Mastering Fenwick Trees (Binary Indexed Trees) for Efficient Range Queries
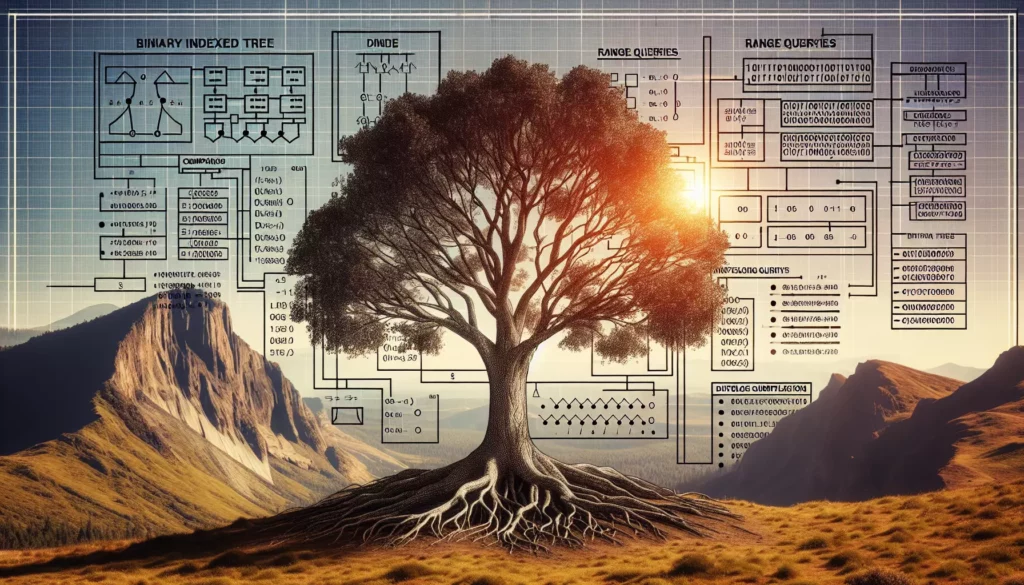
In the world of competitive programming and technical interviews, efficient data structures play a crucial role in solving complex problems. One such powerful yet often underutilized data structure is the Fenwick Tree, also known as the Binary Indexed Tree (BIT). In this comprehensive guide, we’ll dive deep into the concept, implementation, and applications of Fenwick Trees, equipping you with the knowledge to tackle a wide range of coding challenges.
What is a Fenwick Tree?
A Fenwick Tree, named after its inventor Peter Fenwick, is a data structure that provides efficient methods for updating elements and calculating prefix sums in a table of numbers. It was first described in the paper “A New Data Structure for Cumulative Frequency Tables” (1994).
The key features of a Fenwick Tree include:
- Space-efficient storage of cumulative frequency information
- Fast updates to the frequency table
- Quick retrieval of prefix sums
- Time complexity of O(log n) for both update and query operations
These characteristics make Fenwick Trees particularly useful for scenarios where you need to perform frequent updates and range sum queries on an array of numbers.
Understanding the Binary Indexed Tree Concept
To grasp the concept of a Fenwick Tree, it’s essential to understand its binary representation and how it stores cumulative frequencies. The name “Binary Indexed Tree” comes from the way it uses the binary representation of indices to organize and access data.
In a Fenwick Tree:
- Each index i in the tree is responsible for a certain range of elements in the original array.
- The range size is determined by the least significant bit (LSB) of the index.
- The tree structure allows for efficient updates and queries by leveraging these binary properties.
Let’s break down how the Fenwick Tree organizes data:
- Index 1 (binary: 001) is responsible for element 1
- Index 2 (binary: 010) is responsible for elements 1-2
- Index 3 (binary: 011) is responsible for element 3
- Index 4 (binary: 100) is responsible for elements 1-4
- Index 5 (binary: 101) is responsible for element 5
- Index 6 (binary: 110) is responsible for elements 5-6
- Index 7 (binary: 111) is responsible for element 7
- Index 8 (binary: 1000) is responsible for elements 1-8
This organization allows for efficient updates and queries by traversing the tree structure based on the binary representation of indices.
Implementing a Fenwick Tree
Now that we understand the concept, let’s implement a Fenwick Tree in C++. We’ll create a class that supports the two primary operations: update and query.
class FenwickTree {
private:
vector<int> tree;
int n;
public:
FenwickTree(int size) : n(size + 1) {
tree.resize(n, 0);
}
void update(int index, int value) {
index++; // 1-based indexing
while (index < n) {
tree[index] += value;
index += index & (-index); // Add LSB
}
}
int query(int index) {
index++; // 1-based indexing
int sum = 0;
while (index > 0) {
sum += tree[index];
index -= index & (-index); // Remove LSB
}
return sum;
}
};
Let’s break down the key components of this implementation:
Constructor
The constructor initializes the Fenwick Tree with a given size. We add 1 to the size to accommodate 1-based indexing, which simplifies the implementation of update and query operations.
Update Operation
The update function adds a value to an element at a specific index and updates all the necessary ranges in the tree. It uses the following steps:
- Increment the index to convert to 1-based indexing.
- Add the value to the current node.
- Move to the next responsible node by adding the least significant bit (LSB) to the index.
- Repeat steps 2-3 until we reach the end of the tree.
The line index += index & (-index);
is a clever way to add the LSB to the index. The expression index & (-index)
isolates the least significant bit of the index.
Query Operation
The query function calculates the prefix sum up to a given index. It follows these steps:
- Increment the index to convert to 1-based indexing.
- Initialize a sum variable to 0.
- Add the value at the current node to the sum.
- Move to the parent node by removing the LSB from the index.
- Repeat steps 3-4 until we reach the root (index 0).
The line index -= index & (-index);
removes the LSB from the index, effectively moving up the tree to the parent node.
Using the Fenwick Tree
Now that we have implemented the Fenwick Tree, let’s see how to use it to solve a common problem: range sum queries with updates.
int main() {
vector<int> arr = {1, 3, 5, 7, 9, 11};
int n = arr.size();
FenwickTree ft(n);
// Build the Fenwick Tree
for (int i = 0; i < n; i++) {
ft.update(i, arr[i]);
}
// Query range sum [0, 3]
cout << "Sum of range [0, 3]: " << ft.query(3) << endl;
// Update value at index 2
ft.update(2, 2); // Add 2 to the element at index 2
// Query range sum [0, 3] again
cout << "Sum of range [0, 3] after update: " << ft.query(3) << endl;
return 0;
}
This example demonstrates how to build a Fenwick Tree from an array, perform range sum queries, and update values efficiently.
Time and Space Complexity
One of the main advantages of using a Fenwick Tree is its efficiency in both time and space complexity:
- Time Complexity:
- Update operation: O(log n)
- Query operation: O(log n)
- Construction: O(n log n) if done naively, O(n) if done optimally
- Space Complexity: O(n)
These complexities make Fenwick Trees particularly efficient for scenarios with frequent updates and queries, outperforming naive approaches that might require O(n) time for each operation.
Advanced Operations with Fenwick Trees
While the basic Fenwick Tree supports point updates and range sum queries, it can be extended to support more advanced operations. Let’s explore some of these extensions:
1. Range Updates and Point Queries
To support range updates and point queries, we can use a technique called “difference array” or “delta encoding”. The idea is to store the differences between consecutive elements instead of the actual values.
class RangeUpdateFenwickTree {
private:
FenwickTree ft;
public:
RangeUpdateFenwickTree(int size) : ft(size) {}
void rangeUpdate(int left, int right, int value) {
ft.update(left, value);
ft.update(right + 1, -value);
}
int pointQuery(int index) {
return ft.query(index);
}
};
In this implementation, rangeUpdate
adds the value to the left boundary and subtracts it from the right boundary + 1. The pointQuery
function returns the cumulative sum up to the given index, which represents the actual value at that point.
2. Range Updates and Range Queries
To support both range updates and range queries, we need to maintain two Fenwick Trees:
class RangeUpdateRangeQueryFenwickTree {
private:
FenwickTree ft1, ft2;
int n;
public:
RangeUpdateRangeQueryFenwickTree(int size) : ft1(size), ft2(size), n(size) {}
void rangeUpdate(int left, int right, int value) {
ft1.update(left, value);
ft1.update(right + 1, -value);
ft2.update(left, value * (left - 1));
ft2.update(right + 1, -value * right);
}
int rangeQuery(int left, int right) {
return prefixSum(right) - prefixSum(left - 1);
}
private:
int prefixSum(int index) {
return ft1.query(index) * index - ft2.query(index);
}
};
This implementation uses two Fenwick Trees to handle both range updates and range queries efficiently. The rangeUpdate
function updates both trees, while the rangeQuery
function calculates the difference between two prefix sums.
Common Applications of Fenwick Trees
Fenwick Trees find applications in various programming problems and real-world scenarios. Here are some common use cases:
1. Dynamic Range Sum Queries
This is the most straightforward application of Fenwick Trees. They excel at handling scenarios where you need to perform frequent updates to an array and calculate range sums efficiently.
2. Count of Smaller Numbers After Self
This problem involves finding, for each element in an array, the count of smaller elements that appear after it. Fenwick Trees can solve this efficiently by processing the array from right to left and using the tree to keep track of element frequencies.
3. Inversion Count
Fenwick Trees can be used to count the number of inversions in an array (pairs of elements where a larger element appears before a smaller one) in O(n log n) time.
4. Range Minimum/Maximum Query (RMQ)
While segment trees are more commonly used for RMQ problems, Fenwick Trees can also be adapted to solve these problems efficiently, especially when updates are involved.
5. 2D Range Sum Queries
Fenwick Trees can be extended to two dimensions to handle 2D range sum queries, which are common in image processing and computational geometry problems.
Fenwick Trees vs. Segment Trees
Both Fenwick Trees and Segment Trees are powerful data structures for range query problems. Here’s a comparison to help you choose between them:
Aspect | Fenwick Tree | Segment Tree |
---|---|---|
Implementation Complexity | Simpler, more compact | More complex, requires more code |
Memory Usage | More memory-efficient | Requires more memory |
Flexibility | Limited to certain types of queries | More flexible, can handle various query types |
Performance | Slightly faster for supported operations | Comparable performance, but more versatile |
Ease of Extension | More challenging to extend to new operations | Easier to adapt for different operations |
Choose Fenwick Trees when:
- You need a simple and memory-efficient solution for range sum queries with updates
- The problem can be reduced to prefix sum computations
- You’re working with 1D arrays or can decompose the problem into 1D subproblems
Choose Segment Trees when:
- You need to support a wider range of query types (e.g., min, max, GCD)
- The problem requires more complex range operations
- You’re dealing with 2D or higher-dimensional problems that can’t be easily decomposed
Optimizing Fenwick Tree Implementations
While the basic Fenwick Tree implementation is already efficient, there are several optimizations and variations you can consider:
1. In-place Construction
Instead of building the Fenwick Tree by individual updates, you can construct it in-place in O(n) time:
void constructInPlace(vector<int>& arr) {
for (int i = 1; i < arr.size(); i++) {
int parent = i + (i & -i);
if (parent < arr.size()) {
arr[parent] += arr[i];
}
}
}
This method directly transforms the input array into a Fenwick Tree, saving both time and space.
2. Compressed Fenwick Tree
For sparse datasets, you can use a compressed Fenwick Tree that only stores non-zero elements, reducing memory usage:
class CompressedFenwickTree {
private:
map<int, int> tree;
public:
void update(int index, int value) {
while (index < INT_MAX) {
tree[index] += value;
index += index & (-index);
}
}
int query(int index) {
int sum = 0;
while (index > 0) {
sum += tree[index];
index -= index & (-index);
}
return sum;
}
};
This implementation uses a map to store only the non-zero elements, making it more memory-efficient for sparse data.
3. Fenwick Tree with Range Updates
To support range updates more efficiently, you can use a variation that stores both the value and its coefficient:
class RangeUpdateFenwickTree {
private:
vector<int> value, coef;
int n;
public:
RangeUpdateFenwickTree(int size) : n(size + 1) {
value.resize(n, 0);
coef.resize(n, 0);
}
void rangeUpdate(int left, int right, int delta) {
update(value, left, delta);
update(value, right + 1, -delta);
update(coef, left, delta * (left - 1));
update(coef, right + 1, -delta * right);
}
int pointQuery(int index) {
return query(value, index) * index - query(coef, index);
}
private:
void update(vector<int>& tree, int index, int delta) {
while (index < n) {
tree[index] += delta;
index += index & (-index);
}
}
int query(vector<int>& tree, int index) {
int sum = 0;
while (index > 0) {
sum += tree[index];
index -= index & (-index);
}
return sum;
}
};
This implementation allows for efficient range updates and point queries, which can be useful in many scenarios.
Fenwick Trees in Competitive Programming
Fenwick Trees are a favorite among competitive programmers due to their efficiency and versatility. Here are some tips for using Fenwick Trees effectively in coding competitions:
- Practice Implementation: Implement Fenwick Trees from scratch multiple times until you can do it quickly and without errors.
- Recognize Applicable Problems: Learn to identify problems that can be solved efficiently using Fenwick Trees, especially those involving range sum queries or frequency counting.
- Combine with Other Techniques: Fenwick Trees often work well in combination with other algorithms and data structures. For example, you might use a Fenwick Tree as part of a solution involving binary search or coordinate compression.
- Be Aware of Variations: Familiarize yourself with different variations of Fenwick Trees, such as 2D Fenwick Trees or those supporting range updates, as these can be crucial for solving certain types of problems.
- Consider Space Complexity: In some contests, memory usage is as important as time complexity. Fenwick Trees are generally more memory-efficient than segment trees, which can be an advantage.
- Use Templates: Prepare a well-tested template for Fenwick Trees that you can quickly adapt to different problem requirements.
Real-world Applications of Fenwick Trees
While Fenwick Trees are commonly associated with competitive programming, they also have practical applications in various fields:
1. Database Systems
Fenwick Trees can be used to efficiently maintain cumulative statistics in database systems, especially for scenarios requiring frequent updates and range queries.
2. Financial Analysis
In financial applications, Fenwick Trees can be employed to quickly compute running totals or moving averages over large datasets of financial transactions.
3. Network Flow Analysis
Fenwick Trees can be used to maintain and query cumulative network traffic statistics efficiently, allowing for real-time analysis of network flows.
4. Computational Biology
In bioinformatics, Fenwick Trees can be applied to problems involving sequence analysis, such as efficiently counting occurrences of subsequences in DNA or protein sequences.
5. Geographic Information Systems (GIS)
2D Fenwick Trees can be used in GIS applications for efficient querying of cumulative data over rectangular regions of maps or satellite imagery.
Conclusion
Fenwick Trees, or Binary Indexed Trees, are powerful data structures that provide an efficient solution for range sum queries and updates. Their simplicity, memory efficiency, and fast performance make them an invaluable tool in a programmer’s toolkit, especially for those tackling complex algorithmic problems or working on systems requiring frequent updates to cumulative data.
By mastering Fenwick Trees, you’ll be well-equipped to solve a wide range of problems in competitive programming, technical interviews, and real-world applications. Remember to practice implementing and using Fenwick Trees in various scenarios to fully grasp their potential and limitations.
As you continue your journey in algorithmic problem-solving, consider exploring related data structures like segment trees and sparse tables to expand your problem-solving capabilities further. Happy coding!