Mastering LeetCode in Different Languages: A Comprehensive Guide for Technical Interviews
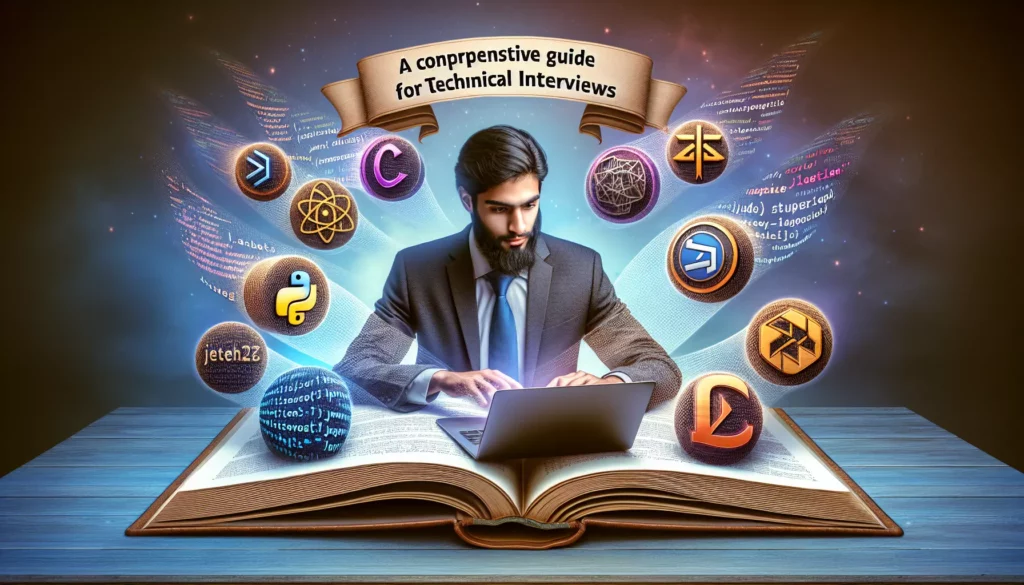
In today’s competitive tech landscape, mastering coding challenges on platforms like LeetCode has become an essential part of preparing for technical interviews, especially when targeting positions at major tech companies. While solving problems is crucial, doing so in multiple programming languages can significantly boost your chances of success. This comprehensive guide will explore the benefits of tackling LeetCode problems in different languages and provide strategies to help you excel in your interview preparation.
Table of Contents
- Why Practice LeetCode in Multiple Languages?
- Choosing the Right Languages
- Strategies for Multi-Language LeetCode Practice
- Language-Specific Tips and Tricks
- Common Pitfalls and How to Avoid Them
- Improving Interview Performance with Multi-Language Skills
- Additional Resources and Tools
- Conclusion
Why Practice LeetCode in Multiple Languages?
Solving LeetCode problems in different programming languages offers numerous advantages for aspiring software engineers:
- Versatility: Being proficient in multiple languages makes you a more versatile candidate, capable of adapting to various tech stacks and projects.
- Deep Understanding: Implementing solutions in different languages reinforces your understanding of core computer science concepts and algorithms.
- Language-Specific Optimizations: Each language has its strengths and weaknesses. Learning to optimize solutions in different languages enhances your problem-solving skills.
- Interview Readiness: Many companies allow candidates to choose their preferred language during interviews. Being comfortable with multiple options gives you an edge.
- Broader Job Opportunities: Proficiency in multiple languages opens up more job opportunities across different tech sectors and companies.
Choosing the Right Languages
When deciding which languages to focus on for LeetCode practice, consider the following factors:
- Industry Demand: Research the most in-demand languages in your target industry or desired companies.
- Personal Interest: Choose languages you enjoy working with to maintain motivation during practice.
- Language Paradigms: Include a mix of object-oriented, functional, and scripting languages for a well-rounded skill set.
- Company Preferences: If targeting specific companies, research their preferred languages and tech stacks.
Some popular language choices for LeetCode practice include:
- Python
- Java
- C++
- JavaScript
- Go
- Ruby
- Swift
Strategies for Multi-Language LeetCode Practice
To effectively practice LeetCode problems in multiple languages, consider the following strategies:
1. Start with a Primary Language
Begin by mastering one language thoroughly. This will help you focus on problem-solving techniques and algorithmic thinking without getting bogged down by syntax differences.
2. Gradual Language Introduction
Once comfortable with your primary language, gradually introduce a second language. Start by solving easier problems you’ve already tackled in your primary language.
3. Parallel Problem Solving
For new problems, try solving them in multiple languages simultaneously. This approach helps highlight the strengths and weaknesses of each language for different problem types.
4. Language Rotation
Implement a language rotation schedule, focusing on a different language each week or month. This ensures consistent practice across all your target languages.
5. Comparative Analysis
After solving a problem in multiple languages, compare your solutions. Analyze the differences in performance, readability, and implementation details.
6. Leverage Language-Specific Features
For each language, learn and utilize its unique features and standard libraries to write more efficient and idiomatic code.
Language-Specific Tips and Tricks
Here are some language-specific tips to help you optimize your LeetCode solutions:
Python
- Utilize list comprehensions for concise array manipulations.
- Take advantage of Python’s powerful built-in functions like
map()
,filter()
, andreduce()
. - Use collections like
defaultdict
andCounter
for efficient data handling.
Example of using list comprehension in Python:
# Generate a list of squares for numbers 1 to 10
squares = [x**2 for x in range(1, 11)]
print(squares)
# Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Java
- Leverage Java’s robust Collections framework for data structure operations.
- Use streams and lambda expressions for functional-style programming.
- Implement custom comparators for efficient sorting of complex objects.
Example of using streams in Java:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenSquares = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.collect(Collectors.toList());
System.out.println(evenSquares);
// Output: [4, 16, 36, 64, 100]
}
}
C++
- Use STL containers and algorithms for efficient data manipulation.
- Implement custom hash functions for unordered containers when needed.
- Utilize C++11 features like auto keyword and range-based for loops for cleaner code.
Example of using STL algorithms in C++:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 8, 1, 9, 3, 7, 4, 6};
// Sort the vector
std::sort(numbers.begin(), numbers.end());
// Find the sum of all elements
int sum = std::accumulate(numbers.begin(), numbers.end(), 0);
std::cout << "Sorted vector: ";
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << "\nSum: " << sum << std::endl;
return 0;
}
// Output:
// Sorted vector: 1 2 3 4 5 6 7 8 9
// Sum: 45
JavaScript
- Make use of ES6+ features like arrow functions, destructuring, and spread operator.
- Leverage functional programming concepts with methods like
map()
,filter()
, andreduce()
. - Use Sets and Maps for efficient data storage and retrieval.
Example of using ES6+ features in JavaScript:
// Using arrow functions, destructuring, and spread operator
const numbers = [1, 2, 3, 4, 5];
const [first, second, ...rest] = numbers;
const doubleAndFilter = (arr) => {
return arr
.map(num => num * 2)
.filter(num => num > 5);
};
console.log(doubleAndFilter(numbers));
// Output: [6, 8, 10]
Common Pitfalls and How to Avoid Them
When practicing LeetCode in multiple languages, be aware of these common pitfalls:
1. Neglecting Language-Specific Best Practices
Pitfall: Writing code that works but doesn’t follow language-specific conventions and best practices.
Solution: Study and apply idiomatic patterns for each language. Read style guides and learn from well-written open-source projects.
2. Overreliance on a Single Language’s Paradigm
Pitfall: Trying to force solutions in one language’s style onto another language where it doesn’t fit well.
Solution: Embrace each language’s unique strengths and paradigms. Adapt your problem-solving approach to fit the language you’re using.
3. Inconsistent Practice Across Languages
Pitfall: Focusing too much on one language while neglecting others.
Solution: Implement a structured practice schedule that ensures equal attention to all target languages.
4. Ignoring Performance Differences
Pitfall: Assuming a solution that performs well in one language will have similar performance in another.
Solution: Study and benchmark the performance characteristics of your solutions in each language. Understand the underlying implementation details that affect performance.
5. Lack of Deep Understanding
Pitfall: Memorizing solutions without truly understanding the underlying concepts.
Solution: Focus on understanding the core algorithms and data structures. Implement solutions from scratch in multiple languages to reinforce your understanding.
Improving Interview Performance with Multi-Language Skills
Mastering LeetCode problems in multiple languages can significantly enhance your performance in technical interviews. Here’s how to leverage your multi-language skills effectively:
1. Language Selection Strategy
Develop a strategy for choosing the most appropriate language for each interview or problem type. Consider factors such as:
- The company’s primary tech stack
- The specific role you’re applying for
- The nature of the problem (e.g., string manipulation, graph algorithms, etc.)
- Your comfort level with each language for live coding
2. Highlight Versatility
During interviews, showcase your ability to think in multiple languages. If appropriate, mention alternative implementations or optimizations possible in other languages.
3. Adapt to Interviewer Preferences
Be prepared to switch languages if the interviewer suggests it. Your flexibility can demonstrate adaptability and breadth of knowledge.
4. Leverage Language-Specific Strengths
For each problem, quickly assess which language might offer the most elegant or efficient solution. Explain your choice to the interviewer, showcasing your analytical skills.
5. Discuss Trade-offs
When solving problems, discuss the trade-offs between implementations in different languages. This demonstrates a deep understanding of language characteristics and system design considerations.
6. Code Translation
Practice translating solutions between languages. This skill can be valuable if an interviewer asks how you would implement a solution in a different language.
Additional Resources and Tools
To support your multi-language LeetCode practice, consider utilizing these resources and tools:
Online Platforms and Courses
- LeetCode: The primary platform for practicing coding problems in multiple languages.
- HackerRank: Offers language-specific tracks and problem-solving challenges.
- Coursera: Provides courses on algorithms and data structures in various programming languages.
- edX: Offers computer science courses from top universities, often in multiple languages.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” (available for Java, Python, and C++)
- “Programming Pearls” by Jon Bentley
Tools
- Repl.it: An online IDE supporting multiple languages, great for quick coding and testing.
- Jupyter Notebooks: Excellent for practicing and documenting your solutions in Python and other languages.
- GitHub: Use repositories to organize and version control your solutions across different languages.
Community and Forums
- r/learnprogramming on Reddit: A supportive community for programmers of all levels.
- Stack Overflow: For language-specific questions and problem-solving discussions.
- Language-specific Discord servers: Join communities dedicated to the languages you’re practicing.
Conclusion
Mastering LeetCode problems in multiple programming languages is a powerful strategy for excelling in technical interviews and building a versatile skill set as a software engineer. By following the strategies outlined in this guide, leveraging language-specific features, and consistently practicing across different languages, you’ll develop a deep understanding of algorithms and data structures that transcends any single programming language.
Remember that the goal is not just to solve problems, but to understand the underlying concepts and be able to apply them flexibly across different languages and scenarios. This approach will not only prepare you for interviews but also make you a more adaptable and valuable software engineer in your future career.
As you embark on your multi-language LeetCode journey, stay curious, embrace the unique characteristics of each language, and enjoy the process of becoming a more well-rounded programmer. With dedication and consistent practice, you’ll be well-prepared to tackle any coding challenge that comes your way in your next technical interview.