Mastering Linked List Problems for Interviews: A Comprehensive Guide
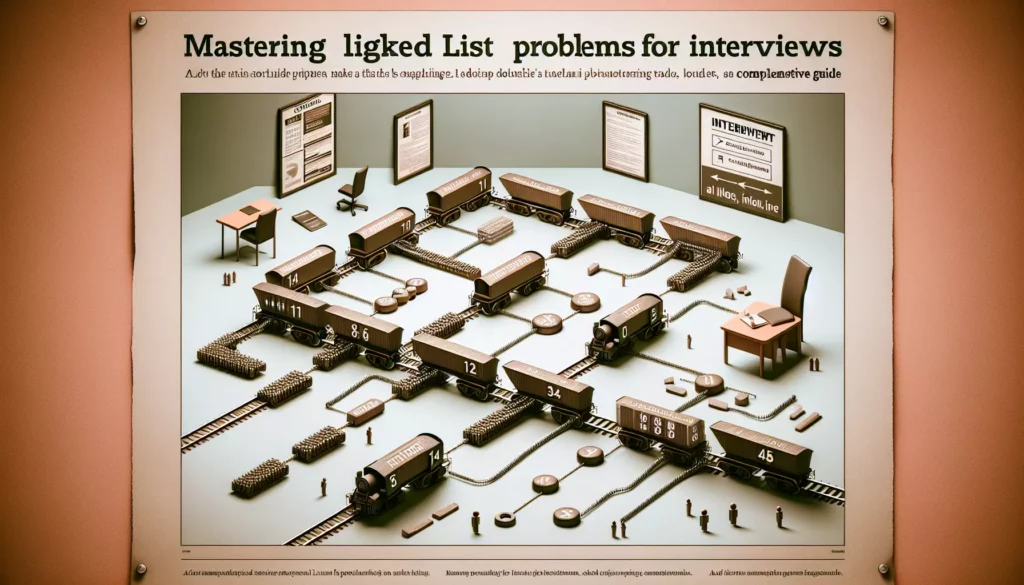
Linked lists are a fundamental data structure that frequently appear in coding interviews, especially for top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). Mastering linked list problems is crucial for success in these high-stakes interviews. In this comprehensive guide, we’ll explore various types of linked list problems, common techniques to solve them, and provide detailed examples to help you prepare effectively.
Table of Contents
- Introduction to Linked Lists
- Basic Linked List Operations
- Common Linked List Problems
- Advanced Techniques for Linked List Problems
- Interview Strategies for Linked List Questions
- Practice Problems and Solutions
- Conclusion
1. Introduction to Linked Lists
A linked list is a linear data structure where elements are stored in nodes. Each node contains a data field and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists do not store elements in contiguous memory locations, allowing for efficient insertions and deletions.
Types of Linked Lists
- Singly Linked List: Each node points to the next node in the sequence.
- Doubly Linked List: Each node has pointers to both the next and previous nodes.
- Circular Linked List: The last node points back to the first node, forming a circle.
Here’s a basic implementation of a singly linked list node in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
2. Basic Linked List Operations
Before diving into complex problems, it’s essential to master the basic operations on linked lists. These operations form the foundation for solving more advanced problems.
Traversal
Traversing a linked list involves visiting each node in the list sequentially.
def traverse(head):
current = head
while current:
print(current.val)
current = current.next
Insertion
Inserting a new node can be done at the beginning, end, or at a specific position in the list.
def insert_at_beginning(head, val):
new_node = ListNode(val)
new_node.next = head
return new_node
def insert_at_end(head, val):
new_node = ListNode(val)
if not head:
return new_node
current = head
while current.next:
current = current.next
current.next = new_node
return head
def insert_at_position(head, val, position):
if position == 0:
return insert_at_beginning(head, val)
new_node = ListNode(val)
current = head
for _ in range(position - 1):
if not current:
return head
current = current.next
new_node.next = current.next
current.next = new_node
return head
Deletion
Deleting a node can be done at the beginning, end, or at a specific position in the list.
def delete_at_beginning(head):
if not head:
return None
return head.next
def delete_at_end(head):
if not head or not head.next:
return None
current = head
while current.next.next:
current = current.next
current.next = None
return head
def delete_at_position(head, position):
if position == 0:
return delete_at_beginning(head)
current = head
for _ in range(position - 1):
if not current or not current.next:
return head
current = current.next
if current.next:
current.next = current.next.next
return head
3. Common Linked List Problems
Now that we’ve covered the basics, let’s explore some common linked list problems that frequently appear in coding interviews.
Reversing a Linked List
Reversing a linked list is a classic problem that tests your understanding of pointer manipulation.
def reverse_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Detecting a Cycle
Detecting a cycle in a linked list is often solved using the Floyd’s Cycle-Finding Algorithm (also known as the “tortoise and hare” algorithm).
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
Finding the Middle Node
Finding the middle node of a linked list can be efficiently done using the two-pointer technique.
def find_middle(head):
if not head:
return None
slow = fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
return slow
Merging Two Sorted Lists
Merging two sorted linked lists is a common problem that tests your ability to manipulate multiple lists simultaneously.
def merge_sorted_lists(l1, l2):
dummy = ListNode(0)
current = dummy
while l1 and l2:
if l1.val < l2.val:
current.next = l1
l1 = l1.next
else:
current.next = l2
l2 = l2.next
current = current.next
current.next = l1 if l1 else l2
return dummy.next
4. Advanced Techniques for Linked List Problems
As you progress to more challenging linked list problems, you’ll need to employ advanced techniques to solve them efficiently.
Two-Pointer Technique
The two-pointer technique is versatile and can be applied to various linked list problems. It involves using two pointers that move through the list at different speeds or from different starting points.
Example: Remove Nth Node From End of List
def remove_nth_from_end(head, n):
dummy = ListNode(0)
dummy.next = head
first = dummy
second = dummy
for _ in range(n + 1):
first = first.next
while first:
first = first.next
second = second.next
second.next = second.next.next
return dummy.next
Dummy Node Technique
Using a dummy node can simplify operations on the head of the list and help avoid edge cases.
Example: Remove Duplicates from Sorted List
def delete_duplicates(head):
dummy = ListNode(0)
dummy.next = head
current = dummy
while current.next and current.next.next:
if current.next.val == current.next.next.val:
val = current.next.val
while current.next and current.next.val == val:
current.next = current.next.next
else:
current = current.next
return dummy.next
Recursion
Recursive solutions can be elegant for certain linked list problems, though they may not always be the most efficient.
Example: Reverse Linked List (Recursive Approach)
def reverse_list_recursive(head):
if not head or not head.next:
return head
new_head = reverse_list_recursive(head.next)
head.next.next = head
head.next = None
return new_head
Fast and Slow Pointers
This technique is particularly useful for problems involving cycles or finding a specific position in the list.
Example: Find the Start of the Cycle
def detect_cycle(head):
if not head or not head.next:
return None
slow = fast = head
while fast and fast.next:
slow = slow.next
fast = fast.next.next
if slow == fast:
slow = head
while slow != fast:
slow = slow.next
fast = fast.next
return slow
return None
5. Interview Strategies for Linked List Questions
When facing linked list problems in an interview, consider the following strategies:
- Clarify the problem: Ask questions to understand the input, expected output, and any constraints.
- Consider edge cases: Think about empty lists, single-node lists, and other special cases.
- Visualize the problem: Draw the linked list and walk through your approach step by step.
- Think out loud: Explain your thought process as you develop your solution.
- Start with a brute-force approach: If you can’t immediately see an optimal solution, start with a simple approach and then optimize.
- Analyze time and space complexity: Be prepared to discuss the efficiency of your solution.
- Test your code: Walk through your code with a small example to catch any logical errors.
6. Practice Problems and Solutions
To further hone your skills, here are some additional practice problems with their solutions:
Problem 1: Add Two Numbers
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
def add_two_numbers(l1, l2):
dummy = ListNode(0)
current = dummy
carry = 0
while l1 or l2 or carry:
val1 = l1.val if l1 else 0
val2 = l2.val if l2 else 0
total = val1 + val2 + carry
carry = total // 10
current.next = ListNode(total % 10)
current = current.next
l1 = l1.next if l1 else None
l2 = l2.next if l2 else None
return dummy.next
Problem 2: Reorder List
Given a singly linked list L: L0→L1→…→Ln-1→Ln, reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→…
def reorder_list(head):
if not head or not head.next:
return
# Find the middle of the list
slow = fast = head
while fast.next and fast.next.next:
slow = slow.next
fast = fast.next.next
# Reverse the second half of the list
second = slow.next
slow.next = None
prev = None
while second:
next_node = second.next
second.next = prev
prev = second
second = next_node
# Merge the two halves
first = head
second = prev
while second:
next_first = first.next
next_second = second.next
first.next = second
second.next = next_first
first = next_first
second = next_second
Problem 3: Copy List with Random Pointer
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null. Return a deep copy of the list.
class Node:
def __init__(self, x, next=None, random=None):
self.val = int(x)
self.next = next
self.random = random
def copy_random_list(head):
if not head:
return None
# Create new nodes and insert them between original nodes
current = head
while current:
new_node = Node(current.val)
new_node.next = current.next
current.next = new_node
current = new_node.next
# Set random pointers for new nodes
current = head
while current:
if current.random:
current.next.random = current.random.next
current = current.next.next
# Separate the new list from the original list
dummy = Node(0)
new_current, current = dummy, head
while current:
new_current.next = current.next
new_current = new_current.next
current.next = current.next.next
current = current.next
return dummy.next
7. Conclusion
Mastering linked list problems is crucial for success in coding interviews, especially for top tech companies. By understanding the fundamental concepts, practicing common problems, and applying advanced techniques, you can significantly improve your problem-solving skills and confidence when tackling linked list questions.
Remember that the key to success lies in consistent practice and a deep understanding of the underlying principles. As you work through more problems, you’ll start to recognize patterns and develop intuition for efficient solutions.
Keep the following points in mind as you continue your preparation:
- Always consider edge cases and handle them appropriately.
- Practice implementing linked list operations from scratch to build a strong foundation.
- Focus on optimizing your solutions for both time and space complexity.
- Learn to explain your thought process clearly, as communication is a crucial aspect of technical interviews.
- Don’t hesitate to use techniques like the two-pointer method or dummy nodes when appropriate.
- Regularly revisit and review problems to reinforce your understanding.
With dedication and practice, you’ll be well-prepared to tackle even the most challenging linked list problems in your coding interviews. Good luck with your interview preparation!