey, not a destination. Embrace the learning process, stay curious, and view each interview as an opportunity to grow as a software engineer. With dedication and the right approach, you’ll be well-equipped to tackle even the most challenging software engineering interviews and land your dream job in the tech industry.
Platforms like AlgoCademy can be invaluable resources in your preparation journey, offering interactive coding tutorials, AI-powered assistance, and a structured approach to learning algorithms and data structures. Leverage these tools to supplement your studies and gain practical coding experience that will serve you well in your interviews and throughout your career.