Mastering Pair Programming Interviews: A Comprehensive Guide
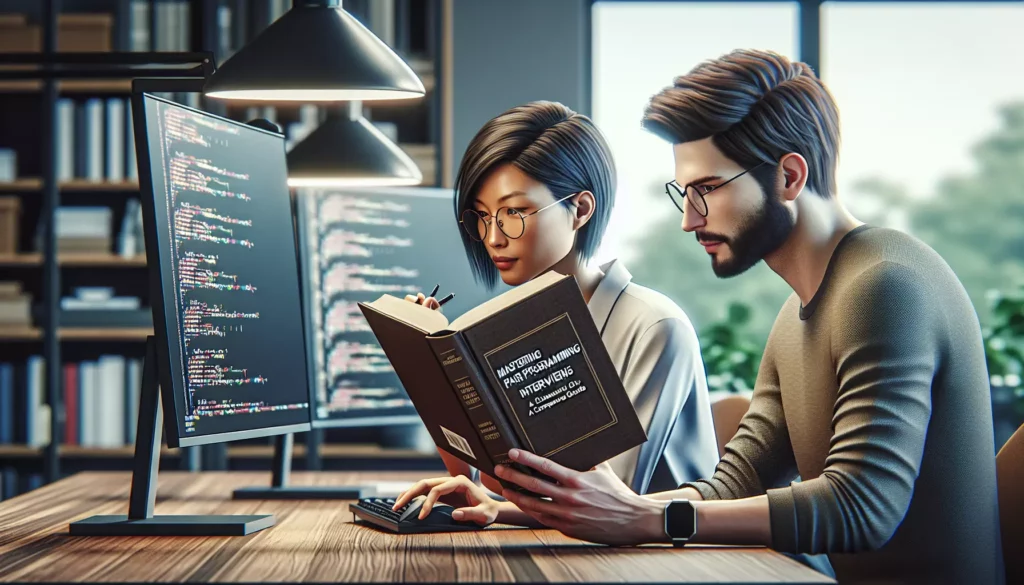
In the ever-evolving landscape of software development, the interview process has become increasingly sophisticated. One of the most challenging and insightful methods employed by top tech companies is the pair programming interview. This approach not only tests a candidate’s coding skills but also evaluates their ability to collaborate, communicate, and problem-solve in real-time. In this comprehensive guide, we’ll dive deep into the world of pair programming interviews, exploring their nuances, best practices, and how you can prepare to excel in this unique interview format.
What is a Pair Programming Interview?
A pair programming interview is a technical assessment where the candidate works collaboratively with an interviewer to solve a coding problem. This format mimics the real-world scenario of pair programming, a practice where two developers work together on the same codebase, often sharing a single workstation.
Key characteristics of a pair programming interview include:
- Real-time collaboration between the candidate and interviewer
- Shared coding environment, typically an online platform
- Focus on problem-solving and communication skills
- Interactive dialogue throughout the coding process
- Opportunity for the interviewer to provide hints or guidance
Why Companies Use Pair Programming Interviews
Pair programming interviews have gained popularity among tech companies, especially those embracing Agile methodologies, for several reasons:
- Assessing Collaboration Skills: They provide insight into how well a candidate can work with others, a crucial skill in modern development teams.
- Evaluating Communication: The format tests a candidate’s ability to articulate their thoughts and explain technical concepts clearly.
- Simulating Real Work Environments: It closely resembles actual work scenarios, giving both the candidate and the company a realistic preview of potential future interactions.
- Gauging Problem-Solving Approaches: Interviewers can observe how candidates tackle problems, adapt to suggestions, and handle roadblocks.
- Assessing Code Quality and Best Practices: Beyond just solving the problem, interviewers can evaluate a candidate’s coding style, adherence to best practices, and attention to detail.
Skills Tested in Pair Programming Interviews
Pair programming interviews are designed to evaluate a comprehensive set of skills that are essential for successful software development:
1. Technical Proficiency
While this is a given in any coding interview, pair programming interviews assess not just the ability to write code, but to do so efficiently and explain the process simultaneously.
2. Problem-Solving
Candidates are evaluated on their approach to breaking down complex problems, considering edge cases, and developing efficient solutions.
3. Communication
Clear articulation of thoughts, ideas, and technical concepts is crucial. This includes the ability to ask clarifying questions and explain coding decisions.
4. Collaboration
The interview tests how well candidates can work with others, incorporate feedback, and build upon suggestions from their interviewer.
5. Adaptability
As the interviewer may provide hints or suggest alternative approaches, candidates must demonstrate flexibility and openness to new ideas.
6. Code Organization and Best Practices
Writing clean, well-organized, and maintainable code is essential, as is following industry-standard best practices.
7. Debugging and Testing
Candidates may need to identify and fix bugs in their code or write unit tests to ensure code correctness.
The Format of a Pair Programming Interview
While the specific format can vary between companies, a typical pair programming interview often follows this structure:
1. Introduction (5-10 minutes)
The interviewer introduces themselves and explains the format of the interview. They may also ask some brief questions about the candidate’s background and experience.
2. Problem Presentation (5 minutes)
The interviewer presents the coding problem or task. This could range from implementing a specific algorithm to building a small feature or component.
3. Clarification and Planning (5-10 minutes)
The candidate asks questions to clarify the requirements and constraints of the problem. They may also discuss their initial approach with the interviewer.
4. Coding Session (30-45 minutes)
This is the main part of the interview where the candidate writes code to solve the problem. The interviewer observes, asks questions, and may provide hints or guidance as needed.
5. Testing and Refinement (10-15 minutes)
The candidate tests their solution, identifies and fixes any bugs, and optimizes the code if time allows.
6. Discussion and Wrap-up (5-10 minutes)
The interviewer may ask follow-up questions about the solution, discuss potential improvements, or explore how the code might be extended or scaled.
Example Pair Programming Interview Scenario
Let’s walk through a hypothetical pair programming interview to illustrate how the process might unfold:
Problem: Implement a Function to Find the Longest Palindromic Substring
Interviewer: “Today, we’ll be working on implementing a function that finds the longest palindromic substring in a given string. Do you have any initial questions about the problem?”
Candidate: “Yes, a few clarifications. Should we consider single characters as palindromes? Also, are we dealing with only lowercase letters, or should we account for uppercase and special characters?”
Interviewer: “Great questions. Yes, single characters count as palindromes. For simplicity, let’s assume we’re only dealing with lowercase letters. How would you approach this problem?”
Candidate: “I’m thinking we could use the expand around center approach. We’ll iterate through each character, treating it as the center of a potential palindrome, and expand outwards to find the longest palindrome. We’ll do this for both odd and even length palindromes. Does that sound reasonable?”
Interviewer: “That’s a good approach. Let’s start implementing it.”
Candidate: “Alright, I’ll start by defining the function and implementing the helper function to expand around the center.”
def longest_palindrome(s: str) -> str:
if not s:
return ""
def expand_around_center(left: int, right: int) -> int:
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
start, end = 0, 0
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
max_len = max(len1, len2)
if max_len > end - start:
start = i - (max_len - 1) // 2
end = i + max_len // 2
return s[start:end+1]
Interviewer: “That looks good. Can you walk me through your implementation?”
Candidate: “Certainly. The `expand_around_center` function takes two indices and expands outwards as long as the characters match and we’re within the string bounds. It returns the length of the palindrome found.
In the main function, we iterate through each character, considering it as a potential center. We check for both odd-length palindromes (expand from i to i) and even-length palindromes (expand from i to i+1). We keep track of the start and end indices of the longest palindrome found so far.
Finally, we return the substring using these indices.”
Interviewer: “Excellent explanation. Now, let’s test it with a few examples.”
Candidate: “Sure, I’ll add some test cases:”
print(longest_palindrome("babad")) # Expected: "bab" or "aba"
print(longest_palindrome("cbbd")) # Expected: "bb"
print(longest_palindrome("a")) # Expected: "a"
print(longest_palindrome("")) # Expected: ""
Interviewer: “The implementation looks correct, and the test cases cover various scenarios. Can you think of any ways to optimize this solution?”
Candidate: “One potential optimization could be to use dynamic programming to avoid redundant calculations. However, that would increase the space complexity to O(n^2). Another approach could be Manacher’s algorithm, which solves this problem in linear time, but it’s more complex to implement.”
Interviewer: “Good insights. For our purposes, this solution is efficient enough. Let’s discuss the time and space complexity of your implementation.”
Candidate: “The time complexity is O(n^2) in the worst case, where n is the length of the string. This is because for each character, we might expand to cover the entire string. The space complexity is O(1) as we’re only using a constant amount of extra space.”
Interviewer: “Excellent. That concludes our pair programming session. Do you have any questions for me?”
Tips for Excelling in Pair Programming Interviews
To perform well in a pair programming interview, consider the following tips:
1. Communicate Clearly and Constantly
Verbalize your thought process throughout the interview. Explain your approach, decisions, and any assumptions you’re making. This gives the interviewer insight into your problem-solving skills and allows them to provide guidance if needed.
2. Ask Clarifying Questions
Don’t hesitate to ask questions about the problem statement, requirements, or constraints. This shows that you’re thorough and helps ensure you’re solving the right problem.
3. Plan Before Coding
Take a few minutes to outline your approach before diving into coding. This demonstrates your ability to think strategically and can help prevent major course corrections later.
4. Write Clean, Readable Code
Even under time pressure, strive to write clean, well-organized code. Use meaningful variable names, proper indentation, and follow language-specific conventions.
5. Test Your Code
After implementing a solution, test it with various inputs, including edge cases. This shows attention to detail and a commitment to code quality.
6. Be Open to Feedback
If the interviewer suggests an alternative approach or points out an issue, be receptive and adaptable. The ability to incorporate feedback is a valuable skill.
7. Manage Your Time
Keep an eye on the clock and pace yourself accordingly. If you’re spending too much time on one aspect, communicate this to the interviewer and consider moving on.
8. Stay Calm Under Pressure
It’s normal to feel nervous, but try to remain calm and focused. If you get stuck, take a deep breath and consider explaining your thought process to the interviewer, who may offer hints.
How to Prepare for Pair Programming Interviews
Effective preparation can significantly boost your performance in pair programming interviews. Here are some strategies to help you prepare:
1. Practice Coding Aloud
Get comfortable explaining your code and thought process while you’re writing it. This can feel unnatural at first, so practice is key.
2. Solve Problems on Coding Platforms
Websites like LeetCode, HackerRank, and AlgoCademy offer a wide range of coding problems similar to those you might encounter in interviews. Practice solving these problems within time constraints.
3. Participate in Mock Interviews
Find a study buddy or use platforms that offer mock interview services. This will help you get comfortable with the format and receive feedback on your performance.
4. Review Core Computer Science Concepts
Ensure you have a solid grasp of data structures, algorithms, time and space complexity analysis, and problem-solving techniques.
5. Brush Up on Your Chosen Programming Language
Be very familiar with the syntax, standard libraries, and best practices of your preferred programming language.
6. Practice Good Coding Habits
Always write clean, well-documented code. This should become second nature, even under the pressure of an interview.
7. Learn to Use Online Coding Environments
Familiarize yourself with popular online coding platforms that are often used in remote interviews.
8. Work on Your Soft Skills
Improve your communication, active listening, and collaboration skills. These are just as important as your technical abilities in a pair programming interview.
Common Challenges in Pair Programming Interviews
While pair programming interviews can be an effective assessment tool, they come with their own set of challenges. Being aware of these can help you better prepare and navigate the interview process:
1. Performance Anxiety
Coding under observation can be nerve-wracking. Remember that the interviewer is there to collaborate, not just to judge. Take deep breaths and try to view the experience as a pair programming session rather than a test.
2. Time Management
Balancing thorough problem-solving with time constraints can be tricky. Practice timing yourself when solving problems to get a feel for pacing.
3. Overexplaining or Underexplaining
Finding the right balance in communication can be challenging. Aim to provide clear, concise explanations without becoming overly verbose.
4. Adapting to the Interviewer’s Style
Every interviewer has a different style of interaction. Some may be more hands-on, while others might take a more observational approach. Be prepared to adapt to different styles.
5. Handling Hints and Feedback
Interviewers often provide hints or feedback during the session. Learning to incorporate these effectively without losing your train of thought is a valuable skill.
6. Technical Difficulties
For remote interviews, issues with the coding environment or video conferencing tools can arise. Familiarize yourself with common platforms and have a backup plan (like a phone number) in case of technical issues.
The Role of AlgoCademy in Preparing for Pair Programming Interviews
AlgoCademy, as a platform focused on coding education and interview preparation, can be an invaluable resource for those gearing up for pair programming interviews. Here’s how AlgoCademy can help:
1. Interactive Coding Tutorials
AlgoCademy offers step-by-step coding tutorials that can help you build a strong foundation in various programming concepts and problem-solving techniques.
2. Algorithm and Data Structure Practice
The platform provides a wide range of problems focusing on algorithms and data structures, which are often the core of pair programming interview questions.
3. AI-Powered Assistance
AlgoCademy’s AI-powered features can provide hints and explanations, similar to how an interviewer might guide you during a pair programming session.
4. Real-Time Coding Environment
Practicing in AlgoCademy’s coding environment can help you get comfortable with online coding platforms commonly used in remote interviews.
5. Progress Tracking
The platform allows you to track your progress, helping you identify areas where you need more practice and ensuring a well-rounded preparation.
6. Community and Resources
AlgoCademy’s community features and additional resources can provide valuable insights and tips from others who have gone through similar interview processes.
Conclusion
Pair programming interviews represent a unique and insightful approach to assessing a candidate’s coding skills, problem-solving abilities, and teamwork capabilities. While they can be challenging, with the right preparation and mindset, they also offer an opportunity to showcase your skills in a realistic, collaborative environment.
Remember that the key to success in pair programming interviews lies not just in your coding abilities, but in your communication skills, adaptability, and ability to work effectively with others. By focusing on these aspects alongside your technical preparation, you’ll be well-equipped to excel in this interview format.
Platforms like AlgoCademy can play a crucial role in your preparation journey, offering structured learning paths, hands-on practice, and valuable resources. Embrace the challenge, practice regularly, and approach each interview as an opportunity to learn and grow. With dedication and the right resources, you can master the art of pair programming interviews and take a significant step forward in your software development career.