Mastering Functional Reactive Programming (FRP): A Comprehensive Guide for Modern Developers
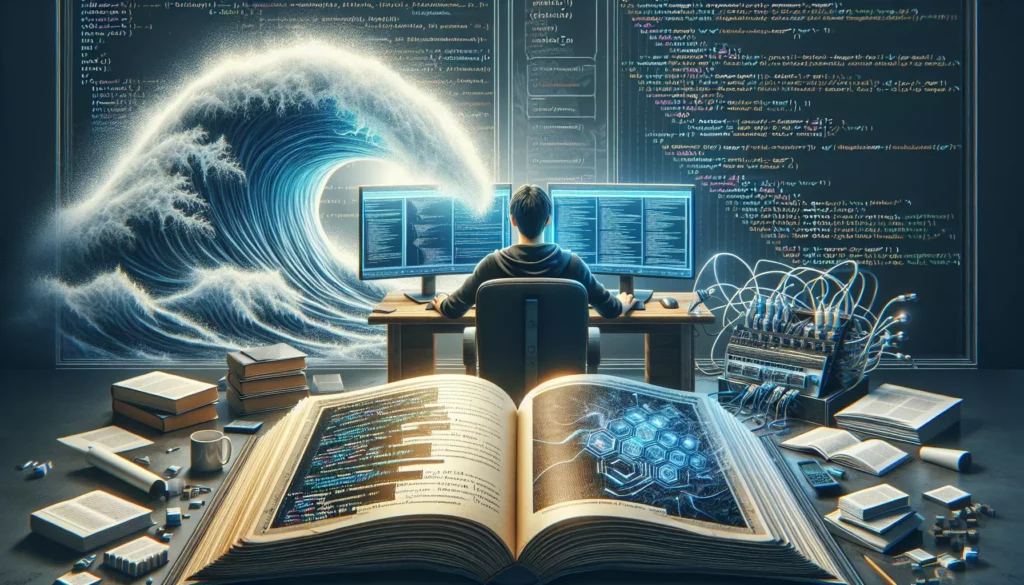
In the ever-evolving landscape of software development, staying ahead of the curve is crucial for both aspiring and seasoned programmers. One paradigm that has gained significant traction in recent years is Functional Reactive Programming (FRP). This powerful approach combines the principles of functional programming with reactive programming, resulting in a robust framework for building responsive and scalable systems. In this comprehensive guide, we’ll dive deep into the world of FRP, exploring its core concepts, benefits, and practical applications.
What is Functional Reactive Programming?
Functional Reactive Programming is a programming paradigm that merges two distinct concepts: functional programming and reactive programming. To truly understand FRP, let’s first break down these individual components:
Functional Programming
Functional programming is a declarative programming paradigm that treats computation as the evaluation of mathematical functions. It emphasizes immutability, pure functions, and the avoidance of side effects. Key principles of functional programming include:
- Immutability: Data cannot be changed once created
- Pure functions: Functions always produce the same output for a given input
- Higher-order functions: Functions can be passed as arguments or returned as values
- Recursion: Solving problems through self-referential functions
- Lazy evaluation: Delaying the evaluation of expressions until their results are needed
Reactive Programming
Reactive programming is a declarative programming paradigm concerned with data streams and the propagation of change. It focuses on building systems that respond to events and data changes in real-time. Key concepts in reactive programming include:
- Observables: Streams of data that can be observed over time
- Operators: Functions that transform, filter, or combine observables
- Subscribers: Components that react to changes in observables
- Asynchronous programming: Handling non-blocking operations efficiently
- Backpressure: Managing the flow of data between fast producers and slow consumers
The Synergy of Functional and Reactive Programming
Functional Reactive Programming combines these two paradigms to create a powerful approach for building responsive systems. FRP leverages the principles of functional programming to work with reactive streams of data, resulting in code that is both declarative and responsive to change.
In FRP, we model our program as a series of time-varying values (often called signals or behaviors) and event streams. These can be combined, transformed, and reacted to using functional programming techniques, creating a system that elegantly handles asynchronous data flows and user interactions.
Core Concepts of Functional Reactive Programming
To truly grasp FRP, it’s essential to understand its fundamental concepts. Let’s explore the key building blocks that make up the FRP paradigm:
1. Signals (Behaviors)
Signals, also known as behaviors in some FRP implementations, represent values that change over time. They can be thought of as continuous streams of data that always have a current value. For example, a signal could represent the current mouse position, the elapsed time since the program started, or the current value of a text input field.
In code, a signal might look something like this:
// Pseudo-code representation of a signal
let mouseX = Signal.from(mouseMove, event => event.clientX)
2. Events
Events are discrete occurrences that happen at specific points in time. Unlike signals, events don’t have a continuous value but instead represent moments when something interesting happens. Examples of events include button clicks, key presses, or network responses.
Here’s how an event might be represented:
// Pseudo-code representation of an event
let buttonClicks = Event.from(button, "click")
3. Observables
Observables are a more general concept that can represent both signals and events. They are streams of data that can be observed over time. Observables are a key component in many FRP implementations and libraries, such as RxJS.
// Example using RxJS
import { fromEvent } from "rxjs"
const buttonClicks$ = fromEvent(button, "click")
4. Operators
Operators are functions that transform, combine, or filter streams of data. They allow you to manipulate signals, events, or observables in various ways. Common operators include map, filter, merge, and combine.
// Example using RxJS
import { fromEvent } from "rxjs"
import { map, filter } from "rxjs/operators"
const evenClicks$ = fromEvent(button, "click").pipe(
map((event, index) => index),
filter(index => index % 2 === 0)
)
5. Subscriptions
Subscriptions represent the act of listening to a stream and reacting to its values. When you subscribe to an observable, you provide a function that will be called whenever a new value is emitted.
// Example using RxJS
evenClicks$.subscribe(index => {
console.log(`Even click number ${index}`)
})
Benefits of Functional Reactive Programming
Now that we’ve covered the core concepts, let’s explore why FRP has gained popularity and the benefits it offers to developers:
1. Declarative Code
FRP allows you to express what you want to achieve rather than how to achieve it. This declarative approach leads to code that is easier to read, understand, and maintain. Instead of imperatively describing each step of a process, you define relationships between data streams and how they should be transformed.
2. Improved Handling of Asynchronous Operations
FRP provides a unified way to handle asynchronous operations, whether they’re user interactions, network requests, or time-based events. This consistency simplifies complex asynchronous workflows and helps avoid callback hell.
3. Better State Management
By modeling your application state as streams of data, FRP can lead to more predictable and manageable state changes. This is particularly beneficial in complex user interfaces where multiple components need to react to state changes.
4. Composability
FRP’s functional nature allows for high composability. Complex behaviors can be built by combining simpler ones, leading to more modular and reusable code.
5. Testability
Pure functions and immutable data, core principles of functional programming, make FRP code easier to test. You can test individual transformations and combinations of streams without worrying about hidden side effects.
6. Scalability
FRP’s approach to handling data streams and asynchronous operations makes it well-suited for building scalable applications, especially those dealing with real-time data or complex user interactions.
Practical Applications of Functional Reactive Programming
FRP isn’t just a theoretical concept; it has practical applications across various domains of software development. Let’s explore some areas where FRP shines:
1. User Interface Development
FRP is particularly well-suited for building reactive user interfaces. Libraries like React combined with RxJS or frameworks like Cycle.js leverage FRP principles to create responsive and interactive UIs.
Example: Implementing a real-time search feature
import { fromEvent } from "rxjs"
import { debounceTime, map, switchMap } from "rxjs/operators"
const searchInput = document.getElementById("search-input")
const searchResults = document.getElementById("search-results")
const search$ = fromEvent(searchInput, "input").pipe(
debounceTime(300),
map(event => event.target.value),
switchMap(query => fetch(`https://api.example.com/search?q=${query}`).then(res => res.json()))
)
search$.subscribe(results => {
searchResults.innerHTML = results.map(result => `<li>${result.title}</li>`).join("")
})
In this example, we create a reactive search feature that debounces user input, fetches search results from an API, and updates the UI accordingly.
2. Game Development
FRP can be used to model game state and handle user inputs in a declarative way. This approach can lead to more maintainable game logic, especially for complex game mechanics.
Example: Simple game loop using FRP principles
import { interval } from "rxjs"
import { scan } from "rxjs/operators"
const initialGameState = { score: 0, playerPosition: { x: 0, y: 0 } }
const gameLoop$ = interval(16).pipe(
scan((state, _) => ({
...state,
score: state.score + 1,
playerPosition: {
x: state.playerPosition.x + 1,
y: state.playerPosition.y + 1
}
}), initialGameState)
)
gameLoop$.subscribe(state => {
console.log(`Score: ${state.score}, Player Position: (${state.playerPosition.x}, ${state.playerPosition.y})`)
// Update game rendering here
})
This example demonstrates a simple game loop that updates the game state (score and player position) every 16ms (approximately 60 FPS).
3. Data Visualization
FRP is excellent for creating dynamic and interactive data visualizations. It allows for seamless updates of charts and graphs as data changes over time.
Example: Updating a chart with real-time data
import { interval } from "rxjs"
import { map } from "rxjs/operators"
const data$ = interval(1000).pipe(
map(() => Math.random() * 100)
)
data$.subscribe(value => {
// Assuming we're using a charting library like Chart.js
chart.data.datasets[0].data.push(value)
chart.data.labels.push(new Date().toLocaleTimeString())
chart.update()
})
This example shows how to use FRP to update a chart with new data points every second.
4. Robotics and IoT
FRP can be used to handle streams of sensor data and control signals in robotics and Internet of Things (IoT) applications. It provides a natural way to process and react to real-time data from multiple sources.
Example: Processing sensor data in an IoT application
import { merge } from "rxjs"
import { map, filter } from "rxjs/operators"
const temperatureSensor$ = // ... stream of temperature readings
const humiditySensor$ = // ... stream of humidity readings
const environmentalAlert$ = merge(
temperatureSensor$.pipe(
map(temp => ({ type: "temperature", value: temp })),
filter(reading => reading.value > 30)
),
humiditySensor$.pipe(
map(humidity => ({ type: "humidity", value: humidity })),
filter(reading => reading.value > 60)
)
)
environmentalAlert$.subscribe(alert => {
console.log(`Alert: ${alert.type} is too high (${alert.value})`)
// Trigger appropriate action (e.g., activate cooling system, dehumidifier)
})
This example demonstrates how FRP can be used to process and react to sensor data in an IoT scenario.
Challenges and Considerations
While Functional Reactive Programming offers many benefits, it’s important to be aware of potential challenges and considerations:
1. Learning Curve
FRP introduces new concepts and ways of thinking about programming that may take time to master. Developers accustomed to imperative programming paradigms might find the initial learning curve steep.
2. Debugging Complexity
Debugging FRP code can be challenging, especially when dealing with complex stream combinations. Tools and techniques for debugging reactive streams are still evolving.
3. Performance Considerations
While FRP can lead to efficient code, improper use of operators or excessive stream creation can impact performance. It’s important to understand the performance characteristics of different FRP operations.
4. Overuse
Not every problem is best solved with FRP. It’s important to use FRP where it provides clear benefits and not force it into scenarios where simpler approaches would suffice.
5. Library and Framework Choices
There are various libraries and frameworks that implement FRP concepts, each with its own API and set of features. Choosing the right tool for your project requires careful consideration.
Getting Started with Functional Reactive Programming
If you’re interested in exploring FRP further, here are some steps to get started:
1. Learn the Fundamentals
Start by thoroughly understanding the core concepts of functional programming and reactive programming. Resources like “Functional Programming in JavaScript” by Luis Atencio and “Reactive Programming with RxJS” by Sergi Mansilla are excellent starting points.
2. Choose a Library or Framework
Popular choices for FRP include:
- RxJS: A widely used reactive programming library for JavaScript
- Bacon.js: A smaller, more focused FRP library
- Cycle.js: A framework for building fully reactive web applications
- React with RxJS: Combining React’s component model with RxJS for reactivity
3. Practice with Small Projects
Start with small projects to apply FRP concepts. Good beginner projects include:
- A real-time search feature
- A simple game (e.g., Pong or Snake)
- A data visualization dashboard
- A form with complex validation logic
4. Explore Advanced Topics
As you become more comfortable with FRP, explore advanced topics such as:
- Hot vs Cold Observables
- Backpressure handling
- Testing reactive code
- Integrating FRP with existing codebases
5. Join the Community
Engage with the FRP community through forums, meetups, and conferences. Platforms like Stack Overflow, Reddit’s r/functionalprogramming, and GitHub discussions are great places to ask questions and share knowledge.
Conclusion
Functional Reactive Programming represents a powerful paradigm shift in how we approach software development, particularly for systems that deal with asynchronous data flows and complex user interactions. By combining the principles of functional programming with reactive programming, FRP offers a declarative and composable way to build responsive and scalable applications.
While it comes with its own set of challenges, the benefits of FRP – including improved code readability, better handling of asynchronous operations, and enhanced state management – make it a valuable tool in a modern developer’s toolkit.
As you embark on your journey into Functional Reactive Programming, remember that mastery comes with practice and patience. Start small, build your understanding incrementally, and don’t hesitate to experiment. With time and experience, you’ll find that FRP can significantly enhance your ability to create robust, responsive, and maintainable software systems.
Whether you’re building interactive user interfaces, processing real-time data streams, or developing complex event-driven systems, Functional Reactive Programming offers a powerful paradigm that can elevate your programming skills and the quality of your software. Embrace the reactive world, and unlock new possibilities in your development journey!