Mastering Parentheses Problems: Essential Techniques for Coding Interviews
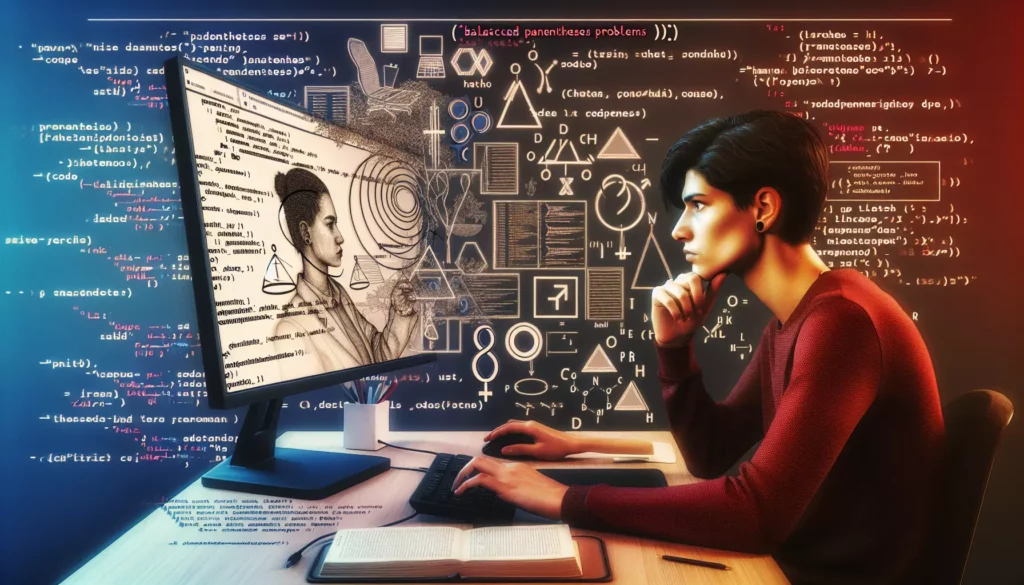
Welcome to AlgoCademy’s comprehensive guide on solving parentheses problems! If you’re preparing for coding interviews, especially with top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering these techniques is crucial. Parentheses problems are a common type of coding challenge that test your ability to work with data structures, particularly stacks, and your understanding of balanced expressions.
In this article, we’ll dive deep into various techniques for tackling parentheses problems, provide step-by-step explanations, and offer practical coding examples to help you sharpen your skills. Whether you’re a beginner looking to improve your algorithmic thinking or an experienced developer preparing for technical interviews, this guide will equip you with the knowledge and strategies you need to excel.
Table of Contents
- Understanding Parentheses Problems
- The Stack Approach: A Fundamental Technique
- The Counting Method: A Simple Alternative
- Advanced Techniques for Complex Parentheses Problems
- Common Variations of Parentheses Problems
- Time and Space Complexity Analysis
- Practice Problems and Solutions
- Interview Tips for Parentheses Problems
- Conclusion and Next Steps
1. Understanding Parentheses Problems
Parentheses problems typically involve determining whether a given string of brackets (parentheses, square brackets, curly braces) is balanced or not. A balanced string of brackets means that every opening bracket has a corresponding closing bracket in the correct order.
For example:
- “()” is balanced
- “()[]{}” is balanced
- “(]” is not balanced
- “([)]” is not balanced
- “{[]}” is balanced
These problems test your ability to keep track of opening and closing brackets, match them correctly, and handle nested structures. They are excellent for assessing your problem-solving skills and your proficiency with fundamental data structures like stacks.
2. The Stack Approach: A Fundamental Technique
The stack is the most common and efficient data structure used to solve parentheses problems. Its Last-In-First-Out (LIFO) nature makes it perfect for matching opening and closing brackets.
How the Stack Approach Works:
- Iterate through each character in the input string.
- If the character is an opening bracket, push it onto the stack.
- If the character is a closing bracket:
- If the stack is empty, return false (unmatched closing bracket).
- If the top of the stack doesn’t match the current closing bracket, return false (mismatched brackets).
- If it matches, pop the top element from the stack.
- After processing all characters, check if the stack is empty. If it is, the string is balanced; if not, it’s unbalanced.
Example Implementation in Python:
def is_balanced(s):
stack = []
opening = set('([{')
closing = set(')]}')
pair = {')': '(', ']': '[', '}': '{'}
for char in s:
if char in opening:
stack.append(char)
elif char in closing:
if not stack or stack[-1] != pair[char]:
return False
stack.pop()
return len(stack) == 0
This implementation uses a dictionary to match closing brackets with their corresponding opening brackets, making it easy to check for correct pairing.
Advantages of the Stack Approach:
- Efficient: O(n) time complexity, where n is the length of the input string.
- Versatile: Can handle multiple types of brackets and nested structures.
- Intuitive: Mimics the natural way we process brackets when reading.
3. The Counting Method: A Simple Alternative
For simpler parentheses problems involving only one type of bracket (usually round parentheses), the counting method can be an elegant solution.
How the Counting Method Works:
- Initialize a counter to 0.
- Iterate through the string:
- Increment the counter for each opening parenthesis.
- Decrement the counter for each closing parenthesis.
- If the counter ever becomes negative, return false (more closing than opening).
- After processing all characters, check if the counter is 0. If it is, the string is balanced; if not, it’s unbalanced.
Example Implementation in Python:
def is_balanced_parentheses(s):
count = 0
for char in s:
if char == '(':
count += 1
elif char == ')':
count -= 1
if count < 0:
return False
return count == 0
Advantages of the Counting Method:
- Simple and easy to implement
- Requires less memory than the stack approach (constant space complexity)
- Can be extended to handle multiple types of brackets with separate counters
4. Advanced Techniques for Complex Parentheses Problems
While the stack and counting methods cover most basic parentheses problems, you may encounter more complex variations in coding interviews. Here are some advanced techniques to tackle these challenges:
4.1 Using a Stack with Additional Information
For problems that require keeping track of additional information along with the brackets, you can modify the stack to store tuples or custom objects.
Example: Finding the maximum nesting depth of parentheses
def max_nesting_depth(s):
stack = []
max_depth = 0
current_depth = 0
for char in s:
if char == '(':
current_depth += 1
stack.append(current_depth)
max_depth = max(max_depth, current_depth)
elif char == ')':
if stack:
stack.pop()
current_depth -= 1
else:
return -1 # Unbalanced string
return max_depth if not stack else -1 # Check if all brackets are closed
4.2 Dynamic Programming for Optimization Problems
Some parentheses problems involve finding the longest valid substring or the minimum number of removals to make a string valid. These often benefit from dynamic programming approaches.
Example: Longest Valid Parentheses Substring
def longest_valid_parentheses(s):
n = len(s)
dp = [0] * n
stack = []
for i in range(n):
if s[i] == '(':
stack.append(i)
else:
if stack:
last = stack.pop()
dp[i] = dp[last - 1] + i - last + 1 if last > 0 else i - last + 1
return max(dp) if dp else 0
4.3 Two-Pass Algorithms
Some problems can be solved efficiently by scanning the string twice, once from left to right and once from right to left.
Example: Check if a string can be made valid by removing at most one character
def can_be_valid(s):
def check(s, open_char, close_char):
count = 0
for char in s:
if char == open_char:
count += 1
elif char == close_char:
count -= 1
if count < 0:
return False
return True
return check(s, '(', ')') and check(s[::-1], ')', '(')
5. Common Variations of Parentheses Problems
In coding interviews, you might encounter various twists on the basic parentheses problem. Here are some common variations and approaches to solve them:
5.1 Generate All Valid Parentheses Strings
This problem asks you to generate all possible valid combinations of n pairs of parentheses.
def generate_parentheses(n):
def backtrack(s, left, right):
if len(s) == 2 * n:
result.append(s)
return
if left < n:
backtrack(s + '(', left + 1, right)
if right < left:
backtrack(s + ')', left, right + 1)
result = []
backtrack('', 0, 0)
return result
5.2 Minimum Additions to Make Valid Parentheses
This problem asks for the minimum number of parentheses to add to make a string valid.
def min_additions_for_valid(s):
left = right = additions = 0
for char in s:
if char == '(':
left += 1
elif char == ')':
if left == 0:
additions += 1
else:
left -= 1
return additions + left
5.3 Score of Parentheses
This problem assigns a score to a balanced parentheses string based on certain rules.
def score_of_parentheses(s):
stack = [0]
for char in s:
if char == '(':
stack.append(0)
else:
v = stack.pop()
stack[-1] += max(2 * v, 1)
return stack.pop()
6. Time and Space Complexity Analysis
Understanding the time and space complexity of your solutions is crucial for coding interviews. Here’s a breakdown for common approaches:
Stack Approach:
- Time Complexity: O(n), where n is the length of the input string.
- Space Complexity: O(n) in the worst case, when all characters are opening brackets.
Counting Method:
- Time Complexity: O(n)
- Space Complexity: O(1), as it uses only a single counter variable.
Dynamic Programming (e.g., Longest Valid Parentheses):
- Time Complexity: O(n)
- Space Complexity: O(n) for the DP array
Generating All Valid Parentheses:
- Time Complexity: O(4^n / √n), as the nth Catalan number is bounded by 4^n / (n√n)
- Space Complexity: O(4^n / √n) to store all valid combinations
When solving parentheses problems, always consider the trade-offs between time and space complexity. In some cases, you might be able to optimize one at the expense of the other, depending on the specific requirements of the problem.
7. Practice Problems and Solutions
To reinforce your understanding and prepare for coding interviews, here are some practice problems along with their solutions:
Problem 1: Valid Parentheses
Given a string s containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid.
def is_valid(s):
stack = []
mapping = {")": "(", "}": "{", "]": "["}
for char in s:
if char in mapping:
if not stack or stack[-1] != mapping[char]:
return False
stack.pop()
else:
stack.append(char)
return not stack
Problem 2: Remove Invalid Parentheses
Remove the minimum number of invalid parentheses to make the input string valid. Return all possible results.
def remove_invalid_parentheses(s):
def is_valid(s):
count = 0
for char in s:
if char == '(':
count += 1
elif char == ')':
count -= 1
if count < 0:
return False
return count == 0
level = {s}
while True:
valid = list(filter(is_valid, level))
if valid:
return valid
next_level = set()
for s in level:
for i in range(len(s)):
if s[i] in '()':
next_level.add(s[:i] + s[i+1:])
level = next_level
Problem 3: Minimum Remove to Make Valid Parentheses
Given a string s of ‘(‘ , ‘)’ and lowercase English characters, remove the minimum number of parentheses to make the input string valid.
def min_remove_to_make_valid(s):
stack = []
s = list(s)
for i, char in enumerate(s):
if char == '(':
stack.append(i)
elif char == ')':
if stack:
stack.pop()
else:
s[i] = ''
while stack:
s[stack.pop()] = ''
return ''.join(s)
Practice these problems and variations to improve your skills in handling parentheses-related challenges. Remember to analyze the time and space complexity of your solutions and consider edge cases when testing your code.
8. Interview Tips for Parentheses Problems
When tackling parentheses problems in a coding interview, keep these tips in mind:
- Clarify the problem: Ask questions to ensure you understand the requirements. For example, clarify if the input can contain characters other than parentheses.
- Consider edge cases: Think about empty strings, strings with only opening or closing brackets, and very long inputs.
- Start with a simple approach: Begin with the stack method or counting method, then optimize if needed.
- Explain your thought process: As you code, explain your approach and any trade-offs you’re considering.
- Test your solution: After implementing, run through a few test cases to catch any bugs.
- Analyze complexity: Be prepared to discuss the time and space complexity of your solution.
- Consider optimizations: If your initial solution works, think about ways to improve it, such as reducing space complexity.
- Handle invalid inputs: Discuss how your solution handles invalid inputs or unexpected characters.
Remember, interviewers are often more interested in your problem-solving approach and communication skills than in a perfect solution. Demonstrate your ability to think through the problem systematically and explain your reasoning clearly.
9. Conclusion and Next Steps
Mastering parentheses problems is an essential skill for coding interviews, particularly when aiming for positions at top tech companies. These problems test your understanding of fundamental data structures like stacks, your ability to handle edge cases, and your skill in optimizing algorithms.
To continue improving your skills:
- Practice regularly with a variety of parentheses problems
- Implement solutions in different programming languages to broaden your expertise
- Study related topics like dynamic programming and backtracking
- Participate in coding competitions or online judge platforms to test your skills
- Review and understand common variations of parentheses problems
Remember, solving parentheses problems efficiently is just one aspect of coding interview preparation. Continue to broaden your knowledge in other areas of algorithms and data structures, and practice explaining your thought process clearly.
At AlgoCademy, we offer a range of resources to help you prepare for coding interviews, including interactive tutorials, AI-powered assistance, and step-by-step guidance. Keep practicing, stay curious, and you’ll be well-prepared to tackle any parentheses problem that comes your way in your next coding interview!