Mastering Embedded Systems Programming: A Comprehensive Guide to Microcontroller Programming with C and Embedded C++
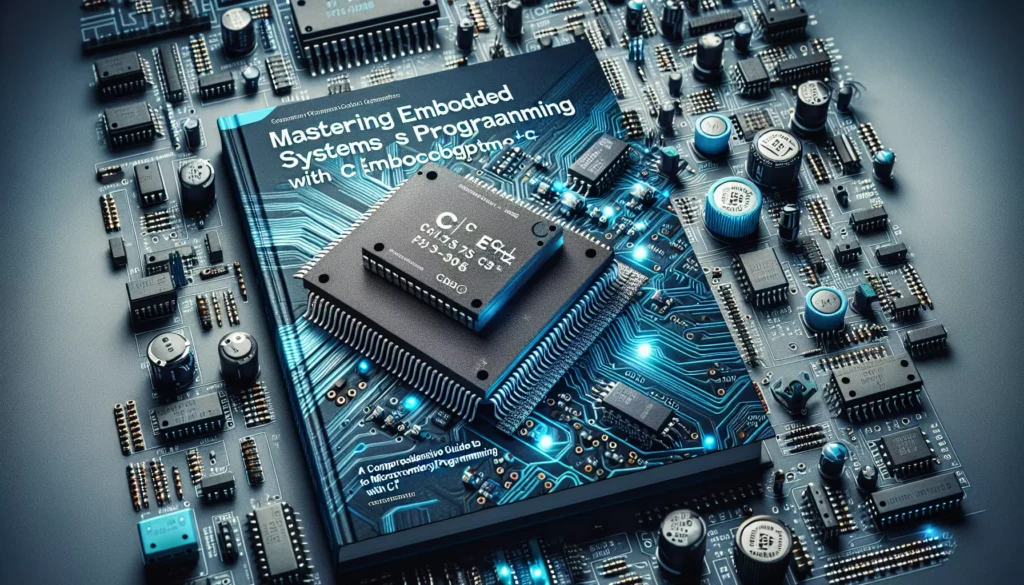
In the ever-evolving world of technology, embedded systems play a crucial role in powering countless devices we use daily. From smart home appliances to automotive systems, embedded systems are the hidden intelligence behind modern technology. As the demand for skilled embedded systems programmers continues to grow, it’s essential for aspiring developers to master the art of programming microcontrollers using languages like C and Embedded C++. In this comprehensive guide, we’ll dive deep into the world of embedded systems programming, exploring key concepts, best practices, and practical examples to help you become proficient in this exciting field.
Understanding Embedded Systems
Before we delve into the intricacies of programming microcontrollers, it’s important to understand what embedded systems are and why they’re so important in today’s technological landscape.
What are Embedded Systems?
An embedded system is a combination of computer hardware and software designed to perform a specific function within a larger system. These systems are typically built to handle dedicated tasks and are often subject to real-time computing constraints. Examples of embedded systems include:
- Digital watches
- Traffic light controllers
- Home automation systems
- Industrial control systems
- Automotive engine control units
- Medical devices
The Role of Microcontrollers in Embedded Systems
At the heart of many embedded systems lies a microcontroller. A microcontroller is a small computer on a single integrated circuit, containing a processor, memory, and programmable input/output peripherals. Microcontrollers are designed to be compact, energy-efficient, and cost-effective, making them ideal for embedded applications.
Getting Started with Microcontroller Programming
To begin programming microcontrollers, you’ll need to familiarize yourself with the development environment and the programming languages commonly used in embedded systems.
Choosing the Right Development Environment
Selecting an appropriate development environment is crucial for efficient microcontroller programming. Some popular options include:
- Arduino IDE: An excellent choice for beginners, especially when working with Arduino boards.
- PlatformIO: A cross-platform IDE that supports multiple microcontroller platforms.
- Keil MDK: A comprehensive development environment for ARM-based microcontrollers.
- IAR Embedded Workbench: A powerful IDE supporting a wide range of microcontrollers.
C vs. Embedded C++: Choosing the Right Language
Both C and C++ are widely used in embedded systems programming, each with its own strengths and use cases.
C for Embedded Systems
C remains the most popular language for embedded systems programming due to its efficiency, low-level hardware control, and small memory footprint. It’s an excellent choice for resource-constrained systems and when direct hardware manipulation is required.
Embedded C++
Embedded C++ is a subset of the C++ language tailored for embedded systems. It offers object-oriented programming features while maintaining the efficiency of C. Embedded C++ is particularly useful for larger, more complex embedded systems where code organization and reusability are important.
Essential Concepts in Microcontroller Programming
To become proficient in microcontroller programming, you’ll need to master several key concepts:
1. Memory Management
Efficient memory management is crucial in embedded systems due to limited resources. Understanding different types of memory (RAM, ROM, Flash) and how to allocate and deallocate memory properly is essential.
2. Interrupt Handling
Interrupts are essential for real-time responsiveness in embedded systems. Learning how to configure and handle interrupts effectively is a fundamental skill.
3. Peripheral Interfacing
Microcontrollers often need to interface with various peripherals such as sensors, displays, and communication modules. Understanding how to configure and communicate with these peripherals is crucial.
4. Real-Time Operating Systems (RTOS)
For more complex embedded systems, using an RTOS can help manage tasks, timing, and resource allocation more efficiently.
5. Low-Power Design
Many embedded systems run on battery power, making low-power design techniques essential for extending battery life.
Programming Microcontrollers with C
Let’s explore some practical examples of programming microcontrollers using C.
Example 1: Blinking an LED
One of the simplest examples of microcontroller programming is blinking an LED. Here’s a basic C code snippet for an AVR microcontroller:
#include <avr/io.h>
#include <util/delay.h>
#define LED_PIN PB5
int main(void) {
DDRB |= (1 << LED_PIN); // Set LED pin as output
while (1) {
PORTB |= (1 << LED_PIN); // Turn LED on
_delay_ms(500); // Wait for 500 ms
PORTB &= ~(1 << LED_PIN); // Turn LED off
_delay_ms(500); // Wait for 500 ms
}
return 0;
}
Example 2: Reading an Analog Sensor
Here’s an example of reading an analog sensor using the ADC (Analog-to-Digital Converter) on an AVR microcontroller:
#include <avr/io.h>
#include <util/delay.h>
void adc_init() {
ADMUX = (1<<REFS0); // Set reference voltage to AVCC
ADCSRA = (1<<ADEN) | (1<<ADPS2) | (1<<ADPS1) | (1<<ADPS0); // Enable ADC, set prescaler
}
uint16_t adc_read(uint8_t channel) {
ADMUX = (ADMUX & 0xF0) | (channel & 0x0F); // Select ADC channel
ADCSRA |= (1<<ADSC); // Start conversion
while (ADCSRA & (1<<ADSC)); // Wait for conversion to complete
return ADC; // Return result
}
int main(void) {
adc_init();
while (1) {
uint16_t sensor_value = adc_read(0); // Read from ADC channel 0
// Process sensor_value as needed
_delay_ms(100); // Wait before next reading
}
return 0;
}
Programming Microcontrollers with Embedded C++
Now, let’s look at some examples of using Embedded C++ for microcontroller programming.
Example 1: Object-Oriented LED Control
Here’s an example of how we can use object-oriented programming in Embedded C++ to control an LED:
#include <avr/io.h>
#include <util/delay.h>
class LED {
private:
uint8_t pin;
public:
LED(uint8_t pin) : pin(pin) {
DDRB |= (1 << pin); // Set LED pin as output
}
void on() {
PORTB |= (1 << pin);
}
void off() {
PORTB &= ~(1 << pin);
}
void toggle() {
PORTB ^= (1 << pin);
}
};
int main() {
LED led(PB5); // Create LED object for pin PB5
while (1) {
led.on();
_delay_ms(500);
led.off();
_delay_ms(500);
}
return 0;
}
Example 2: Template-Based Peripheral Interface
Embedded C++ allows us to use templates for creating flexible, reusable code. Here’s an example of a template-based peripheral interface:
#include <avr/io.h>
template<typename T, volatile uint8_t* port, uint8_t pin>
class DigitalOutput {
public:
static void init() {
*(&DDR<T>) |= (1 << pin);
}
static void set() {
*port |= (1 << pin);
}
static void clear() {
*port &= ~(1 << pin);
}
static void toggle() {
*port ^= (1 << pin);
}
};
int main() {
DigitalOutput<B, &PORTB, PB5>::init();
while (1) {
DigitalOutput<B, &PORTB, PB5>::set();
// Wait...
DigitalOutput<B, &PORTB, PB5>::clear();
// Wait...
}
return 0;
}
Best Practices for Embedded Systems Programming
To write efficient and reliable code for embedded systems, consider the following best practices:
1. Optimize for Resource Constraints
Always be mindful of the limited resources available on microcontrollers. Optimize your code for memory usage and execution speed.
2. Use Appropriate Data Types
Choose the smallest data type that can accommodate your data to conserve memory. For example, use uint8_t for small unsigned integers instead of int.
3. Avoid Dynamic Memory Allocation
Dynamic memory allocation can lead to fragmentation and unpredictable behavior. Prefer static allocation whenever possible.
4. Implement Robust Error Handling
Embedded systems often operate in critical environments. Implement thorough error checking and handling to ensure system reliability.
5. Use Interrupts Judiciously
While interrupts are powerful, overuse can lead to complex, hard-to-debug code. Use them wisely and keep interrupt service routines (ISRs) short.
6. Document Your Code Thoroughly
Clear documentation is crucial, especially for embedded systems where hardware-specific details are important. Document your code, including hardware connections and configurations.
7. Implement Watchdog Timers
Use watchdog timers to detect and recover from software malfunctions, ensuring your system can recover from unexpected errors.
Advanced Topics in Embedded Systems Programming
As you progress in your embedded systems programming journey, you’ll encounter more advanced topics:
1. Real-Time Operating Systems (RTOS)
For complex embedded systems, using an RTOS like FreeRTOS or Zephyr can help manage tasks, timing, and resources more effectively.
2. Communication Protocols
Learn to implement various communication protocols such as I2C, SPI, UART, and CAN for interfacing with other devices and systems.
3. Bootloaders
Understand how to implement bootloaders for firmware updates and system recovery.
4. Power Management
Explore advanced power management techniques to optimize battery life in portable embedded systems.
5. Security in Embedded Systems
As embedded systems become more connected, understanding and implementing security measures becomes crucial.
Conclusion
Mastering embedded systems programming with C and Embedded C++ opens up a world of possibilities in the realm of technology. From simple microcontroller projects to complex IoT devices, the skills you develop in this field are invaluable in today’s tech-driven world.
Remember, the key to becoming proficient in embedded systems programming is practice. Start with simple projects, gradually increase complexity, and always strive to optimize your code for the specific constraints of your target system.
As you continue your journey in embedded systems programming, keep exploring new microcontroller platforms, stay updated with the latest industry trends, and never stop learning. The field of embedded systems is constantly evolving, and there’s always something new to discover and master.
Whether you’re aiming to build innovative IoT devices, develop cutting-edge automotive systems, or create the next generation of smart appliances, your skills in embedded systems programming will be the foundation of your success. Embrace the challenges, enjoy the process of bringing hardware to life with your code, and get ready to make your mark in the exciting world of embedded systems!