Mastering Domain-Specific Languages (DSLs): A Comprehensive Guide for Developers
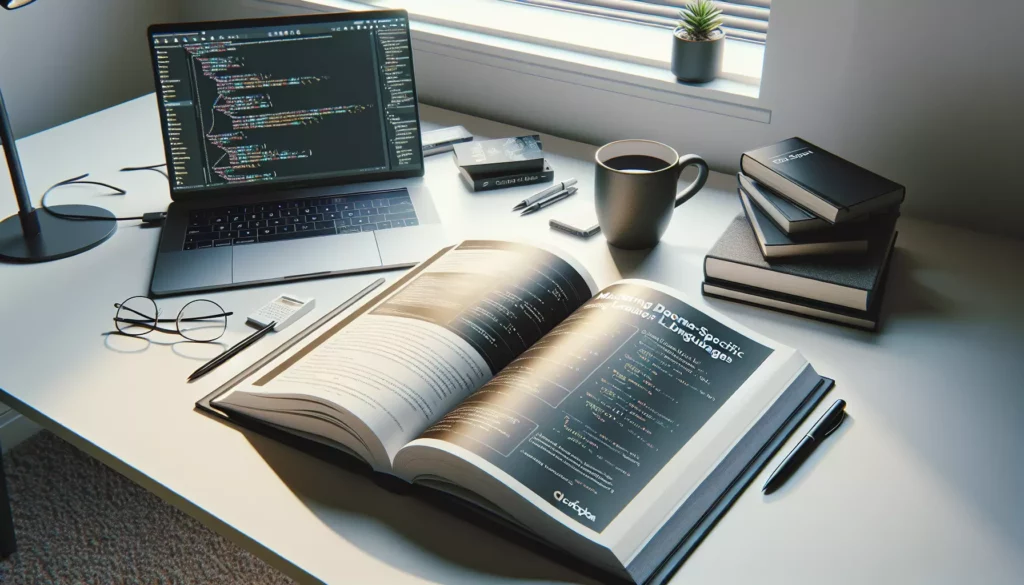
In the ever-evolving landscape of software development, efficiency and precision are paramount. As developers, we’re constantly seeking ways to streamline our processes and create more powerful, targeted solutions. This is where Domain-Specific Languages (DSLs) come into play. DSLs are specialized programming languages designed to address specific problem domains, offering a level of expressiveness and productivity that general-purpose languages often can’t match.
In this comprehensive guide, we’ll dive deep into the world of Domain-Specific Languages, exploring their benefits, design principles, and implementation strategies. Whether you’re a seasoned programmer looking to expand your toolkit or a coding enthusiast eager to explore new horizons, this article will equip you with the knowledge and insights needed to harness the power of DSLs in your projects.
Table of Contents
- Understanding Domain-Specific Languages
- Benefits of Using Domain-Specific Languages
- Types of Domain-Specific Languages
- Designing Effective Domain-Specific Languages
- Implementing Domain-Specific Languages
- Real-World Examples of Successful DSLs
- Challenges and Considerations in DSL Development
- The Future of Domain-Specific Languages
- Conclusion
1. Understanding Domain-Specific Languages
Before we delve into the intricacies of Domain-Specific Languages, let’s establish a clear understanding of what they are and how they differ from general-purpose programming languages.
What is a Domain-Specific Language?
A Domain-Specific Language (DSL) is a programming language or specification language dedicated to a particular problem domain, a specific problem representation technique, and/or a particular solution technique. Unlike general-purpose languages like Python, Java, or C++, which are designed to solve a wide range of problems across various domains, DSLs are tailored to address specific needs within a narrowly defined area.
Characteristics of DSLs
- Focused Vocabulary: DSLs use terms and concepts that are familiar to domain experts, making them more intuitive and easier to use for specific tasks.
- Limited Expressiveness: While DSLs are highly expressive within their domain, they typically have limited functionality outside their intended use case.
- Declarative Nature: Many DSLs are declarative, focusing on what needs to be done rather than how to do it, which can lead to more concise and readable code.
- Increased Productivity: By providing domain-specific abstractions and syntax, DSLs can significantly boost productivity for tasks within their domain.
DSLs vs. General-Purpose Languages
To better understand DSLs, it’s helpful to compare them with general-purpose languages:
Aspect | Domain-Specific Languages | General-Purpose Languages |
---|---|---|
Scope | Narrow, focused on a specific domain | Broad, applicable to various domains |
Learning Curve | Generally easier for domain experts | Steeper learning curve, more versatile |
Expressiveness | Highly expressive within the domain | Broadly expressive across domains |
Abstraction Level | Higher, domain-specific abstractions | Lower, more general abstractions |
Flexibility | Limited to the specific domain | Highly flexible for various tasks |
2. Benefits of Using Domain-Specific Languages
The adoption of Domain-Specific Languages offers numerous advantages for both developers and domain experts. Let’s explore some of the key benefits:
Increased Productivity
One of the primary advantages of DSLs is the significant boost in productivity they offer. By providing a language tailored to a specific domain, DSLs allow developers to express complex ideas more concisely and intuitively. This results in:
- Faster development cycles
- Reduced code complexity
- Easier maintenance and updates
Improved Communication
DSLs bridge the gap between developers and domain experts by using familiar terminology and concepts. This leads to:
- Better collaboration between technical and non-technical team members
- Clearer expression of domain-specific requirements
- Reduced misunderstandings and errors in implementation
Enhanced Quality and Reliability
By constraining the language to a specific domain, DSLs can enforce best practices and domain-specific rules, resulting in:
- Fewer bugs and errors
- More consistent and standardized code
- Easier validation and testing of domain-specific logic
Empowerment of Domain Experts
Well-designed DSLs can enable domain experts to directly contribute to or even write parts of the application logic, leading to:
- Reduced dependency on software developers for certain tasks
- More accurate representation of domain knowledge in the code
- Faster iteration and prototyping of domain-specific features
Improved Maintainability
DSLs often result in more readable and self-documenting code, which contributes to:
- Easier onboarding of new team members
- Reduced need for extensive documentation
- Simpler long-term maintenance of the codebase
3. Types of Domain-Specific Languages
Domain-Specific Languages come in various forms, each with its own characteristics and use cases. Understanding these different types can help you choose the right approach for your specific needs.
External DSLs
External DSLs are standalone languages with their own syntax and parser. They are completely separate from the host language used to implement them.
Characteristics:
- Custom syntax tailored to the domain
- Requires a separate parser and interpreter/compiler
- Offers maximum flexibility in language design
Examples:
- SQL for database queries
- Regular expressions for pattern matching
- HTML for web markup
Internal DSLs
Also known as embedded DSLs, these are implemented within a host general-purpose language, leveraging its syntax and infrastructure.
Characteristics:
- Uses the syntax of the host language
- Easier to implement and integrate with existing codebases
- Limited by the constraints of the host language
Examples:
- Ruby on Rails for web development in Ruby
- LINQ for querying in C#
- Scala’s parser combinators for parsing
Language Workbenches
Language workbenches are specialized IDEs or tools designed for creating and using DSLs efficiently.
Characteristics:
- Provide integrated tools for DSL design, implementation, and use
- Often support multiple DSLs working together
- Can generate code, interpreters, or other artifacts from DSL definitions
Examples:
- JetBrains MPS
- Xtext
- Spoofax
Graphical DSLs
These DSLs use visual elements instead of text to represent domain concepts and relationships.
Characteristics:
- Visual representation of domain concepts
- Often more intuitive for non-programmers
- Can be challenging to version control and diff
Examples:
- UML for software modeling
- LabVIEW for instrument control and industrial automation
- Scratch for educational programming
4. Designing Effective Domain-Specific Languages
Creating a successful Domain-Specific Language requires careful planning and consideration of various factors. Here are key principles and steps to follow when designing your DSL:
Understand the Domain
Before you start designing your DSL, it’s crucial to have a deep understanding of the problem domain:
- Collaborate closely with domain experts
- Identify key concepts, relationships, and operations within the domain
- Understand the workflows and processes that the DSL will need to support
Define Clear Goals and Scope
Establish clear objectives for your DSL:
- What specific problems will the DSL solve?
- Who are the intended users of the DSL?
- What level of expressiveness is required?
Choose the Right Level of Abstraction
Strike a balance between simplicity and power:
- Provide high-level abstractions that match domain concepts
- Avoid unnecessary complexity that might confuse users
- Ensure the DSL is expressive enough to handle all required use cases
Design a Clear and Intuitive Syntax
The syntax of your DSL should be easy to read and write:
- Use terminology familiar to domain experts
- Keep the syntax consistent and predictable
- Provide clear error messages and guidance
Consider Extensibility and Interoperability
Plan for future growth and integration:
- Design the DSL to be extensible for future requirements
- Consider how the DSL will interact with other languages and systems
- Provide mechanisms for customization and user-defined extensions
Prioritize Readability and Maintainability
Remember that DSL code will need to be maintained:
- Favor readability over extreme conciseness
- Provide ways to organize and structure larger DSL programs
- Consider versioning and backward compatibility
Iterate and Refine
DSL design is an iterative process:
- Create prototypes and gather feedback early
- Be prepared to refine and adjust the design based on user input
- Continuously evaluate the DSL against its goals and user needs
5. Implementing Domain-Specific Languages
Once you’ve designed your Domain-Specific Language, the next step is implementation. The approach you take will depend on whether you’re creating an external or internal DSL, as well as the specific requirements of your project.
Implementing External DSLs
Creating an external DSL involves building a complete language processing system:
1. Define the Grammar
Use a formal grammar notation like EBNF (Extended Backus-Naur Form) to define the syntax of your language.
2. Build a Lexer and Parser
Use parser generator tools like ANTLR, Yacc, or Bison to create a lexer and parser for your DSL.
3. Construct an Abstract Syntax Tree (AST)
Design and implement an AST to represent the structure of programs written in your DSL.
4. Implement Semantic Analysis
Perform type checking, scope resolution, and other semantic analyses on the AST.
5. Generate Code or Interpret
Either generate code in a target language or implement an interpreter to execute the DSL directly.
Example: A Simple Arithmetic DSL Parser in Python using ANTLR
// Arithmetic.g4
grammar Arithmetic;
expr: term ((PLUS | MINUS) term)*;
term: factor ((MUL | DIV) factor)*;
factor: NUMBER | LPAREN expr RPAREN;
PLUS: '+';
MINUS: '-';
MUL: '*';
DIV: '/';
LPAREN: '(';
RPAREN: ')';
NUMBER: [0-9]+;
WS: [ \t\r\n]+ -> skip;
To use this grammar with ANTLR and Python:
from antlr4 import *
from ArithmeticLexer import ArithmeticLexer
from ArithmeticParser import ArithmeticParser
def main():
input_stream = InputStream("3 + 4 * (2 - 1)")
lexer = ArithmeticLexer(input_stream)
stream = CommonTokenStream(lexer)
parser = ArithmeticParser(stream)
tree = parser.expr()
print(tree.toStringTree(recog=parser))
if __name__ == '__main__':
main()
Implementing Internal DSLs
Creating an internal DSL involves leveraging the host language’s features:
1. Choose a Suitable Host Language
Select a host language that provides features conducive to DSL creation, such as Ruby, Scala, or Kotlin.
2. Design the API
Create a fluent API that represents your DSL’s concepts and operations.
3. Implement Domain Objects
Create classes and methods that encapsulate the domain logic.
4. Use Language Features
Leverage features like operator overloading, implicit conversions, and metaprogramming to create a natural syntax.
5. Provide Context and Scoping
Use techniques like builders or context objects to manage state and provide scoping.
Example: A Simple Internal DSL for Creating HTML in Ruby
class HTMLBuilder
def initialize
@html = ""
end
def method_missing(name, *args, &block)
tag(name, *args, &block)
end
def tag(name, content = nil, attributes = {})
@html << "<#{name}#{attributes_to_s(attributes)}>"
if block_given?
yield
else
@html << content.to_s if content
end
@html << "</#{name}>"
end
def attributes_to_s(attributes)
attributes.map { |k, v| " #{k}=\"#{v}\"" }.join
end
def to_s
@html
end
end
def html(&block)
builder = HTMLBuilder.new
builder.instance_eval(&block)
builder.to_s
end
# Usage
puts html {
html {
head {
title "My Page"
}
body {
h1 "Welcome"
p "This is a paragraph"
ul {
li "Item 1"
li "Item 2"
}
}
}
}
6. Real-World Examples of Successful DSLs
To better understand the power and versatility of Domain-Specific Languages, let’s explore some successful real-world examples across various domains:
1. SQL (Structured Query Language)
SQL is perhaps one of the most widely used DSLs, designed specifically for managing and querying relational databases.
Key Features:
- Declarative syntax for querying data
- Standardized language across different database systems
- Powerful operations for data manipulation and definition
Example SQL Query:
SELECT customers.name, orders.order_date
FROM customers
JOIN orders ON customers.id = orders.customer_id
WHERE orders.total_amount > 1000
ORDER BY orders.order_date DESC;
2. HTML (Hypertext Markup Language)
HTML is a markup language specifically designed for creating web pages and web applications.
Key Features:
- Simple, tag-based syntax
- Hierarchical structure representing document layout
- Extensible through attributes and custom tags
Example HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Web Page</title>
</head>
<body>
<h1>Welcome to My Page</h1>
<p>This is a paragraph of text.</p>
<ul>
<li>List item 1</li>
<li>List item 2</li>
</ul>
</body>
</html>
3. Ansible
Ansible is a DSL for IT automation, configuration management, and application deployment.
Key Features:
- YAML-based syntax for defining infrastructure as code
- Declarative approach to system configuration
- Extensive library of modules for various tasks
Example Ansible Playbook:
---
- name: Install and configure web server
hosts: webservers
become: yes
tasks:
- name: Install Apache
apt:
name: apache2
state: present
- name: Start Apache service
service:
name: apache2
state: started
enabled: yes
- name: Copy index.html
copy:
src: files/index.html
dest: /var/www/html/index.html
4. Gradle
Gradle is a build automation DSL that uses a Groovy or Kotlin-based DSL for defining build scripts.
Key Features:
- Flexible, programmable build scripts
- Dependency management and resolution
- Extensible plugin system
Example Gradle Build Script:
plugins {
id 'java'
id 'application'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'com.google.guava:guava:30.1-jre'
testImplementation 'junit:junit:4.13.2'
}
application {
mainClass = 'com.example.MyApp'
}
tasks.named('test') {
useJUnitPlatform()
}
5. R for Statistical Computing
While R is a general-purpose language, it can be considered a DSL for statistical computing and data analysis.
Key Features:
- Rich set of statistical and graphical techniques
- Vector-based operations for efficient data manipulation
- Extensive ecosystem of packages for various statistical tasks
Example R Code for Data Analysis:
# Load libraries
library(ggplot2)
library(dplyr)
# Load and prepare data
data(mtcars)
mtcars$am <- factor(mtcars$am, labels = c("Automatic", "Manual"))
# Perform analysis
summary_stats <- mtcars %>%
group_by(am) >>
summarise(
mean_mpg = mean(mpg),
sd_mpg = sd(mpg)
)
# Create visualization
ggplot(mtcars, aes(x = am, y = mpg)) +
geom_boxplot() +
geom_jitter(width = 0.2, alpha = 0.5) +
labs(title = "MPG by Transmission Type",
x = "Transmission",
y = "Miles per Gallon") +
theme_minimal()
7. Challenges and Considerations in DSL Development
While Domain-Specific Languages offer numerous benefits, their development and implementation come with their own set of challenges. Being aware of these considerations can help you make informed decisions and avoid common pitfalls in DSL development.
1. Balancing Power and Simplicity
One of the primary challenges in DSL design is striking the right balance between expressiveness and simplicity. A DSL that’s too simple may not adequately address all the needs of the domain, while one that’s too complex can be difficult to learn and use.
Considerations:
- Carefully analyze the domain to identify essential features
- Prioritize the most common use cases
- Consider providing extension mechanisms for advanced users
2. Learning Curve and Adoption
Introducing a new DSL requires users to learn a new language, which can be a barrier to adoption, especially in larger organizations or for widely distributed tools.
Strategies to Address This:
- Provide comprehensive documentation and examples
- Offer training sessions or workshops
- Create interactive tutorials or playground environments
- Ensure the DSL aligns closely with domain experts’ mental models
3. Maintenance and Evolution
As the problem domain evolves, the DSL must evolve with it. This can be challenging, especially if the DSL is widely adopted.
Considerations:
- Design for extensibility from the start
- Implement versioning for the DSL
- Provide migration tools or scripts for updating existing code
- Maintain clear deprecation policies and communication channels
4. Tool Support
General-purpose languages often have rich ecosystems of development tools, which may not be available for custom DSLs.
Potential Solutions:
- Develop custom IDE plugins or extensions
- Use language workbenches that provide integrated tool support
- Create linters, formatters, and other development aids
5. Performance Considerations
Depending on the implementation, DSLs may introduce performance overhead compared to general-purpose languages.
Strategies to Mitigate:
- Optimize the DSL implementation, especially for frequently used operations
- Consider compiling the DSL to a lower-level language for performance-critical applications
- Provide profiling and optimization tools specific to the DSL
6. Integration with Existing Systems
DSLs often need to integrate with existing codebases, tools, and workflows, which can be challenging.
Approaches:
- Design clear interfaces between the DSL and other systems
- Provide APIs or libraries for interoperability
- Consider generating code in a general-purpose language as an intermediate step
7. Testing and Validation
Ensuring the correctness and reliability of DSL programs can be more challenging than with general-purpose languages.
Best Practices:
- Develop a comprehensive test suite for the DSL implementation
- Create domain-specific testing tools or frameworks
- Implement static analysis tools for common errors or anti-patterns
8. Skill Set Requirements
Developing a DSL requires a unique combination of skills, including domain expertise, language design, and compiler/interpreter implementation.
Strategies:
- Foster collaboration between domain experts and language designers
- Invest in training and skill development for the team
- Consider partnering with language design experts or consultants
8. The Future of Domain-Specific Languages
As we look towards the future, Domain-Specific Languages are poised to play an increasingly important role in software development and domain modeling. Several trends and advancements are shaping the evolution of DSLs:
1. Integration with Artificial Intelligence and Machine Learning
The convergence of DSLs with AI and ML technologies is opening up new possibilities:
- AI-assisted DSL design and optimization
- Machine learning models for improving DSL usability and error detection
- DSLs specifically designed for defining and training ML models
2. Polyglot Programming and Language Interoperability
As software systems become more complex, the ability to seamlessly integrate multiple DSLs and general-purpose languages is becoming crucial:
- Improved tooling for managing multi-language projects
- Standardized interfaces for language interoperability
- DSLs designed to work across different platforms and environments
3. Domain-Specific IDEs and Development Environments
The rise of specialized development environments tailored for specific DSLs:
- Advanced code completion and error detection for DSLs
- Visual programming interfaces for certain types of DSLs
- Integrated debugging and profiling tools specific to each DSL
4. Increased Focus on Domain-Driven Design
As domain-driven design principles gain popularity, DSLs will play a crucial role in bridging the gap between domain experts and software implementation:
- DSLs that directly represent domain models and ubiquitous language
- Tools for automatically generating DSLs from domain models
- Closer integration between DSLs and business process modeling
5. Advancements in Language Workbenches
Continued evolution of language workbenches will make DSL development more accessible and powerful:
- Improved support for collaborative DSL design
- More sophisticated code generation and transformation capabilities
- Better integration with version control and continuous integration systems
6. DSLs for Emerging Technologies
As new technologies emerge, specialized DSLs will be developed to address their unique challenges:
- DSLs for quantum computing
- Specialized languages for IoT and edge computing
- DSLs for augmented and virtual reality development
7. Increased Adoption in Non-Technical Domains
DSLs will continue to expand beyond traditional software development into other fields:
- DSLs for legal contracts and compliance
- Specialized languages for financial modeling and risk assessment
- DSLs for educational content creation and assessment
9. Conclusion
Domain-Specific Languages represent a powerful tool in the modern developer’s toolkit, offering unparalleled expressiveness and efficiency within their targeted domains. By encapsulating domain knowledge and providing tailored syntax, DSLs enable developers and domain experts alike to work at a higher level of abstraction, resulting in more maintainable, readable, and efficient code.
Throughout this comprehensive guide, we’ve explored the fundamental concepts of DSLs, their various types, and the process of designing and implementing them. We’ve seen real-world examples of successful DSLs and discussed the challenges and considerations involved in their development. Looking towards the future, it’s clear that DSLs will continue to evolve and play an increasingly important role in software development, particularly as they integrate with emerging technologies and expand into new domains.
As you embark on your journey with Domain-Specific Languages, remember that the key to success lies in deeply understanding both the problem domain and the principles of language design. Whether you’re creating a small internal DSL to streamline a specific workflow or developing a full-fledged external DSL for a complex domain, the insights and strategies outlined in this guide will serve as a valuable resource.
Embrace the power of Domain-Specific Languages, and unlock new levels of productivity, clarity, and domain alignment in your software projects. The future of programming is domain-specific, and by mastering DSLs, you’re positioning yourself at the forefront of this exciting and transformative trend in software development