Mastering Multi-Part Interview Questions: Strategies for Success in Tech Interviews
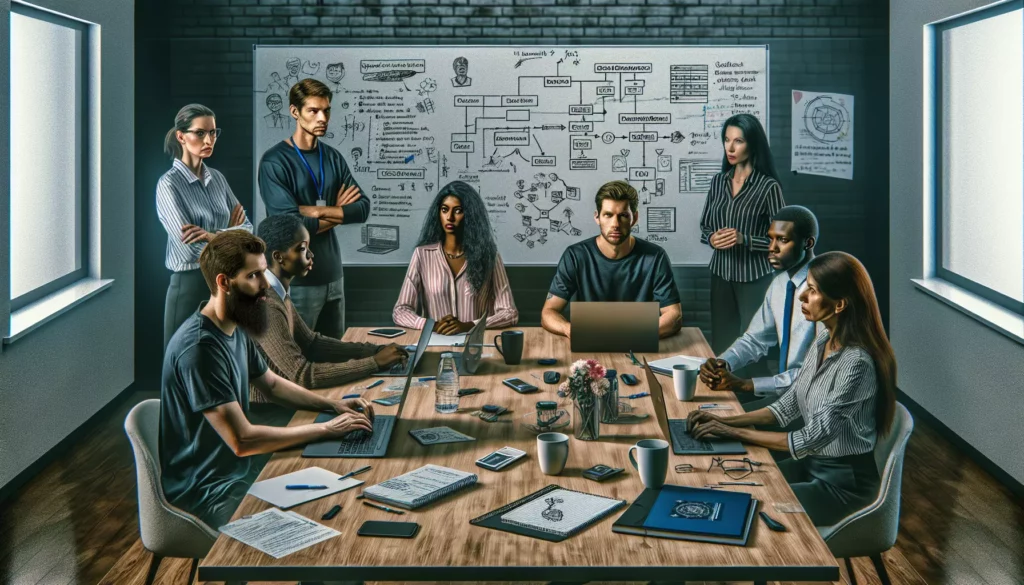
In the competitive world of tech interviews, particularly for positions at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), candidates often face complex, multi-part questions that test not only their coding skills but also their problem-solving abilities and thought processes. These questions can be intimidating, but with the right strategies, you can navigate them successfully. In this comprehensive guide, we’ll explore effective techniques for handling multi-part interview questions, helping you showcase your skills and stand out in your next technical interview.
Understanding Multi-Part Interview Questions
Before diving into strategies, it’s crucial to understand what multi-part interview questions are and why they’re used. These questions typically consist of several interconnected components or stages, each building upon the previous one. They’re designed to assess various aspects of a candidate’s abilities, including:
- Problem-solving skills
- Ability to break down complex issues
- Code optimization and efficiency
- Communication and explanation of thought processes
- Adaptability and handling of changing requirements
Interviewers use these questions to simulate real-world scenarios where problems evolve and requirements change, mirroring the dynamic nature of software development.
Key Strategies for Tackling Multi-Part Questions
1. Active Listening and Note-Taking
The first step in successfully handling multi-part questions is to listen carefully and take notes. As the interviewer presents the problem:
- Pay close attention to each part of the question
- Jot down key points, requirements, and constraints
- Ask for clarification if any part is unclear
This approach ensures you have a clear understanding of the entire problem before you start solving it.
2. Divide and Conquer
Break down the multi-part question into smaller, manageable components. This strategy helps in several ways:
- It makes the overall problem less overwhelming
- Allows you to focus on one part at a time
- Helps in identifying dependencies between different parts
For example, if asked to design a system with multiple features, tackle each feature individually while keeping the overall system architecture in mind.
3. Prioritize and Plan
After breaking down the question, prioritize the parts based on their importance and dependencies. Create a high-level plan outlining how you’ll approach each part. This demonstrates your ability to strategize and manage complex tasks efficiently.
4. Start with the Simplest Solution
Begin with the most straightforward solution for each part. This approach has several benefits:
- It helps you gain momentum and confidence
- Provides a foundation that you can build upon or optimize later
- Allows you to show your thought process clearly
Remember, it’s often better to have a working solution that you can improve than to get stuck trying to create the perfect solution from the start.
5. Communicate Continuously
Keep the interviewer informed about your thought process throughout. This includes:
- Explaining your approach for each part
- Discussing trade-offs and alternatives you’re considering
- Asking for feedback or hints if you’re stuck
Clear communication demonstrates your ability to work collaboratively and shows how you’d interact in a team environment.
6. Be Prepared for Follow-up Questions
Interviewers often add new requirements or ask follow-up questions as you progress. Be prepared to:
- Adapt your solution to new constraints
- Explain how changes in requirements affect your approach
- Discuss potential optimizations or alternative solutions
This flexibility showcases your ability to handle changing scenarios, a valuable skill in real-world software development.
7. Time Management
Multi-part questions often have time constraints. Manage your time effectively by:
- Allocating time for each part based on its complexity
- Setting mini-deadlines for yourself
- Being prepared to move on if you’re spending too much time on one part
Effective time management shows your ability to work under pressure and prioritize tasks.
Practical Examples and Scenarios
Let’s look at some examples of multi-part interview questions and how to approach them using the strategies discussed above.
Example 1: Design a Social Media Feed
Consider this multi-part question: “Design a social media feed system. Start with a basic feed, then add features for personalization, and finally implement a notification system.”
Approach:
- Basic Feed:
- Start with a simple chronological feed of posts from friends
- Implement basic CRUD operations for posts
- Personalization:
- Add user preferences and interests
- Implement a basic ranking algorithm based on user engagement
- Notification System:
- Design a system to track user interactions
- Implement push notifications for important events
For each part, start with a high-level design, then dive into specific implementation details. Discuss scalability considerations and potential optimizations as you progress.
Example 2: Implement a File System
Another multi-part question might be: “Implement a basic file system. Start with creating and listing files, then add support for directories, and finally implement a search functionality.”
Approach:
- Basic File Operations:
- Implement create, read, update, and delete operations for files
- Design a simple data structure to store file metadata
- Directory Support:
- Extend the data structure to support a hierarchical system
- Implement operations for creating and navigating directories
- Search Functionality:
- Implement a basic search algorithm (e.g., depth-first search)
- Discuss potential optimizations like indexing for faster searches
For each part, start by implementing the core functionality, then discuss how you would handle edge cases and scale the system.
Common Pitfalls and How to Avoid Them
When tackling multi-part interview questions, be aware of these common pitfalls:
1. Rushing to Code
Pitfall: Jumping into coding without fully understanding the problem or planning your approach.
Avoidance Strategy: Take time to analyze the question, break it down, and plan your approach before writing any code. Communicate your thought process to the interviewer.
2. Overcomplicating Initial Solutions
Pitfall: Trying to implement an overly complex or optimized solution from the start.
Avoidance Strategy: Begin with a simple, working solution. You can always optimize and refine it later if time permits.
3. Losing Sight of the Big Picture
Pitfall: Getting too focused on one part and forgetting how it fits into the overall problem.
Avoidance Strategy: Regularly step back and review how your current work fits into the larger context of the problem. Keep your initial plan in mind.
4. Poor Time Management
Pitfall: Spending too much time on one part, leaving insufficient time for others.
Avoidance Strategy: Set time limits for each part. If you’re stuck, communicate with the interviewer and consider moving on to the next part.
5. Neglecting to Test
Pitfall: Failing to test your solution or consider edge cases.
Avoidance Strategy: Allocate time for testing. Walk through your solution with sample inputs, including edge cases, to demonstrate its correctness.
Advanced Techniques for Excelling in Multi-Part Questions
To truly stand out in technical interviews, consider these advanced techniques:
1. Algorithmic Thinking
Develop a strong foundation in algorithmic thinking. This involves:
- Recognizing common patterns in problems
- Knowing when to apply specific data structures or algorithms
- Understanding time and space complexity trade-offs
Practice solving diverse problems to build this skill. Platforms like AlgoCademy offer a wide range of algorithmic challenges to help you prepare.
2. System Design Principles
For questions involving system design:
- Familiarize yourself with scalable architectures
- Understand concepts like load balancing, caching, and database sharding
- Practice explaining high-level designs and diving into specifics when asked
3. Code Refactoring and Optimization
As you progress through parts of a question:
- Look for opportunities to refactor your code for better readability or efficiency
- Discuss potential optimizations, even if you don’t implement them due to time constraints
- Show awareness of performance implications in your design choices
4. Handling Ambiguity
Multi-part questions often have intentional ambiguities. Develop skills to:
- Identify assumptions you’re making
- Ask clarifying questions effectively
- Make and justify decisions when information is limited
5. Demonstrating Breadth and Depth
Use multi-part questions as an opportunity to showcase:
- Broad knowledge across different areas of computer science
- Deep expertise in specific areas relevant to the role
- Ability to connect concepts from different domains
Preparing for Multi-Part Interview Questions
Effective preparation is key to success in handling multi-part interview questions. Here are some strategies to help you prepare:
1. Practice with Mock Interviews
Engage in mock interviews that simulate real interview conditions:
- Use platforms that offer realistic interview simulations
- Practice with peers or mentors who can provide feedback
- Record yourself to review your performance and communication style
2. Study Common Question Patterns
Familiarize yourself with frequently asked multi-part questions in tech interviews:
- Review interview experiences shared on platforms like Glassdoor or LeetCode
- Study company-specific interview patterns
- Identify recurring themes and question structures
3. Build a Strong Foundation
Strengthen your fundamental knowledge:
- Review core computer science concepts
- Practice implementing basic data structures and algorithms from scratch
- Stay updated with current technologies and industry trends
4. Develop Your Problem-Solving Process
Create a structured approach to problem-solving:
- Practice breaking down complex problems into smaller parts
- Work on explaining your thought process clearly and concisely
- Learn to manage your time effectively during problem-solving
5. Utilize Online Resources
Take advantage of online platforms and resources:
- Use coding practice websites like AlgoCademy, LeetCode, or HackerRank
- Watch video tutorials on problem-solving strategies
- Participate in coding competitions to improve your skills under pressure
Handling Stress and Maintaining Confidence
Multi-part questions can be stressful, but maintaining composure is crucial. Here are some tips:
1. Practice Stress Management Techniques
- Deep breathing exercises to calm nerves
- Positive self-talk to boost confidence
- Visualization techniques to imagine successful outcomes
2. Remember It’s a Dialogue
View the interview as a collaborative problem-solving session:
- Don’t hesitate to ask for clarification or hints
- Engage the interviewer in your thought process
- Be open to feedback and suggestions
3. Focus on Progress, Not Perfection
- Understand that it’s okay not to solve everything perfectly
- Demonstrate your problem-solving approach and ability to learn
- Show resilience in facing challenges
Post-Interview Reflection and Improvement
After each interview, take time to reflect and improve:
1. Review Your Performance
- Analyze what went well and areas for improvement
- Consider how you handled different parts of the questions
- Reflect on your communication and problem-solving approach
2. Seek Feedback
- If possible, ask the interviewer for feedback
- Discuss your experience with mentors or peers
- Use any feedback to refine your approach for future interviews
3. Continuous Learning
- Identify knowledge gaps revealed during the interview
- Study and practice areas where you felt less confident
- Stay updated with new technologies and interview trends
Conclusion
Mastering multi-part interview questions is a valuable skill that can significantly boost your chances of success in technical interviews, especially for positions at top tech companies. By employing strategies such as active listening, breaking down problems, effective communication, and continuous practice, you can approach these complex questions with confidence.
Remember, the key is not just to solve the problem but to demonstrate your thought process, adaptability, and problem-solving skills. With dedicated preparation and the right mindset, you can turn challenging multi-part questions into opportunities to showcase your abilities and stand out as a candidate.
As you continue your journey in tech interviews, platforms like AlgoCademy can be invaluable resources, offering targeted practice, AI-powered assistance, and a wealth of learning materials to help you refine your skills and approach interviews with confidence. Keep practicing, stay curious, and embrace each interview as a chance to learn and grow. With persistence and the right strategies, you’ll be well-equipped to tackle any multi-part question that comes your way in your tech interview journey.