Mastering Problem-Solving Skills in Coding: A Comprehensive Guide
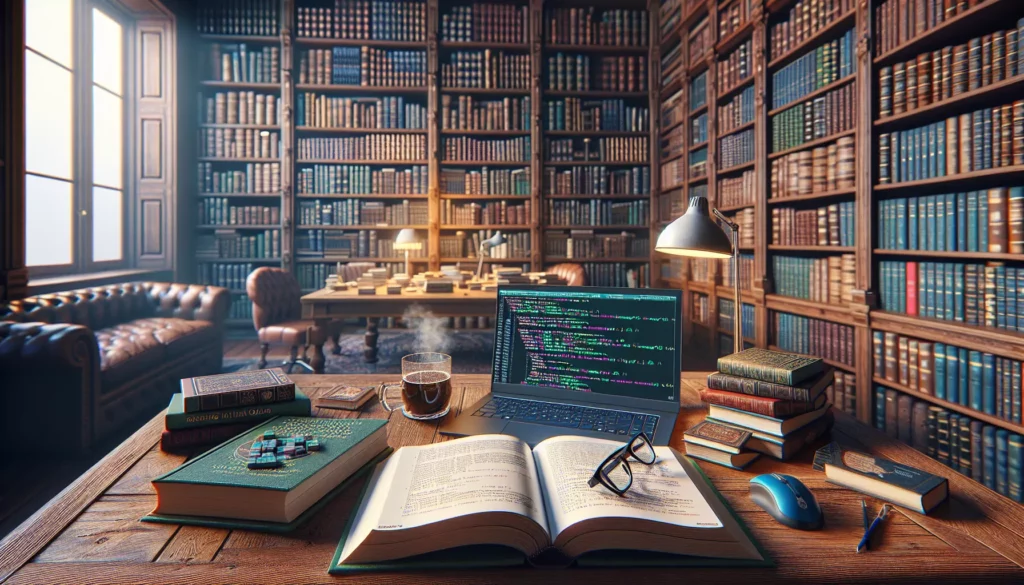
In the ever-evolving world of technology, problem-solving skills are the cornerstone of successful coding. Whether you’re a beginner just starting your journey or an experienced developer looking to sharpen your skills, improving your ability to tackle complex coding challenges is crucial. This comprehensive guide will explore various strategies and techniques to enhance your problem-solving prowess in coding, helping you become a more efficient and effective programmer.
1. Understanding the Importance of Problem-Solving in Coding
Before diving into specific techniques, it’s essential to recognize why problem-solving skills are so vital in coding:
- Foundation of Programming: At its core, coding is about solving problems. Every line of code you write is a solution to a specific challenge.
- Career Advancement: Strong problem-solving skills can set you apart in job interviews and lead to better career opportunities, especially in top tech companies.
- Efficiency: Improved problem-solving abilities lead to more efficient coding, reducing development time and resources.
- Innovation: The ability to approach problems creatively can lead to innovative solutions and breakthroughs in technology.
2. Developing a Structured Approach to Problem-Solving
One of the most effective ways to improve your problem-solving skills is to adopt a structured approach. Here’s a step-by-step method you can follow:
- Understand the Problem: Clearly define what the problem is and what outcome you’re trying to achieve.
- Break It Down: Divide the main problem into smaller, manageable sub-problems.
- Plan Your Approach: Outline the steps you’ll take to solve each sub-problem.
- Implement the Solution: Write the code to solve each sub-problem.
- Test and Debug: Verify your solution and fix any issues.
- Optimize: Look for ways to improve your solution’s efficiency.
- Reflect: Analyze your approach and consider alternative solutions.
3. Enhancing Your Algorithmic Thinking
Algorithmic thinking is a crucial skill for problem-solving in coding. Here are some ways to improve it:
3.1 Study Classic Algorithms
Familiarize yourself with fundamental algorithms and data structures. This knowledge forms the building blocks for solving more complex problems. Some essential algorithms to study include:
- Sorting algorithms (e.g., Bubble Sort, Merge Sort, Quick Sort)
- Searching algorithms (e.g., Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms
3.2 Practice Regularly
Consistent practice is key to improving your problem-solving skills. Set aside time each day to solve coding challenges. Platforms like AlgoCademy, LeetCode, HackerRank, and CodeSignal offer a wide range of problems to practice on.
3.3 Analyze Time and Space Complexity
Understanding the efficiency of your solutions is crucial. Learn to analyze the time and space complexity of your algorithms using Big O notation. This skill will help you optimize your code and choose the most appropriate solutions for different scenarios.
4. Leveraging Problem-Solving Techniques
Several problem-solving techniques can be applied to coding challenges. Here are some powerful methods to add to your toolkit:
4.1 Divide and Conquer
This technique involves breaking down a complex problem into smaller, more manageable sub-problems. Solve each sub-problem independently and then combine the solutions to solve the original problem.
4.2 Dynamic Programming
Dynamic programming is used to solve problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems and can significantly improve the efficiency of your solutions.
4.3 Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While not always guaranteed to find the best solution, they can be very efficient for certain types of problems.
4.4 Backtracking
Backtracking is a general algorithm for finding all (or some) solutions to some computational problems that incrementally builds candidates to the solutions and abandons a candidate as soon as it determines that the candidate cannot possibly be completed to a valid solution.
5. Improving Your Coding Skills
While problem-solving is crucial, it goes hand in hand with strong coding skills. Here are some ways to enhance your coding abilities:
5.1 Master Your Programming Language
Develop a deep understanding of your chosen programming language. Know its syntax, built-in functions, and standard libraries inside out. This knowledge will allow you to implement your solutions more efficiently.
5.2 Write Clean and Readable Code
Practice writing clean, well-organized code. Use meaningful variable names, add comments where necessary, and follow coding best practices. Clean code is easier to debug and maintain, which is crucial for solving complex problems.
5.3 Learn to Use Debugging Tools
Familiarize yourself with debugging tools available for your programming language and IDE. Effective debugging skills can save you countless hours when solving difficult problems.
6. Cultivating a Problem-Solving Mindset
Developing the right mindset is just as important as learning techniques. Here’s how you can cultivate a problem-solving mindset:
6.1 Embrace Challenges
View difficult problems as opportunities to learn and grow rather than insurmountable obstacles. Embrace the challenge and enjoy the process of finding a solution.
6.2 Be Persistent
Don’t give up when faced with a tough problem. Take breaks if needed, but come back to the problem with renewed energy and perspective.
6.3 Learn from Failures
When your solution doesn’t work, don’t get discouraged. Analyze what went wrong and use it as a learning opportunity. Every failure brings you one step closer to success.
6.4 Stay Curious
Cultivate a curiosity about how things work. When you encounter a new concept or algorithm, dig deeper to understand its underlying principles.
7. Utilizing Resources and Tools
Take advantage of the wealth of resources available to improve your problem-solving skills:
7.1 Online Platforms and Courses
Utilize platforms like AlgoCademy that offer structured learning paths, interactive coding tutorials, and AI-powered assistance. These resources can guide you from basic concepts to advanced problem-solving techniques.
7.2 Coding Communities
Join coding communities and forums where you can discuss problems, share solutions, and learn from others. Platforms like Stack Overflow, Reddit’s programming communities, and GitHub can be invaluable resources.
7.3 Books and Publications
Read books on algorithms, data structures, and problem-solving techniques. Some recommended titles include:
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “The Algorithm Design Manual” by Steven S. Skiena
8. Practicing with Real-World Scenarios
While solving algorithmic puzzles is important, it’s equally crucial to apply your skills to real-world scenarios:
8.1 Work on Personal Projects
Develop your own projects or contribute to open-source projects. This will expose you to real-world problems and help you apply your problem-solving skills in practical contexts.
8.2 Participate in Hackathons
Hackathons provide an excellent opportunity to solve problems under time constraints and collaborate with others. They can help you improve your ability to think on your feet and come up with creative solutions quickly.
8.3 Simulate Technical Interviews
Practice solving problems in a mock interview setting. This can help you get comfortable with explaining your thought process and coding under pressure, which is crucial for technical interviews at top tech companies.
9. Continuous Learning and Improvement
The field of technology is constantly evolving, and so should your problem-solving skills. Here’s how to ensure continuous improvement:
9.1 Stay Updated
Keep up with the latest developments in algorithms, data structures, and programming languages. Follow tech blogs, attend webinars, and participate in coding competitions to stay current.
9.2 Reflect on Your Progress
Regularly assess your problem-solving skills. Keep a log of the problems you’ve solved and analyze your approach. Identify areas where you’ve improved and areas that need more work.
9.3 Teach Others
One of the best ways to solidify your understanding is to teach others. Consider mentoring beginners or writing blog posts explaining complex concepts. This will deepen your own understanding and help you articulate your problem-solving process more clearly.
10. Applying Problem-Solving Skills Beyond Coding
Remember that the problem-solving skills you develop in coding can be applied to other areas of your life and career:
10.1 Analytical Thinking
The analytical thinking you develop through coding can help you approach complex problems in any field more systematically.
10.2 Creative Solutions
The creativity required to solve coding problems can inspire innovative solutions in other areas of your work and personal life.
10.3 Persistence and Resilience
The persistence you cultivate in tackling difficult coding challenges can help you overcome obstacles in various aspects of your life.
Conclusion
Improving your problem-solving skills in coding is a journey that requires dedication, practice, and a willingness to learn. By following the strategies outlined in this guide, you can enhance your ability to tackle complex coding challenges, advance your career, and become a more effective programmer.
Remember, the key to success is consistent practice and a growth mindset. Embrace each problem as an opportunity to learn and grow. With time and effort, you’ll find yourself solving problems that once seemed insurmountable, opening doors to exciting opportunities in the world of technology.
Keep coding, keep learning, and never stop challenging yourself. Your journey to becoming a master problem-solver in coding starts now!