Mastering NVIDIA Technical Interview Prep: A Comprehensive Guide
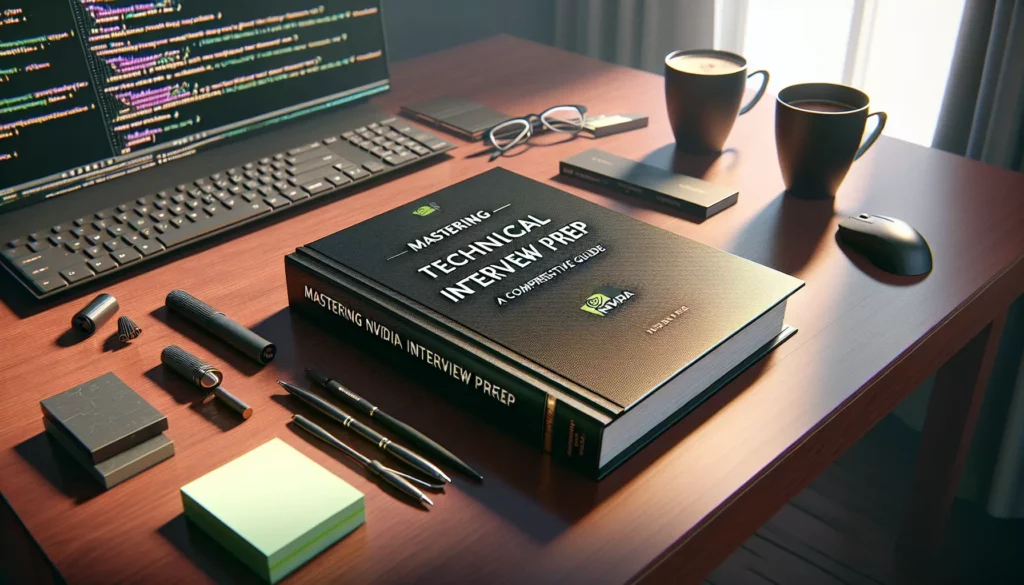
As one of the leading technology companies specializing in graphics processing units (GPUs) and artificial intelligence, NVIDIA presents a unique and challenging interview process for aspiring software engineers and developers. This comprehensive guide will walk you through the essential steps and strategies to prepare for an NVIDIA technical interview, helping you showcase your skills and stand out from the competition.
Table of Contents
- Understanding NVIDIA and Its Technology Focus
- The NVIDIA Interview Process
- Key Technical Skills to Master
- Coding Practice and Problem-Solving Strategies
- System Design and Architecture
- GPU Architecture and CUDA Programming
- Artificial Intelligence and Machine Learning Concepts
- Behavioral Questions and Soft Skills
- Conducting Mock Interviews
- Additional Resources and Study Materials
1. Understanding NVIDIA and Its Technology Focus
Before diving into the technical preparation, it’s crucial to understand NVIDIA’s role in the tech industry and its primary focus areas. NVIDIA is renowned for:
- Graphics Processing Units (GPUs) for gaming and professional visualization
- Parallel computing and CUDA programming
- Artificial Intelligence and Deep Learning
- Autonomous vehicles and robotics
- Data center solutions and high-performance computing
Familiarize yourself with NVIDIA’s products, recent developments, and their impact on various industries. This knowledge will not only help you during the interview but also demonstrate your genuine interest in the company.
2. The NVIDIA Interview Process
The NVIDIA interview process typically consists of several stages:
- Initial phone screen with a recruiter
- Technical phone interview with an engineer
- Online coding assessment
- On-site interviews (or virtual equivalent)
The on-site interviews usually include:
- Multiple technical interviews focusing on algorithms, data structures, and problem-solving
- System design and architecture discussion
- Domain-specific questions related to graphics, CUDA, or AI (depending on the role)
- Behavioral interviews to assess cultural fit and soft skills
3. Key Technical Skills to Master
To excel in NVIDIA’s technical interviews, focus on honing the following skills:
3.1. Data Structures and Algorithms
Master the fundamental data structures and algorithms, including:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching algorithms
- Dynamic Programming
- Greedy Algorithms
3.2. Programming Languages
Be proficient in at least one of the following languages:
- C++
- Python
- CUDA (for GPU-related roles)
3.3. Object-Oriented Programming (OOP)
Understand OOP concepts and be able to apply them in your code:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
3.4. Operating Systems and Computer Architecture
Have a solid grasp of:
- Process management
- Memory management
- File systems
- Concurrency and multithreading
- CPU architecture
4. Coding Practice and Problem-Solving Strategies
To sharpen your coding skills and problem-solving abilities, follow these strategies:
4.1. Solve Algorithmic Problems
Practice solving problems on platforms like:
- LeetCode
- HackerRank
- CodeForces
- AlgoExpert
Focus on medium to hard difficulty problems, especially those related to graphics, parallel processing, and optimization.
4.2. Implement Data Structures from Scratch
Gain a deeper understanding by implementing common data structures yourself:
class LinkedList:
def __init__(self):
self.head = None
class Node:
def __init__(self, data):
self.data = data
self.next = None
def append(self, data):
if not self.head:
self.head = self.Node(data)
return
current = self.head
while current.next:
current = current.next
current.next = self.Node(data)
def print_list(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
# Usage
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
ll.print_list() # Output: 1 -> 2 -> 3 -> None
4.3. Time and Space Complexity Analysis
Practice analyzing the time and space complexity of your solutions. NVIDIA interviewers often ask about the efficiency of your code and potential optimizations.
4.4. Mock Coding Interviews
Participate in mock coding interviews on platforms like Pramp or InterviewBit to simulate real interview conditions and receive feedback.
5. System Design and Architecture
For more senior positions, be prepared to discuss system design and architecture concepts:
5.1. Scalability
- Horizontal vs. Vertical scaling
- Load balancing
- Caching strategies
- Database sharding
5.2. Distributed Systems
- CAP theorem
- Consistency models
- Partitioning and replication
5.3. Design Patterns
Familiarize yourself with common design patterns and their applications:
- Singleton
- Factory
- Observer
- Strategy
5.4. Practice System Design Questions
Work on designing scalable systems like:
- A distributed file storage system
- A real-time multiplayer game server
- A video streaming platform
6. GPU Architecture and CUDA Programming
Given NVIDIA’s focus on GPUs, having knowledge in this area can be a significant advantage:
6.1. GPU Architecture
- Understand the differences between CPU and GPU architectures
- Learn about NVIDIA’s GPU architectures (e.g., Turing, Ampere)
- Familiarize yourself with concepts like SIMD (Single Instruction, Multiple Data)
6.2. CUDA Programming
If you’re applying for a GPU-related role, learn the basics of CUDA programming:
#include <cuda_runtime.h>
#include <stdio.h>
__global__ void vectorAdd(float *a, float *b, float *c, int n) {
int i = blockDim.x * blockIdx.x + threadIdx.x;
if (i < n) {
c[i] = a[i] + b[i];
}
}
int main() {
int n = 1000000;
size_t size = n * sizeof(float);
float *h_a = (float *)malloc(size);
float *h_b = (float *)malloc(size);
float *h_c = (float *)malloc(size);
float *d_a, *d_b, *d_c;
cudaMalloc(&d_a, size);
cudaMalloc(&d_b, size);
cudaMalloc(&d_c, size);
// Initialize input vectors
for (int i = 0; i < n; i++) {
h_a[i] = rand() / (float)RAND_MAX;
h_b[i] = rand() / (float)RAND_MAX;
}
cudaMemcpy(d_a, h_a, size, cudaMemcpyHostToDevice);
cudaMemcpy(d_b, h_b, size, cudaMemcpyHostToDevice);
int threadsPerBlock = 256;
int blocksPerGrid = (n + threadsPerBlock - 1) / threadsPerBlock;
vectorAdd<<<blocksPerGrid, threadsPerBlock>>>(d_a, d_b, d_c, n);
cudaMemcpy(h_c, d_c, size, cudaMemcpyDeviceToHost);
// Verify the result
for (int i = 0; i < n; i++) {
if (fabs(h_a[i] + h_b[i] - h_c[i]) > 1e-5) {
fprintf(stderr, "Result verification failed at element %d!\n", i);
exit(1);
}
}
printf("Test PASSED\n");
// Free device memory
cudaFree(d_a);
cudaFree(d_b);
cudaFree(d_c);
// Free host memory
free(h_a);
free(h_b);
free(h_c);
return 0;
}
6.3. Parallel Computing Concepts
- Thread hierarchy in CUDA
- Memory hierarchy and coalescing
- Synchronization and atomic operations
7. Artificial Intelligence and Machine Learning Concepts
Given NVIDIA’s significant involvement in AI and ML, having knowledge in these areas can be beneficial:
7.1. Machine Learning Fundamentals
- Supervised vs. Unsupervised learning
- Common algorithms (e.g., Linear Regression, Decision Trees, Neural Networks)
- Model evaluation metrics
7.2. Deep Learning
- Neural network architectures (CNNs, RNNs, Transformers)
- Backpropagation and gradient descent
- Activation functions and loss functions
7.3. AI Frameworks
Familiarize yourself with popular AI frameworks, especially those optimized for NVIDIA GPUs:
- TensorFlow
- PyTorch
- NVIDIA’s CUDA-X AI libraries
8. Behavioral Questions and Soft Skills
Prepare for behavioral questions that assess your problem-solving approach, teamwork, and cultural fit:
8.1. Common Behavioral Questions
- Describe a challenging project you worked on and how you overcame obstacles.
- How do you handle disagreements with team members?
- Give an example of a time when you had to learn a new technology quickly.
8.2. STAR Method
Use the STAR (Situation, Task, Action, Result) method to structure your responses:
- Situation: Describe the context
- Task: Explain your responsibility
- Action: Detail the steps you took
- Result: Share the outcome and what you learned
8.3. Demonstrate Your Passion
Show your enthusiasm for technology and NVIDIA’s work. Discuss relevant personal projects or contributions to open-source initiatives.
9. Conducting Mock Interviews
Practice makes perfect. Conduct mock interviews to simulate the real experience:
9.1. Find a Study Partner
Team up with a friend or use online platforms to find interview partners.
9.2. Simulate Interview Conditions
- Use a whiteboard or shared coding environment
- Set a timer to practice working under pressure
- Verbalize your thought process as you solve problems
9.3. Seek Feedback
Ask your mock interviewer for honest feedback on your performance, including areas for improvement.
10. Additional Resources and Study Materials
Leverage these resources to enhance your preparation:
10.1. Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Programming Massively Parallel Processors” by David B. Kirk and Wen-mei W. Hwu
10.2. Online Courses
- Coursera: GPU Programming
- Udacity: Intro to Parallel Programming
- edX: Deep Learning with NVIDIA GPUs
10.3. NVIDIA Resources
- NVIDIA Developer Blog
- NVIDIA Deep Learning Institute
- CUDA Toolkit Documentation
10.4. Coding Platforms
- LeetCode
- HackerRank
- CodeForces
Conclusion
Preparing for an NVIDIA technical interview requires a multifaceted approach, combining strong algorithmic skills, knowledge of GPU architecture and parallel computing, and an understanding of AI and machine learning concepts. By following this comprehensive guide and consistently practicing, you’ll be well-equipped to tackle the challenges of NVIDIA’s interview process and showcase your skills effectively.
Remember that the key to success lies not just in memorizing information, but in developing a deep understanding of the underlying concepts and their practical applications. Stay curious, keep learning, and approach your preparation with enthusiasm. With dedication and the right strategy, you’ll be well on your way to impressing your interviewers and landing that dream job at NVIDIA.
Good luck with your preparation, and may your future shine as bright as the graphics NVIDIA’s GPUs render!