Mastering Problem-Solving Skills for Coding Interviews: A Comprehensive Guide
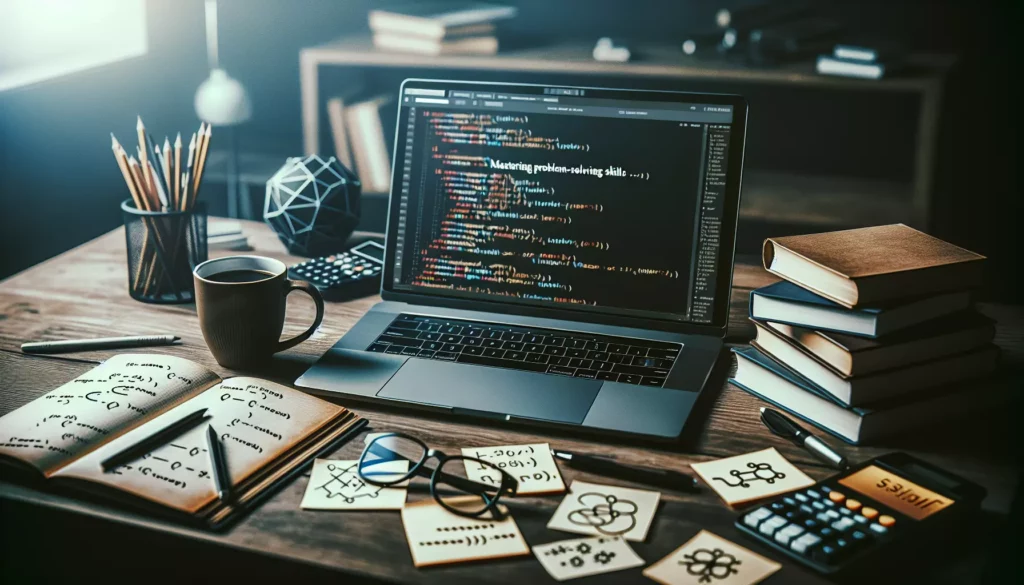
In the competitive world of tech, coding interviews are often the gateway to landing your dream job. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, your problem-solving skills will be put to the test. This comprehensive guide will walk you through effective strategies to enhance your problem-solving abilities and ace those coding interviews.
1. Understanding the Importance of Problem-Solving Skills
Before diving into improvement strategies, it’s crucial to understand why problem-solving skills are so vital in coding interviews. These skills demonstrate your ability to:
- Think critically and analytically
- Break down complex problems into manageable parts
- Develop efficient and optimized solutions
- Adapt to new challenges and unfamiliar scenarios
- Communicate your thought process effectively
Interviewers are not just looking for candidates who can code; they want problem solvers who can contribute to their teams and tackle real-world challenges.
2. Develop a Structured Approach to Problem-Solving
Having a systematic approach to problem-solving can significantly improve your performance in coding interviews. Here’s a step-by-step method you can follow:
- Understand the problem: Carefully read the question and clarify any ambiguities with the interviewer.
- Identify the inputs and outputs: Determine what data you’re working with and what result is expected.
- Break down the problem: Divide the main problem into smaller, manageable sub-problems.
- Plan your approach: Outline the steps you’ll take to solve the problem before writing any code.
- Implement the solution: Write clean, readable code to implement your planned approach.
- Test and debug: Run through test cases and fix any issues in your code.
- Optimize: Look for ways to improve the efficiency of your solution.
Practicing this structured approach will help you tackle problems more effectively and confidently during interviews.
3. Master Essential Data Structures and Algorithms
A solid foundation in data structures and algorithms is crucial for solving coding problems efficiently. Focus on understanding and implementing the following:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (e.g., Quicksort, Mergesort)
- Searching (e.g., Binary Search)
- Graph traversal (BFS, DFS)
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
Understanding these fundamentals will equip you with the tools to tackle a wide range of coding problems.
4. Practice, Practice, Practice
There’s no substitute for consistent practice when it comes to improving your problem-solving skills. Here are some effective ways to practice:
4.1 Solve Coding Problems Regularly
Set aside time each day to solve coding problems. Platforms like LeetCode, HackerRank, and CodeSignal offer a vast array of problems to practice with. Start with easier problems and gradually increase the difficulty as you improve.
4.2 Participate in Coding Competitions
Join coding contests on platforms like Codeforces or TopCoder. These competitions simulate the pressure of real interviews and expose you to a variety of problem types.
4.3 Implement Data Structures and Algorithms from Scratch
Build common data structures and implement algorithms in your preferred programming language. This deepens your understanding and helps you recognize when to apply them in problem-solving scenarios.
4.4 Use Interactive Coding Platforms
Leverage platforms like AlgoCademy that offer interactive coding tutorials and AI-powered assistance. These tools can provide step-by-step guidance and immediate feedback, helping you learn and improve more efficiently.
5. Analyze and Learn from Your Mistakes
Making mistakes is an integral part of the learning process. After solving a problem or attempting a coding challenge:
- Review your solution and compare it with other efficient solutions
- Understand why certain approaches are more optimal
- Identify patterns in problems that gave you difficulty
- Keep a log of problems you struggled with and revisit them periodically
This reflective practice will help you recognize areas for improvement and reinforce your learning.
6. Improve Your Time Management Skills
Coding interviews often come with time constraints, so it’s essential to work on your time management skills. Here are some strategies:
- Practice solving problems under timed conditions
- Learn to quickly identify the core of the problem and focus on it
- Develop the ability to provide a basic solution quickly, then optimize if time allows
- Know when to ask for hints or clarification to avoid wasting time
Remember, it’s often better to have a working solution that may not be fully optimized than to run out of time with an incomplete solution.
7. Enhance Your Coding Skills
While problem-solving is crucial, your coding skills also play a significant role in interviews. Focus on:
7.1 Writing Clean and Readable Code
Practice writing code that is well-organized, properly indented, and easy to understand. Use meaningful variable names and add comments where necessary.
7.2 Mastering Your Chosen Programming Language
Deepen your knowledge of the programming language you’ll use in interviews. Understand its nuances, built-in functions, and best practices.
7.3 Learning to Debug Efficiently
Develop strong debugging skills to quickly identify and fix issues in your code during interviews.
8. Improve Your Communication Skills
Problem-solving in coding interviews isn’t just about writing code; it’s also about effectively communicating your thought process. Practice:
- Explaining your approach before you start coding
- Thinking out loud as you solve the problem
- Asking clarifying questions when needed
- Discussing trade-offs between different solutions
Strong communication skills can set you apart from other candidates and demonstrate your ability to work collaboratively in a team environment.
9. Learn and Apply Problem-Solving Techniques
Familiarize yourself with common problem-solving techniques that can be applied to a wide range of coding challenges:
9.1 Two-Pointer Technique
Useful for problems involving arrays or linked lists, where you need to find a pair of elements or a subarray that meets certain conditions.
9.2 Sliding Window
Efficient for problems requiring the computation of a function on a sliding window of an array or string.
9.3 Divide and Conquer
Breaking down a problem into smaller subproblems, solving them, and then combining the results.
9.4 Dynamic Programming
Solving complex problems by breaking them down into simpler subproblems and storing the results for future use.
9.5 Backtracking
A technique for solving problems recursively by trying to build a solution incrementally, abandoning solutions that fail to meet the problem’s constraints.
Understanding these techniques will give you a toolkit to approach a variety of problem types effectively.
10. Simulate Real Interview Conditions
To truly prepare for coding interviews, it’s important to simulate real interview conditions:
- Practice coding on a whiteboard or using a simple text editor without auto-completion
- Set up mock interviews with friends or use platforms that offer mock interview services
- Time yourself to get comfortable working under pressure
- Practice explaining your thought process out loud as you solve problems
The more you can replicate actual interview conditions, the more comfortable and prepared you’ll be when facing real interviews.
11. Stay Updated with Industry Trends
The tech industry is constantly evolving, and staying current can give you an edge in interviews:
- Follow tech blogs and news sites to stay informed about new technologies and industry trends
- Participate in coding forums and communities to learn from others and share your knowledge
- Attend tech meetups or conferences to network and gain insights into current industry practices
This knowledge can help you understand the context of certain interview questions and demonstrate your passion for technology to interviewers.
12. Develop a Growth Mindset
Lastly, cultivate a growth mindset that embraces challenges and sees failures as opportunities to learn:
- View difficult problems as chances to improve rather than insurmountable obstacles
- Embrace feedback and learn from criticism
- Persist in the face of setbacks and use them as motivation to work harder
- Celebrate small victories and progress in your problem-solving journey
A growth mindset will not only help you in interviews but will also serve you well throughout your career in tech.
Conclusion
Improving your problem-solving skills for coding interviews is a journey that requires dedication, consistent practice, and a strategic approach. By following the strategies outlined in this guide, you’ll be well-equipped to tackle even the most challenging coding problems with confidence.
Remember, the goal is not just to pass interviews but to become a better problem solver and programmer overall. Each problem you solve and each concept you master brings you one step closer to your goals in the tech industry.
As you continue your preparation, consider leveraging resources like AlgoCademy, which offers tailored learning paths, interactive coding exercises, and AI-powered assistance to help you progress from beginner-level coding to mastering technical interviews. With persistence and the right approach, you’ll be well on your way to acing those coding interviews and landing your dream job in tech.
Happy coding, and best of luck in your interviews!