Mastering Recursion in Programming: A Comprehensive Guide
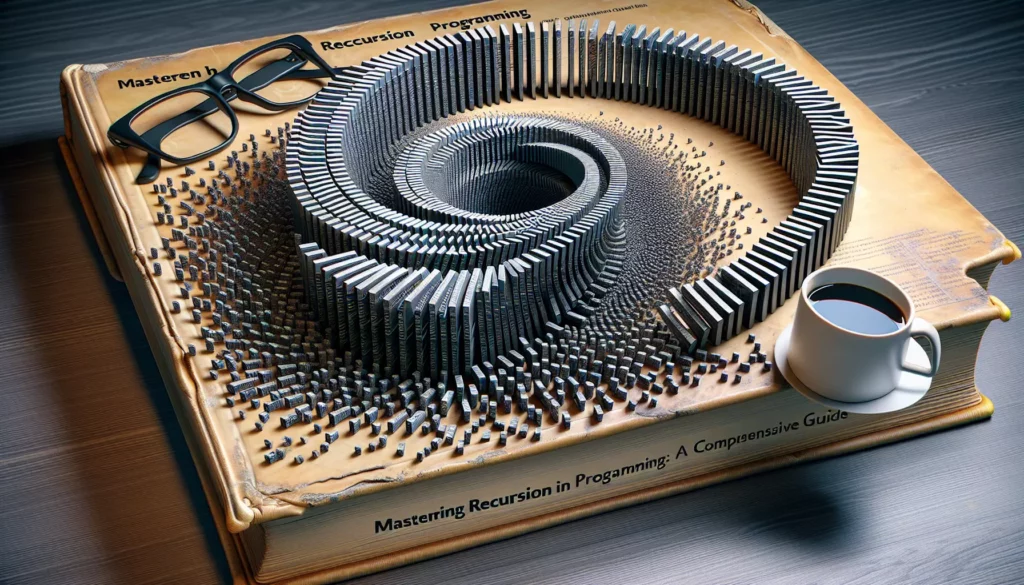
Recursion is a fundamental concept in programming that often challenges both beginners and experienced developers. It’s a powerful technique that allows you to solve complex problems by breaking them down into smaller, more manageable pieces. In this comprehensive guide, we’ll explore the ins and outs of recursion, providing you with the knowledge and tools to master this essential programming skill.
Table of Contents
- What is Recursion?
- How Recursion Works
- Anatomy of a Recursive Function
- Types of Recursion
- Common Recursive Problems
- Advantages and Disadvantages of Recursion
- Recursion vs. Iteration
- Debugging Recursive Functions
- Optimization Techniques for Recursive Functions
- Real-World Applications of Recursion
- Best Practices for Writing Recursive Functions
- Common Pitfalls and How to Avoid Them
- Recursion in Technical Interviews
- Conclusion
1. What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. It’s based on the principle of solving a complex problem by breaking it down into simpler subproblems. Each recursive call works on a smaller instance of the same problem, eventually reaching a base case that can be solved directly.
The concept of recursion is deeply rooted in mathematics and computer science. It’s often used to solve problems that have a naturally recursive structure, such as traversing tree-like data structures or implementing divide-and-conquer algorithms.
2. How Recursion Works
To understand how recursion works, let’s break it down into steps:
- Base Case: Every recursive function must have at least one base case. This is the condition that stops the recursion and provides a direct answer for the simplest version of the problem.
- Recursive Case: This is where the function calls itself with a modified input, working towards the base case.
- Progress Towards Base Case: Each recursive call should move closer to the base case, ensuring that the recursion will eventually terminate.
- Combine Results: As the recursive calls return, their results are combined to solve the original problem.
Let’s illustrate this with a classic example: calculating the factorial of a number.
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
else:
return n * factorial(n - 1)
In this example:
- The base case is when n is 0 or 1, where the factorial is defined as 1.
- The recursive case multiplies n by the factorial of (n-1).
- Each recursive call reduces n by 1, progressing towards the base case.
- The results are combined through multiplication as the calls return.
3. Anatomy of a Recursive Function
A well-structured recursive function typically consists of the following components:
- Base Case(s): The condition(s) that determine when the recursion should stop. There can be one or more base cases.
- Recursive Case: The part of the function where it calls itself with a modified input.
- Processing Logic: Any additional logic needed to process or combine the results of recursive calls.
- Return Statement: The value that the function returns, which can be a direct value (in base cases) or a combination of recursive call results.
Here’s a template for a basic recursive function:
def recursive_function(input):
# Base case(s)
if base_condition:
return base_result
# Recursive case
else:
# Process the input
modified_input = process(input)
# Recursive call
recursive_result = recursive_function(modified_input)
# Combine results
final_result = combine(input, recursive_result)
return final_result
4. Types of Recursion
There are several types of recursion, each with its own characteristics and use cases:
4.1 Direct Recursion
This is the most common type, where a function calls itself directly. The factorial example above is a case of direct recursion.
4.2 Indirect Recursion
In indirect recursion, function A calls function B, which in turn calls function A. This creates a cycle of function calls.
def is_even(n):
if n == 0:
return True
else:
return is_odd(n - 1)
def is_odd(n):
if n == 0:
return False
else:
return is_even(n - 1)
4.3 Tail Recursion
Tail recursion occurs when the recursive call is the last operation in the function. Many modern compilers can optimize tail-recursive functions to be as efficient as iterative solutions.
def factorial_tail(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return factorial_tail(n - 1, n * accumulator)
4.4 Multiple Recursion
Multiple recursion happens when a function makes more than one recursive call. The Fibonacci sequence is a classic example of multiple recursion.
def fibonacci(n):
if n
5. Common Recursive Problems
Many classic programming problems are naturally suited to recursive solutions. Here are some common examples:
5.1 Factorial Calculation
We’ve already seen this example earlier. It’s a straightforward application of recursion.
5.2 Fibonacci Sequence
The Fibonacci sequence is defined recursively, making it a natural fit for a recursive solution (although not always the most efficient one).
5.3 Tree Traversal
Traversing tree-like data structures (such as binary trees or file systems) is often implemented recursively.
class TreeNode:
def __init__(self, value, left=None, right=None):
self.value = value
self.left = left
self.right = right
def inorder_traversal(node):
if node:
inorder_traversal(node.left)
print(node.value)
inorder_traversal(node.right)
5.4 Tower of Hanoi
The Tower of Hanoi puzzle is a classic problem that demonstrates the power of recursion in solving complex problems.
def tower_of_hanoi(n, source, auxiliary, destination):
if n == 1:
print(f"Move disk 1 from {source} to {destination}")
return
tower_of_hanoi(n-1, source, destination, auxiliary)
print(f"Move disk {n} from {source} to {destination}")
tower_of_hanoi(n-1, auxiliary, source, destination)
5.5 Quicksort
The Quicksort algorithm is an efficient sorting algorithm that uses recursion to divide and conquer the sorting problem.
def quicksort(arr):
if len(arr)
6. Advantages and Disadvantages of Recursion
Advantages:
- Elegant and Concise: Recursive solutions can often express complex algorithms in a more readable and elegant way.
- Naturally Fits Problem Structure: For problems with a recursive structure, like tree traversal, recursive solutions can be more intuitive.
- Divide and Conquer: Recursion is excellent for implementing divide-and-conquer algorithms.
- Reduced Need for Complex Loop Structures: Some problems that would require complex nested loops can be solved more cleanly with recursion.
Disadvantages:
- Stack Overflow Risk: Deep recursion can lead to stack overflow errors if not managed properly.
- Memory Overhead: Each recursive call adds a new layer to the call stack, which can be memory-intensive for deep recursions.
- Potential for Inefficiency: Naive recursive implementations of some algorithms (like Fibonacci) can be significantly slower than their iterative counterparts.
- Debugging Challenges: Recursive functions can be more difficult to debug and understand, especially for beginners.
7. Recursion vs. Iteration
Both recursion and iteration are fundamental programming techniques used to repeat a set of instructions. While they can often be used interchangeably, each has its strengths and weaknesses.
Recursion:
- More intuitive for problems with a recursive nature
- Can lead to cleaner, more readable code for certain problems
- May have higher memory usage due to the call stack
- Can be slower due to function call overhead
Iteration:
- Generally more efficient in terms of memory usage
- Often faster due to lack of function call overhead
- Can be more intuitive for simple repetitive tasks
- May lead to more complex code for problems with a recursive structure
Let’s compare recursive and iterative solutions for calculating factorial:
def factorial_recursive(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial_recursive(n - 1)
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
In this case, the iterative solution might be slightly more efficient, but the recursive solution is arguably more elegant and closer to the mathematical definition of factorial.
8. Debugging Recursive Functions
Debugging recursive functions can be challenging due to their self-referential nature. Here are some strategies to make the process easier:
8.1 Use Print Statements
Adding print statements at key points in your recursive function can help you visualize the flow of execution and the values of variables at each recursive call.
def factorial(n):
print(f"Calculating factorial({n})")
if n == 0 or n == 1:
print(f"Base case reached: factorial({n}) = 1")
return 1
else:
result = n * factorial(n - 1)
print(f"factorial({n}) = {result}")
return result
8.2 Limit Recursion Depth
When testing, you can add a parameter to limit the recursion depth. This can help prevent stack overflow errors and make it easier to debug the function’s behavior for small inputs.
def factorial(n, depth=0, max_depth=10):
if depth > max_depth:
raise Exception("Max recursion depth exceeded")
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1, depth + 1, max_depth)
8.3 Use a Debugger
Most modern IDEs come with powerful debuggers that allow you to step through your code line by line. This can be particularly useful for understanding the flow of recursive functions.
8.4 Visualize the Recursion Tree
For more complex recursive algorithms, it can be helpful to draw out the recursion tree on paper or using a diagramming tool. This can give you a visual representation of how the function calls itself and how the results are combined.
9. Optimization Techniques for Recursive Functions
While recursion can lead to elegant solutions, it’s not always the most efficient approach. Here are some techniques to optimize recursive functions:
9.1 Tail Recursion Optimization
Tail recursion occurs when the recursive call is the last operation in the function. Many modern compilers can optimize tail-recursive functions to be as efficient as iterative solutions.
def factorial_tail(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return factorial_tail(n - 1, n * accumulator)
9.2 Memoization
Memoization involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This can significantly improve performance for functions with overlapping subproblems, like the naive recursive Fibonacci implementation.
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n
9.3 Dynamic Programming
Dynamic programming is an optimization technique that solves complex problems by breaking them down into simpler subproblems. It’s often used to optimize recursive solutions by solving and storing subproblems iteratively.
def fibonacci_dp(n):
if n
10. Real-World Applications of Recursion
Recursion isn’t just a theoretical concept; it has numerous practical applications in software development:
10.1 File System Operations
Recursion is often used for operations that need to traverse directory structures, such as searching for files or calculating the total size of a directory.
import os
def get_directory_size(path):
total_size = 0
for dirpath, dirnames, filenames in os.walk(path):
for f in filenames:
fp = os.path.join(dirpath, f)
total_size += os.path.getsize(fp)
return total_size
10.2 Parsing and Compiling
Recursive descent parsers use recursion to parse complex language structures. This technique is used in many compilers and interpreters.
10.3 Graph Algorithms
Many graph algorithms, such as depth-first search, are naturally recursive.
def dfs(graph, node, visited=None):
if visited is None:
visited = set()
visited.add(node)
print(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
10.4 Fractal Generation
Fractals, with their self-similar structures, are often generated using recursive algorithms.
10.5 Backtracking Algorithms
Many puzzle-solving algorithms, like solving Sudoku or generating mazes, use recursive backtracking.
11. Best Practices for Writing Recursive Functions
To write effective and efficient recursive functions, consider the following best practices:
11.1 Ensure a Valid Base Case
Always include at least one base case that doesn’t involve a recursive call. This ensures that your function will terminate.
11.2 Progress Towards the Base Case
Each recursive call should move closer to the base case. Failing to do so will result in infinite recursion.
11.3 Consider the Stack
Be mindful of the call stack’s size. Deep recursion can lead to stack overflow errors. Consider tail recursion or iterative solutions for very deep recursions.
11.4 Avoid Redundant Calculations
Use techniques like memoization to avoid recalculating the same values multiple times.
11.5 Keep It Simple
Recursive functions should be focused on a single task. If your function is becoming too complex, consider breaking it down into smaller functions.
11.6 Test with Small Inputs First
Always test your recursive functions with small, manageable inputs before moving on to larger ones. This makes debugging easier.
12. Common Pitfalls and How to Avoid Them
When working with recursion, be aware of these common pitfalls:
12.1 Infinite Recursion
This occurs when the base case is never reached. Always ensure that your recursive calls are moving towards the base case.
12.2 Stack Overflow
Deep recursion can exhaust the call stack. Use tail recursion or consider an iterative solution for very deep recursions.
12.3 Excessive Memory Usage
Each recursive call adds to the memory usage. For problems with many recursive calls, consider memoization or dynamic programming techniques.
12.4 Redundant Calculations
Naive recursive implementations can lead to the same subproblems being solved multiple times. Use memoization to cache results.
12.5 Overlooking Edge Cases
Make sure your base cases cover all possible edge cases, including negative numbers or empty inputs where applicable.
13. Recursion in Technical Interviews
Recursion is a popular topic in technical interviews, especially for positions at major tech companies. Here’s how to approach recursive problems in an interview setting:
13.1 Identify the Recursive Structure
Look for problems that can be broken down into smaller, similar subproblems. Tree and graph problems often have a recursive nature.
13.2 Start with the Base Case
Begin by identifying and coding the base case(s). This sets the foundation for your recursive solution.
13.3 Develop the Recursive Case
Think about how to reduce the problem to a smaller instance of itself.
13.4 Consider Time and Space Complexity
Be prepared to discuss the time and space complexity of your recursive solution. Consider optimizations if necessary.
13.5 Practice Common Recursive Problems
Familiarize yourself with classic recursive problems like tree traversal, backtracking problems, and divide-and-conquer algorithms.
13.6 Be Ready to Explain Your Thought Process
Interviewers are often more interested in your problem-solving approach than in perfect code. Explain your thinking as you develop your solution.
14. Conclusion
Mastering recursion is a crucial step in becoming a proficient programmer. It’s a powerful technique that can lead to elegant solutions for complex problems. However, it’s important to use recursion judiciously, considering its advantages and limitations.
Remember that becoming comfortable with recursion takes practice. Start with simple problems and gradually work your way up to more complex ones. Don’t be discouraged if it doesn’t click immediately – recursion can be a challenging concept to grasp at first, but with persistence, it will become a valuable tool in your programming toolkit.
As you continue your journey in programming, you’ll find that understanding recursion deeply will not only help you solve specific problems but will also enhance your overall problem-solving skills and algorithmic thinking. Whether you’re preparing for technical interviews, working on complex software projects, or simply expanding your coding knowledge, a strong grasp of recursion will serve you well.
Keep practicing, stay curious, and don’t hesitate to dive deep into recursive problems. With time and effort, you’ll find yourself confidently tackling recursive challenges and using this powerful technique to create efficient and elegant solutions.