Mastering Custom Data Structures: Advanced Techniques for Efficient Programming
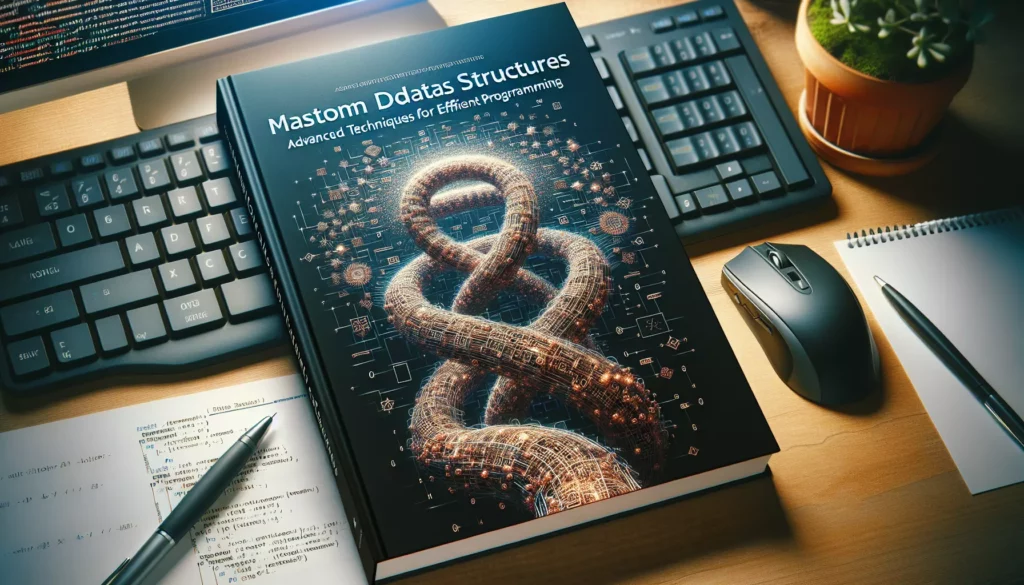
In the world of software development, understanding and implementing custom data structures is a crucial skill that separates novice programmers from seasoned professionals. As we delve into the realm of advanced programming techniques, we’ll explore various methods for creating and optimizing custom data structures that can significantly enhance the performance and flexibility of your code. This comprehensive guide will equip you with the knowledge and tools necessary to design, implement, and utilize custom data structures effectively in your projects.
1. Understanding the Importance of Custom Data Structures
Before we dive into the techniques for implementing custom data structures, it’s essential to understand why they are so valuable in programming. Custom data structures allow developers to:
- Optimize memory usage and performance for specific use cases
- Create more efficient algorithms tailored to particular problem domains
- Implement complex data relationships that may not be easily represented by built-in structures
- Enhance code readability and maintainability by encapsulating related data and operations
By mastering the art of creating custom data structures, you’ll be better equipped to tackle challenging programming problems and design more efficient software solutions.
2. Fundamental Principles of Custom Data Structure Design
When designing custom data structures, it’s crucial to adhere to several fundamental principles:
2.1. Abstraction
Abstraction involves hiding the complex implementation details of a data structure behind a simple and intuitive interface. This principle allows users of your data structure to interact with it without needing to understand its inner workings.
2.2. Encapsulation
Encapsulation is the practice of bundling data and the methods that operate on that data within a single unit. This principle helps in maintaining data integrity and provides a clear separation between the internal implementation and the external interface.
2.3. Modularity
Designing your custom data structure in a modular fashion allows for easier maintenance, testing, and reusability. Each component of the data structure should have a well-defined purpose and interface.
2.4. Efficiency
Custom data structures should be designed with performance in mind. Consider the time and space complexity of operations and optimize accordingly.
3. Techniques for Implementing Custom Data Structures
Now that we’ve covered the fundamental principles, let’s explore some advanced techniques for implementing custom data structures:
3.1. Composition vs. Inheritance
When creating custom data structures, you’ll often need to decide between using composition or inheritance. While inheritance can be useful for creating specialized versions of existing data structures, composition is generally preferred for building entirely new structures.
Example of composition in Python:
class Node:
def __init__(self, value):
self.value = value
self.next = None
class CustomLinkedList:
def __init__(self):
self.head = None
self.tail = None
self.size = 0
def append(self, value):
new_node = Node(value)
if self.head is None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
self.size += 1
In this example, we use composition to create a custom linked list by combining Node objects.
3.2. Generic Programming
Generic programming allows you to create data structures that can work with different data types without sacrificing type safety. This technique is particularly useful when implementing container-like data structures.
Example of generic programming in C++:
template <typename T>
class CustomStack {
private:
std::vector<T> elements;
public:
void push(const T& item) {
elements.push_back(item);
}
T pop() {
if (elements.empty()) {
throw std::out_of_range("Stack is empty");
}
T item = elements.back();
elements.pop_back();
return item;
}
bool isEmpty() const {
return elements.empty();
}
};
// Usage
CustomStack<int> intStack;
CustomStack<std::string> stringStack;
This generic CustomStack class can be used with any data type, providing flexibility and type safety.
3.3. Memory Management Techniques
Efficient memory management is crucial when implementing custom data structures, especially in languages without automatic garbage collection. Consider using techniques such as:
- Custom allocators for fine-grained control over memory allocation
- Memory pools for improved performance in scenarios with frequent allocations and deallocations
- Reference counting or smart pointers for automatic memory management
Example of a simple memory pool in C++:
template <typename T, size_t PoolSize>
class MemoryPool {
private:
char buffer[PoolSize * sizeof(T)];
bool is_allocated[PoolSize];
size_t next_free;
public:
MemoryPool() : next_free(0) {
std::fill(is_allocated, is_allocated + PoolSize, false);
}
T* allocate() {
if (next_free >= PoolSize) {
throw std::bad_alloc();
}
size_t index = next_free;
while (is_allocated[index]) {
index = (index + 1) % PoolSize;
}
is_allocated[index] = true;
next_free = (index + 1) % PoolSize;
return reinterpret_cast<T*>(&buffer[index * sizeof(T)]);
}
void deallocate(T* ptr) {
size_t index = (reinterpret_cast<char*>(ptr) - buffer) / sizeof(T);
is_allocated[index] = false;
}
};
// Usage
MemoryPool<int, 100> intPool;
int* num = intPool.allocate();
*num = 42;
intPool.deallocate(num);
This simple memory pool can be used to efficiently allocate and deallocate objects of a specific type.
3.4. Lazy Initialization and Copy-on-Write
Lazy initialization and copy-on-write are optimization techniques that can significantly improve the performance of custom data structures in certain scenarios.
Lazy initialization involves delaying the creation of an object until it’s actually needed. This can be particularly useful for large or resource-intensive data structures.
Copy-on-write is a technique where multiple references to the same resource are allowed, but a copy is only created when a modification is made. This can be beneficial for large data structures that are frequently read but rarely modified.
Example of lazy initialization in Python:
class LazyArray:
def __init__(self, size):
self.size = size
self._data = None
def _initialize(self):
if self._data is None:
self._data = [0] * self.size
def __getitem__(self, index):
self._initialize()
return self._data[index]
def __setitem__(self, index, value):
self._initialize()
self._data[index] = value
# Usage
lazy_array = LazyArray(1000000) # No memory allocated yet
lazy_array[0] = 42 # Memory is allocated only when accessed
In this example, the large array is only initialized when it’s first accessed, potentially saving memory if the array is never used.
3.5. Thread-Safe Data Structures
When designing custom data structures for multi-threaded environments, it’s crucial to ensure thread safety. This can be achieved through various synchronization mechanisms such as locks, atomic operations, and lock-free algorithms.
Example of a thread-safe queue in C++:
#include <mutex>
#include <queue>
#include <condition_variable>
template <typename T>
class ThreadSafeQueue {
private:
std::queue<T> queue;
mutable std::mutex mutex;
std::condition_variable cond;
public:
void push(T value) {
std::lock_guard<std::mutex> lock(mutex);
queue.push(std::move(value));
cond.notify_one();
}
T pop() {
std::unique_lock<std::mutex> lock(mutex);
cond.wait(lock, [this] { return !queue.empty(); });
T value = std::move(queue.front());
queue.pop();
return value;
}
bool empty() const {
std::lock_guard<std::mutex> lock(mutex);
return queue.empty();
}
};
// Usage
ThreadSafeQueue<int> safeQueue;
safeQueue.push(42);
int value = safeQueue.pop();
This thread-safe queue uses a mutex and a condition variable to ensure safe concurrent access from multiple threads.
4. Advanced Data Structure Implementations
Now that we’ve covered various techniques, let’s explore some advanced custom data structure implementations:
4.1. Trie (Prefix Tree)
A trie is an efficient data structure for storing and retrieving strings, particularly useful for tasks like autocomplete and spell checking.
Example implementation in Python:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
# Usage
trie = Trie()
trie.insert("apple")
print(trie.search("apple")) # True
print(trie.search("app")) # False
print(trie.starts_with("app")) # True
4.2. LRU Cache
An LRU (Least Recently Used) Cache is a data structure that combines a hash map for fast lookups with a doubly linked list for efficient ordering of elements based on their access time.
Example implementation in C++:
#include <unordered_map>
#include <list>
template <typename K, typename V>
class LRUCache {
private:
int capacity;
std::list<std::pair<K, V>> items;
std::unordered_map<K, typename std::list<std::pair<K, V>>::iterator> cache;
public:
LRUCache(int cap) : capacity(cap) {}
V get(K key) {
auto it = cache.find(key);
if (it == cache.end()) {
return V();
}
items.splice(items.begin(), items, it->second);
return it->second->second;
}
void put(K key, V value) {
auto it = cache.find(key);
if (it != cache.end()) {
it->second->second = value;
items.splice(items.begin(), items, it->second);
} else {
if (cache.size() == capacity) {
cache.erase(items.back().first);
items.pop_back();
}
items.push_front({key, value});
cache[key] = items.begin();
}
}
};
// Usage
LRUCache<int, std::string> cache(2);
cache.put(1, "one");
cache.put(2, "two");
std::cout << cache.get(1) << std::endl; // Outputs: one
cache.put(3, "three");
std::cout << cache.get(2) << std::endl; // Outputs: "" (empty string)
4.3. Segment Tree
A segment tree is a tree data structure used for storing information about intervals, or segments. It allows for efficient querying of cumulative information for any segment.
Example implementation in Java:
class SegmentTree {
private int[] tree;
private int n;
public SegmentTree(int[] arr) {
n = arr.length;
tree = new int[4 * n];
buildTree(arr, 0, 0, n - 1);
}
private void buildTree(int[] arr, int node, int start, int end) {
if (start == end) {
tree[node] = arr[start];
} else {
int mid = (start + end) / 2;
buildTree(arr, 2 * node + 1, start, mid);
buildTree(arr, 2 * node + 2, mid + 1, end);
tree[node] = tree[2 * node + 1] + tree[2 * node + 2];
}
}
public void update(int index, int value) {
updateTree(0, 0, n - 1, index, value);
}
private void updateTree(int node, int start, int end, int index, int value) {
if (start == end) {
tree[node] = value;
} else {
int mid = (start + end) / 2;
if (start <= index && index <= mid) {
updateTree(2 * node + 1, start, mid, index, value);
} else {
updateTree(2 * node + 2, mid + 1, end, index, value);
}
tree[node] = tree[2 * node + 1] + tree[2 * node + 2];
}
}
public int querySum(int left, int right) {
return queryTreeSum(0, 0, n - 1, left, right);
}
private int queryTreeSum(int node, int start, int end, int left, int right) {
if (right < start || end < left) {
return 0;
}
if (left <= start && end <= right) {
return tree[node];
}
int mid = (start + end) / 2;
int leftSum = queryTreeSum(2 * node + 1, start, mid, left, right);
int rightSum = queryTreeSum(2 * node + 2, mid + 1, end, left, right);
return leftSum + rightSum;
}
}
// Usage
int[] arr = {1, 3, 5, 7, 9, 11};
SegmentTree segTree = new SegmentTree(arr);
System.out.println(segTree.querySum(1, 3)); // Outputs: 15
segTree.update(2, 10);
System.out.println(segTree.querySum(1, 3)); // Outputs: 20
5. Best Practices for Custom Data Structure Implementation
When implementing custom data structures, it’s important to follow these best practices:
5.1. Documentation
Thoroughly document your custom data structure, including its purpose, usage, time and space complexity of operations, and any assumptions or limitations.
5.2. Testing
Implement comprehensive unit tests to verify the correctness of your data structure under various scenarios, including edge cases.
5.3. Performance Benchmarking
Conduct performance benchmarks to ensure your custom data structure meets the required efficiency standards and compare it with alternative implementations.
5.4. Code Review
Have your implementation reviewed by peers to catch potential issues and improve the overall design and implementation.
5.5. Iterative Refinement
Be prepared to refine and optimize your data structure based on real-world usage patterns and feedback.
6. Conclusion
Mastering the techniques for implementing custom data structures is a valuable skill that can significantly enhance your programming abilities. By understanding the fundamental principles, applying advanced techniques, and following best practices, you can create efficient and robust custom data structures tailored to your specific needs.
As you continue to develop your skills in this area, remember that the key to success lies in practice and continuous learning. Experiment with different implementations, analyze their performance characteristics, and strive to understand the trade-offs involved in various design decisions.
By incorporating these advanced techniques into your programming toolkit, you’ll be well-equipped to tackle complex problems and optimize your code for maximum efficiency. Whether you’re preparing for technical interviews at top tech companies or working on challenging software projects, your expertise in custom data structures will prove invaluable in your journey as a skilled programmer.