Mastering Number Conversion: Essential Strategies for Coding Interviews
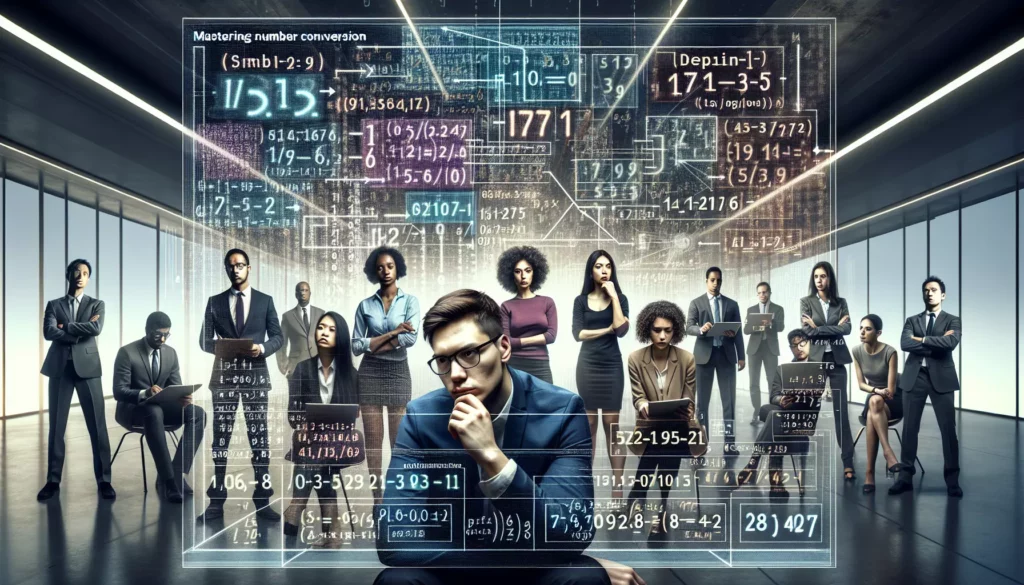
In the world of computer science and programming, number conversion problems are a fundamental concept that often appears in coding interviews, especially for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). As you prepare for these interviews or aim to enhance your coding skills, understanding and mastering number conversion strategies is crucial. This comprehensive guide will walk you through various techniques and approaches to solve number conversion problems efficiently.
1. Understanding the Basics of Number Systems
Before diving into conversion strategies, it’s essential to have a solid grasp of different number systems. The most common number systems in computer science are:
- Binary (Base-2)
- Decimal (Base-10)
- Hexadecimal (Base-16)
- Octal (Base-8)
Each system uses a different set of digits to represent numbers. For instance, binary uses only 0 and 1, while hexadecimal uses digits 0-9 and letters A-F. Understanding the fundamentals of these systems is crucial for tackling conversion problems effectively.
2. Decimal to Binary Conversion
Converting decimal numbers to binary is a common problem in coding interviews. Here are two primary methods to approach this conversion:
2.1. Division Method
This method involves repeatedly dividing the decimal number by 2 and keeping track of the remainders. The steps are as follows:
- Divide the decimal number by 2.
- Note down the remainder (0 or 1).
- Continue dividing the quotient by 2 until you reach 0.
- Read the remainders from bottom to top to get the binary number.
Here’s a Python implementation of this method:
def decimal_to_binary(decimal):
if decimal == 0:
return "0"
binary = ""
while decimal > 0:
binary = str(decimal % 2) + binary
decimal //= 2
return binary
# Example usage
print(decimal_to_binary(25)) # Output: 11001
2.2. Bitwise Method
This method uses bitwise operations to perform the conversion. It’s often faster and more efficient for larger numbers. Here’s how it works:
def decimal_to_binary_bitwise(decimal):
return bin(decimal)[2:] # [2:] removes the "0b" prefix
# Example usage
print(decimal_to_binary_bitwise(25)) # Output: 11001
The bin()
function in Python converts an integer to a binary string prefixed with “0b”. We slice off this prefix to get the pure binary representation.
3. Binary to Decimal Conversion
Converting binary numbers back to decimal is another essential skill. Here are two methods to accomplish this:
3.1. Positional Value Method
This method involves multiplying each digit by its positional value (power of 2) and summing the results. Here’s a Python implementation:
def binary_to_decimal(binary):
decimal = 0
for i, digit in enumerate(binary[::-1]):
if digit == '1':
decimal += 2 ** i
return decimal
# Example usage
print(binary_to_decimal("11001")) # Output: 25
3.2. Built-in Method
Python provides a built-in function to convert binary strings to integers:
def binary_to_decimal_builtin(binary):
return int(binary, 2)
# Example usage
print(binary_to_decimal_builtin("11001")) # Output: 25
This method is concise and efficient, especially for interview situations where time is limited.
4. Hexadecimal Conversions
Hexadecimal numbers are frequently used in computer systems, particularly for representing memory addresses and color values. Let’s explore conversions involving hexadecimal numbers.
4.1. Decimal to Hexadecimal
To convert decimal to hexadecimal, we can use a similar approach to the decimal-to-binary conversion, but with a base of 16:
def decimal_to_hex(decimal):
hex_chars = "0123456789ABCDEF"
if decimal == 0:
return "0"
hex_string = ""
while decimal > 0:
hex_string = hex_chars[decimal % 16] + hex_string
decimal //= 16
return hex_string
# Example usage
print(decimal_to_hex(2748)) # Output: ABC
4.2. Hexadecimal to Decimal
Converting hexadecimal to decimal involves multiplying each digit by its positional value (power of 16) and summing the results:
def hex_to_decimal(hex_string):
hex_chars = "0123456789ABCDEF"
decimal = 0
for i, char in enumerate(hex_string[::-1]):
decimal += hex_chars.index(char.upper()) * (16 ** i)
return decimal
# Example usage
print(hex_to_decimal("ABC")) # Output: 2748
5. Octal Conversions
While less common than binary and hexadecimal, octal numbers still appear in some systems and interview questions. Let’s look at conversions involving octal numbers.
5.1. Decimal to Octal
The process is similar to decimal-to-binary conversion, but using base 8:
def decimal_to_octal(decimal):
if decimal == 0:
return "0"
octal = ""
while decimal > 0:
octal = str(decimal % 8) + octal
decimal //= 8
return octal
# Example usage
print(decimal_to_octal(78)) # Output: 116
5.2. Octal to Decimal
Converting octal to decimal follows the same principle as binary and hexadecimal to decimal conversions:
def octal_to_decimal(octal):
decimal = 0
for i, digit in enumerate(octal[::-1]):
decimal += int(digit) * (8 ** i)
return decimal
# Example usage
print(octal_to_decimal("116")) # Output: 78
6. Advanced Conversion Strategies
As you progress in your coding journey and face more complex interview questions, you may encounter advanced conversion problems. Here are some strategies to tackle them:
6.1. Bitwise Operations for Base-2 Power Conversions
When dealing with conversions between bases that are powers of 2 (e.g., binary, hexadecimal), bitwise operations can be extremely efficient. For instance, to convert between binary and hexadecimal:
def binary_to_hex(binary):
# Pad the binary string to a multiple of 4
padded_binary = binary.zfill((len(binary) + 3) // 4 * 4)
hex_string = ""
for i in range(0, len(padded_binary), 4):
chunk = padded_binary[i:i+4]
hex_digit = hex(int(chunk, 2))[2:]
hex_string += hex_digit
return hex_string
def hex_to_binary(hex_string):
binary = ""
for digit in hex_string:
binary += bin(int(digit, 16))[2:].zfill(4)
return binary
# Example usage
print(binary_to_hex("1010111100")) # Output: 2BC
print(hex_to_binary("2BC")) # Output: 001010111100
6.2. Custom Base Conversions
In some advanced scenarios, you might need to convert between custom bases. Here’s a generalized function for base-to-base conversion:
def convert_base(number, from_base, to_base):
# First, convert to decimal
decimal = int(str(number), from_base)
# Then, convert decimal to the target base
if to_base == 10:
return str(decimal)
digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ"
result = ""
while decimal > 0:
result = digits[decimal % to_base] + result
decimal //= to_base
return result or "0"
# Example usage
print(convert_base("1010", 2, 16)) # Binary to Hex: A
print(convert_base("FF", 16, 2)) # Hex to Binary: 11111111
print(convert_base("42", 10, 36)) # Decimal to Base-36: 16
This function can handle conversions between any bases from 2 to 36 (using digits 0-9 and letters A-Z).
7. Optimization Techniques
When solving number conversion problems in coding interviews, optimization is key. Here are some techniques to improve the efficiency of your solutions:
7.1. Lookup Tables
For conversions involving a fixed set of values (like hexadecimal digits), using a lookup table can significantly speed up the process:
hex_lookup = {
0: '0', 1: '1', 2: '2', 3: '3', 4: '4', 5: '5', 6: '6', 7: '7',
8: '8', 9: '9', 10: 'A', 11: 'B', 12: 'C', 13: 'D', 14: 'E', 15: 'F'
}
def optimized_decimal_to_hex(decimal):
if decimal == 0:
return "0"
hex_string = ""
while decimal > 0:
hex_string = hex_lookup[decimal % 16] + hex_string
decimal //= 16
return hex_string
# Example usage
print(optimized_decimal_to_hex(2748)) # Output: ABC
7.2. Bitwise Operations
For binary-related conversions, bitwise operations can be much faster than arithmetic operations:
def optimized_decimal_to_binary(decimal):
if decimal == 0:
return "0"
binary = ""
while decimal:
binary = str(decimal & 1) + binary
decimal >>= 1
return binary
# Example usage
print(optimized_decimal_to_binary(25)) # Output: 11001
7.3. Built-in Functions
Many programming languages offer built-in functions for common conversions. While it’s important to understand the underlying principles, using these functions can save time in interview situations:
def quick_conversions(decimal):
binary = bin(decimal)[2:]
octal = oct(decimal)[2:]
hexadecimal = hex(decimal)[2:].upper()
return binary, octal, hexadecimal
# Example usage
print(quick_conversions(42)) # Output: ('101010', '52', '2A')
8. Common Pitfalls and How to Avoid Them
When working on number conversion problems, there are several common mistakes that candidates often make. Being aware of these pitfalls can help you avoid them during interviews:
8.1. Forgetting to Handle Zero
Many conversion algorithms fail when the input is zero. Always include a check for zero at the beginning of your function:
def safe_decimal_to_binary(decimal):
if decimal == 0:
return "0"
# Rest of the conversion logic
8.2. Incorrect Handling of Negative Numbers
Some conversion problems may involve negative numbers. Be sure to handle them correctly:
def decimal_to_binary_with_sign(decimal):
if decimal == 0:
return "0"
is_negative = decimal < 0
decimal = abs(decimal)
binary = ""
while decimal:
binary = str(decimal & 1) + binary
decimal >>= 1
return ("-" if is_negative else "") + binary
# Example usage
print(decimal_to_binary_with_sign(-25)) # Output: -11001
8.3. Overlooking Integer Overflow
In languages with fixed-size integers, be mindful of potential overflow issues, especially when dealing with large numbers:
def safe_binary_to_decimal(binary):
decimal = 0
for digit in binary:
decimal = decimal * 2 + int(digit)
if decimal > 2**31 - 1: # Check for 32-bit integer overflow
raise OverflowError("Result exceeds 32-bit integer range")
return decimal
# Example usage
try:
print(safe_binary_to_decimal("11111111111111111111111111111111"))
except OverflowError as e:
print(f"Error: {e}")
9. Practice Problems and Solutions
To reinforce your understanding of number conversion strategies, here are some practice problems along with their solutions:
Problem 1: Binary Addition
Given two binary strings, return their sum (also a binary string).
def add_binary(a, b):
return bin(int(a, 2) + int(b, 2))[2:]
# Example usage
print(add_binary("1010", "1011")) # Output: 10101
Problem 2: Roman to Integer
Convert a Roman numeral to an integer.
def roman_to_int(s):
roman_values = {'I': 1, 'V': 5, 'X': 10, 'L': 50, 'C': 100, 'D': 500, 'M': 1000}
total = 0
prev_value = 0
for char in reversed(s):
current_value = roman_values[char]
if current_value >= prev_value:
total += current_value
else:
total -= current_value
prev_value = current_value
return total
# Example usage
print(roman_to_int("MCMLIV")) # Output: 1954
Problem 3: Excel Sheet Column Number
Given a column title as it appears in an Excel sheet, return its corresponding column number.
def title_to_number(column_title):
result = 0
for char in column_title:
result = result * 26 + (ord(char) - ord('A') + 1)
return result
# Example usage
print(title_to_number("AB")) # Output: 28
10. Interview Strategies and Tips
When tackling number conversion problems in coding interviews, keep these strategies and tips in mind:
- Clarify the problem: Always start by asking clarifying questions. Confirm the input and output formats, and any constraints on the input values.
- Think out loud: Explain your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach.
- Start with a simple approach: Begin with a straightforward solution, even if it’s not the most efficient. You can optimize later if time permits.
- Consider edge cases: Think about special cases like zero, negative numbers, or very large inputs. Mention these to the interviewer and explain how you’ll handle them.
- Optimize incrementally: If your initial solution works, discuss potential optimizations. This shows your ability to balance correctness with efficiency.
- Use built-in functions judiciously: While it’s important to know how to implement conversions from scratch, don’t hesitate to mention built-in functions if they’re more efficient.
- Test your code: Before declaring you’re done, walk through your code with a few test cases to catch any logical errors.
- Analyze time and space complexity: Be prepared to discuss the time and space complexity of your solution.
Conclusion
Mastering number conversion strategies is crucial for success in coding interviews, especially for positions at top tech companies. By understanding the fundamental principles, practicing various conversion techniques, and being aware of common pitfalls, you’ll be well-prepared to tackle these problems confidently.
Remember that the key to excelling in coding interviews is not just about knowing the solutions, but also about demonstrating your problem-solving process, communication skills, and ability to write clean, efficient code. Keep practicing, stay curious, and approach each problem as an opportunity to showcase your skills and learn something new.
As you continue your journey in coding education and skill development, platforms like AlgoCademy can provide valuable resources, interactive tutorials, and AI-powered assistance to help you refine your algorithmic thinking and prepare effectively for technical interviews. With dedication and the right strategies, you’ll be well-equipped to tackle any number conversion problem that comes your way in your coding career.