Mastering Hard Coding Interview Problems: The Ultimate Strategy Guide
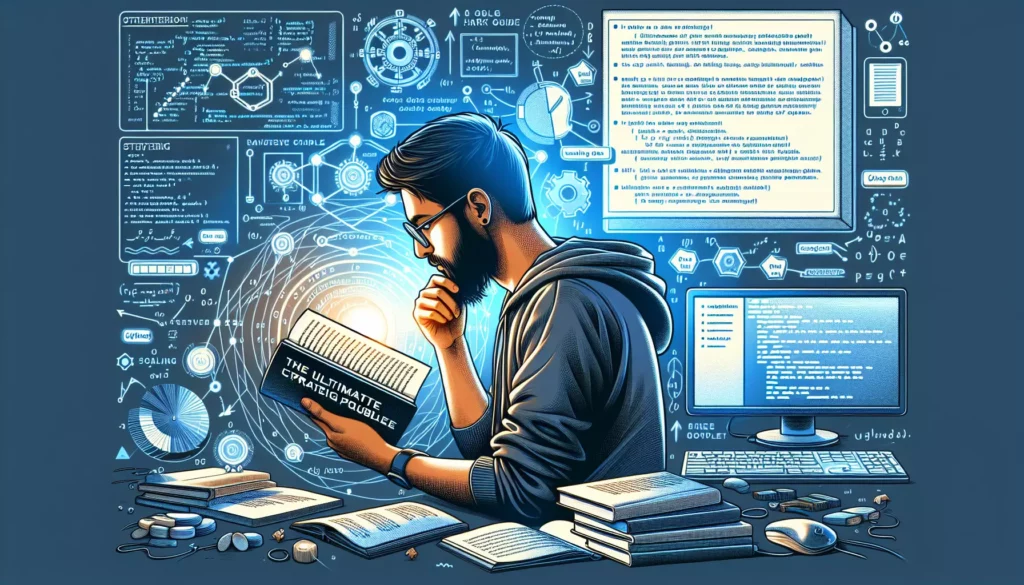
Coding interviews, especially those at top tech companies, can be incredibly challenging. They often involve complex algorithmic problems that require not just coding skills, but also a strategic approach to problem-solving. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, having a solid strategy to tackle hard coding interview problems is crucial. In this comprehensive guide, we’ll explore the best strategies to help you excel in your next coding interview.
1. Build a Strong Foundation
Before diving into advanced problem-solving strategies, it’s essential to have a solid grasp of fundamental computer science concepts and data structures. This foundation will serve as the building blocks for solving more complex problems.
1.1 Master Core Data Structures
Ensure you have a deep understanding of the following data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
1.2 Understand Basic Algorithms
Familiarize yourself with common algorithmic paradigms:
- Sorting algorithms (e.g., Quicksort, Mergesort)
- Searching algorithms (e.g., Binary Search)
- Recursion
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
1.3 Practice Regularly
Consistent practice is key to improving your problem-solving skills. Aim to solve at least one coding problem daily. Platforms like AlgoCademy, LeetCode, HackerRank, and CodeSignal offer a wide range of problems to practice with.
2. Develop a Systematic Approach
When faced with a hard coding interview problem, having a systematic approach can help you navigate the challenge more effectively. Follow these steps:
2.1 Understand the Problem
Take the time to fully comprehend the problem statement. Ask clarifying questions if needed. Key points to consider:
- What are the inputs and outputs?
- Are there any constraints or edge cases?
- Can you identify any patterns or similarities to problems you’ve solved before?
2.2 Analyze and Plan
Before writing any code, think through your approach:
- Break down the problem into smaller, manageable sub-problems
- Consider multiple approaches and their trade-offs
- Think about time and space complexity
- Sketch out a high-level algorithm or flowchart
2.3 Implement the Solution
Once you have a plan, start coding your solution:
- Write clean, readable code
- Use meaningful variable and function names
- Comment your code to explain your thought process
- Start with a brute-force solution if necessary, then optimize
2.4 Test and Debug
After implementation, thoroughly test your solution:
- Use sample inputs provided in the problem statement
- Create your own test cases, including edge cases
- Step through your code mentally or use a debugger
- Optimize your solution if time allows
3. Learn to Recognize Problem Patterns
Many hard coding interview problems fall into specific categories or patterns. Recognizing these patterns can help you quickly identify the most appropriate approach to solve a problem.
3.1 Common Problem Patterns
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a Linked List
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
By familiarizing yourself with these patterns and practicing problems that utilize them, you’ll be better equipped to tackle a wide range of coding challenges.
4. Optimize Your Problem-Solving Skills
Developing strong problem-solving skills is crucial for tackling hard coding interview problems. Here are some techniques to enhance your abilities:
4.1 Think Aloud
Practice verbalizing your thought process as you solve problems. This skill is valuable during interviews, as it allows the interviewer to understand your approach and provide guidance if needed.
4.2 Time Management
Learn to manage your time effectively during interviews. Allocate time for understanding the problem, planning your approach, implementing the solution, and testing. If you’re stuck, know when to move on or ask for hints.
4.3 Learn from Solutions
After solving a problem (or attempting to), study other solutions. This can expose you to different approaches and help you learn new techniques. Platforms like AlgoCademy often provide detailed explanations and multiple solutions for each problem.
4.4 Implement Multiple Solutions
For each problem you solve, try to implement multiple solutions with different time and space complexities. This will help you understand the trade-offs between different approaches and improve your ability to optimize solutions.
5. Utilize Advanced Techniques
As you progress in your preparation, incorporate these advanced techniques to tackle particularly challenging problems:
5.1 Dynamic Programming
Dynamic Programming (DP) is a powerful technique for solving optimization problems. Key steps in applying DP:
- Identify if the problem can be solved using DP
- Define the subproblems
- Find the recurrence relation between subproblems
- Identify the base cases
- Decide on a bottom-up or top-down approach
- Implement the solution, often using memoization or tabulation
5.2 Graph Algorithms
Many hard problems involve graph traversal or manipulation. Familiarize yourself with:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Dijkstra’s Algorithm
- Floyd-Warshall Algorithm
- Union-Find (Disjoint Set)
- Topological Sort
5.3 Bit Manipulation
Bit manipulation can lead to highly optimized solutions for certain problems. Key concepts to understand:
- Bitwise operators (AND, OR, XOR, NOT)
- Bit shifting
- Setting, clearing, and toggling bits
- Checking if a number is a power of 2
- Finding the single number in an array where all other numbers appear twice
5.4 Mathematical Approaches
Some problems require mathematical insights. Brush up on:
- Number theory
- Combinatorics
- Probability
- Geometry
6. Mock Interviews and Real-world Practice
To truly prepare for hard coding interview problems, simulating the actual interview environment is crucial.
6.1 Conduct Mock Interviews
Practice with a friend or use platforms that offer mock interview services. This helps you:
- Get comfortable explaining your thought process
- Practice coding under time pressure
- Receive feedback on your performance
- Identify areas for improvement
6.2 Participate in Coding Competitions
Engage in coding competitions or hackathons to:
- Solve problems under time constraints
- Expose yourself to a variety of problem types
- Learn from other participants’ solutions
- Improve your speed and accuracy
6.3 Contribute to Open Source Projects
Contributing to open source projects can help you:
- Gain experience working with large codebases
- Improve your ability to read and understand others’ code
- Practice writing clean, maintainable code
- Collaborate with other developers
7. Develop a Growth Mindset
Tackling hard coding interview problems requires more than just technical skills. Cultivating a growth mindset is essential for continuous improvement and resilience in the face of challenges.
7.1 Embrace Challenges
View difficult problems as opportunities to learn and grow, rather than insurmountable obstacles.
7.2 Learn from Failures
When you struggle with a problem, analyze what went wrong and how you can improve for next time.
7.3 Seek Feedback
Actively seek feedback on your solutions and approach from peers, mentors, or online communities.
7.4 Stay Curious
Continuously explore new algorithms, data structures, and problem-solving techniques to expand your knowledge.
8. Leverage AI-Powered Tools
In the age of artificial intelligence, leveraging AI-powered tools can significantly enhance your preparation for coding interviews. Platforms like AlgoCademy offer AI-assisted features that can provide personalized guidance and support.
8.1 AI-Powered Code Analysis
Use AI tools to analyze your code for:
- Efficiency and optimization opportunities
- Potential bugs or edge cases
- Adherence to best practices and coding standards
8.2 Personalized Learning Paths
AI can help create customized learning paths based on your strengths and weaknesses, ensuring you focus on areas that need the most improvement.
8.3 Intelligent Problem Recommendations
AI algorithms can suggest problems that are most relevant to your skill level and learning goals, helping you progress more efficiently.
8.4 Real-time Hints and Explanations
When stuck on a problem, AI-powered hints can provide just enough guidance to help you move forward without giving away the entire solution.
9. Time Complexity and Space Complexity Analysis
Understanding and optimizing the time and space complexity of your solutions is crucial for tackling hard coding interview problems effectively.
9.1 Big O Notation
Familiarize yourself with Big O notation and be able to analyze the time and space complexity of your algorithms. Common complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
9.2 Optimize for Time
Learn techniques to optimize your algorithms for better time complexity:
- Use appropriate data structures (e.g., hash tables for O(1) lookup)
- Implement efficient sorting and searching algorithms
- Avoid unnecessary nested loops when possible
- Use memoization or dynamic programming to avoid redundant calculations
9.3 Optimize for Space
Consider ways to reduce the space complexity of your solutions:
- Use in-place algorithms when possible
- Release memory that’s no longer needed
- Consider using bit manipulation to compress data
- Use generators or iterators for large datasets
9.4 Analyze Trade-offs
Understand the trade-offs between time and space complexity. Sometimes, you may need to sacrifice one for the other. Be prepared to explain your choices in an interview setting.
10. Continuous Learning and Improvement
The field of computer science and software engineering is constantly evolving. To stay competitive and excel in coding interviews, it’s essential to adopt a mindset of continuous learning and improvement.
10.1 Stay Updated with New Technologies
Keep abreast of new programming languages, frameworks, and tools that are relevant to your field. While coding interviews often focus on fundamental concepts, showing awareness of current trends can be beneficial.
10.2 Read Technical Books and Papers
Dive deeper into computer science concepts by reading books like:
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “The Algorithm Design Manual” by Steven S. Skiena
10.3 Engage in Coding Communities
Participate in online forums, coding communities, and local meetups to:
- Discuss complex problems and solutions
- Learn from others’ experiences
- Stay motivated and accountable
- Network with other professionals in the field
10.4 Reflect on Your Progress
Regularly assess your progress and adjust your study plan accordingly:
- Keep a log of problems you’ve solved and concepts you’ve learned
- Revisit challenging problems after some time to see if your approach has improved
- Set specific, measurable goals for your interview preparation
- Celebrate your achievements and learn from your setbacks
Conclusion
Mastering hard coding interview problems is a challenging but rewarding journey. By building a strong foundation, developing a systematic approach, recognizing problem patterns, and continuously improving your skills, you can significantly increase your chances of success in technical interviews.
Remember that the process of preparing for these interviews is as valuable as the outcome. The problem-solving skills, algorithmic thinking, and coding practices you develop will serve you well throughout your career in software development.
Platforms like AlgoCademy offer comprehensive resources, AI-powered assistance, and a structured learning path to help you on this journey. Utilize these tools, practice consistently, and approach each problem as an opportunity to learn and grow. With dedication and the right strategies, you can confidently tackle even the most challenging coding interview problems and take a significant step towards your dream job in the tech industry.
Happy coding, and best of luck in your interviews!