Mastering Island Problems: Essential Techniques for Coding Interviews
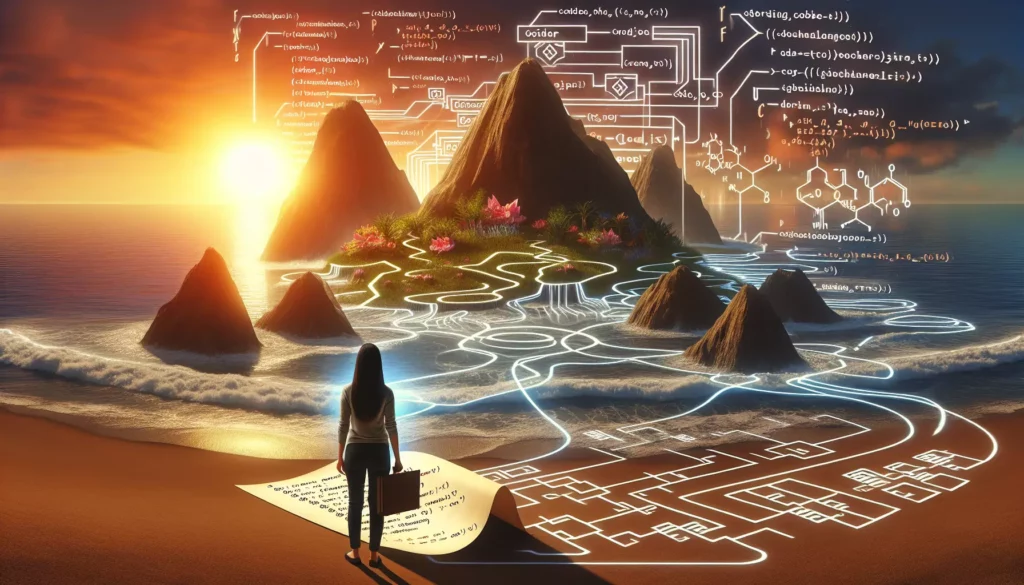
Welcome to AlgoCademy’s comprehensive guide on solving island problems in coding interviews. If you’re preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering island problems is crucial. These problems are a common subset of graph and matrix-based questions that test your ability to navigate 2D spaces and perform connected component analysis. In this article, we’ll dive deep into various techniques and strategies to tackle island problems effectively.
What are Island Problems?
Island problems typically involve a 2D grid or matrix where you need to identify, count, or manipulate connected regions. These regions are often represented by similar values (like 1s) surrounded by different values (like 0s). The term “island” comes from the visual representation of land (1s) surrounded by water (0s).
Common variations of island problems include:
- Counting the number of islands
- Finding the largest island
- Determining if islands are connected
- Transforming islands
Key Techniques for Solving Island Problems
Let’s explore the essential techniques you’ll need to master to solve island problems efficiently:
1. Depth-First Search (DFS)
DFS is a fundamental technique for exploring connected components in a graph or grid. It’s particularly useful for island problems because it allows you to traverse an entire island efficiently.
Here’s a basic implementation of DFS for a grid:
def dfs(grid, i, j):
if i < 0 or i >= len(grid) or j < 0 or j >= len(grid[0]) or grid[i][j] != '1':
return
grid[i][j] = '0' # Mark as visited
# Explore neighbors
dfs(grid, i+1, j)
dfs(grid, i-1, j)
dfs(grid, i, j+1)
dfs(grid, i, j-1)
This DFS function explores an island by recursively visiting all connected land cells and marking them as visited (changing ‘1’ to ‘0’).
2. Breadth-First Search (BFS)
BFS is another crucial technique for exploring islands. It’s particularly useful when you need to find the shortest path or when the problem involves levels or distances.
Here’s a basic BFS implementation for grid traversal:
from collections import deque
def bfs(grid, i, j):
queue = deque([(i, j)])
grid[i][j] = '0' # Mark as visited
while queue:
x, y = queue.popleft()
for dx, dy in [(1,0), (-1,0), (0,1), (0,-1)]:
nx, ny = x + dx, y + dy
if 0 <= nx < len(grid) and 0 <= ny < len(grid[0]) and grid[nx][ny] == '1':
queue.append((nx, ny))
grid[nx][ny] = '0' # Mark as visited
This BFS function uses a queue to explore an island level by level, marking visited cells along the way.
3. Union-Find (Disjoint Set)
The Union-Find data structure is excellent for problems involving connected components, especially when you need to efficiently determine if two cells belong to the same island or merge islands.
Here’s a basic implementation of Union-Find:
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x])
return self.parent[x]
def union(self, x, y):
px, py = self.find(x), self.find(y)
if px == py:
return
if self.rank[px] < self.rank[py]:
self.parent[px] = py
elif self.rank[px] > self.rank[py]:
self.parent[py] = px
else:
self.parent[py] = px
self.rank[px] += 1
This Union-Find implementation uses path compression and union by rank for optimal performance.
4. Matrix Traversal Techniques
Efficient matrix traversal is crucial for island problems. Here are some key techniques:
- Direction arrays: Use arrays to represent the four (or eight) directions for easy neighbor access.
- Boundary checking: Always check if you’re within the grid bounds before accessing a cell.
- In-place marking: Mark visited cells to avoid revisiting them (e.g., changing ‘1’ to ‘0’).
Here’s an example of using direction arrays:
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)] # Right, Down, Left, Up
for dx, dy in directions:
new_x, new_y = x + dx, y + dy
if 0 <= new_x < rows and 0 <= new_y < cols:
# Process the neighbor cell
5. Flood Fill Algorithm
The Flood Fill algorithm is a specific application of DFS or BFS that’s particularly useful for island problems. It’s used to color or mark connected regions in a multi-dimensional array.
Here’s a basic implementation of Flood Fill using DFS:
def flood_fill(image, sr, sc, new_color):
rows, cols = len(image), len(image[0])
color = image[sr][sc]
if color == new_color:
return image
def dfs(r, c):
if image[r][c] == color:
image[r][c] = new_color
if r >= 1: dfs(r-1, c)
if r+1 < rows: dfs(r+1, c)
if c >= 1: dfs(r, c-1)
if c+1 < cols: dfs(r, c+1)
dfs(sr, sc)
return image
This Flood Fill function changes all connected cells of the same color to a new color, starting from a given cell.
Common Island Problems and Solutions
Now that we’ve covered the key techniques, let’s look at some common island problems and how to solve them:
1. Number of Islands
Problem: Given a 2D grid map of ‘1’s (land) and ‘0’s (water), count the number of islands.
Solution:
def numIslands(grid):
if not grid:
return 0
count = 0
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == '1':
dfs(grid, i, j)
count += 1
return count
def dfs(grid, i, j):
if i < 0 or j < 0 or i >= len(grid) or j >= len(grid[0]) or grid[i][j] != '1':
return
grid[i][j] = '0'
dfs(grid, i+1, j)
dfs(grid, i-1, j)
dfs(grid, i, j+1)
dfs(grid, i, j-1)
This solution uses DFS to explore each island and mark it as visited. The outer loop counts the number of times we start a new DFS, which corresponds to the number of islands.
2. Max Area of Island
Problem: Given a 2D grid map of ‘1’s (land) and ‘0’s (water), find the maximum area of an island.
Solution:
def maxAreaOfIsland(grid):
max_area = 0
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == 1:
max_area = max(max_area, dfs(grid, i, j))
return max_area
def dfs(grid, i, j):
if i < 0 or j < 0 or i >= len(grid) or j >= len(grid[0]) or grid[i][j] != 1:
return 0
grid[i][j] = 0
return 1 + dfs(grid, i+1, j) + dfs(grid, i-1, j) + dfs(grid, i, j+1) + dfs(grid, i, j-1)
This solution uses DFS to explore each island and calculate its area. The maximum area is updated as we explore each island.
3. Island Perimeter
Problem: Given a 2D grid map of ‘1’s (land) and ‘0’s (water), return the perimeter of the island.
Solution:
def islandPerimeter(grid):
perimeter = 0
rows, cols = len(grid), len(grid[0])
for i in range(rows):
for j in range(cols):
if grid[i][j] == 1:
perimeter += 4
if i > 0 and grid[i-1][j] == 1:
perimeter -= 2
if j > 0 and grid[i][j-1] == 1:
perimeter -= 2
return perimeter
This solution counts the perimeter by adding 4 for each land cell and subtracting 2 for each adjacent land cell (to avoid double counting).
4. Number of Distinct Islands
Problem: Given a 2D grid map of ‘1’s (land) and ‘0’s (water), count the number of distinct islands.
Solution:
def numDistinctIslands(grid):
def dfs(i, j, direction):
if i < 0 or j < 0 or i >= len(grid) or j >= len(grid[0]) or grid[i][j] != 1:
return
grid[i][j] = 0
path.append(direction)
dfs(i+1, j, 'D')
dfs(i-1, j, 'U')
dfs(i, j+1, 'R')
dfs(i, j-1, 'L')
path.append('0') # Backtrack
distinct_islands = set()
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == 1:
path = []
dfs(i, j, 'S') # Start
distinct_islands.add(''.join(path))
return len(distinct_islands)
This solution uses DFS to explore each island and creates a unique string representation of the island’s shape. We use a set to count distinct shapes.
Advanced Techniques for Island Problems
As you progress in your coding interview preparation, you may encounter more complex island problems. Here are some advanced techniques to tackle them:
1. Dynamic Programming on Islands
Some island problems can be solved more efficiently using dynamic programming. This is particularly useful when you need to calculate properties of islands that depend on their substructures.
Example: Calculating the number of distinct ways to traverse an island.
def uniquePathsOnIsland(grid):
if not grid or not grid[0]:
return 0
m, n = len(grid), len(grid[0])
dp = [[0] * n for _ in range(m)]
# Initialize first row and column
for i in range(m):
if grid[i][0] == 1:
dp[i][0] = 1
else:
break
for j in range(n):
if grid[0][j] == 1:
dp[0][j] = 1
else:
break
# Fill dp table
for i in range(1, m):
for j in range(1, n):
if grid[i][j] == 1:
dp[i][j] = dp[i-1][j] + dp[i][j-1]
return dp[m-1][n-1]
This DP solution calculates the number of unique paths to reach each cell of the island, considering only right and down movements.
2. Multi-Source BFS
When dealing with multiple starting points or when you need to process all islands simultaneously, multi-source BFS can be very effective.
Example: Finding the distance of each water cell to the nearest land.
from collections import deque
def distanceToNearestLand(grid):
m, n = len(grid), len(grid[0])
queue = deque()
# Initialize queue with all land cells
for i in range(m):
for j in range(n):
if grid[i][j] == 1:
queue.append((i, j))
else:
grid[i][j] = float('inf')
directions = [(1,0), (-1,0), (0,1), (0,-1)]
while queue:
x, y = queue.popleft()
for dx, dy in directions:
nx, ny = x + dx, y + dy
if 0 <= nx < m and 0 <= ny < n and grid[nx][ny] > grid[x][y] + 1:
grid[nx][ny] = grid[x][y] + 1
queue.append((nx, ny))
return grid
This multi-source BFS starts from all land cells simultaneously and propagates distances to water cells.
3. Island Compression
For very large grids or when memory is a concern, you can use techniques to compress the island representation. One common approach is run-length encoding.
Example: Compressing an island representation.
def compressIsland(grid):
compressed = []
for row in grid:
count = 0
last = row[0]
encoded_row = []
for cell in row:
if cell == last:
count += 1
else:
encoded_row.append((last, count))
last = cell
count = 1
encoded_row.append((last, count))
compressed.append(encoded_row)
return compressed
# Example usage
grid = [
[1,1,0,0,1],
[1,1,0,0,0],
[0,0,1,1,1],
[0,0,0,1,1]
]
compressed = compressIsland(grid)
print(compressed)
# Output: [[(1, 2), (0, 2), (1, 1)], [(1, 2), (0, 3)], [(0, 2), (1, 3)], [(0, 3), (1, 2)]]
This compression technique can significantly reduce memory usage for large sparse grids.
4. Bit Manipulation for Small Islands
For small islands (up to 32×32), you can use bit manipulation techniques to represent and process the island more efficiently.
Example: Representing a small island using bits.
def islandToBits(grid):
bits = 0
for i, row in enumerate(grid):
for j, cell in enumerate(row):
if cell == 1:
bits |= 1 << (i * len(row) + j)
return bits
# Example usage
grid = [
[1,0,1],
[0,1,0],
[1,0,1]
]
bits = islandToBits(grid)
print(bin(bits)) # Output: 0b100010001
This bit representation allows for fast operations and comparisons between islands.
Common Pitfalls and How to Avoid Them
When solving island problems, there are several common mistakes that interviewees often make. Here’s how to avoid them:
1. Forgetting to Mark Visited Cells
One of the most common mistakes is forgetting to mark cells as visited, leading to infinite loops or incorrect results.
Solution: Always mark cells as visited (e.g., changing ‘1’ to ‘0’) as soon as you process them in your DFS or BFS.
2. Incorrect Boundary Checking
Failing to properly check grid boundaries can lead to index out of range errors.
Solution: Always check if the indices are within the grid bounds before accessing a cell. Use a helper function if necessary:
def is_valid(grid, i, j):
return 0 <= i < len(grid) and 0 <= j < len(grid[0])
3. Inefficient Island Representation
Using inefficient data structures to represent islands can lead to poor performance.
Solution: Choose appropriate data structures. For small grids, a 2D list is fine. For larger grids, consider using sets, dictionaries, or bit manipulation techniques.
4. Overlooking Edge Cases
Failing to consider edge cases like empty grids or single-cell islands can lead to errors.
Solution: Always start with edge cases. Check for empty grids and handle single-cell scenarios explicitly if necessary.
5. Unnecessary Recursion
Overusing recursion can lead to stack overflow errors for large islands.
Solution: Consider using iterative approaches or tail recursion optimization. For very large islands, BFS might be preferable to DFS.
Practice Problems for Island Techniques
To master island problems, practice is key. Here are some problems you can try on coding platforms like LeetCode:
- Number of Islands (LeetCode 200)
- Max Area of Island (LeetCode 695)
- Island Perimeter (LeetCode 463)
- Number of Distinct Islands (LeetCode 694)
- Making A Large Island (LeetCode 827)
- Surrounded Regions (LeetCode 130)
- Pacific Atlantic Water Flow (LeetCode 417)
- Flood Fill (LeetCode 733)
- Count Sub Islands (LeetCode 1905)
- As Far from Land as Possible (LeetCode 1162)
Remember to approach each problem systematically:
- Understand the problem and clarify any ambiguities
- Think about which technique (DFS, BFS, Union-Find, etc.) is most appropriate
- Consider edge cases and potential optimizations
- Implement your solution, starting with a basic working version
- Test your code with various inputs, including edge cases
- Optimize your solution if necessary
Conclusion
Mastering island problems is crucial for success in coding interviews, especially for top tech companies. By understanding and practicing the techniques we’ve covered – DFS, BFS, Union-Find, and advanced methods like DP and bit manipulation – you’ll be well-prepared to tackle a wide range of island-related challenges.
Remember, the key to success is not just knowing the algorithms, but understanding when and how to apply them effectively. As you practice, focus on pattern recognition and problem-solving strategies, not just memorizing solutions.
Keep practicing, stay curious, and don’t hesitate to explore variations and optimizations of these techniques. With dedication and consistent effort, you’ll be well on your way to mastering island problems and acing your coding interviews!
Happy coding, and best of luck with your interview preparation!