Mastering Graph Cycle Problems: Essential Techniques for Coding Interviews
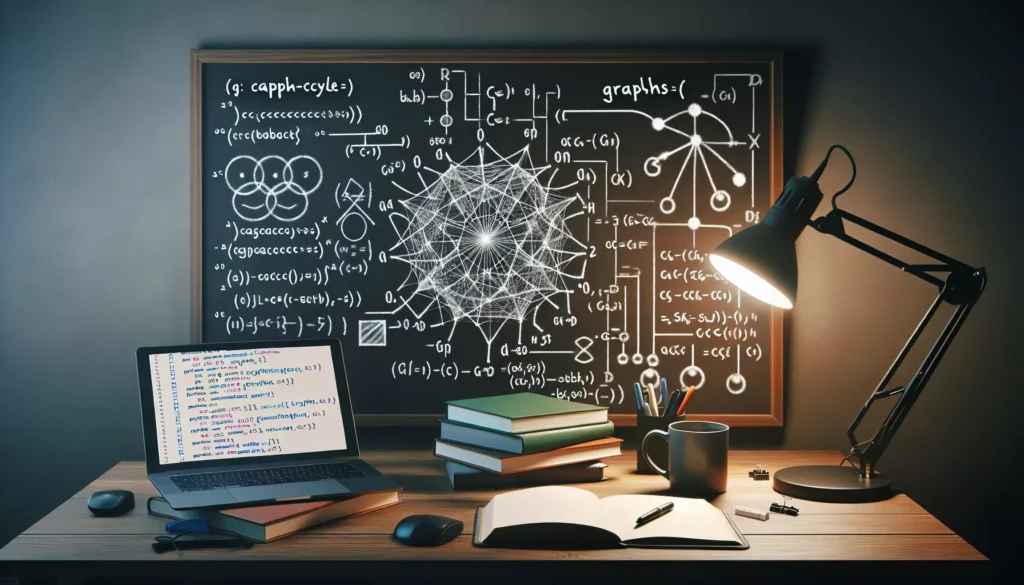
Welcome to another in-depth tutorial from AlgoCademy! Today, we’re diving into an essential topic for coding interviews and algorithmic problem-solving: techniques for solving graph cycle problems. Whether you’re preparing for a technical interview at a FAANG company or simply looking to enhance your programming skills, understanding how to detect and manipulate cycles in graphs is crucial.
Graphs are versatile data structures that can represent a wide variety of real-world scenarios, from social networks to computer networks, from road systems to dependency relationships in software. Cycles in graphs often represent important patterns or issues that need to be identified and addressed. In this comprehensive guide, we’ll explore various techniques to tackle graph cycle problems, providing you with the tools you need to excel in your coding interviews and beyond.
Table of Contents
- Introduction to Graph Cycles
- Depth-First Search (DFS) for Cycle Detection
- Breadth-First Search (BFS) for Cycle Detection
- Union-Find (Disjoint Set) Method
- Topological Sort for Cycle Detection in Directed Graphs
- Floyd’s Cycle-Finding Algorithm
- Graph Coloring Method
- Advanced Techniques and Optimizations
- Practice Problems and Solutions
- Conclusion and Next Steps
1. Introduction to Graph Cycles
Before we dive into the techniques, let’s establish a solid understanding of what graph cycles are and why they’re important.
What is a Graph Cycle?
A cycle in a graph is a path that starts and ends at the same vertex, without repeating any edges. In other words, it’s a closed loop in the graph structure. Cycles can occur in both directed and undirected graphs, though their properties and detection methods may differ.
Why are Graph Cycles Important?
Detecting and analyzing cycles in graphs is crucial for various reasons:
- In dependency graphs, cycles can indicate circular dependencies, which are often problematic in software design.
- In network topologies, cycles provide alternative routes, which can be important for reliability and load balancing.
- In scheduling problems, cycles may represent deadlock situations or impossible schedules.
- In social network analysis, cycles can reveal interesting relationship patterns.
Now that we understand the importance of graph cycles, let’s explore the various techniques to detect and work with them.
2. Depth-First Search (DFS) for Cycle Detection
Depth-First Search is one of the most fundamental and versatile algorithms for graph traversal and cycle detection. It’s particularly effective for detecting cycles in both directed and undirected graphs.
How DFS Works for Cycle Detection
The basic idea behind using DFS for cycle detection is to keep track of vertices currently in the recursion stack. If we encounter a vertex that is already in the recursion stack, we have found a cycle.
Implementation for Undirected Graphs
Here’s a Python implementation for detecting cycles in an undirected graph using DFS:
def has_cycle(graph):
visited = set()
def dfs(vertex, parent):
visited.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
if dfs(neighbor, vertex):
return True
elif neighbor != parent:
return True
return False
for vertex in graph:
if vertex not in visited:
if dfs(vertex, None):
return True
return False
# Example usage
graph = {
0: [1, 2],
1: [0, 2],
2: [0, 1, 3],
3: [2]
}
print(has_cycle(graph)) # Output: True
Implementation for Directed Graphs
For directed graphs, we need to modify our approach slightly. Instead of just keeping track of visited nodes, we also need to track nodes in the current recursion stack:
def has_cycle_directed(graph):
visited = set()
rec_stack = set()
def dfs(vertex):
visited.add(vertex)
rec_stack.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
if dfs(neighbor):
return True
elif neighbor in rec_stack:
return True
rec_stack.remove(vertex)
return False
for vertex in graph:
if vertex not in visited:
if dfs(vertex):
return True
return False
# Example usage
directed_graph = {
0: [1, 2],
1: [2],
2: [3],
3: [1]
}
print(has_cycle_directed(directed_graph)) # Output: True
Time and Space Complexity
The time complexity of DFS-based cycle detection is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because we visit each vertex and edge once. The space complexity is O(V) to store the visited set and the recursion stack.
3. Breadth-First Search (BFS) for Cycle Detection
While Depth-First Search is often the go-to method for cycle detection, Breadth-First Search can also be used, particularly in scenarios where you want to find the shortest cycle or when working with very large graphs where the recursion depth of DFS might be a concern.
BFS Approach for Cycle Detection
The BFS approach for cycle detection involves keeping track of the parent of each node as we traverse the graph. If we encounter a node that has already been visited and it’s not the parent of the current node, we’ve found a cycle.
Implementation for Undirected Graphs
Here’s a Python implementation using BFS to detect cycles in an undirected graph:
from collections import deque
def has_cycle_bfs(graph):
visited = set()
for start_vertex in graph:
if start_vertex not in visited:
queue = deque([(start_vertex, None)])
while queue:
vertex, parent = queue.popleft()
visited.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
queue.append((neighbor, vertex))
elif neighbor != parent:
return True
return False
# Example usage
graph = {
0: [1, 2],
1: [0, 2],
2: [0, 1, 3],
3: [2]
}
print(has_cycle_bfs(graph)) # Output: True
Advantages and Disadvantages of BFS
Advantages of using BFS for cycle detection:
- It can find the shortest cycle in an unweighted graph.
- It’s useful for graphs where DFS might exceed the maximum recursion depth.
- It can be more intuitive for some problems, especially those involving shortest paths.
Disadvantages:
- It typically uses more memory than DFS due to the queue.
- It may be less efficient for sparse graphs.
Time and Space Complexity
The time complexity of BFS-based cycle detection is O(V + E), the same as DFS. The space complexity is O(V) to store the visited set and the queue.
4. Union-Find (Disjoint Set) Method
The Union-Find data structure, also known as a disjoint set data structure, is particularly useful for detecting cycles in undirected graphs. This method is especially efficient when you’re adding edges to the graph one by one and want to check if each new edge creates a cycle.
How Union-Find Works for Cycle Detection
The basic idea is to maintain disjoint sets of vertices. When we add an edge, we check if the vertices it connects are already in the same set. If they are, adding this edge would create a cycle. If not, we union the sets containing these vertices.
Implementation
Here’s a Python implementation of cycle detection using Union-Find:
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def has_cycle_union_find(n, edges):
uf = UnionFind(n)
for x, y in edges:
if uf.find(x) == uf.find(y):
return True
uf.union(x, y)
return False
# Example usage
n = 4
edges = [(0, 1), (1, 2), (2, 3), (3, 1)]
print(has_cycle_union_find(n, edges)) # Output: True
Advantages of Union-Find
- Very efficient for graphs that are being built incrementally.
- Can handle dynamic graphs where edges are being added or removed.
- With path compression and union by rank, operations are nearly constant time.
Time and Space Complexity
With path compression and union by rank optimizations, the amortized time complexity for each operation (find and union) is nearly O(1). For a graph with V vertices and E edges, the overall time complexity is O(E α(V)), where α(V) is the inverse Ackermann function, which grows very slowly and is effectively constant for all practical values of V. The space complexity is O(V) to store the parent and rank arrays.
5. Topological Sort for Cycle Detection in Directed Graphs
Topological sorting is an algorithm for ordering the vertices of a directed acyclic graph (DAG) such that for every directed edge (u, v), vertex u comes before v in the ordering. This property makes topological sort particularly useful for detecting cycles in directed graphs.
How Topological Sort Detects Cycles
The key insight is that a directed graph has a valid topological ordering if and only if it is acyclic. Therefore, if we attempt to perform a topological sort and find that it’s not possible to complete it, we know the graph contains a cycle.
Implementation Using Kahn’s Algorithm
Here’s an implementation of cycle detection using Kahn’s algorithm for topological sorting:
from collections import deque
def has_cycle_topological_sort(graph):
in_degree = {node: 0 for node in graph}
for node in graph:
for neighbor in graph[node]:
in_degree[neighbor] += 1
queue = deque([node for node in graph if in_degree[node] == 0])
visited_count = 0
while queue:
node = queue.popleft()
visited_count += 1
for neighbor in graph[node]:
in_degree[neighbor] -= 1
if in_degree[neighbor] == 0:
queue.append(neighbor)
return visited_count != len(graph)
# Example usage
graph = {
0: [1, 2],
1: [2],
2: [3],
3: [1]
}
print(has_cycle_topological_sort(graph)) # Output: True
Advantages of Topological Sort
- It can detect cycles in directed graphs efficiently.
- If there’s no cycle, it provides a valid topological ordering of the graph, which can be useful for dependency resolution and scheduling problems.
- It’s particularly useful when you need to know not just if there’s a cycle, but also a valid ordering if there isn’t one.
Time and Space Complexity
The time complexity of Kahn’s algorithm is O(V + E), where V is the number of vertices and E is the number of edges. We process each vertex and edge once. The space complexity is O(V) to store the in-degree map and the queue.
6. Floyd’s Cycle-Finding Algorithm
Floyd’s Cycle-Finding Algorithm, also known as the “tortoise and hare” algorithm, is a pointer algorithm that uses only two pointers, moving through the sequence at different speeds. While it’s most commonly used for detecting cycles in linked lists, it can also be applied to certain types of graphs, particularly those that can be represented as a sequence.
How Floyd’s Algorithm Works
The algorithm uses two pointers, often called the “tortoise” and the “hare”. The tortoise moves one step at a time, while the hare moves two steps. If there’s a cycle, the hare will eventually catch up to the tortoise inside the cycle.
Implementation for a Graph Represented as a Sequence
Here’s an implementation of Floyd’s algorithm for a graph represented as a sequence where each index points to the next node:
def has_cycle_floyd(graph):
tortoise = hare = 0
while True:
tortoise = graph[tortoise]
hare = graph[graph[hare]]
if tortoise == hare:
return True
if hare == len(graph) - 1:
return False
# Example usage
graph = [1, 2, 3, 4, 2] # Node 4 points back to node 2, creating a cycle
print(has_cycle_floyd(graph)) # Output: True
Advantages of Floyd’s Algorithm
- It uses constant extra space, making it very memory-efficient.
- It’s simple to implement and understand.
- It can detect cycles in infinite sequences without getting stuck.
Limitations
Floyd’s algorithm is primarily useful for graphs that can be represented as a sequence where each node has a single “next” node. It’s not directly applicable to general graphs with multiple edges per node.
Time and Space Complexity
The time complexity is O(n), where n is the number of nodes in the graph. In the worst case, the hare will go around the cycle twice before meeting the tortoise. The space complexity is O(1) as it only uses two pointers regardless of the input size.
7. Graph Coloring Method
The graph coloring method is another approach to detect cycles in both directed and undirected graphs. This method assigns colors to vertices to keep track of their state during traversal.
How Graph Coloring Works for Cycle Detection
We use three colors:
- White: Unvisited vertices
- Gray: Vertices being visited (in the current DFS path)
- Black: Completely visited vertices
If we encounter a gray vertex during traversal, we’ve found a cycle.
Implementation for Directed Graphs
from enum import Enum
class Color(Enum):
WHITE = 0
GRAY = 1
BLACK = 2
def has_cycle_coloring(graph):
colors = {node: Color.WHITE for node in graph}
def dfs(node):
colors[node] = Color.GRAY
for neighbor in graph[node]:
if colors[neighbor] == Color.GRAY:
return True
if colors[neighbor] == Color.WHITE and dfs(neighbor):
return True
colors[node] = Color.BLACK
return False
for node in graph:
if colors[node] == Color.WHITE:
if dfs(node):
return True
return False
# Example usage
graph = {
0: [1, 2],
1: [2],
2: [3],
3: [1]
}
print(has_cycle_coloring(graph)) # Output: True
Advantages of Graph Coloring
- It provides a clear visual understanding of the graph traversal process.
- It can be easily extended to solve more complex graph problems.
- It works well for both directed and undirected graphs with minimal modifications.
Time and Space Complexity
The time complexity is O(V + E), where V is the number of vertices and E is the number of edges, as we visit each vertex and edge once. The space complexity is O(V) to store the color of each vertex.
8. Advanced Techniques and Optimizations
As you become more proficient with graph cycle problems, you’ll encounter scenarios that require more advanced techniques or optimizations. Here are a few to consider:
Tarjan’s Strongly Connected Components Algorithm
Tarjan’s algorithm can be used to find all strongly connected components in a directed graph. A directed graph with no strongly connected components of size greater than one is acyclic. This algorithm can be particularly useful when you need to identify all cycles in a directed graph.
Parallel and Distributed Algorithms
For very large graphs, parallel or distributed algorithms for cycle detection can be employed. These algorithms distribute the work across multiple processors or machines, allowing for faster processing of massive graphs.
Incremental Cycle Detection
In scenarios where the graph is dynamically changing (edges being added or removed), incremental algorithms can be more efficient than recomputing from scratch each time.
Memory-Efficient Techniques
For graphs that are too large to fit entirely in memory, external memory algorithms or streaming algorithms can be used to detect cycles while minimizing memory usage.
9. Practice Problems and Solutions
To reinforce your understanding of graph cycle detection techniques, here are some practice problems along with their solutions:
Problem 1: Detect Cycle in a Directed Graph
Given a directed graph, determine if it contains a cycle.
Solution:
def has_cycle_directed(graph):
visited = set()
rec_stack = set()
def dfs(node):
visited.add(node)
rec_stack.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
if dfs(neighbor):
return True
elif neighbor in rec_stack:
return True
rec_stack.remove(node)
return False
for node in graph:
if node not in visited:
if dfs(node):
return True
return False
# Test the function
graph = {
0: [1, 2],
1: [2],
2: [3],
3: [1]
}
print(has_cycle_directed(graph)) # Output: True
Problem 2: Course Schedule
There are a total of n courses you have to take, labeled from 0 to n-1. Some courses may have prerequisites. For example, to take course 0 you have to first take course 1, which is expressed as a pair: [0,1]. Given the total number of courses and a list of prerequisite pairs, is it possible for you to finish all courses?
Solution:
from collections import defaultdict
def can_finish(num_courses, prerequisites):
graph = defaultdict(list)
for course, prereq in prerequisites:
graph[course].append(prereq)
visited = set()
rec_stack = set()
def has_cycle(course):
visited.add(course)
rec_stack.add(course)
for prereq in graph[course]:
if prereq not in visited:
if has_cycle(prereq):
return True
elif prereq in rec_stack:
return True
rec_stack.remove(course)
return False
for course in range(num_courses):
if course not in visited:
if has_cycle(course):
return False
return True
# Test the function
num_courses = 4
prerequisites = [[1,0],[2,1],[3,2],[1,3]]
print(can_finish(num_courses, prerequisites)) # Output: False
10. Conclusion and Next Steps
Congratulations! You’ve now explored a variety of techniques for solving graph cycle problems. From basic DFS and BFS approaches to more advanced methods like Union-Find and Topological Sort, you have a robust toolkit for tackling cycle-related challenges in your coding interviews and real-world applications.
Remember, the key to mastering these techniques is practice. Here are some next steps to further enhance your skills:
- Implement each of these algorithms from scratch without referring to the examples.
- Solve more complex graph problems on coding platforms like LeetCode, HackerRank, or CodeForces.
- Study the time and space complexity trade-offs of different approaches and practice optimizing your solutions.
- Explore how these cycle detection techniques can be applied to real-world problems in areas like network analysis, dependency management, or scheduling systems.
- Dive deeper into advanced graph algorithms and their applications.
Keep coding, keep learning, and remember that every challenging problem you solve brings you one step closer to your goals in software development and algorithmic mastery. Happy coding!