Mastering Segment Trees: A Comprehensive Guide for Coding Interviews
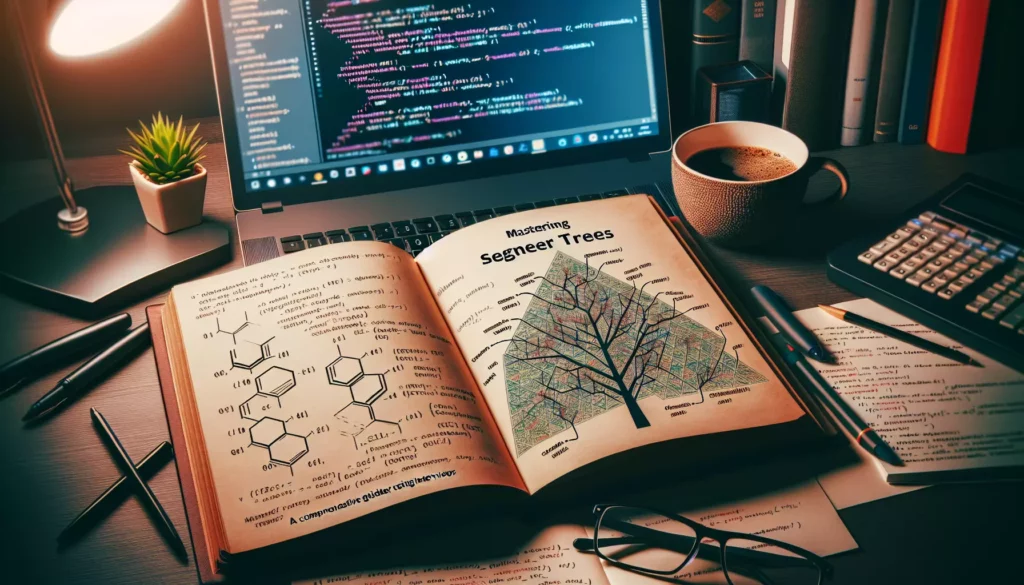
In the world of competitive programming and technical interviews, particularly for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), having a strong grasp of advanced data structures is crucial. Among these, the Segment Tree stands out as a powerful and versatile tool that can efficiently solve a wide range of problems involving range queries and updates. In this comprehensive guide, we’ll dive deep into the world of Segment Trees, exploring their structure, implementation, and various applications.
Table of Contents
- Introduction to Segment Trees
- Structure and Concept
- Implementing a Basic Segment Tree
- Basic Operations: Range Query and Point Update
- Lazy Propagation for Range Updates
- Applications and Problem-Solving
- Variations and Advanced Concepts
- Interview Tips and Common Pitfalls
- Practice Problems and Resources
- Conclusion
1. Introduction to Segment Trees
A Segment Tree is a tree-like data structure used for storing information about intervals, or segments. It allows for efficient querying of cumulative information for contiguous ranges in the array and supports modification of the underlying array elements. The power of Segment Trees lies in their ability to perform both range queries and updates in logarithmic time complexity, making them ideal for scenarios where we need to handle dynamic ranges efficiently.
Key features of Segment Trees include:
- Efficient range queries (e.g., sum, minimum, maximum) in O(log n) time
- Quick point updates in O(log n) time
- Ability to handle range updates with lazy propagation
- Versatility in solving various types of range-based problems
2. Structure and Concept
At its core, a Segment Tree is a binary tree where each node represents a segment (or range) of the original array. The root node represents the entire array, and each child node represents a half of its parent’s segment. This recursive division continues until we reach the leaf nodes, which represent individual elements of the original array.
Here’s a visual representation of a Segment Tree for an array of size 8:
[0-7] / \ [0-3] [4-7] / \ / \ [0-1] [2-3] [4-5] [6-7] / \ / \ / \ / \ [0][1][2][3][4][5][6][7]
In this structure:
- The root node [0-7] represents the entire array (indices 0 to 7).
- Each internal node represents a range that is the union of its children’s ranges.
- Leaf nodes correspond to individual array elements.
The tree is typically implemented as an array, where for a node at index i:
- Its left child is at index 2i + 1
- Its right child is at index 2i + 2
- Its parent is at index (i – 1) / 2
3. Implementing a Basic Segment Tree
Let’s implement a basic Segment Tree in C++ that supports range sum queries and point updates. We’ll start with the structure and the build function:
#include <vector>
#include <cmath>
class SegmentTree {
private:
std::vector<int> tree;
int n;
void build(const std::vector<int>& arr, int v, int tl, int tr) {
if (tl == tr) {
tree[v] = arr[tl];
} else {
int tm = (tl + tr) / 2;
build(arr, v*2+1, tl, tm);
build(arr, v*2+2, tm+1, tr);
tree[v] = tree[v*2+1] + tree[v*2+2];
}
}
public:
SegmentTree(const std::vector<int>& arr) {
n = arr.size();
tree.resize(4 * n);
build(arr, 0, 0, n - 1);
}
// Other methods will be added here
};
In this implementation:
- The
tree
vector stores the Segment Tree. - The
build
function recursively constructs the tree from the input array. - We allocate 4 * n space for the tree to ensure we have enough nodes (this is an upper bound).
4. Basic Operations: Range Query and Point Update
Now, let’s add methods for range sum query and point update:
int sum(int v, int tl, int tr, int l, int r) {
if (l > r) return 0;
if (l == tl && r == tr) return tree[v];
int tm = (tl + tr) / 2;
return sum(v*2+1, tl, tm, l, std::min(r, tm))
+ sum(v*2+2, tm+1, tr, std::max(l, tm+1), r);
}
void update(int v, int tl, int tr, int pos, int new_val) {
if (tl == tr) {
tree[v] = new_val;
} else {
int tm = (tl + tr) / 2;
if (pos <= tm)
update(v*2+1, tl, tm, pos, new_val);
else
update(v*2+2, tm+1, tr, pos, new_val);
tree[v] = tree[v*2+1] + tree[v*2+2];
}
}
// Public methods
int query(int l, int r) {
return sum(0, 0, n - 1, l, r);
}
void update(int pos, int new_val) {
update(0, 0, n - 1, pos, new_val);
}
These methods allow us to:
- Query the sum of any range [l, r] in O(log n) time.
- Update any single element in O(log n) time.
5. Lazy Propagation for Range Updates
While our basic Segment Tree supports point updates efficiently, sometimes we need to update entire ranges. Lazy propagation is a technique that allows us to perform range updates efficiently by deferring the updates to children nodes until they are needed.
Here’s how we can modify our Segment Tree to support lazy propagation:
class LazySegmentTree {
private:
std::vector<int> tree, lazy;
int n;
void build(const std::vector<int>& arr, int v, int tl, int tr) {
if (tl == tr) {
tree[v] = arr[tl];
} else {
int tm = (tl + tr) / 2;
build(arr, v*2+1, tl, tm);
build(arr, v*2+2, tm+1, tr);
tree[v] = tree[v*2+1] + tree[v*2+2];
}
}
void push(int v, int tl, int tr) {
if (lazy[v] != 0) {
tree[v] += (tr - tl + 1) * lazy[v];
if (tl != tr) {
lazy[v*2+1] += lazy[v];
lazy[v*2+2] += lazy[v];
}
lazy[v] = 0;
}
}
int sum(int v, int tl, int tr, int l, int r) {
push(v, tl, tr);
if (l > r) return 0;
if (l == tl && r == tr) return tree[v];
int tm = (tl + tr) / 2;
return sum(v*2+1, tl, tm, l, std::min(r, tm))
+ sum(v*2+2, tm+1, tr, std::max(l, tm+1), r);
}
void update(int v, int tl, int tr, int l, int r, int addend) {
push(v, tl, tr);
if (l > r) return;
if (l == tl && r == tr) {
lazy[v] += addend;
push(v, tl, tr);
} else {
int tm = (tl + tr) / 2;
update(v*2+1, tl, tm, l, std::min(r, tm), addend);
update(v*2+2, tm+1, tr, std::max(l, tm+1), r, addend);
tree[v] = tree[v*2+1] + tree[v*2+2];
}
}
public:
LazySegmentTree(const std::vector<int>& arr) {
n = arr.size();
tree.resize(4 * n);
lazy.resize(4 * n, 0);
build(arr, 0, 0, n - 1);
}
int query(int l, int r) {
return sum(0, 0, n - 1, l, r);
}
void update(int l, int r, int addend) {
update(0, 0, n - 1, l, r, addend);
}
};
Key points about lazy propagation:
- We introduce a
lazy
array to store pending updates. - The
push
function propagates lazy updates to children when necessary. - Both
sum
andupdate
functions now callpush
before processing a node. - Range updates are now possible and efficient, with O(log n) complexity.
6. Applications and Problem-Solving
Segment Trees are incredibly versatile and can be applied to a wide range of problems. Here are some common applications:
- Range Minimum/Maximum Query (RMQ): Find the minimum or maximum element in a given range.
- Range Sum Query: Calculate the sum of elements in a given range.
- Range GCD Query: Find the Greatest Common Divisor of elements in a range.
- Range Update Operations: Perform updates on a range of elements efficiently.
- Finding k-th smallest/largest element in a range: With some modifications, Segment Trees can solve this problem efficiently.
Let’s solve a classic problem: Range Minimum Query (RMQ) with point updates.
class RMQSegmentTree {
private:
std::vector<int> tree;
int n;
void build(const std::vector<int>& arr, int v, int tl, int tr) {
if (tl == tr) {
tree[v] = arr[tl];
} else {
int tm = (tl + tr) / 2;
build(arr, v*2+1, tl, tm);
build(arr, v*2+2, tm+1, tr);
tree[v] = std::min(tree[v*2+1], tree[v*2+2]);
}
}
int getMin(int v, int tl, int tr, int l, int r) {
if (l > r) return INT_MAX;
if (l == tl && r == tr) return tree[v];
int tm = (tl + tr) / 2;
return std::min(getMin(v*2+1, tl, tm, l, std::min(r, tm)),
getMin(v*2+2, tm+1, tr, std::max(l, tm+1), r));
}
void update(int v, int tl, int tr, int pos, int new_val) {
if (tl == tr) {
tree[v] = new_val;
} else {
int tm = (tl + tr) / 2;
if (pos <= tm)
update(v*2+1, tl, tm, pos, new_val);
else
update(v*2+2, tm+1, tr, pos, new_val);
tree[v] = std::min(tree[v*2+1], tree[v*2+2]);
}
}
public:
RMQSegmentTree(const std::vector<int>& arr) {
n = arr.size();
tree.resize(4 * n);
build(arr, 0, 0, n - 1);
}
int query(int l, int r) {
return getMin(0, 0, n - 1, l, r);
}
void update(int pos, int new_val) {
update(0, 0, n - 1, pos, new_val);
}
};
This implementation allows us to find the minimum element in any range and update individual elements, both in O(log n) time.
7. Variations and Advanced Concepts
As you delve deeper into Segment Trees, you’ll encounter various advanced concepts and variations:
- 2D Segment Trees: Used for handling 2D range queries, often in problems involving matrices.
- Persistent Segment Trees: Allow querying previous versions of the tree after updates.
- Merge Sort Tree: A variation that stores sorted lists of elements in each node, useful for more complex range queries.
- Dynamic Segment Trees: Segment Trees that can grow or shrink dynamically.
- Segment Trees with Fractional Cascading: An optimization technique for faster range queries in certain scenarios.
Here’s a brief example of a 2D Segment Tree for range sum queries:
class SegmentTree2D {
private:
std::vector<std::vector<int>> tree;
int n, m;
void build_y(const std::vector<std::vector<int>>& a, int vx, int lx, int rx, int vy, int ly, int ry) {
if (ly == ry) {
if (lx == rx)
tree[vx][vy] = a[lx][ly];
else
tree[vx][vy] = tree[vx*2+1][vy] + tree[vx*2+2][vy];
} else {
int my = (ly + ry) / 2;
build_y(a, vx, lx, rx, vy*2+1, ly, my);
build_y(a, vx, lx, rx, vy*2+2, my+1, ry);
tree[vx][vy] = tree[vx][vy*2+1] + tree[vx][vy*2+2];
}
}
void build_x(const std::vector<std::vector<int>>& a, int vx, int lx, int rx) {
if (lx != rx) {
int mx = (lx + rx) / 2;
build_x(a, vx*2+1, lx, mx);
build_x(a, vx*2+2, mx+1, rx);
}
build_y(a, vx, lx, rx, 0, 0, m-1);
}
int sum_y(int vx, int vy, int tly, int try_, int ly, int ry) {
if (ly > ry) return 0;
if (ly == tly && try_ == ry) return tree[vx][vy];
int tmy = (tly + try_) / 2;
return sum_y(vx, vy*2+1, tly, tmy, ly, std::min(ry, tmy))
+ sum_y(vx, vy*2+2, tmy+1, try_, std::max(ly, tmy+1), ry);
}
int sum_x(int vx, int tlx, int trx, int lx, int rx, int ly, int ry) {
if (lx > rx) return 0;
if (lx == tlx && trx == rx) return sum_y(vx, 0, 0, m-1, ly, ry);
int tmx = (tlx + trx) / 2;
return sum_x(vx*2+1, tlx, tmx, lx, std::min(rx, tmx), ly, ry)
+ sum_x(vx*2+2, tmx+1, trx, std::max(lx, tmx+1), rx, ly, ry);
}
public:
SegmentTree2D(const std::vector<std::vector<int>>& a) {
n = a.size();
m = a[0].size();
tree.resize(4*n, std::vector<int>(4*m));
build_x(a, 0, 0, n-1);
}
int query(int lx, int ly, int rx, int ry) {
return sum_x(0, 0, n-1, lx, rx, ly, ry);
}
};
This 2D Segment Tree allows for efficient range sum queries in a 2D grid, with both queries and updates running in O(log n * log m) time, where n and m are the dimensions of the grid.
8. Interview Tips and Common Pitfalls
When dealing with Segment Trees in coding interviews, keep these tips in mind:
- Understand the problem: Make sure you know whether you need range queries, point updates, or range updates.
- Start simple: Begin with a basic implementation and add complexity (like lazy propagation) only if needed.
- Be mindful of edge cases: Pay attention to empty ranges, single-element ranges, and out-of-bounds queries.
- Optimize space: If asked, discuss ways to optimize the space usage of your Segment Tree.
- Consider alternatives: Be prepared to discuss when other data structures (like Fenwick Trees) might be more appropriate.
Common pitfalls to avoid:
- Forgetting to propagate lazy updates
- Incorrect handling of range boundaries
- Overlooking the need for tree updates after modifying the underlying array
- Inefficient implementation leading to Time Limit Exceeded (TLE) errors
9. Practice Problems and Resources
To master Segment Trees, practice is key. Here are some resources and problems to help you improve:
- LeetCode:
- Range Sum Query – Mutable
- Count of Smaller Numbers After Self
- Range Module
- Codeforces:
- Segment Tree for the Sum
- Segment Tree for the Minimum
- Distinct Characters Queries
- HackerRank:
- Array and simple queries
- Roy and Coin Boxes
- SPOJ:
- KQUERY – K-query
- MULTQ3 – Multiples of 3
Additional resources:
- CP-Algorithms: Comprehensive guide on Segment Trees
- Topcoder Tutorials: Segment Trees
- GeeksforGeeks: Segment Tree Data Structure
10. Conclusion
Segment Trees are a powerful and versatile data structure that can significantly improve the efficiency of range query and update operations. By mastering Segment Trees, you’ll be well-equipped to tackle a wide range of problems in competitive programming and technical interviews, especially for top tech companies.
Remember that proficiency comes with practice. Start with basic implementations, gradually move to more complex variations, and consistently solve problems that utilize Segment Trees. With time and effort, you’ll develop the intuition to recognize when and how to apply Segment Trees effectively in various scenarios.
As you continue your journey in algorithmic problem-solving, keep exploring related data structures like Fenwick Trees (Binary Indexed Trees) and sparse tables, which can sometimes offer simpler or more efficient solutions for specific types of range queries. The key is to have a diverse toolkit and the wisdom to choose the right tool for each problem.
Happy coding, and may your Segment Trees always be balanced and efficient!