Mastering Python’s Enumerate Function: A Comprehensive Guide
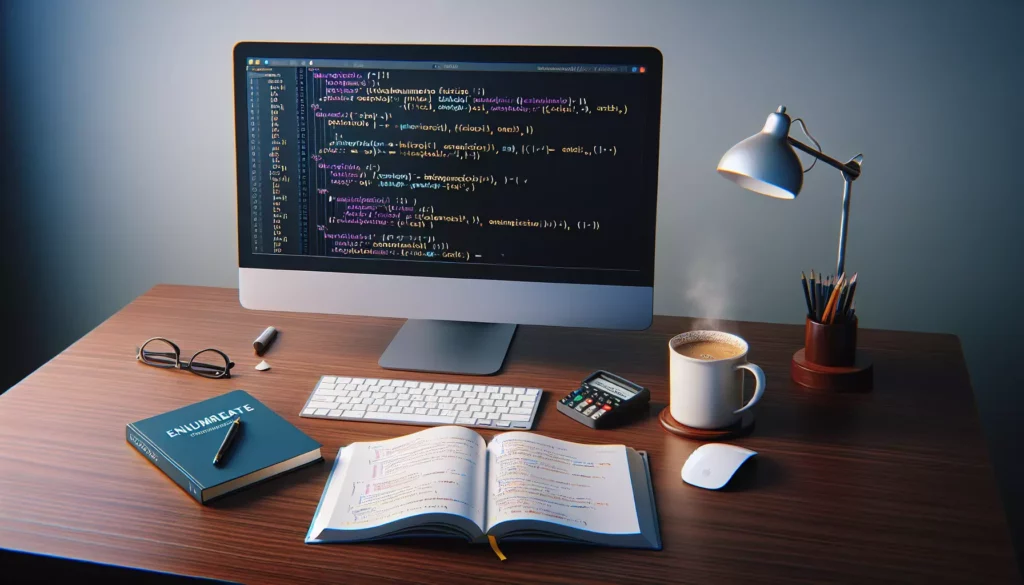
Python is known for its simplicity and readability, and one of the features that contributes to this reputation is the enumerate()
function. This powerful built-in function allows developers to loop over sequences while simultaneously accessing both the index and the value of each element. In this comprehensive guide, we’ll explore the ins and outs of enumerate()
, its various use cases, and how it can make your Python code more efficient and elegant.
Table of Contents
- What is enumerate()?
- Basic Usage of enumerate()
- Customizing the Starting Index
- enumerate() with Different Data Types
- Performance Considerations
- Common Use Cases
- Advanced Techniques with enumerate()
- enumerate() in List Comprehensions
- Combining enumerate() with Other Itertools
- Best Practices and Tips
- Common Pitfalls and How to Avoid Them
- Alternatives to enumerate()
- enumerate() in Python 2 vs Python 3
- Conclusion
1. What is enumerate()?
The enumerate()
function is a built-in Python function that allows you to iterate over a sequence (such as a list, tuple, or string) while keeping track of the index of each element. It returns an iterator of tuples, where each tuple contains the index and the corresponding value from the sequence.
The basic syntax of enumerate()
is as follows:
enumerate(iterable, start=0)
Where:
iterable
is the sequence you want to iterate overstart
is an optional parameter that specifies the starting index (default is 0)
2. Basic Usage of enumerate()
Let’s start with a simple example to demonstrate how enumerate()
works:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(f"Index: {index}, Fruit: {fruit}")
Output:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: cherry
In this example, enumerate()
allows us to access both the index and the value of each element in the fruits
list within a single loop.
3. Customizing the Starting Index
By default, enumerate()
starts counting from 0. However, you can specify a different starting index using the start
parameter:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits, start=1):
print(f"Item {index}: {fruit}")
Output:
Item 1: apple
Item 2: banana
Item 3: cherry
This feature is particularly useful when you want to create numbered lists starting from 1 or any other specific number.
4. enumerate() with Different Data Types
enumerate()
works with various iterable data types in Python. Let’s explore a few examples:
4.1 Strings
word = "Python"
for index, char in enumerate(word):
print(f"Index: {index}, Character: {char}")
Output:
Index: 0, Character: P
Index: 1, Character: y
Index: 2, Character: t
Index: 3, Character: h
Index: 4, Character: o
Index: 5, Character: n
4.2 Tuples
coordinates = (10, 20, 30)
for index, value in enumerate(coordinates):
print(f"Dimension {index + 1}: {value}")
Output:
Dimension 1: 10
Dimension 2: 20
Dimension 3: 30
4.3 Sets
unique_numbers = {5, 2, 8, 1, 9}
for index, number in enumerate(unique_numbers):
print(f"Item {index + 1}: {number}")
Note that sets are unordered, so the output order may vary:
Item 1: 1
Item 2: 2
Item 3: 5
Item 4: 8
Item 5: 9
5. Performance Considerations
enumerate()
is generally very efficient, as it doesn’t create a new list in memory. Instead, it returns an iterator, which generates the index-value pairs on-the-fly as you iterate over it. This makes it memory-efficient, especially when dealing with large sequences.
Let’s compare the performance of enumerate()
with a manual index tracking approach:
import timeit
def manual_indexing():
fruits = ['apple', 'banana', 'cherry'] * 1000
result = []
for i in range(len(fruits)):
result.append((i, fruits[i]))
return result
def using_enumerate():
fruits = ['apple', 'banana', 'cherry'] * 1000
return list(enumerate(fruits))
print("Manual indexing time:", timeit.timeit(manual_indexing, number=1000))
print("enumerate() time:", timeit.timeit(using_enumerate, number=1000))
The results will show that enumerate()
is generally faster than manual indexing, especially for larger sequences.
6. Common Use Cases
enumerate()
has numerous practical applications in Python programming. Here are some common use cases:
6.1 Filtering with Index
numbers = [10, 20, 30, 40, 50]
even_indexed = [num for index, num in enumerate(numbers) if index % 2 == 0]
print(even_indexed) # Output: [10, 30, 50]
6.2 Creating Dictionaries
fruits = ['apple', 'banana', 'cherry']
fruit_dict = {index: fruit for index, fruit in enumerate(fruits, start=1)}
print(fruit_dict) # Output: {1: 'apple', 2: 'banana', 3: 'cherry'}
6.3 Finding Indices of Specific Elements
numbers = [1, 3, 5, 3, 1, 4, 5]
indices_of_3 = [index for index, num in enumerate(numbers) if num == 3]
print(indices_of_3) # Output: [1, 3]
7. Advanced Techniques with enumerate()
Let’s explore some more advanced techniques using enumerate()
:
7.1 Nested enumerate()
You can use nested enumerate()
calls to work with multi-dimensional data structures:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row_index, row in enumerate(matrix):
for col_index, value in enumerate(row):
print(f"Position ({row_index}, {col_index}): {value}")
7.2 enumerate() with zip()
Combine enumerate()
with zip()
to iterate over multiple lists simultaneously:
names = ['Alice', 'Bob', 'Charlie']
ages = [25, 30, 35]
for index, (name, age) in enumerate(zip(names, ages)):
print(f"Person {index + 1}: {name} is {age} years old")
7.3 Reverse enumeration
To enumerate in reverse order, you can combine enumerate()
with reversed()
:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(reversed(fruits)):
print(f"Reverse index: {len(fruits) - index - 1}, Fruit: {fruit}")
8. enumerate() in List Comprehensions
enumerate()
can be used effectively in list comprehensions to create more complex data structures:
words = ['hello', 'world', 'python', 'programming']
result = [(index, word.upper()) for index, word in enumerate(words) if len(word) > 5]
print(result) # Output: [(2, 'PYTHON'), (3, 'PROGRAMMING')]
9. Combining enumerate() with Other Itertools
Python’s itertools
module provides a set of fast, memory-efficient tools for working with iterators. You can combine enumerate()
with these tools for more advanced operations:
9.1 enumerate() with itertools.chain()
from itertools import chain
list1 = ['a', 'b']
list2 = ['c', 'd']
for index, value in enumerate(chain(list1, list2)):
print(f"Index: {index}, Value: {value}")
9.2 enumerate() with itertools.islice()
from itertools import islice
numbers = range(100)
for index, value in enumerate(islice(numbers, 10, 20)):
print(f"Index: {index}, Value: {value}")
10. Best Practices and Tips
To make the most of enumerate()
, consider the following best practices and tips:
- Use meaningful variable names for both the index and value in your loop.
- Consider using the
start
parameter when appropriate, especially for creating numbered lists starting from 1. - When you only need the index or the value, use an underscore (_) as a placeholder for the unused variable.
- Remember that
enumerate()
returns an iterator, so you can’t directly index into it or get its length. - Use
enumerate()
in list comprehensions and generator expressions for more concise code.
11. Common Pitfalls and How to Avoid Them
While enumerate()
is generally straightforward to use, there are a few common pitfalls to be aware of:
11.1 Trying to enumerate a non-iterable
Make sure you’re passing an iterable object to enumerate()
:
# This will raise a TypeError
number = 42
for index, digit in enumerate(number):
print(digit)
# Correct way:
number_str = str(42)
for index, digit in enumerate(number_str):
print(digit)
11.2 Forgetting that enumerate() returns an iterator
You can’t directly index into the result of enumerate()
:
fruits = ['apple', 'banana', 'cherry']
enum_fruits = enumerate(fruits)
print(enum_fruits[0]) # This will raise a TypeError
# Correct way:
enum_fruits = list(enumerate(fruits))
print(enum_fruits[0]) # Output: (0, 'apple')
11.3 Modifying the iterable while enumerating
Be cautious when modifying the iterable you’re enumerating over, as it can lead to unexpected results:
numbers = [1, 2, 3, 4, 5]
for index, num in enumerate(numbers):
if num % 2 == 0:
numbers.remove(num) # This can cause issues
print(numbers) # Output may not be as expected
# Better approach:
numbers = [1, 2, 3, 4, 5]
numbers = [num for num in numbers if num % 2 != 0]
print(numbers) # Output: [1, 3, 5]
12. Alternatives to enumerate()
While enumerate()
is a powerful and convenient function, there are situations where alternative approaches might be more appropriate:
12.1 Using range() and len()
For simple cases, you can use range()
and len()
to achieve similar results:
fruits = ['apple', 'banana', 'cherry']
for i in range(len(fruits)):
print(f"Index: {i}, Fruit: {fruits[i]}")
12.2 Using a counter variable
In some cases, a simple counter variable might suffice:
fruits = ['apple', 'banana', 'cherry']
counter = 0
for fruit in fruits:
print(f"Index: {counter}, Fruit: {fruit}")
counter += 1
12.3 Using itertools.count()
For more advanced use cases, you can use itertools.count()
to generate indices:
from itertools import count
fruits = ['apple', 'banana', 'cherry']
for i, fruit in zip(count(), fruits):
print(f"Index: {i}, Fruit: {fruit}")
13. enumerate() in Python 2 vs Python 3
The enumerate()
function has been available since Python 2.3, but there are some differences between its implementation in Python 2 and Python 3:
13.1 Return Type
In Python 2, enumerate()
returns a list of tuples, while in Python 3, it returns an enumerate object (an iterator).
13.2 Performance
The Python 3 implementation is generally more memory-efficient, especially for large sequences, as it doesn’t create the entire list in memory at once.
13.3 Compatibility
If you need to write code that works in both Python 2 and 3, you can use the following approach:
from builtins import enumerate # From the 'future' library
# Your code using enumerate() here
This ensures that you’re using the Python 3-style enumerate()
even in Python 2.
14. Conclusion
The enumerate()
function is a powerful tool in Python that simplifies the process of iterating over sequences while keeping track of indices. Its versatility, efficiency, and readability make it an essential part of a Python programmer’s toolkit.
Throughout this comprehensive guide, we’ve explored the basics of enumerate()
, its various use cases, advanced techniques, and best practices. We’ve also discussed common pitfalls and alternatives to help you make informed decisions when working with sequences in Python.
By mastering enumerate()
, you can write more elegant, efficient, and Pythonic code. Whether you’re working on simple scripts or complex data processing tasks, enumerate()
can help you streamline your loops and improve the overall quality of your Python programs.
Remember to practice using enumerate()
in your projects, and don’t hesitate to explore its combination with other Python features and libraries. As you become more comfortable with enumerate()
, you’ll find that it opens up new possibilities for elegant and efficient sequence manipulation in your Python code.