Mastering Python’s defaultdict: A Comprehensive Guide
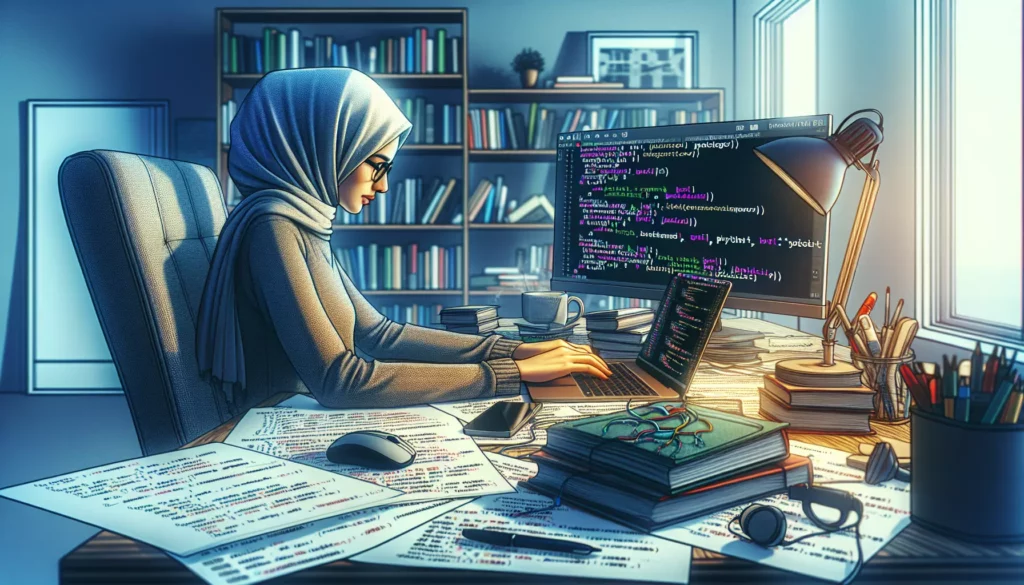
Python’s defaultdict is a powerful and versatile data structure that extends the functionality of the built-in dict class. It provides a convenient way to handle missing keys and automate the creation of default values. In this comprehensive guide, we’ll explore the ins and outs of defaultdict, its use cases, and how it can simplify your code and improve efficiency.
Table of Contents
- Introduction to defaultdict
- How defaultdict Works
- Creating and Using defaultdict
- Common Use Cases for defaultdict
- Advantages of defaultdict over Regular Dictionaries
- Advanced Techniques with defaultdict
- Best Practices and Considerations
- Comparing defaultdict to Other Data Structures
- Real-world Examples
- Conclusion
1. Introduction to defaultdict
The defaultdict is a subclass of the built-in dict class in Python. It’s part of the collections module and was introduced in Python 2.5. The primary purpose of defaultdict is to provide a dictionary-like object that can automatically handle missing keys by creating a default value when a key is accessed that doesn’t exist in the dictionary.
The main advantage of using defaultdict is that it eliminates the need for explicit key checks and initializations, leading to cleaner and more concise code. This is particularly useful when dealing with nested dictionaries or when you need to accumulate values in a dictionary.
2. How defaultdict Works
The key feature of defaultdict is its ability to provide a default value for any new key. This is achieved through a default_factory, which is a callable (such as a function or class) that returns the default value when a key is not found in the dictionary.
When you access a key that doesn’t exist in a defaultdict, instead of raising a KeyError (as a regular dictionary would), it does the following:
- Calls the default_factory to create a default value
- Inserts the key into the dictionary with the default value
- Returns the default value
This process happens automatically, allowing you to work with the dictionary without worrying about key existence or initialization.
3. Creating and Using defaultdict
To use defaultdict, you first need to import it from the collections module:
from collections import defaultdict
When creating a defaultdict, you specify the default_factory as an argument. This can be any callable that returns a value. Some common choices include:
- int: For numeric counters
- list: For creating lists as default values
- set: For creating sets as default values
- dict: For nested dictionaries
- lambda functions: For custom default values
Here’s an example of creating and using a defaultdict with int as the default_factory:
word_count = defaultdict(int)
words = ["apple", "banana", "apple", "cherry", "banana", "date"]
for word in words:
word_count[word] += 1
print(word_count)
# Output: defaultdict(<class 'int'>, {'apple': 2, 'banana': 2, 'cherry': 1, 'date': 1})
In this example, we create a defaultdict that uses int as its default_factory. This means that any new key will have a default value of 0 (the result of calling int()). We then count the occurrences of words in a list, incrementing the count for each word without worrying about initializing the count for new words.
4. Common Use Cases for defaultdict
defaultdict is particularly useful in several scenarios:
4.1. Counting Occurrences
As shown in the previous example, defaultdict is excellent for counting occurrences of items in a collection.
4.2. Grouping Items
defaultdict can be used to group items based on a certain attribute:
from collections import defaultdict
animals = [
("dog", "mammal"),
("cat", "mammal"),
("snake", "reptile"),
("lizard", "reptile"),
("dolphin", "mammal"),
]
animal_groups = defaultdict(list)
for animal, group in animals:
animal_groups[group].append(animal)
print(animal_groups)
# Output: defaultdict(<class 'list'>, {'mammal': ['dog', 'cat', 'dolphin'], 'reptile': ['snake', 'lizard']})
4.3. Building Graphs
defaultdict is useful for representing graphs, where each node can have multiple connections:
graph = defaultdict(set)
edges = [(1, 2), (1, 3), (2, 4), (3, 4), (4, 5)]
for start, end in edges:
graph[start].add(end)
graph[end].add(start) # For undirected graph
print(graph)
# Output: defaultdict(<class 'set'>, {1: {2, 3}, 2: {1, 4}, 3: {1, 4}, 4: {2, 3, 5}, 5: {4}})
4.4. Nested Dictionaries
defaultdict can be used to create nested dictionaries easily:
nested = defaultdict(lambda: defaultdict(int))
nested['outer1']['inner1'] = 10
nested['outer1']['inner2'] = 20
nested['outer2']['inner1'] = 30
print(nested)
# Output: defaultdict(<lambda>, {'outer1': defaultdict(<class 'int'>, {'inner1': 10, 'inner2': 20}), 'outer2': defaultdict(<class 'int'>, {'inner1': 30})})
5. Advantages of defaultdict over Regular Dictionaries
Using defaultdict offers several advantages over regular dictionaries:
5.1. Simplified Code
defaultdict eliminates the need for explicit key checks and initializations, resulting in cleaner and more readable code.
5.2. Reduced Boilerplate
With defaultdict, you don’t need to write repetitive code to handle missing keys, which is especially useful in data processing tasks.
5.3. Improved Performance
In scenarios where you frequently access or modify dictionary values, defaultdict can offer better performance by avoiding repeated key checks and exception handling.
5.4. Automatic Initialization
defaultdict automatically initializes new keys with the specified default value, making it easier to work with complex data structures.
6. Advanced Techniques with defaultdict
6.1. Custom Default Factories
You can create custom default factories to suit your specific needs:
def default_value():
return {'count': 0, 'sum': 0}
stats = defaultdict(default_value)
data = [('A', 10), ('B', 20), ('A', 30), ('C', 40)]
for key, value in data:
stats[key]['count'] += 1
stats[key]['sum'] += value
print(stats)
# Output: defaultdict(<function default_value at ...>, {'A': {'count': 2, 'sum': 40}, 'B': {'count': 1, 'sum': 20}, 'C': {'count': 1, 'sum': 40}})
6.2. Using lambda Functions
Lambda functions can be used as default factories for more complex default values:
from datetime import datetime
log = defaultdict(lambda: {'timestamp': datetime.now(), 'count': 0})
log['error']['count'] += 1
log['warning']['count'] += 1
log['error']['count'] += 1
print(log)
# Output: defaultdict(<lambda>, {'error': {'timestamp': datetime.datetime(...), 'count': 2}, 'warning': {'timestamp': datetime.datetime(...), 'count': 1}})
6.3. Combining with Other Data Structures
defaultdict can be combined with other data structures for more complex use cases:
from collections import defaultdict, Counter
word_stats = defaultdict(Counter)
text = "the quick brown fox jumps over the lazy dog"
for word in text.split():
word_stats[len(word)][word] += 1
print(word_stats)
# Output: defaultdict(<class 'collections.Counter'>, {3: Counter({'the': 2, 'fox': 1, 'dog': 1}), 5: Counter({'quick': 1, 'brown': 1, 'jumps': 1}), 4: Counter({'over': 1, 'lazy': 1})})
7. Best Practices and Considerations
When using defaultdict, keep the following best practices and considerations in mind:
7.1. Choose the Right Default Factory
Select a default factory that makes sense for your use case. Using int for counters, list for grouping, or set for unique collections are common patterns.
7.2. Be Aware of Side Effects
Remember that accessing a non-existent key in a defaultdict will create that key with the default value. This can lead to unexpected behavior if you’re not careful.
7.3. Use Type Annotations
When working with defaultdict in larger projects, consider using type annotations to improve code readability and catch potential errors:
from collections import defaultdict
from typing import DefaultDict, List
word_groups: DefaultDict[str, List[str]] = defaultdict(list)
word_groups['vowels'].extend(['a', 'e', 'i', 'o', 'u'])
word_groups['consonants'].extend(['b', 'c', 'd', 'f', 'g'])
7.4. Consider Performance
While defaultdict can improve performance in many cases, be mindful of the overhead of calling the default_factory for every new key. In some scenarios, a regular dictionary with explicit handling might be more efficient.
8. Comparing defaultdict to Other Data Structures
Let’s compare defaultdict to other similar data structures to understand when to use each:
8.1. defaultdict vs. dict
Use defaultdict when you need automatic handling of missing keys and want to avoid repetitive key checks and initializations. Use dict when you need more control over key access or when you don’t want automatic creation of keys.
8.2. defaultdict vs. Counter
Use defaultdict(int) for general-purpose counting. Use Counter when you specifically need counting functionality and want to use methods like most_common() or arithmetic operations between counters.
8.3. defaultdict vs. OrderedDict
Use defaultdict when order doesn’t matter and you need automatic default values. Use OrderedDict when you need to maintain the order of insertion of keys.
9. Real-world Examples
Let’s look at some real-world examples where defaultdict can be particularly useful:
9.1. Log Analysis
Suppose you’re analyzing server logs and want to group errors by their type:
from collections import defaultdict
import re
error_logs = defaultdict(list)
log_pattern = r"(\w+): (.+)"
logs = [
"Error: Database connection failed",
"Warning: Low disk space",
"Error: Authentication failed",
"Info: Server started",
"Error: File not found"
]
for log in logs:
match = re.match(log_pattern, log)
if match:
log_type, message = match.groups()
error_logs[log_type].append(message)
print(error_logs)
# Output: defaultdict(<class 'list'>, {'Error': ['Database connection failed', 'Authentication failed', 'File not found'], 'Warning': ['Low disk space'], 'Info': ['Server started']})
9.2. Word Frequency Analysis
Here’s an example of using defaultdict to analyze word frequencies in a text:
from collections import defaultdict
import re
def word_frequency(text):
words = re.findall(r'\w+', text.lower())
frequency = defaultdict(int)
for word in words:
frequency[word] += 1
return frequency
text = "To be or not to be, that is the question. Whether 'tis nobler in the mind to suffer the slings and arrows of outrageous fortune, or to take arms against a sea of troubles and by opposing end them."
freq = word_frequency(text)
print(sorted(freq.items(), key=lambda x: x[1], reverse=True)[:10])
# Output: [('to', 4), ('the', 3), ('of', 3), ('or', 2), ('be', 2), ('and', 2), ('is', 1), ('that', 1), ('question', 1), ('whether', 1)]
9.3. Building a Simple Recommendation System
Here’s a basic example of using defaultdict to build a simple recommendation system based on user preferences:
from collections import defaultdict
user_preferences = [
("Alice", "sci-fi"),
("Bob", "romance"),
("Charlie", "sci-fi"),
("David", "fantasy"),
("Alice", "fantasy"),
("Eve", "sci-fi"),
("Bob", "fantasy"),
]
genre_fans = defaultdict(set)
user_genres = defaultdict(set)
for user, genre in user_preferences:
genre_fans[genre].add(user)
user_genres[user].add(genre)
def recommend_genres(user):
recommendations = defaultdict(int)
for genre in user_genres[user]:
for fan in genre_fans[genre]:
if fan != user:
for other_genre in user_genres[fan]:
if other_genre not in user_genres[user]:
recommendations[other_genre] += 1
return sorted(recommendations.items(), key=lambda x: x[1], reverse=True)
print(recommend_genres("Alice"))
# Output: [('romance', 1)]
print(recommend_genres("Bob"))
# Output: [('sci-fi', 2)]
10. Conclusion
Python’s defaultdict is a powerful and flexible data structure that can significantly simplify your code and improve its efficiency in many scenarios. By automatically handling missing keys and providing default values, defaultdict eliminates the need for repetitive key checks and initializations, leading to cleaner and more readable code.
We’ve explored various aspects of defaultdict, including its basic usage, common use cases, advanced techniques, and real-world examples. We’ve also compared it to other data structures and discussed best practices for its use.
While defaultdict is not a silver bullet for all dictionary-related tasks, it’s an invaluable tool in a Python developer’s toolkit. By understanding when and how to use defaultdict effectively, you can write more elegant and efficient code, especially when dealing with data processing, grouping, and counting tasks.
As you continue to work with Python, keep defaultdict in mind as a go-to solution for scenarios involving automatic key initialization and default value handling. Its simplicity and power can often lead to more intuitive and maintainable code, making it a worthy addition to your Python programming arsenal.