Mastering Pair Programming Interviews: Essential Tips for Success
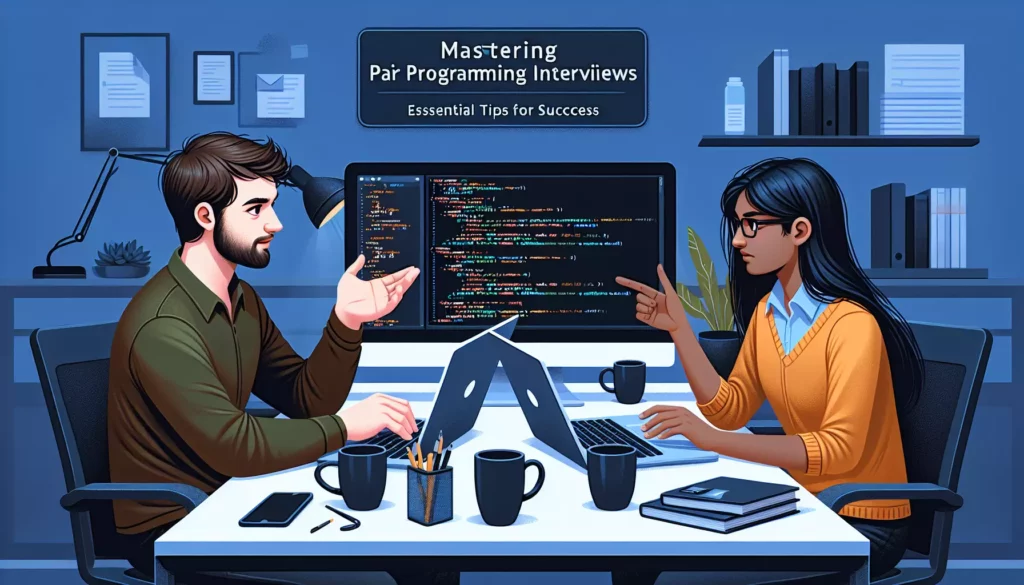
In the competitive world of tech interviews, pair programming sessions have become increasingly common. These collaborative coding exercises offer interviewers a unique glimpse into a candidate’s problem-solving skills, communication abilities, and teamwork capabilities. For many aspiring developers, especially those aiming for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering the art of pair programming interviews is crucial. In this comprehensive guide, we’ll explore essential tips to help you excel in pair programming interviews and boost your chances of landing that dream job.
Understanding Pair Programming Interviews
Before diving into the tips, let’s first understand what pair programming interviews entail. In a pair programming interview, you’ll typically be paired with an interviewer or another candidate to work on a coding problem together. The goal is to simulate a real-world collaborative coding environment, where you’ll need to communicate effectively, share ideas, and work towards a solution as a team.
These interviews assess not just your technical skills, but also your ability to:
- Communicate complex ideas clearly
- Collaborate effectively with others
- Handle feedback and suggestions gracefully
- Adapt to different coding styles and approaches
- Demonstrate leadership and initiative when appropriate
Now that we have a clear understanding of what pair programming interviews involve, let’s explore some key tips to help you succeed.
1. Brush Up on Your Fundamentals
While pair programming interviews focus on collaboration, having a solid foundation in coding fundamentals is still crucial. Make sure you’re comfortable with:
- Basic data structures (arrays, linked lists, trees, graphs)
- Common algorithms (sorting, searching, traversal)
- Time and space complexity analysis
- Object-oriented programming concepts
- Design patterns and best practices
Platforms like AlgoCademy offer interactive coding tutorials and resources that can help you reinforce these fundamentals and prepare for technical interviews.
2. Practice Active Listening
One of the most critical skills in pair programming is active listening. Pay close attention to your partner’s ideas and suggestions. Show that you’re engaged by:
- Asking clarifying questions
- Paraphrasing their ideas to ensure understanding
- Acknowledging their contributions
- Building upon their suggestions
Remember, effective collaboration is a two-way street. By demonstrating strong listening skills, you show that you’re a team player who values others’ input.
3. Communicate Clearly and Constantly
Clear communication is the backbone of successful pair programming. Throughout the interview:
- Articulate your thoughts and ideas clearly
- Explain your approach and reasoning
- Use proper technical terminology
- Be open about any uncertainties or areas where you need clarification
Don’t be afraid to think out loud. Verbalizing your thought process helps your partner understand your reasoning and allows them to provide input or catch potential issues early on.
4. Plan Before You Code
Resist the urge to dive straight into coding. Take some time to discuss and plan your approach with your partner. This might include:
- Clarifying the problem requirements
- Discussing potential solutions and their trade-offs
- Agreeing on a high-level approach
- Breaking down the problem into smaller, manageable tasks
This planning phase not only leads to more organized and efficient coding but also demonstrates your ability to think strategically and collaborate on problem-solving.
5. Be Flexible and Open to Feedback
In pair programming, flexibility is key. Be open to your partner’s ideas and willing to adjust your approach if needed. If your partner suggests an alternative solution or points out a potential issue in your code, respond positively:
- Thank them for their input
- Consider their suggestion carefully
- Discuss the pros and cons of different approaches
- Be willing to change direction if their idea seems better
This flexibility shows that you’re not just focused on your own ideas, but are committed to finding the best solution as a team.
6. Practice Good Coding Etiquette
When it’s your turn to code, follow good coding practices to make your code easy for your partner to understand and work with:
- Use meaningful variable and function names
- Write clean, well-formatted code
- Add comments to explain complex logic
- Break down long functions into smaller, more manageable pieces
Here’s an example of good coding etiquette in Python:
def calculate_average(numbers):
"""
Calculate the average of a list of numbers.
Args:
numbers (list): A list of numbers
Returns:
float: The average of the numbers
"""
if not numbers:
return 0
total = sum(numbers)
count = len(numbers)
return total / count
# Example usage
scores = [85, 90, 78, 92, 88]
average_score = calculate_average(scores)
print(f"The average score is: {average_score:.2f}")
This code demonstrates clear naming, proper comments, and a logical structure that’s easy for your partner to follow and build upon.
7. Handle Disagreements Professionally
In a pair programming session, disagreements are natural and can even be beneficial when handled correctly. If you and your partner have differing opinions:
- Stay calm and professional
- Listen to your partner’s perspective fully
- Explain your reasoning clearly and respectfully
- Focus on the problem, not personal preferences
- Be willing to compromise or try both approaches if time allows
Remember, the goal is to find the best solution together, not to prove who’s right.
8. Manage Time Effectively
Time management is crucial in pair programming interviews. To make the most of your limited time:
- Agree on time allocations for different parts of the problem
- Set checkpoints to review progress
- Be mindful of the time remaining
- Prioritize core functionality over optional features
- Know when to move on if you’re stuck on a particular issue
Effective time management shows that you can work efficiently under pressure and deliver results within constraints.
9. Practice Pair Programming Regularly
Like any skill, pair programming improves with practice. To prepare for your interview:
- Find a coding buddy to practice with regularly
- Participate in online coding challenges that support pair programming
- Join local coding meetups or hackathons that involve collaborative coding
- Use platforms like AlgoCademy that offer pair programming simulations
The more you practice, the more comfortable and proficient you’ll become in collaborative coding environments.
10. Focus on Problem-Solving, Not Just Coding
While coding skills are important, pair programming interviews also assess your problem-solving abilities. Throughout the session:
- Break down complex problems into smaller, manageable parts
- Consider multiple approaches before settling on a solution
- Discuss trade-offs between different solutions
- Think about edge cases and potential issues
- Consider scalability and performance implications
Demonstrating strong problem-solving skills shows that you can tackle complex challenges effectively in a team setting.
11. Be Prepared to Switch Roles
In pair programming, you may need to switch between the roles of “driver” (the person actively coding) and “navigator” (the person reviewing and providing input). Be prepared for both roles:
- As the driver, be open to suggestions and explain your code as you write it
- As the navigator, offer constructive feedback and catch potential issues
- Practice both roles during your preparation to be comfortable with each
Flexibility in switching roles demonstrates your adaptability and teamwork skills.
12. Understand the Importance of Testing
Testing is a crucial part of the development process, and pair programming interviews often include discussions about testing strategies. Be prepared to:
- Discuss unit testing approaches
- Write test cases for your code
- Consider edge cases and boundary conditions
- Explain how you would approach integration testing
Here’s an example of how you might incorporate testing into your pair programming session:
def is_palindrome(s):
"""
Check if a string is a palindrome.
Args:
s (str): The string to check
Returns:
bool: True if the string is a palindrome, False otherwise
"""
# Remove non-alphanumeric characters and convert to lowercase
cleaned = ''.join(c.lower() for c in s if c.isalnum())
return cleaned == cleaned[::-1]
# Test cases
def test_is_palindrome():
assert is_palindrome("A man, a plan, a canal: Panama") == True
assert is_palindrome("race a car") == False
assert is_palindrome("") == True
assert is_palindrome("Madam, I'm Adam") == True
print("All test cases passed!")
test_is_palindrome()
This example demonstrates how you can incorporate simple test cases into your code during a pair programming session, showcasing your understanding of the importance of testing.
13. Stay Calm Under Pressure
Pair programming interviews can be stressful, but it’s important to stay calm and composed. Remember:
- Take deep breaths if you feel overwhelmed
- It’s okay to take a moment to gather your thoughts
- If you’re stuck, communicate this to your partner and brainstorm together
- Don’t be afraid to ask for clarification or hints if needed
Maintaining your composure under pressure is a valuable skill that interviewers look for in potential team members.
14. Reflect on Your Performance
After each pair programming practice session or interview:
- Reflect on what went well and what could be improved
- Ask for feedback from your partner or interviewer if possible
- Identify areas where you can enhance your skills or knowledge
- Set specific goals for improvement in future sessions
Continuous reflection and improvement will help you become more proficient and confident in pair programming situations.
15. Familiarize Yourself with Collaborative Tools
Many pair programming interviews, especially for remote positions, use online collaborative coding environments. To prepare:
- Practice using common coding collaboration tools (e.g., CodePen, JSFiddle, CoderPad)
- Familiarize yourself with screen sharing and video conferencing platforms
- Learn keyboard shortcuts for efficient navigation and editing
- Be prepared to adapt to different IDE settings or configurations
Being comfortable with these tools will allow you to focus on the problem-solving aspects of the interview rather than struggling with the technology.
Conclusion
Pair programming interviews offer a unique opportunity to showcase not just your coding skills, but also your ability to collaborate, communicate, and problem-solve effectively in a team setting. By following these tips and practicing regularly, you can significantly improve your performance in pair programming interviews and increase your chances of success in landing positions at top tech companies.
Remember, the key to excelling in pair programming interviews lies in a combination of strong technical skills, effective communication, adaptability, and a positive, collaborative attitude. With practice and preparation, you can transform the potentially stressful experience of a pair programming interview into an opportunity to demonstrate your strengths as a developer and team player.
As you continue to hone your skills, consider leveraging resources like AlgoCademy, which offers interactive coding tutorials, AI-powered assistance, and tools specifically designed to help you progress from beginner-level coding to mastering technical interviews. With dedication and the right resources, you’ll be well-equipped to tackle any pair programming challenge that comes your way in your journey towards a successful career in tech.