Mastering Dynamic Programming: Essential Techniques for Solving Complex Algorithmic Problems
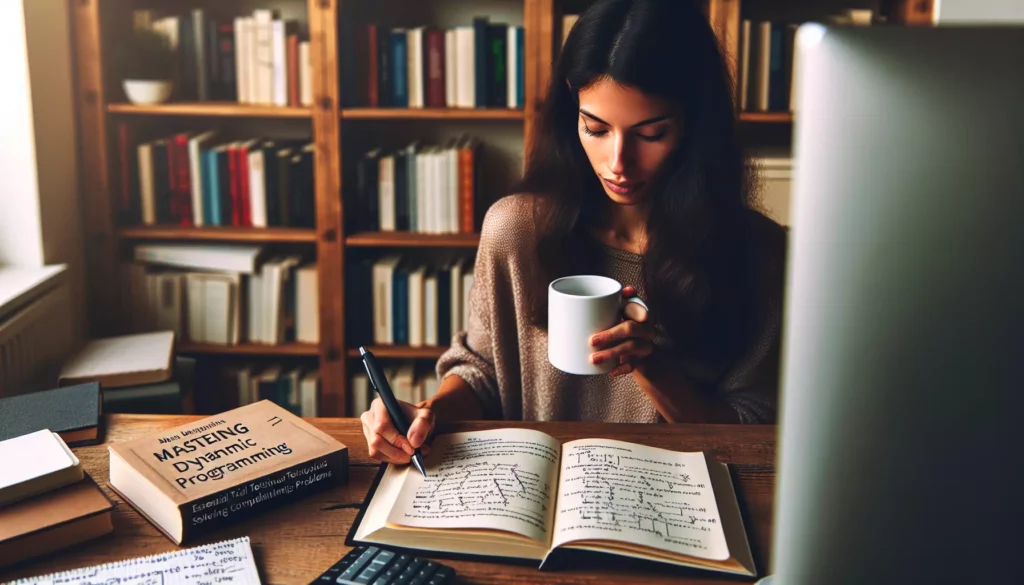
Dynamic programming (DP) is a powerful problem-solving technique that can tackle complex algorithmic challenges by breaking them down into simpler subproblems. It’s a fundamental concept in computer science and a favorite topic in technical interviews, especially for prestigious tech companies. In this comprehensive guide, we’ll explore the best techniques for solving dynamic programming questions, providing you with the tools you need to excel in your coding journey and ace those challenging interview problems.
Understanding Dynamic Programming
Before diving into specific techniques, it’s crucial to understand what dynamic programming is and why it’s so important in algorithmic problem-solving.
What is Dynamic Programming?
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is applicable when the problem has the following characteristics:
- Overlapping Subproblems: The problem can be broken down into subproblems which are reused several times.
- Optimal Substructure: The optimal solution to the problem can be constructed from optimal solutions of its subproblems.
The key idea behind dynamic programming is to store the results of subproblems so that we don’t have to recompute them when needed later. This technique can significantly reduce the time complexity of an algorithm.
Why is Dynamic Programming Important?
Dynamic programming is essential for several reasons:
- It can solve problems that would be infeasible with a naive recursive approach due to exponential time complexity.
- It’s widely used in various fields, including computer science, mathematics, and economics.
- It’s a common topic in coding interviews, especially for top tech companies.
- Mastering DP enhances your problem-solving skills and algorithmic thinking.
Key Techniques for Solving Dynamic Programming Problems
Now that we understand the basics, let’s explore the best techniques for tackling dynamic programming questions.
1. Identify the Problem Type
The first step in solving any DP problem is to identify what type of problem it is. Common types of DP problems include:
- 0/1 Knapsack
- Unbounded Knapsack
- Longest Common Subsequence (LCS)
- Longest Increasing Subsequence (LIS)
- Matrix Chain Multiplication
- Shortest Path Problems
- Edit Distance
Recognizing the problem type can help you apply known patterns and solutions, making the problem-solving process more straightforward.
2. Define the Subproblem
Once you’ve identified the problem type, the next step is to define the subproblem. This involves breaking down the main problem into smaller, manageable pieces. Ask yourself:
- What does a single step in the solution look like?
- How can the problem be expressed in terms of smaller instances of the same problem?
For example, in the Fibonacci sequence problem, the subproblem would be calculating the Fibonacci number for a smaller index.
3. Establish the Recurrence Relation
The recurrence relation is the heart of any DP solution. It describes how the solution to the larger problem can be constructed from solutions to smaller subproblems. To establish the recurrence relation:
- Identify the base cases (smallest possible subproblems)
- Determine how larger cases can be built from smaller ones
For the Fibonacci sequence, the recurrence relation would be:
F(n) = F(n-1) + F(n-2)
Base cases: F(0) = 0, F(1) = 1
4. Choose the Right DP Approach
There are two main approaches to implementing a DP solution:
Top-Down Approach (Memoization)
In this approach, we start with the original problem and recursively break it down into subproblems. We use a data structure (usually an array or a hash map) to store the results of subproblems to avoid redundant computations.
Here’s an example of a top-down approach for the Fibonacci sequence:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
Bottom-Up Approach (Tabulation)
In this approach, we start by solving the smallest subproblems and use their results to build up to the solution of the original problem. This is typically implemented using iteration rather than recursion.
Here’s an example of a bottom-up approach for the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Both approaches have their advantages:
- Top-down is often easier to implement and can be more intuitive.
- Bottom-up usually has better space complexity and avoids the overhead of recursive function calls.
5. Optimize Space Complexity
After implementing a basic DP solution, consider whether you can optimize the space complexity. Often, you don’t need to store all intermediate results. For example, in the Fibonacci sequence, you only need the last two numbers to calculate the next one.
Here’s an optimized version of the Fibonacci function:
def fibonacci(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
This version reduces the space complexity from O(n) to O(1).
6. Practice with Common DP Patterns
Many DP problems follow common patterns. Familiarizing yourself with these patterns can help you solve new problems more quickly. Some common patterns include:
- Linear DP (e.g., Fibonacci sequence)
- Knapsack problems
- Matrix DP (e.g., Unique Paths problem)
- Two-sequence DP (e.g., Longest Common Subsequence)
- Interval DP (e.g., Palindrome Partitioning)
- Tree DP
- State Compression DP
Practice problems that fall into each of these categories to build your DP problem-solving skills.
Advanced Dynamic Programming Techniques
Once you’ve mastered the basics, you can move on to more advanced DP techniques.
1. State Reduction
Sometimes, the straightforward DP solution might use too many states, leading to high time and space complexity. State reduction involves finding a way to represent the problem state with fewer variables.
For example, in the “Paint House” problem, where you need to paint houses with different colors at minimum cost, a naive approach might use three states: the house number, the color of the current house, and the color of the previous house. However, you can reduce this to just two states: the house number and the color of the current house, as the color of the previous house is implicitly considered in the recurrence relation.
2. Divide and Conquer DP
This technique combines the divide-and-conquer approach with dynamic programming. It’s useful when the recurrence relation involves minimizing over all possible partitions of the problem.
A classic example is the “Matrix Chain Multiplication” problem, where you need to find the most efficient way to multiply a chain of matrices.
3. Digit DP
Digit DP is a technique used to solve problems involving digit properties of numbers in a given range. It’s particularly useful for problems that ask to count numbers with certain digit-related properties within a range.
For example, counting the number of integers between A and B (inclusive) that have a sum of digits equal to a given value.
4. DP on Trees
DP can also be applied to problems involving trees. These problems often involve computing some value for each node based on values of its children or parent.
An example is the “Binary Tree Maximum Path Sum” problem, where you need to find the path with the maximum sum in a binary tree.
5. DP with Bitmasks
This technique uses bitmasks to represent sets and is often used in problems involving subsets or permutations. It’s particularly useful when the number of elements is small (usually less than 20).
The “Traveling Salesman Problem” is a classic example where DP with bitmasks can be applied.
Common Pitfalls and How to Avoid Them
Even with a good understanding of DP techniques, it’s easy to fall into certain traps. Here are some common pitfalls and how to avoid them:
1. Overlooking Base Cases
Forgetting to handle base cases properly is a common mistake in DP solutions. Always start by clearly defining and implementing the base cases before moving on to the general case.
2. Incorrect Recurrence Relation
The recurrence relation is the core of your DP solution. If it’s incorrect, the entire solution will be wrong. Take time to carefully derive and verify your recurrence relation.
3. Not Memoizing Recursive Calls
When using the top-down approach, forgetting to memoize results can lead to exponential time complexity. Always store and reuse computed results for subproblems.
4. Unnecessary Computation in Bottom-Up Approach
In the bottom-up approach, computing values that aren’t needed for the final result is a waste of time and space. Make sure you’re only computing necessary values.
5. Not Considering Space Optimization
While your initial solution might work, it’s important to consider whether you can optimize space usage, especially for large inputs.
Practicing Dynamic Programming
The key to mastering dynamic programming is practice. Here are some strategies to improve your DP skills:
1. Solve Problems Incrementally
Start with simple DP problems and gradually move to more complex ones. Websites like LeetCode, HackerRank, and Codeforces offer a wide range of DP problems with varying difficulty levels.
2. Implement Both Approaches
For each problem you solve, try implementing both the top-down and bottom-up approaches. This will help you understand the trade-offs between the two and when to use each.
3. Analyze Time and Space Complexity
For each solution, analyze its time and space complexity. This will help you understand the efficiency of your solutions and how to optimize them.
4. Study Solution Patterns
After solving a problem, study other people’s solutions. You might find more efficient or elegant ways to solve the same problem.
5. Participate in Coding Contests
Participating in coding contests can expose you to a variety of DP problems and help you solve them under time pressure, which is valuable practice for interviews.
Conclusion
Dynamic programming is a powerful technique that can solve a wide range of complex algorithmic problems. By mastering the techniques discussed in this article – from identifying problem types and establishing recurrence relations to implementing top-down and bottom-up approaches – you’ll be well-equipped to tackle even the most challenging DP questions.
Remember, the key to mastering dynamic programming is practice. Start with simpler problems, gradually increase the difficulty, and don’t be discouraged if you struggle at first. With persistence and the right approach, you’ll soon find yourself confidently solving complex DP problems and acing those technical interviews.
Happy coding, and may your dynamic programming journey be filled with optimal solutions!