Mastering Sliding Window Techniques: A Comprehensive Guide for Coding Interviews
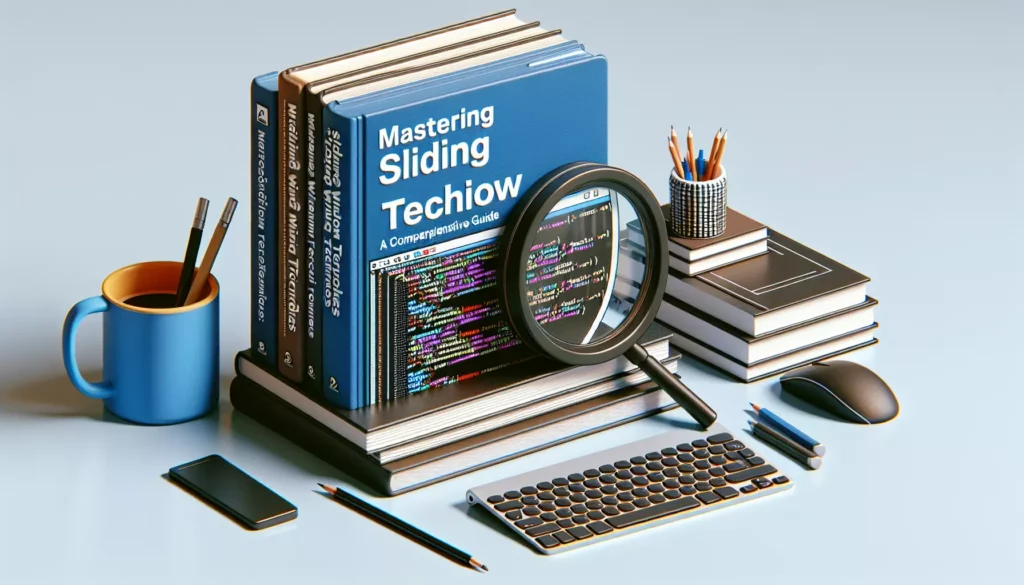
In the world of coding interviews and algorithmic problem-solving, the sliding window technique stands out as a powerful and efficient approach for tackling a wide range of problems. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your programming skills, understanding and mastering sliding window algorithms can give you a significant edge. In this comprehensive guide, we’ll dive deep into the sliding window technique, exploring its core concepts, common patterns, and practical applications.
What is the Sliding Window Technique?
The sliding window technique is an algorithmic paradigm that involves maintaining a subset of elements (the “window”) that slides through an array or string. This method is particularly useful for solving problems that require computing results over a contiguous sequence of elements. The primary advantage of this technique is its ability to reduce the time complexity of algorithms from O(n²) to O(n) in many cases.
Key Characteristics of Sliding Window Problems:
- The problem involves a data structure that is ordered and iterable, like an array or string.
- You’re asked to find or calculate something among all the contiguous subarrays or subsections of a specific size.
- The problem can be solved by expanding or shrinking a “window” of elements.
Types of Sliding Window Techniques
There are primarily two types of sliding window techniques:
1. Fixed-Size Window
In this approach, the size of the window remains constant throughout the iteration. This is typically used when you’re dealing with subarrays or substrings of a fixed length.
2. Variable-Size Window
Here, the size of the window can grow or shrink based on certain conditions. This is useful when dealing with problems where the size of the subarray or substring is not fixed but needs to meet specific criteria.
Common Sliding Window Patterns
Let’s explore some common patterns and techniques used in sliding window problems:
1. The Basic Sliding Window Template
Here’s a general template for implementing a sliding window algorithm:
def sliding_window(arr):
left = 0
window_sum = 0
result = 0
for right in range(len(arr)):
# Expand the window
window_sum += arr[right]
# Contract the window if necessary
while condition:
window_sum -= arr[left]
left += 1
# Update the result
result = max(result, right - left + 1)
return result
2. Two Pointers Technique
The two pointers technique is often used in conjunction with sliding windows. It involves maintaining two pointers that define the boundaries of the current window.
def two_pointers_sliding_window(arr):
left = right = 0
result = 0
while right < len(arr):
# Expand the window
# Process the element at the right pointer
while condition:
# Contract the window
# Process the element at the left pointer
left += 1
# Update the result
result = max(result, right - left + 1)
right += 1
return result
3. Sliding Window with Hash Map
For problems involving counting or tracking frequencies of elements, using a hash map (dictionary in Python) can be very effective.
from collections import defaultdict
def sliding_window_with_hashmap(s):
char_count = defaultdict(int)
left = 0
result = 0
for right in range(len(s)):
# Add the current character to the hash map
char_count[s[right]] += 1
# Contract the window if necessary
while condition:
char_count[s[left]] -= 1
if char_count[s[left]] == 0:
del char_count[s[left]]
left += 1
# Update the result
result = max(result, right - left + 1)
return result
Common Sliding Window Problems and Solutions
Let’s look at some classic sliding window problems and how to solve them:
1. Maximum Sum Subarray of Size K
Problem: Given an array of integers and a positive integer k, find the maximum sum of any contiguous subarray of size k.
def max_sum_subarray(arr, k):
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
print(max_sum_subarray(arr, k)) # Output: 39
2. Longest Substring with K Distinct Characters
Problem: Given a string and an integer k, find the length of the longest substring that contains at most k distinct characters.
from collections import defaultdict
def longest_substring_with_k_distinct(s, k):
char_count = defaultdict(int)
left = 0
max_length = 0
for right in range(len(s)):
char_count[s[right]] += 1
while len(char_count) > k:
char_count[s[left]] -= 1
if char_count[s[left]] == 0:
del char_count[s[left]]
left += 1
max_length = max(max_length, right - left + 1)
return max_length
# Example usage
s = "aabacbebebe"
k = 3
print(longest_substring_with_k_distinct(s, k)) # Output: 7
3. Minimum Window Substring
Problem: Given two strings s and t, return the minimum window in s which will contain all the characters in t.
from collections import Counter
def min_window_substring(s, t):
if not t or not s:
return ""
dict_t = Counter(t)
required = len(dict_t)
left = 0
formed = 0
window_counts = {}
ans = float("inf"), None, None
for right in range(len(s)):
character = s[right]
window_counts[character] = window_counts.get(character, 0) + 1
if character in dict_t and window_counts[character] == dict_t[character]:
formed += 1
while left <= right and formed == required:
character = s[left]
if right - left + 1 < ans[0]:
ans = (right - left + 1, left, right)
window_counts[character] -= 1
if character in dict_t and window_counts[character] < dict_t[character]:
formed -= 1
left += 1
return "" if ans[0] == float("inf") else s[ans[1] : ans[2] + 1]
# Example usage
s = "ADOBECODEBANC"
t = "ABC"
print(min_window_substring(s, t)) # Output: "BANC"
Advanced Sliding Window Techniques
As you become more comfortable with basic sliding window problems, you can explore more advanced techniques and variations:
1. Sliding Window with Multiple Conditions
Some problems may require you to maintain multiple conditions simultaneously. For example, finding the longest substring with at most k distinct characters and at least m repeating characters.
2. Sliding Window on Circular Arrays
When dealing with circular arrays, you might need to modify your sliding window approach to wrap around the array.
3. Sliding Window with Dynamic Window Size
In some cases, the window size might change dynamically based on certain conditions, requiring a more flexible approach.
4. Multi-dimensional Sliding Window
While less common, some problems may require applying the sliding window technique to 2D arrays or matrices.
Tips for Mastering Sliding Window Problems
- Identify the Window: Clearly define what constitutes your window and what information you need to track within it.
- Choose the Right Data Structure: Depending on the problem, you might need to use arrays, hash maps, or more complex data structures to efficiently manage your window.
- Practice Expansion and Contraction: Get comfortable with the process of expanding and contracting your window based on specific conditions.
- Optimize Space Complexity: While sliding window techniques often optimize time complexity, be mindful of space usage, especially when dealing with large inputs.
- Handle Edge Cases: Pay attention to edge cases, such as empty inputs or windows of size 1.
- Visualize the Window: Drawing out the window and how it moves can help you understand and solve complex problems.
Common Pitfalls and How to Avoid Them
When working with sliding window problems, be aware of these common pitfalls:
- Off-by-One Errors: Be careful with your window boundaries, especially when updating indices.
- Forgetting to Reset: When contracting the window, make sure to properly reset or update all relevant variables and data structures.
- Inefficient Window Updates: Avoid recalculating the entire window sum or state with each iteration. Instead, update incrementally.
- Overlooking Variable-Size Windows: Don’t assume all sliding window problems have a fixed window size. Be prepared to adjust your approach for variable-size windows.
- Ignoring Time Complexity: While sliding window techniques are often efficient, make sure your implementation maintains the expected time complexity.
Sliding Window in Real-World Applications
The sliding window technique isn’t just useful for coding interviews; it has practical applications in various domains:
- Network Protocols: Sliding window protocols are used in network communication for flow control and error correction.
- Data Analysis: In time series analysis, sliding windows are used to compute moving averages and other rolling statistics.
- Image Processing: Sliding window techniques are applied in image processing for operations like convolution and feature detection.
- Financial Analysis: Sliding windows are used to calculate moving averages and other technical indicators in stock market analysis.
- Natural Language Processing: In NLP, sliding windows can be used for tasks like n-gram analysis and text segmentation.
Conclusion
Mastering the sliding window technique is a valuable skill for any programmer, particularly those preparing for technical interviews or working on algorithmic problems. By understanding the core concepts, recognizing common patterns, and practicing with diverse problems, you can significantly improve your problem-solving abilities and efficiency in tackling a wide range of coding challenges.
Remember, the key to mastering sliding window problems lies in consistent practice and a deep understanding of the underlying principles. As you work through more problems, you’ll develop an intuition for when and how to apply this powerful technique effectively.
Keep challenging yourself with increasingly complex problems, and don’t hesitate to explore variations and advanced applications of the sliding window technique. With dedication and practice, you’ll be well-equipped to tackle even the most challenging sliding window problems in your coding interviews and beyond.
Additional Resources
To further enhance your understanding and skills with sliding window problems, consider exploring these resources:
- LeetCode’s Sliding Window Problems: Practice with a curated list of sliding window problems on LeetCode.
- Educative.io’s Sliding Window Pattern Course: A comprehensive course focusing on the sliding window pattern and its variations.
- GeeksforGeeks Sliding Window Articles: In-depth articles and problem sets related to sliding window algorithms.
- YouTube Tutorials: Visual learners can benefit from video explanations of sliding window concepts and problem-solving approaches.
- Coding Interview Books: Many popular interview preparation books, such as “Cracking the Coding Interview” and “Elements of Programming Interviews,” contain sections on sliding window techniques.
By combining theoretical knowledge with practical problem-solving, you’ll be well on your way to mastering the sliding window technique and improving your overall coding skills. Happy coding!