Mastering Software Development Kits (SDKs): A Comprehensive Guide for Aspiring Developers
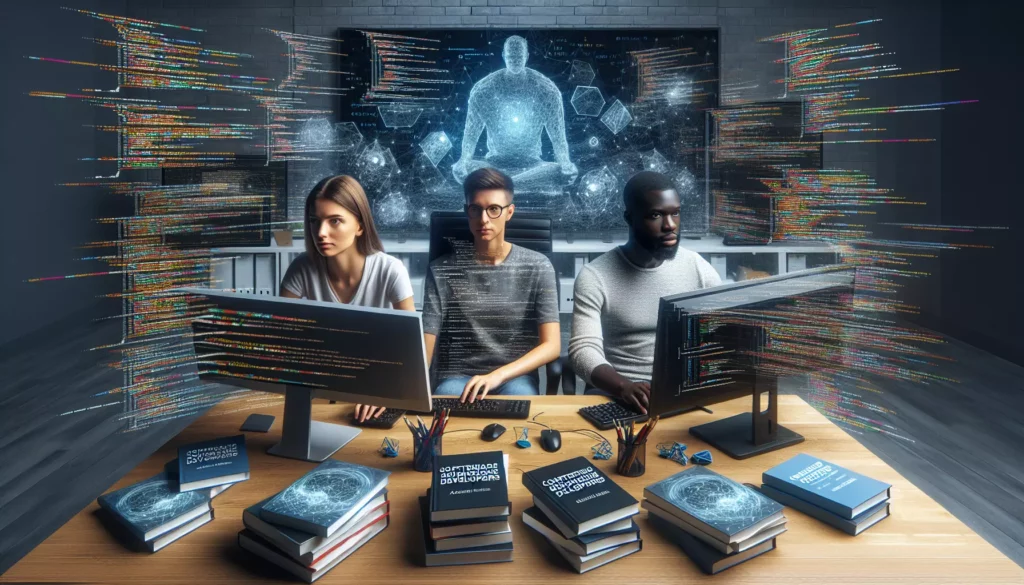
In the ever-evolving world of software development, staying ahead of the curve is crucial for aspiring developers. One of the most valuable skills you can acquire is the ability to effectively use Software Development Kits (SDKs). These powerful tools provided by tech giants like Facebook and Google can significantly enhance your coding capabilities and open doors to exciting opportunities in the industry. In this comprehensive guide, we’ll dive deep into the world of SDKs, exploring their importance, how to use them, and focusing on two of the most popular ones: the Facebook SDK and Google SDK.
What are Software Development Kits (SDKs)?
Before we delve into the specifics, let’s establish a clear understanding of what SDKs are and why they’re so important in the world of coding.
An SDK, or Software Development Kit, is a collection of software development tools, libraries, documentation, code samples, processes, and guides that allow developers to create software applications on a specific platform. SDKs are designed to be used for specific platforms or programming languages and typically contain the following components:
- APIs (Application Programming Interfaces)
- Programming tools
- Documentation
- Code samples
- Debugging tools
- Frameworks
SDKs are essential because they provide developers with a set of tools and resources that simplify the process of integrating specific functionalities into their applications. By using SDKs, developers can save time, reduce errors, and ensure compatibility with the target platform or service.
The Importance of Learning SDKs
As an aspiring developer, learning how to use SDKs is crucial for several reasons:
- Efficiency: SDKs provide pre-built components and tools that can significantly speed up the development process.
- Access to Advanced Features: SDKs often offer access to advanced features and APIs that would be difficult or time-consuming to implement from scratch.
- Industry Standards: Many companies rely on popular SDKs for their development processes, making familiarity with these tools a valuable skill in the job market.
- Cross-platform Development: Some SDKs enable developers to create applications that work across multiple platforms with minimal code changes.
- Community Support: Popular SDKs often have large communities of developers, providing a wealth of resources, tutorials, and support.
Getting Started with SDKs
Before diving into specific SDKs, it’s important to understand the general process of working with them. Here’s a step-by-step guide to getting started with SDKs:
- Choose the Right SDK: Select an SDK that aligns with your project goals and target platform.
- Download and Install: Obtain the SDK from the official source and follow the installation instructions.
- Set Up Your Development Environment: Configure your IDE or text editor to work with the SDK.
- Read the Documentation: Familiarize yourself with the SDK’s features, APIs, and best practices.
- Explore Sample Code: Study and experiment with provided code samples to understand how to implement various features.
- Join the Community: Engage with other developers using the SDK through forums, social media, or developer events.
- Practice and Build Projects: Apply your knowledge by building small projects or integrating SDK features into existing applications.
Facebook SDK: Integrating Social Features into Your Apps
The Facebook SDK is a powerful tool that allows developers to integrate Facebook features into their applications. Whether you’re building a mobile app, a web application, or a game, the Facebook SDK can help you leverage the social network’s vast user base and features.
Key Features of the Facebook SDK:
- Facebook Login: Enable users to sign in to your app using their Facebook credentials.
- Social Plugins: Integrate Facebook likes, shares, and comments into your app.
- Graph API: Access and publish data to Facebook’s social graph.
- Analytics: Track app usage and performance with Facebook Analytics.
- App Events: Log events to measure ad performance and build audiences for ad targeting.
Getting Started with Facebook SDK:
- Create a Facebook Developer Account: Visit the Facebook Developers website and sign up for an account.
- Create a New App: In the Facebook Developers dashboard, create a new app for your project.
- Choose Your Platform: Select the platform you’re developing for (iOS, Android, Web, etc.).
- Install the SDK: Follow the platform-specific instructions to add the Facebook SDK to your project.
- Configure Your App: Set up your app with the necessary permissions and settings.
Example: Implementing Facebook Login
Here’s a simple example of how to implement Facebook Login in a web application using the Facebook SDK for JavaScript:
<!-- Include the Facebook SDK -->
<script async defer crossorigin="anonymous" src="https://connect.facebook.net/en_US/sdk.js"></script>
<script>
window.fbAsyncInit = function() {
FB.init({
appId : 'YOUR_APP_ID',
cookie : true,
xfbml : true,
version : 'v11.0'
});
FB.AppEvents.logPageView();
};
function checkLoginState() {
FB.getLoginStatus(function(response) {
statusChangeCallback(response);
});
}
function statusChangeCallback(response) {
if (response.status === 'connected') {
console.log('Logged in and authenticated');
// You can now make API calls
} else {
console.log('Not authenticated');
}
}
</script>
<!-- Facebook Login Button -->
<fb:login-button scope="public_profile,email" onlogin="checkLoginState();">
</fb:login-button>
This example demonstrates how to initialize the Facebook SDK, create a login button, and handle the login process. Remember to replace ‘YOUR_APP_ID’ with your actual Facebook App ID.
Google SDK: Harnessing the Power of Google Services
Google offers a wide range of SDKs for various services and platforms. These SDKs allow developers to integrate Google’s powerful features and services into their applications. Some of the most popular Google SDKs include:
- Google Maps SDK
- Google Sign-In SDK
- Firebase SDK
- Google Analytics SDK
- Google Cloud SDKs
For this guide, we’ll focus on the Google Maps SDK, which is widely used in mobile and web applications.
Key Features of the Google Maps SDK:
- Interactive Maps: Display fully interactive, customizable maps in your application.
- Markers and Overlays: Add custom markers, polygons, and other overlays to your maps.
- Geocoding: Convert addresses to geographic coordinates and vice versa.
- Directions: Calculate and display routes between locations.
- Street View: Integrate Google’s Street View imagery into your app.
Getting Started with Google Maps SDK:
- Create a Google Cloud Platform Account: Visit the Google Cloud Console and create an account.
- Create a New Project: Set up a new project in the Google Cloud Console.
- Enable the Maps API: In the APIs & Services dashboard, enable the Google Maps JavaScript API.
- Obtain an API Key: Generate an API key for your project.
- Install the SDK: Follow the platform-specific instructions to add the Google Maps SDK to your project.
Example: Implementing a Google Map in a Web Application
Here’s a simple example of how to implement a Google Map in a web application using the Google Maps JavaScript API:
<!-- Include the Google Maps JavaScript API -->
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
<!-- HTML container for the map -->
<div id="map" style="height: 400px; width: 100%;"></div>
<script>
function initMap() {
// Specify the coordinates for the map center
var mapCenter = {lat: 40.7128, lng: -74.0060}; // New York City
// Create a new map instance
var map = new google.maps.Map(document.getElementById('map'), {
center: mapCenter,
zoom: 12
});
// Add a marker to the map
var marker = new google.maps.Marker({
position: mapCenter,
map: map,
title: 'New York City'
});
}
// Call the initMap function when the page loads
google.maps.event.addDomListener(window, 'load', initMap);
</script>
This example demonstrates how to create a simple Google Map centered on New York City with a marker. Remember to replace ‘YOUR_API_KEY’ with your actual Google Maps API key.
Best Practices for Working with SDKs
As you begin to work with SDKs, keep these best practices in mind to ensure smooth development and maintain code quality:
- Stay Updated: Regularly check for SDK updates and migrate to newer versions to access the latest features and security improvements.
- Read the Documentation Thoroughly: SDKs often come with extensive documentation. Take the time to read and understand it to make the most of the available features.
- Use Version Control: Implement version control in your projects to track changes and collaborate effectively with other developers.
- Handle Errors Gracefully: Implement proper error handling to ensure your application remains stable even when SDK calls fail.
- Optimize Performance: Be mindful of the impact SDKs can have on your app’s performance. Use profiling tools to identify and address any bottlenecks.
- Respect Platform Guidelines: When using SDKs for specific platforms (e.g., iOS or Android), adhere to the platform’s design guidelines and best practices.
- Secure Your API Keys: Never expose your API keys in client-side code. Use secure methods to store and access sensitive information.
- Test Thoroughly: Conduct comprehensive testing to ensure the SDK integrations work as expected across different devices and scenarios.
Expanding Your SDK Knowledge
As you become more comfortable with SDKs, consider exploring other popular options to broaden your skill set:
- Twitter SDK: Integrate Twitter features into your apps, such as tweet composition and user authentication.
- Amazon Web Services (AWS) SDK: Access various AWS services, including storage, database, and machine learning capabilities.
- Stripe SDK: Implement secure payment processing in your applications.
- Unity SDK: Develop cross-platform games and interactive experiences.
- Twilio SDK: Add communication features like SMS, voice calls, and video to your apps.
Conclusion
Mastering Software Development Kits (SDKs) is an essential skill for any aspiring developer. By learning to effectively use SDKs like the Facebook SDK and Google SDK, you’ll be able to create more powerful, feature-rich applications while saving time and effort. As you continue your journey in software development, remember to stay curious, keep practicing, and always be on the lookout for new SDKs and tools that can enhance your coding abilities.
The world of SDKs is vast and constantly evolving, offering endless opportunities for growth and innovation. By incorporating SDK knowledge into your skill set, you’ll be better prepared to tackle complex development challenges and create applications that stand out in today’s competitive tech landscape. So, dive in, experiment with different SDKs, and watch as your coding capabilities reach new heights!