Mastering Non-Relational Databases: A Deep Dive into Redis, Neo4j, and CouchDB
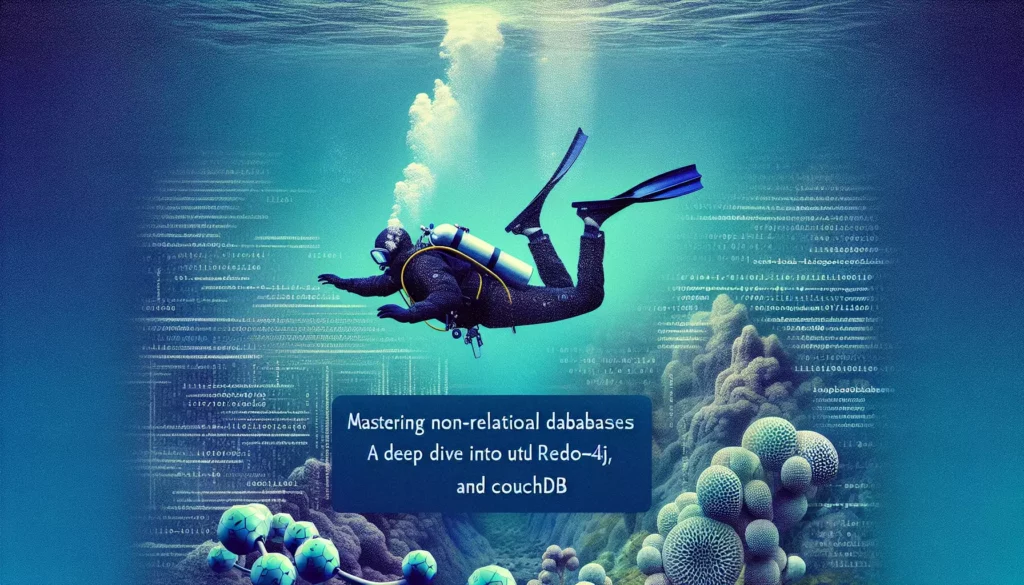
In the ever-evolving landscape of database technologies, non-relational databases have emerged as powerful alternatives to traditional relational database management systems. As aspiring developers and seasoned programmers alike seek to expand their skill sets, understanding and working with non-relational databases has become increasingly important. In this comprehensive guide, we’ll explore three popular non-relational databases: Redis, Neo4j, and CouchDB. We’ll delve into their unique features, use cases, and provide practical examples to help you get started on your journey to mastering these innovative database solutions.
Understanding Non-Relational Databases
Before we dive into the specifics of Redis, Neo4j, and CouchDB, let’s first understand what non-relational databases are and how they differ from their relational counterparts.
Non-relational databases, also known as NoSQL databases, are designed to handle large volumes of unstructured or semi-structured data. Unlike relational databases that organize data into tables with predefined schemas, non-relational databases offer more flexibility in data storage and retrieval. They can handle various data types and structures, making them ideal for scenarios where data models may evolve rapidly or where scalability is a primary concern.
Some key advantages of non-relational databases include:
- Scalability: Many non-relational databases are designed to scale horizontally, allowing for easy distribution across multiple servers.
- Flexibility: They can handle diverse data types and structures without requiring a fixed schema.
- Performance: Non-relational databases often offer better performance for specific use cases, such as real-time data processing or graph-based queries.
- Agility: They allow for faster development cycles and easier adaptation to changing requirements.
Now, let’s explore three popular non-relational databases: Redis, Neo4j, and CouchDB.
Redis: In-Memory Data Structure Store
Redis, which stands for Remote Dictionary Server, is an open-source, in-memory data structure store that can be used as a database, cache, message broker, and queue. Its lightning-fast performance and versatility make it a popular choice for various applications, from real-time analytics to session management.
Key Features of Redis
- In-memory data storage: Redis keeps all data in RAM, enabling extremely fast read and write operations.
- Data structures: Redis supports various data structures, including strings, hashes, lists, sets, sorted sets, bitmaps, and more.
- Persistence: While primarily an in-memory database, Redis offers options for data persistence to disk.
- Pub/Sub messaging: Redis provides publish/subscribe functionality for building real-time applications.
- Lua scripting: Redis allows the execution of Lua scripts for complex operations.
Use Cases for Redis
- Caching: Redis is widely used as a caching layer to improve application performance.
- Session management: It’s an excellent choice for storing and managing user sessions in web applications.
- Real-time analytics: Redis can handle high-velocity data ingestion and processing for real-time analytics.
- Leaderboards and counting: Its sorted sets make it ideal for implementing leaderboards and counting systems.
- Job queues: Redis can be used to implement distributed job queues for background task processing.
Getting Started with Redis
To start working with Redis, you’ll need to install it on your system. Here’s a quick guide to getting Redis up and running:
- Install Redis:
- On Ubuntu:
sudo apt-get install redis-server
- On macOS with Homebrew:
brew install redis
- For other systems, refer to the official Redis download page.
- On Ubuntu:
- Start the Redis server:
- On most systems:
redis-server
- On most systems:
- Connect to Redis using the command-line interface:
redis-cli
Now that you have Redis running, let’s try some basic operations:
# Set a key-value pair
SET user:1 "John Doe"
# Get the value of a key
GET user:1
# Set multiple fields in a hash
HMSET user:2 name "Jane Smith" age 30 city "New York"
# Get all fields from a hash
HGETALL user:2
# Add elements to a set
SADD fruits apple banana orange
# Get all elements in a set
SMEMBERS fruits
# Increment a counter
INCR pageviews
# Get the current value of the counter
GET pageviews
These examples demonstrate some of the basic operations you can perform with Redis. As you become more familiar with Redis, you’ll discover its powerful features for handling complex data structures and operations.
Neo4j: Graph Database Management System
Neo4j is a graph database management system that focuses on storing and querying highly connected data. Unlike traditional relational databases, Neo4j uses a graph data model, which makes it exceptionally efficient for handling complex relationships between entities.
Key Features of Neo4j
- Native graph storage: Neo4j stores data in a graph structure, optimizing for relationship-heavy queries.
- Cypher query language: Neo4j uses Cypher, a declarative graph query language, for efficient data retrieval and manipulation.
- ACID compliance: Neo4j ensures data integrity through ACID (Atomicity, Consistency, Isolation, Durability) transactions.
- Scalability: Neo4j supports both vertical and horizontal scaling to handle large datasets.
- Visualization tools: Neo4j provides built-in tools for visualizing graph data and query results.
Use Cases for Neo4j
- Social networks: Modeling and querying complex social relationships.
- Recommendation engines: Generating personalized recommendations based on user behavior and preferences.
- Fraud detection: Identifying patterns and anomalies in financial transactions.
- Knowledge graphs: Building and querying large-scale knowledge bases.
- Network and IT operations: Modeling and analyzing complex infrastructure dependencies.
Getting Started with Neo4j
To begin working with Neo4j, follow these steps:
- Download and install Neo4j:
- Visit the Neo4j download page and choose the appropriate version for your system.
- Start the Neo4j server:
- On most systems, you can start Neo4j using the provided desktop application or command-line tools.
- Access the Neo4j Browser:
- Open a web browser and navigate to
http://localhost:7474
to access the Neo4j Browser interface.
- Open a web browser and navigate to
Now, let’s explore some basic Cypher queries to interact with Neo4j:
// Create nodes and relationships
CREATE (john:Person {name: "John Doe", age: 30})
CREATE (jane:Person {name: "Jane Smith", age: 28})
CREATE (john)-[:KNOWS]->(jane)
// Query the graph
MATCH (p:Person)
RETURN p
// Find relationships
MATCH (p1:Person)-[:KNOWS]->(p2:Person)
RETURN p1.name, p2.name
// Update node properties
MATCH (p:Person {name: "John Doe"})
SET p.age = 31
RETURN p
// Delete a node and its relationships
MATCH (p:Person {name: "Jane Smith"})
DETACH DELETE p
These examples demonstrate basic operations in Neo4j using the Cypher query language. As you become more familiar with Neo4j and Cypher, you’ll be able to perform more complex queries and analyze intricate relationships within your data.
CouchDB: Document-Oriented Database
CouchDB is an open-source, document-oriented database that emphasizes ease of use and reliability. It stores data as JSON documents and provides a RESTful HTTP API for interacting with the database, making it an excellent choice for web applications and mobile app backends.
Key Features of CouchDB
- Document-oriented storage: CouchDB stores data as JSON documents, allowing for flexible schema design.
- RESTful HTTP API: Interact with CouchDB using standard HTTP methods, making it easy to integrate with various programming languages and frameworks.
- Multi-version concurrency control (MVCC): CouchDB handles concurrent access without the need for locking.
- Replication and synchronization: Built-in support for bi-directional replication between databases.
- Map/Reduce views: Create custom views of your data using JavaScript functions.
Use Cases for CouchDB
- Web applications: CouchDB’s HTTP API makes it ideal for building web applications.
- Mobile app backends: Its synchronization capabilities are perfect for offline-first mobile apps.
- Content management systems: Flexible document storage is well-suited for managing diverse content types.
- Real-time data synchronization: CouchDB’s replication features enable real-time data sync across multiple devices or servers.
- Distributed systems: CouchDB’s design principles make it suitable for building distributed systems with eventual consistency.
Getting Started with CouchDB
To begin working with CouchDB, follow these steps:
- Install CouchDB:
- Visit the CouchDB download page and choose the appropriate version for your system.
- Start the CouchDB server:
- On most systems, CouchDB will start automatically after installation. You can also start it manually using the provided tools or system services.
- Access the CouchDB dashboard:
- Open a web browser and navigate to
http://localhost:5984/_utils
to access the Fauxton web interface.
- Open a web browser and navigate to
Now, let’s explore some basic operations using CouchDB’s HTTP API. We’ll use cURL for these examples, but you can use any HTTP client or programming language of your choice:
# Create a new database
curl -X PUT http://localhost:5984/mydb
# Create a new document
curl -X POST http://localhost:5984/mydb -H "Content-Type: application/json" -d '{"name": "John Doe", "age": 30}'
# Retrieve a document by ID
curl -X GET http://localhost:5984/mydb/<document_id>
# Update a document
curl -X PUT http://localhost:5984/mydb/<document_id> -H "Content-Type: application/json" -d '{"_rev": "<current_revision>", "name": "John Doe", "age": 31}'
# Delete a document
curl -X DELETE http://localhost:5984/mydb/<document_id>?rev=<current_revision>
# Create a view
curl -X PUT http://localhost:5984/mydb/_design/mydesign -H "Content-Type: application/json" -d '{"views": {"by_name": {"map": "function(doc) { emit(doc.name, doc); }"}}}'
# Query a view
curl -X GET http://localhost:5984/mydb/_design/mydesign/_view/by_name
These examples demonstrate basic CRUD operations and view creation in CouchDB using its HTTP API. As you become more familiar with CouchDB, you’ll be able to leverage its powerful features for building scalable and flexible applications.
Choosing the Right Non-Relational Database
When deciding which non-relational database to use for your project, consider the following factors:
- Data model: Choose a database that aligns with your data structure and access patterns.
- Redis: Ideal for simple key-value pairs and data structures.
- Neo4j: Best for highly connected data with complex relationships.
- CouchDB: Suitable for document-oriented data with flexible schemas.
- Performance requirements: Consider the read/write performance needs of your application.
- Redis: Offers extremely fast in-memory operations.
- Neo4j: Optimized for relationship-based queries.
- CouchDB: Provides good performance for document-based operations.
- Scalability: Evaluate the database’s ability to handle growing data and user loads.
- Redis: Supports clustering for horizontal scalability.
- Neo4j: Offers both vertical and horizontal scaling options.
- CouchDB: Designed for easy replication and distribution.
- Consistency and durability: Assess your application’s requirements for data consistency and persistence.
- Redis: Primarily in-memory with optional persistence.
- Neo4j: ACID-compliant with strong consistency.
- CouchDB: Eventually consistent with durable storage.
- Ecosystem and tooling: Consider the availability of libraries, tools, and community support.
- Redis: Wide adoption with extensive language support and tools.
- Neo4j: Strong graph visualization and analysis tools.
- CouchDB: Good integration with web technologies and mobile frameworks.
Conclusion
Non-relational databases like Redis, Neo4j, and CouchDB offer powerful alternatives to traditional relational databases, each with its own strengths and use cases. By understanding the unique features and capabilities of these databases, you can make informed decisions about which technology best suits your project requirements.
As you continue your journey in mastering non-relational databases, consider the following steps:
- Experiment with each database: Set up local instances of Redis, Neo4j, and CouchDB, and try building small projects or prototypes to gain hands-on experience.
- Dive deeper into documentation: Explore the official documentation for each database to learn about advanced features and best practices.
- Join community forums: Engage with the developer communities for these databases to learn from others’ experiences and stay updated on the latest developments.
- Explore real-world use cases: Research how companies are using these databases in production environments to understand their practical applications.
- Consider certification programs: Many of these databases offer certification programs that can help you demonstrate your expertise to potential employers.
By investing time in learning and working with non-relational databases like Redis, Neo4j, and CouchDB, you’ll expand your skill set and be better prepared to tackle diverse data management challenges in your future projects and career.