Mastering Recursion: A Step-by-Step Approach
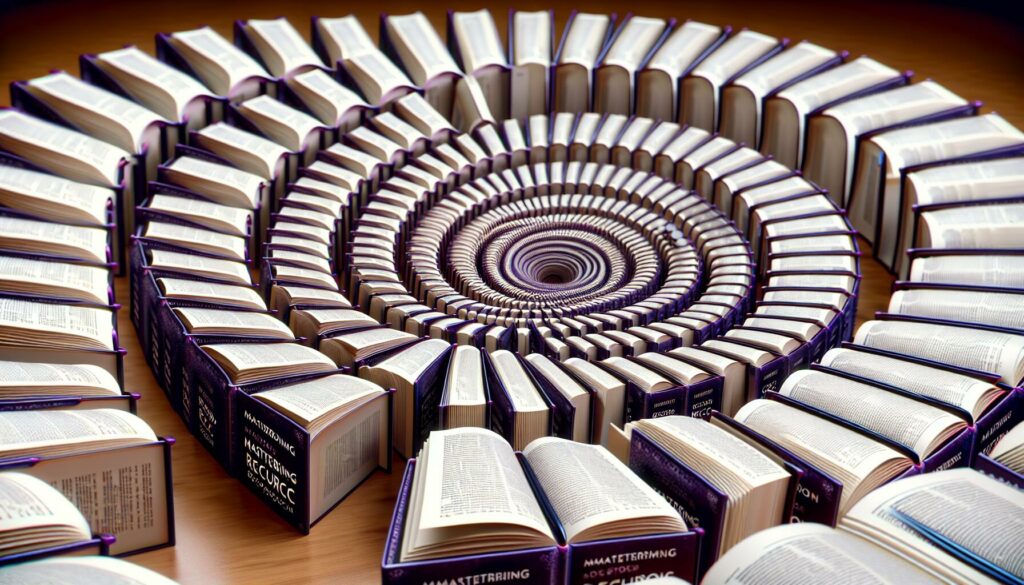
Recursion is a fundamental concept in computer science and programming that often challenges beginners and experienced developers alike. It’s a powerful technique that allows a function to call itself, solving complex problems by breaking them down into smaller, more manageable pieces. In this comprehensive guide, we’ll explore recursion in depth, providing a step-by-step approach to understanding and mastering this essential programming concept.
Table of Contents
- What is Recursion?
- Why Use Recursion?
- The Anatomy of a Recursive Function
- Base Case and Recursive Case
- Examples of Recursion
- Common Pitfalls and How to Avoid Them
- Recursion vs. Iteration
- Tail Recursion and Optimization
- Advanced Recursive Techniques
- Practice Problems
- Conclusion
1. What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. It’s based on the principle of solving a larger problem by breaking it down into smaller, similar subproblems. Each recursive call works on a smaller instance of the same problem, eventually reaching a point where the problem is small enough to be solved directly.
The key to understanding recursion is recognizing that a complex problem can often be reduced to a simpler version of the same problem. For example, calculating the factorial of a number n can be expressed as n multiplied by the factorial of (n-1).
2. Why Use Recursion?
Recursion offers several advantages in programming:
- Elegant Solutions: Recursive solutions can be more elegant and easier to understand for problems that have a naturally recursive structure.
- Divide and Conquer: It’s excellent for solving problems that can be broken down into smaller, similar subproblems.
- Reduced Complexity: Some complex problems become simpler when approached recursively.
- Tree-like Structures: Recursion is particularly useful for working with tree-like data structures, such as file systems, XML parsing, or traversing binary trees.
3. The Anatomy of a Recursive Function
A recursive function typically consists of two main parts:
- Base Case: The condition under which the function stops calling itself.
- Recursive Case: The part where the function calls itself with a modified input.
Here’s a simple example of a recursive function that calculates the factorial of a number:
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
else:
return n * factorial(n - 1)
4. Base Case and Recursive Case
Understanding the base case and recursive case is crucial for writing effective recursive functions:
Base Case
The base case is the condition that stops the recursion. It’s typically the simplest form of the problem that can be solved directly. Without a proper base case, the function would call itself indefinitely, leading to a stack overflow error.
Recursive Case
The recursive case is where the function calls itself with a modified input. This modified input should move the problem closer to the base case with each recursive call.
Let’s break down our factorial example:
def factorial(n):
# Base case
if n == 0 or n == 1:
return 1
# Recursive case
else:
return n * factorial(n - 1)
In this function:
- The base case is when n is 0 or 1, as the factorial of 0 and 1 is 1.
- The recursive case multiplies n by the factorial of (n-1), moving closer to the base case with each call.
5. Examples of Recursion
Let’s explore some classic examples of recursion to deepen our understanding:
Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. It’s a perfect candidate for recursion:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
Binary Search
Binary search is an efficient algorithm for finding an item in a sorted list. Here’s a recursive implementation:
def binary_search(arr, target, low, high):
if high >= low:
mid = (high + low) // 2
if arr[mid] == target:
return mid
elif arr[mid] > target:
return binary_search(arr, target, low, mid - 1)
else:
return binary_search(arr, target, mid + 1, high)
else:
return -1
Tree Traversal
Recursion is particularly useful for traversing tree-like structures. Here’s an example of in-order traversal of a binary tree:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.value)
inorder_traversal(root.right)
6. Common Pitfalls and How to Avoid Them
While recursion is powerful, it comes with potential pitfalls:
Infinite Recursion
This occurs when the base case is never reached. Always ensure your recursive case moves towards the base case.
Stack Overflow
Excessive recursive calls can lead to stack overflow. Consider using tail recursion or iterative solutions for deep recursions.
Redundant Calculations
Some recursive algorithms (like the naive Fibonacci implementation) recalculate the same values multiple times. Use memoization or dynamic programming to optimize these cases.
Excessive Memory Usage
Each recursive call adds a new layer to the call stack, potentially using a lot of memory. Be mindful of this, especially for large inputs.
7. Recursion vs. Iteration
While recursion can provide elegant solutions, it’s not always the best choice. Let’s compare recursion and iteration:
Recursion
- Often leads to more readable and maintainable code for certain problems
- Well-suited for problems with recursive structures (like trees)
- Can be less efficient due to function call overhead
- Risk of stack overflow for deep recursions
Iteration
- Generally more efficient in terms of memory and speed
- No risk of stack overflow
- Can be more complex for problems with recursive nature
- Often preferred for simple repetitive tasks
Here’s an example of calculating factorial using both approaches:
# Recursive approach
def factorial_recursive(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial_recursive(n - 1)
# Iterative approach
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
Choose the approach that best fits your specific problem and constraints.
8. Tail Recursion and Optimization
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Many modern compilers can optimize tail-recursive functions to be as efficient as iterative loops.
Here’s an example of tail recursion for calculating factorial:
def factorial_tail(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return factorial_tail(n - 1, n * accumulator)
In languages that support tail call optimization (TCO), this version can be more efficient and avoid stack overflow for large inputs.
9. Advanced Recursive Techniques
As you become more comfortable with recursion, you can explore advanced techniques:
Memoization
Memoization involves caching the results of expensive function calls to avoid redundant calculations. It’s particularly useful for optimizing recursive algorithms like Fibonacci:
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
Mutual Recursion
Mutual recursion involves two or more functions calling each other. It’s useful for problems that naturally divide into multiple interdependent subproblems:
def is_even(n):
if n == 0:
return True
else:
return is_odd(n - 1)
def is_odd(n):
if n == 0:
return False
else:
return is_even(n - 1)
Nested Recursion
In nested recursion, the recursive call is used as an argument to another recursive call. This is an advanced technique used in complex mathematical functions:
def ackermann(m, n):
if m == 0:
return n + 1
elif n == 0:
return ackermann(m - 1, 1)
else:
return ackermann(m - 1, ackermann(m, n - 1))
10. Practice Problems
To truly master recursion, practice is essential. Here are some problems to work on:
- Sum of Natural Numbers: Write a recursive function to calculate the sum of first n natural numbers.
- Power Function: Implement a recursive function to calculate x^n.
- Palindrome Check: Create a recursive function to check if a string is a palindrome.
- Merge Sort: Implement the merge sort algorithm recursively.
- Tower of Hanoi: Solve the Tower of Hanoi puzzle using recursion.
Here’s a sample solution for the first problem:
def sum_natural_numbers(n):
if n <= 1:
return n
else:
return n + sum_natural_numbers(n - 1)
11. Conclusion
Mastering recursion is a journey that requires practice and patience. It’s a powerful tool in a programmer’s arsenal, enabling elegant solutions to complex problems. As you work through recursive problems, remember these key points:
- Always identify the base case and ensure the recursive case moves towards it.
- Consider the trade-offs between recursion and iteration for each problem.
- Be mindful of potential pitfalls like stack overflow and redundant calculations.
- Explore optimization techniques like memoization and tail recursion when appropriate.
- Practice regularly with a variety of problems to build your recursive thinking skills.
Recursion is not just a programming technique; it’s a way of thinking about problem-solving. As you become more comfortable with recursive approaches, you’ll find that many complex problems become more manageable and elegant to solve. Keep practicing, and soon you’ll be tackling recursive problems with confidence and skill.