Mastering Conway’s Game of Life: A Fascinating Journey into Cellular Automata
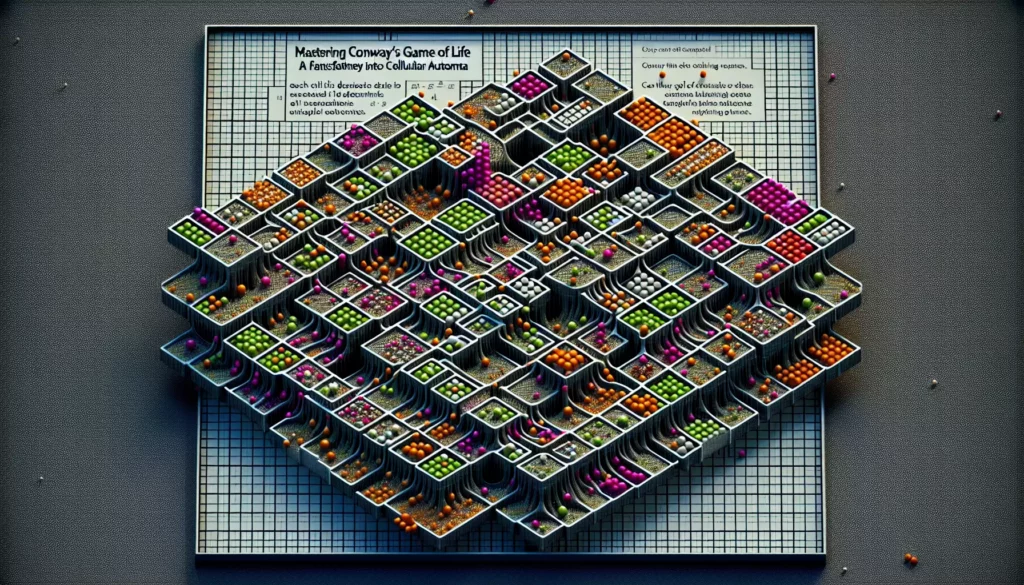
Welcome to another exciting exploration of computer science and programming concepts here at AlgoCademy! Today, we’re diving deep into one of the most intriguing and influential simulations in the world of computing: Conway’s Game of Life. This seemingly simple cellular automaton has captivated mathematicians, computer scientists, and enthusiasts for decades, and understanding it can significantly enhance your algorithmic thinking and problem-solving skills.
What is Conway’s Game of Life?
Conway’s Game of Life, often simply referred to as “Life,” is a cellular automaton devised by the British mathematician John Horton Conway in 1970. Despite its name, it’s not a game in the traditional sense. Instead, it’s a zero-player game, meaning its evolution is determined by its initial state, with no further input from humans.
The “game” takes place on a two-dimensional grid of cells, each of which can be in one of two states: alive or dead. The grid evolves in discrete time steps, with the state of each cell in the next generation determined by a set of simple rules based on the states of its eight neighboring cells.
The Rules of Life
The beauty of Conway’s Game of Life lies in its simplicity. The entire system is governed by just four rules:
- Any live cell with fewer than two live neighbors dies, as if by underpopulation.
- Any live cell with two or three live neighbors lives on to the next generation.
- Any live cell with more than three live neighbors dies, as if by overpopulation.
- Any dead cell with exactly three live neighbors becomes a live cell, as if by reproduction.
These rules are applied simultaneously to every cell in the grid, creating the next generation. The process then repeats, potentially ad infinitum.
Implementing the Game of Life
Now that we understand the rules, let’s implement Conway’s Game of Life in Python. We’ll start with a basic implementation and then explore ways to optimize and enhance it.
Basic Implementation
Here’s a simple implementation of the Game of Life:
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
def create_grid(width, height):
return np.random.choice([0, 1], size=(height, width))
def update(frame_num, img, grid, width, height):
new_grid = grid.copy()
for i in range(height):
for j in range(width):
total = int((grid[i, (j-1)%width] + grid[i, (j+1)%width] +
grid[(i-1)%height, j] + grid[(i+1)%height, j] +
grid[(i-1)%height, (j-1)%width] + grid[(i-1)%height, (j+1)%width] +
grid[(i+1)%height, (j-1)%width] + grid[(i+1)%height, (j+1)%width]))
if grid[i, j] == 1:
if (total < 2) or (total > 3):
new_grid[i, j] = 0
else:
if total == 3:
new_grid[i, j] = 1
img.set_data(new_grid)
grid[:] = new_grid[:]
return img,
width, height = 100, 100
grid = create_grid(width, height)
fig, ax = plt.subplots()
img = ax.imshow(grid, interpolation='nearest')
ani = FuncAnimation(fig, update, fargs=(img, grid, width, height),
frames=200, interval=50, save_count=50)
plt.show()
This implementation uses NumPy for efficient array operations and Matplotlib for visualization. Let’s break down the key components:
create_grid()
: Initializes a random grid of cells.update()
: Applies the rules of the Game of Life to create the next generation.FuncAnimation
: Creates an animation of the evolving grid.
Optimization Techniques
While the above implementation works, it’s not very efficient for large grids or long simulations. Here are some ways we can optimize it:
1. Vectorization
Instead of using nested loops, we can use NumPy’s vectorized operations to speed up the computation:
def update_vectorized(frame_num, img, grid):
N = convolve2d(grid, np.ones((3, 3)), mode='same', boundary='wrap') - grid
new_grid = ((grid == 1) & (N > 1) & (N < 4)) | ((grid == 0) & (N == 3))
img.set_data(new_grid)
grid[:] = new_grid[:]
return img,
This version uses convolve2d
from SciPy to count neighbors efficiently.
2. Sparse Representation
For patterns with relatively few live cells, we can use a sparse representation to save memory and computation time:
from scipy.sparse import csr_matrix
def sparse_to_dense(sparse_grid, shape):
return sparse_grid.toarray()
def update_sparse(sparse_grid, shape):
grid = sparse_to_dense(sparse_grid, shape)
N = convolve2d(grid, np.ones((3, 3)), mode='same', boundary='wrap') - grid
new_grid = ((grid == 1) & (N > 1) & (N < 4)) | ((grid == 0) & (N == 3))
return csr_matrix(new_grid)
3. GPU Acceleration
For even larger grids, we can leverage GPU acceleration using libraries like CuPy:
import cupy as cp
def update_gpu(grid):
N = cp.zeros_like(grid)
for i in range(-1, 2):
for j in range(-1, 2):
if i == 0 and j == 0:
continue
N += cp.roll(grid, (i, j), axis=(0, 1))
return ((grid == 1) & (N > 1) & (N < 4)) | ((grid == 0) & (N == 3))
Interesting Patterns in the Game of Life
One of the most fascinating aspects of Conway’s Game of Life is the wide variety of patterns that can emerge from simple initial configurations. Let’s explore some of the most famous and interesting patterns:
Still Lifes
Still lifes are patterns that do not change from one generation to the next. Some common still lifes include:
- Block: A 2×2 square of live cells
- Beehive: A hexagonal arrangement of six cells
- Loaf: A pattern resembling a loaf of bread
Oscillators
Oscillators are patterns that return to their initial state after a fixed number of generations. Some examples include:
- Blinker: A row of three cells that alternates between horizontal and vertical
- Toad: A pattern that oscillates between two states with a period of 2
- Pulsar: A complex pattern with a period of 3
Spaceships
Spaceships are patterns that move across the grid. The most famous spaceship is the “Glider,” a small pattern that moves diagonally across the grid. Other spaceships include:
- Lightweight spaceship (LWSS)
- Middleweight spaceship (MWSS)
- Heavyweight spaceship (HWSS)
Guns and Puffers
Guns are patterns that periodically emit spaceships. The most famous gun is the “Gosper glider gun,” which emits a new glider every 30 generations. Puffers are similar to guns but emit more complex patterns.
Applications of Conway’s Game of Life
While Conway’s Game of Life might seem like a simple curiosity, it has found applications in various fields:
1. Computer Science and Artificial Intelligence
The Game of Life has been used to study emergent behavior in complex systems. It’s a prime example of how simple rules can lead to complex, unpredictable outcomes, which is relevant to the study of artificial life and cellular automata.
2. Biology and Evolution
Some researchers have used the Game of Life as a simplified model for studying population dynamics and evolution. While it’s a vast simplification of real biological systems, it can provide insights into how simple rules can lead to complex behaviors in populations.
3. Physics and Chaos Theory
The Game of Life exhibits chaotic behavior, making it interesting for studying concepts in chaos theory and non-linear dynamics. It’s been used to explore ideas like self-organization and pattern formation in physical systems.
4. Art and Design
The mesmerizing patterns generated by the Game of Life have inspired artists and designers. It’s been used to create generative art and even in some architectural designs.
5. Cryptography
Some researchers have explored using cellular automata like the Game of Life in cryptographic applications, although this remains largely theoretical.
Challenges and Exercises
To deepen your understanding of Conway’s Game of Life and improve your programming skills, try these challenges:
- Implement the Game of Life using a different programming language or paradigm (e.g., functional programming).
- Create a user interface that allows users to draw initial patterns and observe their evolution.
- Implement a feature to detect and classify common patterns like still lifes, oscillators, and spaceships.
- Modify the rules of the game and observe how it affects the evolution of patterns. Can you create your own interesting cellular automaton?
- Implement a “Garden of Eden” finder – a pattern that has no predecessor in the Game of Life.
Conclusion
Conway’s Game of Life is a fascinating example of how simple rules can lead to complex, emergent behavior. It’s not just a curiosity or a game, but a powerful tool for exploring concepts in computer science, mathematics, and even philosophy. By implementing and experimenting with the Game of Life, you can improve your programming skills, deepen your understanding of algorithms and data structures, and gain insights into the nature of complexity itself.
As you continue your journey in programming and computer science, remember that seemingly simple systems like the Game of Life can offer profound insights. They challenge us to think about the nature of computation, the emergence of complexity, and the fundamental principles that govern both artificial and natural systems.
We hope this deep dive into Conway’s Game of Life has inspired you to explore further and perhaps even create your own cellular automata. Remember, the skills you develop in implementing and optimizing simulations like this are directly applicable to many areas of software development and computer science.
Keep experimenting, keep learning, and most importantly, keep having fun with code!