Mastering Sliding Window Techniques: A Comprehensive Guide to Solving Coding Problems
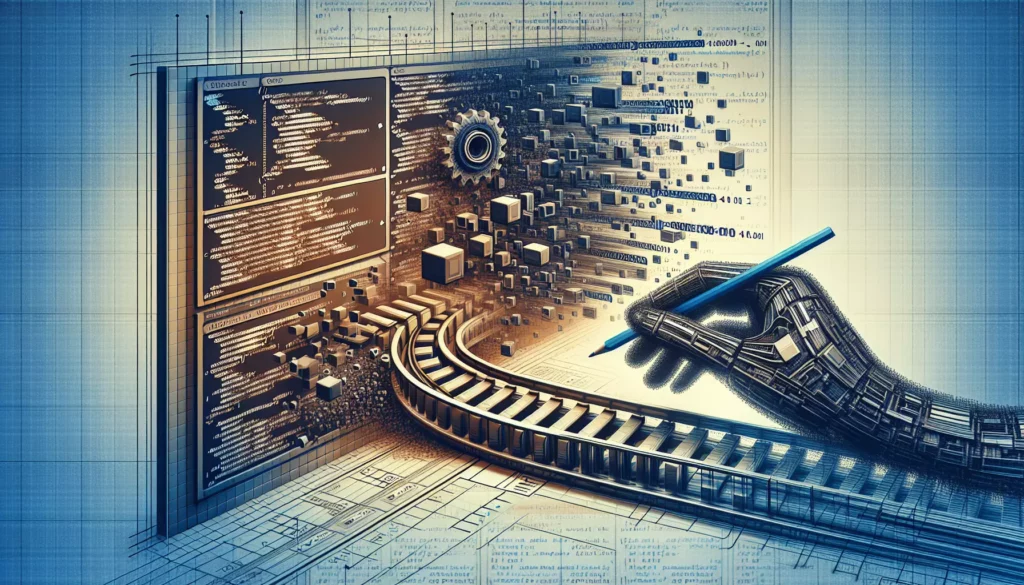
In the world of algorithmic problem-solving, the sliding window technique stands out as a powerful and efficient approach for tackling a wide range of coding challenges. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your programming skills, understanding and mastering sliding window algorithms can significantly boost your problem-solving abilities. In this comprehensive guide, we’ll dive deep into the sliding window technique, exploring its core concepts, common patterns, and practical applications through a series of examples and step-by-step solutions.
What is the Sliding Window Technique?
The sliding window technique is an algorithmic paradigm that involves maintaining a subset of elements (the “window”) as you iterate through a larger collection of data, typically an array or string. This window can grow, shrink, or slide as you process the elements, allowing you to efficiently solve problems that involve contiguous sequences or subarrays.
The key advantage of the sliding window approach is its ability to reduce the time complexity of certain problems from O(n²) or worse to O(n), making it an invaluable tool for optimizing solutions to a variety of coding challenges.
When to Use the Sliding Window Technique
The sliding window technique is particularly useful for problems that exhibit the following characteristics:
- The problem involves a linear data structure like an array or string
- You need to find a contiguous subarray or substring that satisfies certain conditions
- The problem requires calculating a running average or sum over a specific window size
- You need to identify the minimum, maximum, or optimal subarray/substring based on given criteria
Types of Sliding Window Problems
Sliding window problems can generally be categorized into two types:
- Fixed-size window: The size of the window remains constant throughout the algorithm.
- Variable-size window: The size of the window can change dynamically based on certain conditions.
Let’s explore both types with examples and step-by-step solutions.
Fixed-Size Window Problems
Example 1: Maximum Sum Subarray of Size K
Problem Statement: Given an array of integers and a positive integer k, find the maximum sum of any contiguous subarray of size k.
Solution:
public static int maxSubarraySum(int[] arr, int k) {
if (arr == null || arr.length < k) {
return 0;
}
int maxSum = 0;
int windowSum = 0;
// Calculate sum of first window
for (int i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window and update maxSum
for (int i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
Explanation:
- We start by calculating the sum of the first k elements to initialize our window.
- We then slide the window one element at a time, subtracting the element that’s no longer in the window and adding the new element.
- At each step, we update the maximum sum if the current window sum is greater.
This approach has a time complexity of O(n) and space complexity of O(1), making it highly efficient for large arrays.
Example 2: Find All Anagrams in a String
Problem Statement: Given two strings s and p, return an array of all the start indices of p’s anagrams in s.
Solution:
public List<Integer> findAnagrams(String s, String p) {
List<Integer> result = new ArrayList<>();
if (s == null || s.length() == 0 || p == null || p.length() == 0) {
return result;
}
int[] charCount = new int[26];
for (char c : p.toCharArray()) {
charCount[c - 'a']++;
}
int left = 0, right = 0, count = p.length();
while (right < s.length()) {
// Expand the window
if (charCount[s.charAt(right++) - 'a']-- >= 1) {
count--;
}
// Window found
if (count == 0) {
result.add(left);
}
// Shrink the window
if (right - left == p.length() && charCount[s.charAt(left++) - 'a']++ >= 0) {
count++;
}
}
return result;
}
Explanation:
- We use an array to keep track of the character count in string p.
- We maintain a window of size equal to the length of p and slide it across s.
- As we expand the window, we decrement the count of characters.
- When the count reaches zero, we’ve found an anagram and add its starting index to the result.
- As we shrink the window, we increment the count of characters that are no longer in the window.
This solution has a time complexity of O(n) where n is the length of string s, and a space complexity of O(1) since we use a fixed-size array for character counts.
Variable-Size Window Problems
Example 3: Longest Substring with At Most K Distinct Characters
Problem Statement: Given a string s and an integer k, find the length of the longest substring that contains at most k distinct characters.
Solution:
public int lengthOfLongestSubstringKDistinct(String s, int k) {
if (s == null || s.length() == 0 || k == 0) {
return 0;
}
Map<Character, Integer> charCount = new HashMap<>();
int left = 0, maxLength = 0;
for (int right = 0; right < s.length(); right++) {
char rightChar = s.charAt(right);
charCount.put(rightChar, charCount.getOrDefault(rightChar, 0) + 1);
while (charCount.size() > k) {
char leftChar = s.charAt(left);
charCount.put(leftChar, charCount.get(leftChar) - 1);
if (charCount.get(leftChar) == 0) {
charCount.remove(leftChar);
}
left++;
}
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
Explanation:
- We use a HashMap to keep track of the count of each character in the current window.
- We expand the window by adding characters from the right.
- If the number of distinct characters exceeds k, we shrink the window from the left until we have at most k distinct characters.
- We update the maximum length whenever we have a valid window.
This solution has a time complexity of O(n) where n is the length of the string, and a space complexity of O(k) for the HashMap.
Example 4: Minimum Window Substring
Problem Statement: Given two strings s and t, return the minimum window in s which will contain all the characters in t. If there is no such window in s that covers all characters in t, return the empty string “”.
Solution:
public String minWindow(String s, String t) {
if (s == null || s.length() == 0 || t == null || t.length() == 0) {
return "";
}
Map<Character, Integer> targetMap = new HashMap<>();
for (char c : t.toCharArray()) {
targetMap.put(c, targetMap.getOrDefault(c, 0) + 1);
}
int left = 0, minLeft = 0, minLength = Integer.MAX_VALUE;
int count = 0;
Map<Character, Integer> windowMap = new HashMap<>();
for (int right = 0; right < s.length(); right++) {
char rightChar = s.charAt(right);
windowMap.put(rightChar, windowMap.getOrDefault(rightChar, 0) + 1);
if (targetMap.containsKey(rightChar) && windowMap.get(rightChar).intValue() == targetMap.get(rightChar).intValue()) {
count++;
}
while (count == targetMap.size()) {
if (right - left + 1 < minLength) {
minLeft = left;
minLength = right - left + 1;
}
char leftChar = s.charAt(left);
windowMap.put(leftChar, windowMap.get(leftChar) - 1);
if (targetMap.containsKey(leftChar) && windowMap.get(leftChar).intValue() < targetMap.get(leftChar).intValue()) {
count--;
}
left++;
}
}
return minLength == Integer.MAX_VALUE ? "" : s.substring(minLeft, minLeft + minLength);
}
Explanation:
- We use two HashMaps: one for the target string t and one for the current window in s.
- We expand the window by adding characters from the right, updating the window map.
- When we have all the required characters (count equals the size of targetMap), we start shrinking the window from the left to find the minimum valid window.
- We keep track of the minimum window size and its starting position.
- Finally, we return the minimum window substring or an empty string if no valid window is found.
This solution has a time complexity of O(|s| + |t|) and a space complexity of O(|s| + |t|) in the worst case.
Common Patterns and Techniques in Sliding Window Problems
As you solve more sliding window problems, you’ll start to recognize common patterns and techniques. Here are some key strategies to keep in mind:
- Two Pointers: Most sliding window problems involve using two pointers (often called ‘left’ and ‘right’) to define the current window.
- Hash Map or Array for Counting: Use a HashMap or array to keep track of element frequencies within the window.
- Window Expansion and Contraction: Implement logic to expand the window (usually by moving the right pointer) and contract it (by moving the left pointer) based on problem constraints.
- Optimization Variables: Maintain variables to track the optimal solution (e.g., maxLength, minLength) as you slide the window.
- Validity Checks: Implement checks to ensure the current window satisfies the problem constraints before updating the optimal solution.
Tips for Solving Sliding Window Problems
- Identify the Window: Clearly define what constitutes a valid window for the problem at hand.
- Initialize Properly: Set up your data structures and variables correctly before starting the main loop.
- Handle Edge Cases: Consider empty inputs, single-element inputs, and other boundary conditions.
- Optimize as You Go: Update your optimal solution with each valid window, rather than storing all possibilities and choosing at the end.
- Practice Variations: Solve problems with both fixed and variable window sizes to build versatility.
Conclusion
The sliding window technique is a powerful tool in your algorithmic problem-solving toolkit. By mastering this approach, you’ll be well-equipped to tackle a wide range of coding challenges efficiently, particularly those involving subarrays or substrings. As with any algorithmic technique, the key to proficiency lies in consistent practice and exposure to various problem types.
Remember, while the core concept of sliding windows is straightforward, the real challenge often lies in recognizing when to apply this technique and how to adapt it to specific problem constraints. As you continue to practice, you’ll develop an intuition for identifying sliding window opportunities and implementing elegant, efficient solutions.
Whether you’re preparing for technical interviews at top tech companies or simply aiming to enhance your coding skills, mastering the sliding window technique will undoubtedly serve you well in your programming journey. Keep practicing, stay curious, and happy coding!