Mastering Recursion in Programming: A Comprehensive Guide
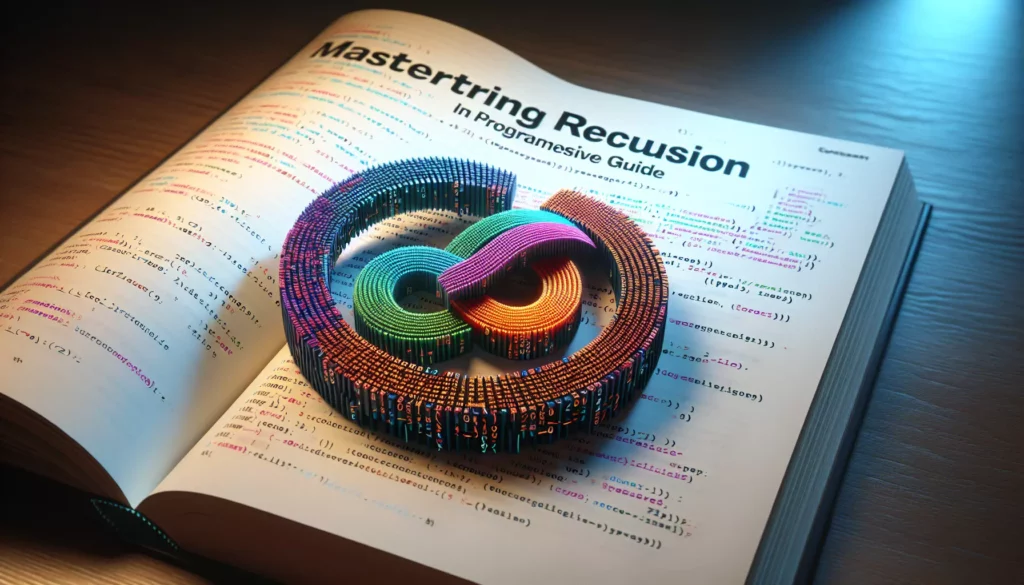
Recursion is a fundamental concept in programming that often challenges beginners and experienced developers alike. It’s a powerful technique that allows a function to call itself, enabling elegant solutions to complex problems. In this comprehensive guide, we’ll explore the ins and outs of recursion, providing you with the knowledge and tools to master this essential programming skill.
Table of Contents
- What is Recursion?
- How Recursion Works
- Base Case and Recursive Case
- Advantages of Recursion
- Common Pitfalls and How to Avoid Them
- Recursive vs. Iterative Approaches
- Classic Recursive Problems
- Advanced Recursion Techniques
- Recursion in Technical Interviews
- Practice and Resources
1. What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, more manageable subproblems. It’s based on the principle of mathematical induction and is widely used in various algorithms and data structures.
At its core, recursion involves:
- A function that calls itself
- A base case that stops the recursion
- A recursive case that continues the process
Recursion can be an incredibly powerful tool when used correctly, allowing for elegant and concise solutions to problems that might otherwise require complex iterative approaches.
2. How Recursion Works
To understand how recursion works, let’s break down the process step by step:
- A function is called with a set of parameters.
- The function checks if the base case is met. If so, it returns a result without further recursion.
- If the base case is not met, the function performs some operations and then calls itself with modified parameters.
- This process repeats until the base case is reached.
- Once the base case is hit, the function starts returning values back up the chain of recursive calls.
Let’s illustrate this with a simple example of calculating the factorial of a number:
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
else: # Recursive case
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
In this example, the function calls itself with a smaller value of n until it reaches the base case (n = 0 or 1). Then it starts returning values, multiplying them as it goes back up the chain of calls.
3. Base Case and Recursive Case
Understanding the base case and recursive case is crucial for mastering recursion:
Base Case
The base case is the condition that stops the recursion. It’s typically the simplest form of the problem that can be solved directly without further recursive calls. In our factorial example, the base case is when n is 0 or 1, as we know the factorial of these numbers is 1.
Recursive Case
The recursive case is where the function calls itself with a modified version of the problem. It should always move towards the base case. In the factorial example, we reduce n by 1 in each recursive call, moving closer to the base case.
A well-designed recursive function must have:
- At least one base case
- At least one recursive case
- A way to progress towards the base case in each recursive call
4. Advantages of Recursion
Recursion offers several advantages in programming:
- Simplicity and Elegance: Recursive solutions can often be more concise and easier to understand than their iterative counterparts, especially for problems that have a naturally recursive structure.
- Solving Complex Problems: Some problems, like traversing tree-like data structures or implementing backtracking algorithms, are more intuitively solved using recursion.
- Code Reusability: Recursive functions can be more modular and reusable, as they often encapsulate the entire problem-solving logic in a single function.
- Mathematical Correspondence: Many mathematical concepts (like factorial, Fibonacci sequence) have a direct recursive definition, making recursive implementations more intuitive.
5. Common Pitfalls and How to Avoid Them
While recursion is powerful, it comes with its own set of challenges. Here are some common pitfalls and how to avoid them:
Infinite Recursion
This occurs when the base case is never reached, causing the function to call itself indefinitely.
How to avoid: Ensure that your recursive case always moves towards the base case. Double-check your base case condition to make sure it’s reachable.
Stack Overflow
Each recursive call adds a new frame to the call stack. Too many recursive calls can lead to a stack overflow error.
How to avoid: Use tail recursion when possible, as some languages optimize it. For deep recursions, consider iterative solutions or techniques like memoization.
Redundant Calculations
Naive recursive implementations can lead to the same subproblems being solved multiple times.
How to avoid: Use memoization or dynamic programming techniques to store and reuse results of subproblems.
Incorrect Base Case
An incorrectly defined base case can lead to wrong results or infinite recursion.
How to avoid: Carefully consider all possible inputs and edge cases when defining your base case.
6. Recursive vs. Iterative Approaches
While recursion can provide elegant solutions, it’s not always the best approach. Let’s compare recursive and iterative solutions:
Recursive Approach
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
print(fibonacci_recursive(10)) # Output: 55
Iterative Approach
def fibonacci_iterative(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n+1):
a, b = b, a + b
return b
print(fibonacci_iterative(10)) # Output: 55
Considerations:
- Performance: Iterative solutions are often more efficient in terms of time and space complexity.
- Readability: Recursive solutions can be more intuitive for naturally recursive problems.
- Stack Usage: Recursive solutions use more stack space, which can be a limitation for large inputs.
- Debugging: Iterative solutions are generally easier to debug and trace.
The choice between recursive and iterative approaches depends on the specific problem, performance requirements, and personal or team preferences.
7. Classic Recursive Problems
To master recursion, it’s essential to practice with classic problems. Here are some well-known recursive problems to tackle:
Factorial Calculation
We’ve already seen this example, but it’s a fundamental recursive problem.
Fibonacci Sequence
We’ve shown both recursive and iterative approaches for this classic problem.
Tower of Hanoi
This problem involves moving a stack of disks from one peg to another, following specific rules.
def tower_of_hanoi(n, source, auxiliary, destination):
if n == 1:
print(f"Move disk 1 from {source} to {destination}")
return
tower_of_hanoi(n-1, source, destination, auxiliary)
print(f"Move disk {n} from {source} to {destination}")
tower_of_hanoi(n-1, auxiliary, source, destination)
tower_of_hanoi(3, 'A', 'B', 'C')
Binary Tree Traversal
Recursion is commonly used for traversing tree-like data structures.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
if not root:
return
inorder_traversal(root.left)
print(root.val, end=' ')
inorder_traversal(root.right)
# Example usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
inorder_traversal(root) # Output: 4 2 5 1 3
8. Advanced Recursion Techniques
As you become more comfortable with basic recursion, you can explore advanced techniques to solve more complex problems efficiently:
Tail Recursion
Tail recursion is a recursive call that is the last operation in a function. Some programming languages and compilers can optimize tail recursion to use constant stack space.
def factorial_tail(n, accumulator=1):
if n == 0:
return accumulator
return factorial_tail(n - 1, n * accumulator)
print(factorial_tail(5)) # Output: 120
Memoization
Memoization is a technique used to store the results of expensive function calls and return the cached result when the same inputs occur again. It’s particularly useful in recursive algorithms that solve overlapping subproblems.
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
print(fibonacci_memo(100)) # Output: 354224848179261915075
Divide and Conquer
This technique involves breaking a problem into smaller subproblems, solving them recursively, and then combining the results. Merge sort is a classic example of this approach.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
print(merge_sort([38, 27, 43, 3, 9, 82, 10])) # Output: [3, 9, 10, 27, 38, 43, 82]
9. Recursion in Technical Interviews
Recursion is a popular topic in technical interviews, especially for roles at major tech companies. Here are some tips for tackling recursive problems in interviews:
- Identify the Base Case: Always start by clearly defining the simplest case that doesn’t require further recursion.
- Formulate the Recursive Case: Determine how the problem can be broken down into smaller subproblems.
- Think Out Loud: Explain your thought process as you develop the recursive solution.
- Consider Edge Cases: Think about potential edge cases and how your recursive solution handles them.
- Analyze Time and Space Complexity: Be prepared to discuss the efficiency of your recursive solution.
- Practice Common Patterns: Familiarize yourself with common recursive patterns like tree traversals, backtracking, and divide-and-conquer algorithms.
Remember, interviewers are often more interested in your problem-solving approach than in a perfect implementation. Don’t hesitate to ask clarifying questions and discuss trade-offs between recursive and iterative solutions.
10. Practice and Resources
To truly master recursion, consistent practice is key. Here are some resources and platforms to help you hone your recursive problem-solving skills:
Online Platforms
- LeetCode: Offers a wide range of problems, many of which can be solved recursively.
- HackerRank: Provides a “Recursion” track with various difficulty levels.
- CodeSignal: Features interview practice problems, including recursive challenges.
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning recursion and other programming concepts.
Books
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Grokking Algorithms” by Aditya Bhargava
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
Online Courses
- Coursera’s “Algorithms” specialization by Stanford University
- edX’s “Algorithm Design and Analysis” by PennX
- Udacity’s “Intro to Algorithms” course
Practice Tips
- Start with simple problems and gradually increase complexity.
- Implement both recursive and iterative solutions to understand trade-offs.
- Analyze the time and space complexity of your solutions.
- Practice explaining your recursive solutions out loud, as if in an interview.
- Join coding communities or study groups to discuss and share recursive problem-solving strategies.
Conclusion
Mastering recursion is a journey that requires patience, practice, and persistence. By understanding the fundamental concepts, recognizing common patterns, and consistently practicing with diverse problems, you can develop a strong intuition for recursive problem-solving.
Remember that recursion is not just a programming technique; it’s a powerful way of thinking about problems. As you become more comfortable with recursive approaches, you’ll find that many complex problems become more manageable and elegant to solve.
Whether you’re preparing for technical interviews, working on algorithmic challenges, or simply aiming to expand your programming toolkit, a solid grasp of recursion will serve you well throughout your coding career. Keep practicing, stay curious, and don’t be afraid to tackle increasingly complex recursive problems. With time and effort, you’ll find recursion becoming a natural and powerful tool in your programming arsenal.