Mastering Matrix Traversal: Essential Techniques for Solving Coding Problems
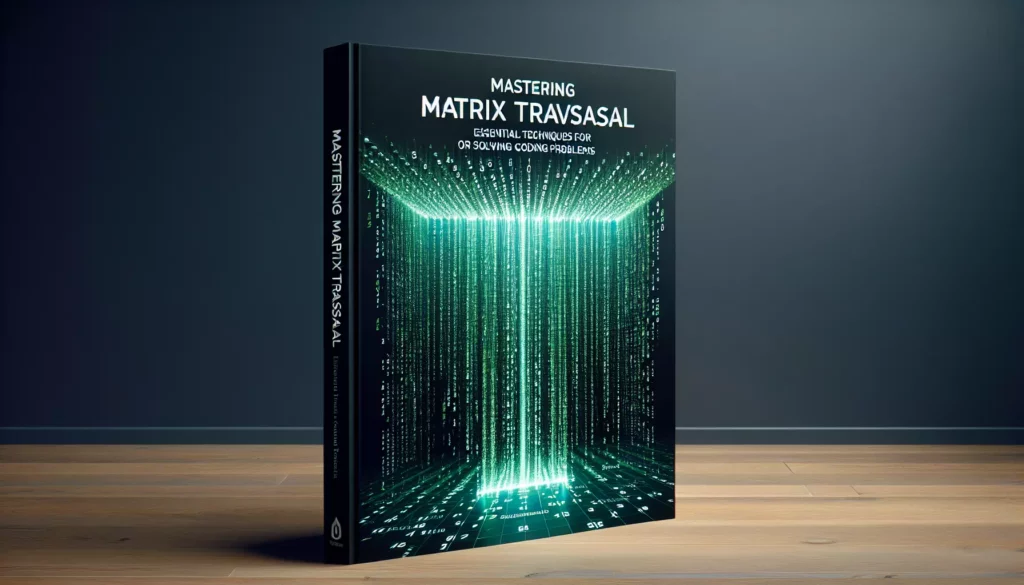
Matrix traversal problems are a common challenge in coding interviews and algorithmic problem-solving. These problems involve navigating through a two-dimensional array or grid, often with specific constraints or objectives. Whether you’re preparing for technical interviews at top tech companies or simply looking to improve your coding skills, understanding matrix traversal techniques is crucial. In this comprehensive guide, we’ll explore various approaches to solving matrix traversal problems, complete with examples and practical tips.
Understanding Matrix Traversal
Before diving into specific techniques, let’s clarify what we mean by matrix traversal. In programming, a matrix is typically represented as a 2D array, where each element is accessed using row and column indices. Traversal refers to the process of visiting each element in the matrix in a specific order or pattern.
Matrix traversal problems can vary widely in their objectives, such as:
- Finding a path from one point to another
- Counting specific elements or patterns
- Transforming the matrix based on certain rules
- Searching for particular sequences or shapes
The key to solving these problems efficiently lies in choosing the right traversal technique and data structures. Let’s explore some of the most common and effective approaches.
1. Basic Traversal Techniques
Row-wise Traversal
The simplest form of matrix traversal is going through each row from left to right, and then moving to the next row. This is often the default approach when you need to visit every element in the matrix exactly once.
def row_wise_traversal(matrix):
rows, cols = len(matrix), len(matrix[0])
for i in range(rows):
for j in range(cols):
print(matrix[i][j], end=' ')
print() # Move to the next line after each row
Column-wise Traversal
Similar to row-wise traversal, but we iterate through each column from top to bottom before moving to the next column.
def column_wise_traversal(matrix):
rows, cols = len(matrix), len(matrix[0])
for j in range(cols):
for i in range(rows):
print(matrix[i][j], end=' ')
print() # Move to the next line after each column
Spiral Traversal
Spiral traversal involves moving around the matrix in a clockwise or counterclockwise spiral pattern. This technique is often used in problems where you need to process elements in a specific order.
def spiral_traversal(matrix):
if not matrix:
return []
result = []
rows, cols = len(matrix), len(matrix[0])
top, bottom, left, right = 0, rows - 1, 0, cols - 1
while top <= bottom and left <= right:
# Traverse right
for j in range(left, right + 1):
result.append(matrix[top][j])
top += 1
# Traverse down
for i in range(top, bottom + 1):
result.append(matrix[i][right])
right -= 1
if top <= bottom:
# Traverse left
for j in range(right, left - 1, -1):
result.append(matrix[bottom][j])
bottom -= 1
if left <= right:
# Traverse up
for i in range(bottom, top - 1, -1):
result.append(matrix[i][left])
left += 1
return result
2. Depth-First Search (DFS)
Depth-First Search is a powerful technique for exploring matrices, especially when dealing with connected components or path-finding problems. DFS explores as far as possible along each branch before backtracking.
Recursive DFS
def dfs(matrix, i, j, visited):
rows, cols = len(matrix), len(matrix[0])
# Check if current position is out of bounds or already visited
if i < 0 or i >= rows or j < 0 or j >= cols or visited[i][j]:
return
visited[i][j] = True
print(matrix[i][j], end=' ') # Process current cell
# Explore neighbors (up, right, down, left)
directions = [(-1, 0), (0, 1), (1, 0), (0, -1)]
for di, dj in directions:
dfs(matrix, i + di, j + dj, visited)
def dfs_traversal(matrix):
rows, cols = len(matrix), len(matrix[0])
visited = [[False for _ in range(cols)] for _ in range(rows)]
for i in range(rows):
for j in range(cols):
if not visited[i][j]:
dfs(matrix, i, j, visited)
Iterative DFS using Stack
from collections import deque
def iterative_dfs(matrix):
rows, cols = len(matrix), len(matrix[0])
visited = [[False for _ in range(cols)] for _ in range(rows)]
stack = deque([(0, 0)]) # Start from top-left corner
while stack:
i, j = stack.pop()
if i < 0 or i >= rows or j < 0 or j >= cols or visited[i][j]:
continue
visited[i][j] = True
print(matrix[i][j], end=' ') # Process current cell
# Add neighbors to stack (up, right, down, left)
directions = [(-1, 0), (0, 1), (1, 0), (0, -1)]
for di, dj in directions:
stack.append((i + di, j + dj))
3. Breadth-First Search (BFS)
Breadth-First Search is excellent for finding the shortest path in unweighted graphs or exploring levels in a matrix. It visits all the neighbors at the present depth before moving to nodes at the next depth level.
from collections import deque
def bfs(matrix):
rows, cols = len(matrix), len(matrix[0])
visited = [[False for _ in range(cols)] for _ in range(rows)]
queue = deque([(0, 0)]) # Start from top-left corner
while queue:
i, j = queue.popleft()
if i < 0 or i >= rows or j < 0 or j >= cols or visited[i][j]:
continue
visited[i][j] = True
print(matrix[i][j], end=' ') # Process current cell
# Add neighbors to queue (up, right, down, left)
directions = [(-1, 0), (0, 1), (1, 0), (0, -1)]
for di, dj in directions:
queue.append((i + di, j + dj))
4. Dynamic Programming in Matrix Traversal
Dynamic Programming (DP) is a powerful technique for solving optimization problems in matrix traversal. It’s particularly useful when the problem involves finding the best path or counting the number of ways to reach a destination.
Example: Minimum Path Sum
Let’s solve a classic DP problem: finding the minimum path sum from the top-left to the bottom-right of a matrix, moving only right and down.
def min_path_sum(grid):
m, n = len(grid), len(grid[0])
dp = [[0 for _ in range(n)] for _ in range(m)]
# Initialize first cell
dp[0][0] = grid[0][0]
# Initialize first row
for j in range(1, n):
dp[0][j] = dp[0][j-1] + grid[0][j]
# Initialize first column
for i in range(1, m):
dp[i][0] = dp[i-1][0] + grid[i][0]
# Fill the DP table
for i in range(1, m):
for j in range(1, n):
dp[i][j] = min(dp[i-1][j], dp[i][j-1]) + grid[i][j]
return dp[m-1][n-1] # Return the minimum path sum
5. Sliding Window Technique
The sliding window technique is useful for problems that involve subarrays or submatrices. While it’s more commonly used with 1D arrays, it can be adapted for 2D matrices as well.
Example: Maximum Sum of a 3×3 Submatrix
def max_sum_submatrix(matrix, k):
rows, cols = len(matrix), len(matrix[0])
if k > rows or k > cols:
return None
# Compute prefix sum matrix
prefix_sum = [[0 for _ in range(cols+1)] for _ in range(rows+1)]
for i in range(1, rows+1):
for j in range(1, cols+1):
prefix_sum[i][j] = (prefix_sum[i-1][j] + prefix_sum[i][j-1]
- prefix_sum[i-1][j-1] + matrix[i-1][j-1])
max_sum = float('-inf')
for i in range(k, rows+1):
for j in range(k, cols+1):
current_sum = (prefix_sum[i][j] - prefix_sum[i-k][j]
- prefix_sum[i][j-k] + prefix_sum[i-k][j-k])
max_sum = max(max_sum, current_sum)
return max_sum
6. Flood Fill Algorithm
The Flood Fill algorithm is used to determine and modify connected regions in a multi-dimensional array. It’s commonly used in image processing and game development.
def flood_fill(image, sr, sc, new_color):
rows, cols = len(image), len(image[0])
old_color = image[sr][sc]
if old_color == new_color:
return image
def dfs(r, c):
if (r < 0 or r >= rows or c < 0 or c >= cols
or image[r][c] != old_color):
return
image[r][c] = new_color
# Recursively fill in all directions
dfs(r+1, c)
dfs(r-1, c)
dfs(r, c+1)
dfs(r, c-1)
dfs(sr, sc)
return image
7. Binary Search on Matrix
For sorted matrices, binary search can be an efficient way to find elements or solve certain types of problems.
Example: Search in a Sorted Matrix
def search_matrix(matrix, target):
if not matrix or not matrix[0]:
return False
rows, cols = len(matrix), len(matrix[0])
left, right = 0, rows * cols - 1
while left <= right:
mid = (left + right) // 2
mid_value = matrix[mid // cols][mid % cols]
if mid_value == target:
return True
elif mid_value < target:
left = mid + 1
else:
right = mid - 1
return False
8. Union-Find (Disjoint Set) for Matrix Problems
The Union-Find data structure can be useful for problems involving connected components in a matrix, especially when dealing with dynamic connectivity.
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x])
return self.parent[x]
def union(self, x, y):
px, py = self.find(x), self.find(y)
if px == py:
return False
if self.rank[px] < self.rank[py]:
self.parent[px] = py
elif self.rank[px] > self.rank[py]:
self.parent[py] = px
else:
self.parent[py] = px
self.rank[px] += 1
return True
def num_islands(grid):
if not grid or not grid[0]:
return 0
rows, cols = len(grid), len(grid[0])
uf = UnionFind(rows * cols)
count = 0
for i in range(rows):
for j in range(cols):
if grid[i][j] == '1':
count += 1
for di, dj in [(0, 1), (1, 0)]:
ni, nj = i + di, j + dj
if (0 <= ni < rows and 0 <= nj < cols
and grid[ni][nj] == '1'):
if uf.union(i*cols+j, ni*cols+nj):
count -= 1
return count
Conclusion
Mastering matrix traversal techniques is essential for solving a wide range of coding problems efficiently. The approaches we’ve covered – from basic traversals to advanced algorithms like DFS, BFS, and Dynamic Programming – form a solid foundation for tackling matrix-related challenges in coding interviews and real-world applications.
Remember, the key to success in solving these problems lies not just in knowing the techniques, but in practicing their application across various problem types. As you encounter new matrix traversal problems, try to identify which of these techniques might be most suitable, and don’t hesitate to combine multiple approaches when necessary.
Keep practicing, and you’ll find that your ability to navigate and manipulate matrices will improve significantly, making you better prepared for technical interviews and algorithmic problem-solving in general.
Additional Resources
To further enhance your matrix traversal skills, consider exploring these additional resources:
- LeetCode’s Matrix category for practice problems
- Geeksforgeeks’ articles on matrix algorithms
- Advanced algorithm textbooks like “Introduction to Algorithms” by Cormen et al.
- Online courses on data structures and algorithms
Remember, consistent practice and exposure to various problem types will help solidify your understanding and improve your problem-solving skills. Happy coding!